python rgb 图像_在Python中显示RGB图像的不同平面
python rgb 图像
A colored image can be represented as a 3 order matrix. The first order is for the rows, the second order is for the columns and the third order is for specifying the color of the corresponding pixel. Here, we use the BGR color format(because OpenCV python library works on BGR format, not on RGB), so the third order will take 3 values of Blue, Green, and Red respectively.
彩色图像可以表示为3阶矩阵。 第一个顺序用于行,第二个顺序用于列,第三个顺序用于指定相应像素的颜色。 在这里,我们使用BGR颜色格式(因为OpenCV python库适用于BGR格式,而不适用于RGB),因此三阶分别采用蓝色,绿色和红色这3个值。
Colour planes of BGR image:
BGR图像的彩色平面:
Consider a BGR image array I then,
考虑一个BGR图像阵列我然后,
I[:, :, 0] represents the Blue colour plane of the BGR image
I [:,:,0]表示BGR图像的蓝色平面
I[:, :, 1] represents the Green colour plane of the BGR image
I [:,:,1]表示BGR图像的绿色平面
I[:, :, 2] represents the Red colour plane of the BGR image
I [:,:,2]表示BGR图像的红色平面
In this program, we will be using two functions of OpenCV-python (cv2) module. let's see their syntax and descriptions first :
在此程序中,我们将使用OpenCV-python(cv2)模块的两个功能。 我们先来看一下它们的语法和描述:
1) imread():
It takes an absolute path/relative path of your image file as an argument and returns its corresponding image matrix.
1)imread():
它以图像文件的绝对路径/相对路径作为参数,并返回其对应的图像矩阵。
2) imshow():
It takes window name and image matrix as an argument in order to display an image in a display window with a specified window name.
2)imshow():
它以窗口名称和图像矩阵为参数,以便在具有指定窗口名称的显示窗口中显示图像。
3) shape: This is the attribute of an image matrix which return shape of an image i.e. consisting of number of rows ,columns and number of planes.
3)形状:这是图像矩阵的属性,其返回图像的形状,即由行数,列数和平面数组成。
Python程序可显示RGB图像的不同平面 (Python program to display different planes of an RGB image)
# open-cv library is installed as cv2 in python
# import cv2 library into this program
import cv2
# import numpy library as np
import numpy as np
# read an image using imread() function of cv2
# we have to pass only the path of the image
img = cv2.imread(r'C:/Users/user/Desktop/pic4.jpg')
# displaying the image using imshow() function of cv2
# In this : 1st argument is name of the frame
# 2nd argument is the image matrix
cv2.imshow('original image',img)
# shape attribute of an image matrix gives the dimensions
row,col,plane = img.shape
# here image is of class 'uint8', the range of values
# that each colour component can have is [0 - 255]
# create a zero matrix of order same as
# original image matrix order of same dimension
temp = np.zeros((row,col,plane),np.uint8)
# store blue plane contents or data of image matrix
# to the corresponding plane(blue) of temp matrix
temp[:,:,0] = img[:,:,0]
# displaying the Blue plane image
cv2.imshow('Blue plane image',temp)
# again take a zero matrix of image matrix shape
temp = np.zeros((row,col,plane),np.uint8)
# store green plane contents or data of image matrix
# to the corresponding plane(green) of temp matrix
temp[:,:,1] = img[:,:,1]
# displaying the Green plane image
cv2.imshow('Green plane image',temp)
# again take a zero matrix of image matrix shape
temp = np.zeros((row,col,plane),np.uint8)
# store red plane contents or data of image matrix
# to the corresponding plane(red) of temp matrix
temp[:,:,2] = img[:,:,2]
# displaying the Red plane image
cv2.imshow('Red plane image',temp)
Output
输出量
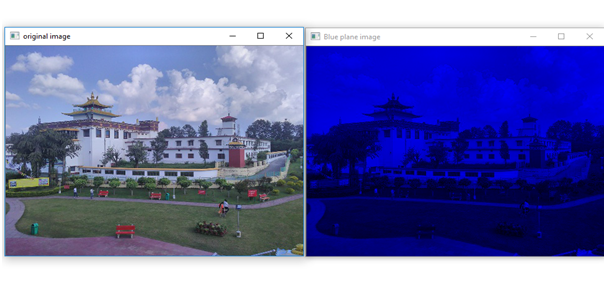
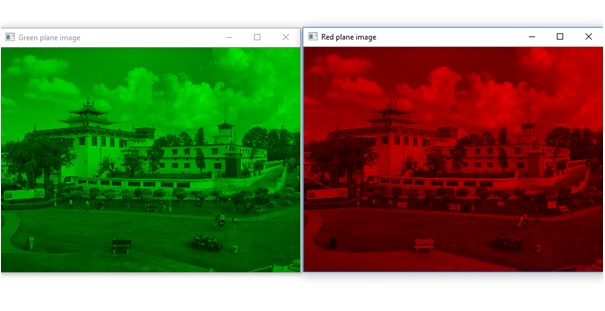
翻译自: https://www.includehelp.com/python/show-different-planes-of-an-rgb-image.aspx
python rgb 图像
python rgb 图像_在Python中显示RGB图像的不同平面相关推荐
- python 时间序列预测_使用Python进行动手时间序列预测
python 时间序列预测 Time series analysis is the endeavor of extracting meaningful summary and statistical ...
