Castle.ActiveRecord 学与练[3]
Castle.ActiveRecord引入了特性映射这个概念,使用Attribute来代替.hbm.xml文件,从而更加清晰的呈现出了实体类跟数据库表之间的关系,使持久化的数据操作更加简单,易用.
下面就让咱们一起来通过一个完整的实例了解一下Castle.ActiveRecord的Attribute.
我们先来创建三个数据库表,接下来的文章练习都是围绕着这三个表来操作,便于演示一对多,多对一及相应的级联更新,删除等操作.
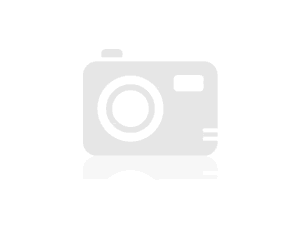
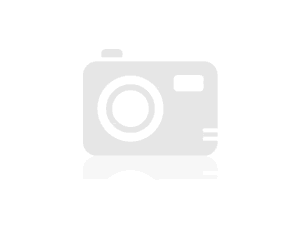
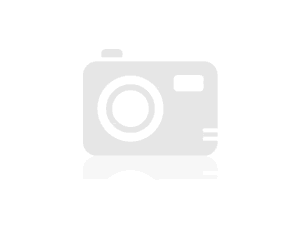
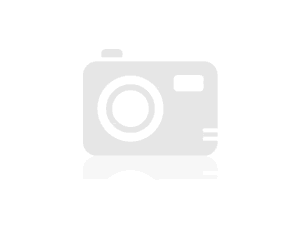
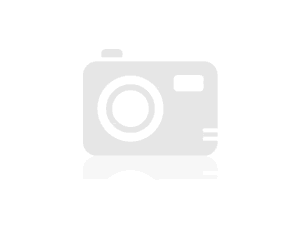
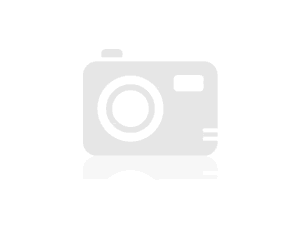
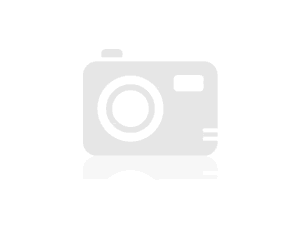
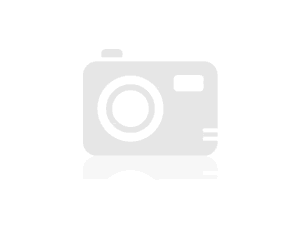
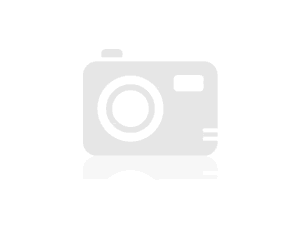
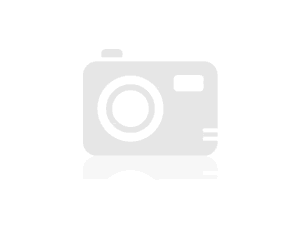
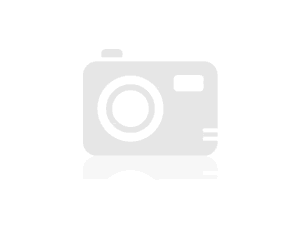
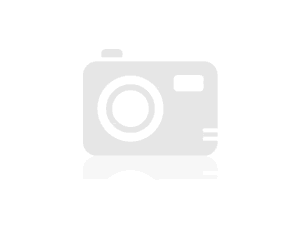
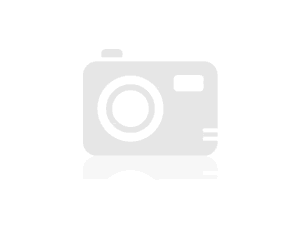
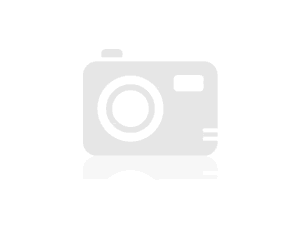