- python 概率分布模型_使用python的概率模型进行公司估值
python 概率分布模型 Note from Towards Data Science's editors: While we allow independent authors to publis ...
- html在浏览器显示图片,html - 在所有Web浏览器中显示TIFF图像
html - 在所有Web浏览器中显示TIFF图像 如何在HTML页面中处理TIFF文件? 我想在HTML页面中显示TIFF文件. 我尝试使用嵌入式标签,对象ID,img等.但我无法在HTML页面中显 ...
- python opencv创建图像_使用Python中OpenCV库创建一幅图片的RGB通道图片
我们知道,在使用PhotoShop进行图片的抠取.创建和存储选区.存储图像的色彩资料等复杂操作时,经常会用到一个功能,那就是"RGB"通道,它能从三原色角度对一幅图片进行精准处理. ...
- python rgb 图像_在Python中查找RGB图像的互补图像
python rgb 图像 Complementary image is a transformed image such that it consists of complementary colo ...
- html 展示 python结果_在HTML中显示Python值
如何在html中显示python变量的值(在这种情况下,它是我的Entity类的键)? from google.appengine.ext import db class Entity(db.Expa ...
- python画图模糊_使用python matplotlib 画图导入到word中如何保证分辨率
在写论文时,如果是菜鸟级别,可能不会花太多时间去学latex,直接用word去写,但是这有一个问题,当我们用其他工具画完实验彩色图时,放到word中会有比较模糊,这有两个原因导致的. 原因一:图片导入 ...
- python反向缩进_在Pycharm中对代码进行注释和缩进的方法详解
在Pycharm中对代码进行注释和缩进的方法详解 一.注释 1. #单行注释 2. """ 多行注释 """ 3. pycharm多行注释快 ...
- python opencv图像匹配_关于python:OpenCV功能匹配多个图像
如何使用FLANN优化许多图片的SIFT功能匹配? 我有一个从Python OpenCV文档中获取的工作示例.然而,这是将一个图像与另一个图像进行比较而且速度很慢.我需要它来搜索一系列图像(几千个)中 ...
最新文章
- 决策树算法原理(ID3,C4.5)
- 提升购物体验,跨境电商如何做企业管理?
- 馒头,国庆节快乐啊!!~~~!
- OpenCV删除面积小的区域 实现图像二值化分割 标记连通区域
- CSS 学习路线(二)选择器
- 30个免费资源:涵盖机器学习、深度学习、NLP及自动驾驶
- 终于明白上一篇的一顿误操作是什么了,是$,不是S !!!!!
- Linux下数据库mariaDB的管理
- 按平均成绩排行c语言文件操作,学生成绩管理系统(c语言结构体以及文件操作)实验报告精选.doc...
- Spark Mllib里数据集如何取前M行(图文详解)
- 正则表达式用法及实例
- 我是如何零基础开始能写爬虫的
- inno setup 卸载程序
- GET请求里的body问题
- opencv获取摄像头的个数及名字
- 通过分辨率区分iPhone型号(更新至13系列)
- RPA机器人流程自动化(Robotic process automation)
- css美化radio
- 函授计算机应用基础答案,计算机应用基础函授本科考试题库
- docker containerd.io、docker-ce、docker-ce-cli的区别(docker版本安装docker安装方法)(Docker CE和Docker EE,docker.io)