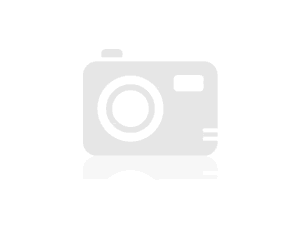
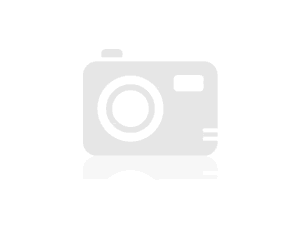
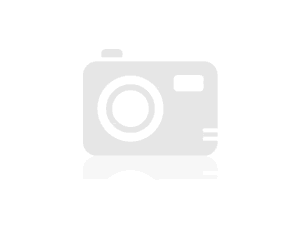
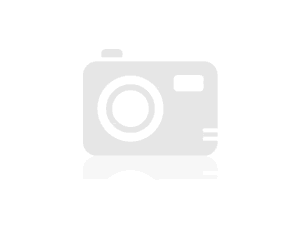
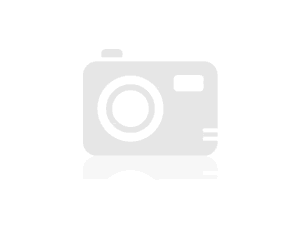
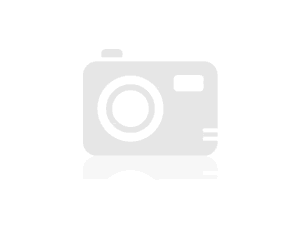
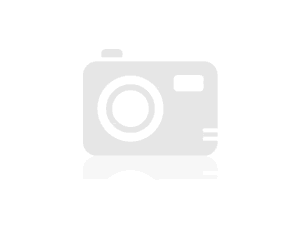
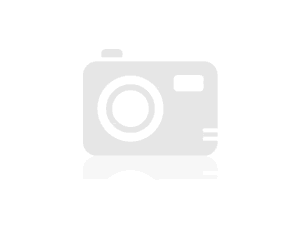
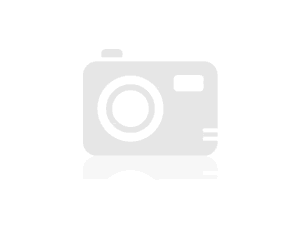
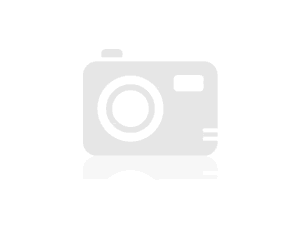
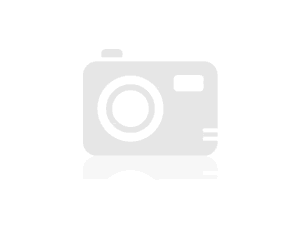
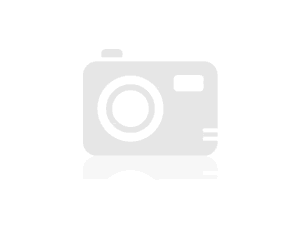
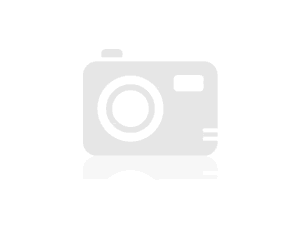
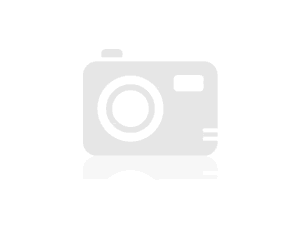
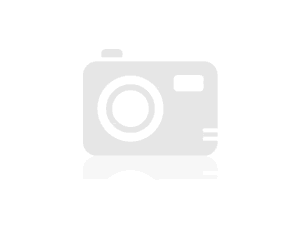
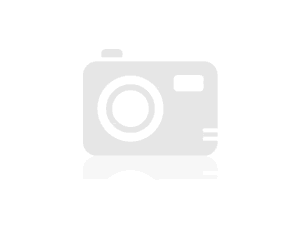
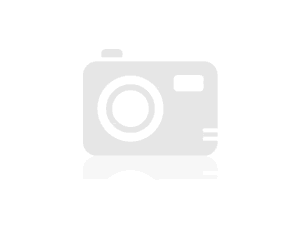
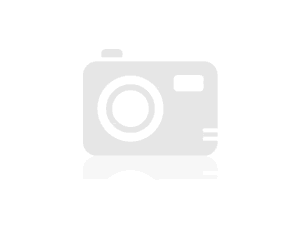
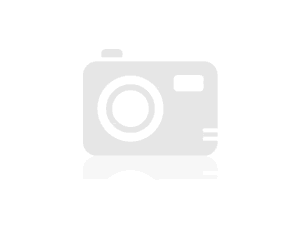
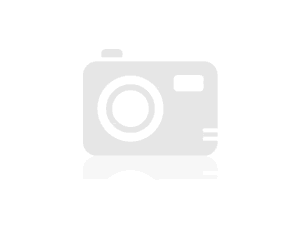
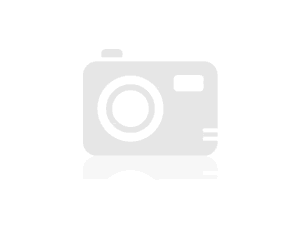
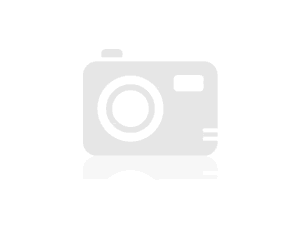
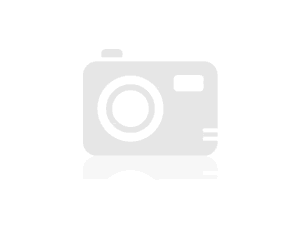
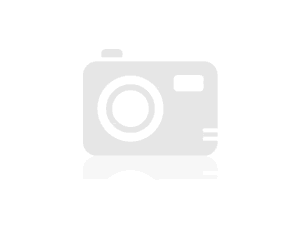
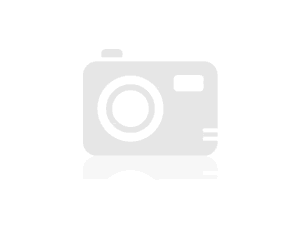
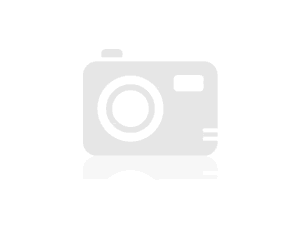
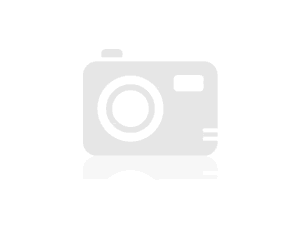
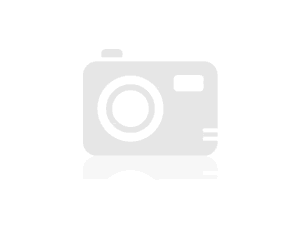
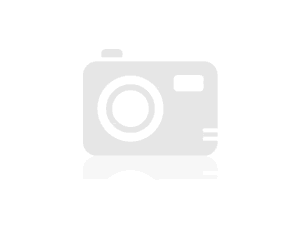
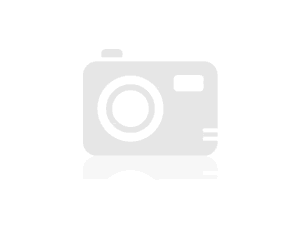
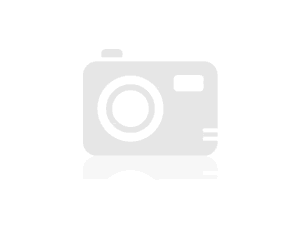
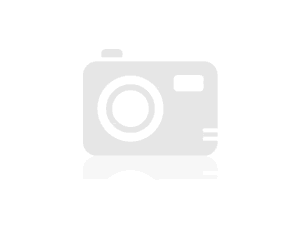
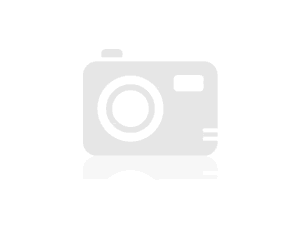
第一部分:特性介绍
ActiveRecordAttribute:
--------------------------------------------------------------------------------------------------------------------------------------
Name | Description | |
---|---|---|
BatchSize |
From NHibernate documentation: Specify a "batch size" for fetching instances of this class by identifier.
|
|
DiscriminatorColumn |
Gets or sets the Discriminator column for a table inheritance modeling
为表设置一个识别器字段 |
|
DiscriminatorType |
Gets or sets the column type (like string or integer) for the discriminator column
为识别器字段设置一个字段类型(例如:String或者Int) |
|
DiscriminatorValue |
Gets or sets the value that represents the target class on the discriminator column
为识别器字段设置一个可以描述目标类的值 |
|
DynamicInsert |
From NHibernate documentation: Specifies that INSERT SQL should be generated at runtime and contain only the columns whose values are not null.
|
|
DynamicUpdate |
From NHibernate documentation: Specifies that UPDATE SQL should be generated at runtime and contain only those columns whose values have changed.
|
|
Lazy |
Enable lazy loading for the type
打开延迟加载 |
|
LazySpecified |
Gets a value indicating whether explicit lazy behavior was specified. If explicit lazy behavior was not specified, it goes to the configuration to decide if the type should be lazy or not.
|
|
Locking |
From NHibernate documentation: Determines the optimistic locking strategy.
|
|
Mutable |
From NHibernate documentation: Specifies that instances of the class are (not) mutable.
|
|
Persister |
From NHibernate documentation: Specifies a custom IEntityPersister.
|
|
Polymorphism |
From NHibernate documentation: Determines whether implicit or explicit query polymorphism is used.
|
|
Proxy |
Associates a proxy type with the target type
|
|
Schema |
Gets or sets the schema name associated with the type
设置概要的信息 |
|
SelectBeforeUpdate |
From NHibernate documentation: Specifies that NHibernate should never perform an SQL UPDATE unless it is certain that an object is actually modified. In certain cases (actually, only when a transient object has been associated with a new session using update()), this means that NHibernate will perform an extra SQL SELECT to determine if an UPDATE is actually required.
|
|
Table |
Gets or sets the table name associated with the type
表名,类名与表名相同可为空 |
|
UseAutoImport |
From NHibernate documentation: The auto-import attribute lets us use unqualified class names in the query language, by default. The assembly and namespace attributes specify the assembly where persistent classes are located and the namespace they are declared in.
|
|
Where |
SQL condition to retrieve objects
指定一个附加SQL的WHERE子句 |
--------------------------------------------------------------------------------------------------------------------------------------
PrimaryKeyAttribute:
--------------------------------------------------------------------------------------------------------------------------------------
Name | Description | |
---|---|---|
Column |
Gets or sets the column name
设置字段名 |
|
ColumnType |
Gets or sets the type of the column.
设置字段类型 |
|
CustomGenerator |
Gets or sets the custom generator. The generator must implement IIdentifierGenerator
设置一个自定义的生成器.这个生成器必要实现IIdentifierGenerator接口 |
|
Generator |
Gets or sets the generator.
设置一个生成器,是一个.NET类的名字,用来为该持久化类的实例生成惟一的标识. |
|
Length |
Gets or sets the length of values in the column
设置字段长度 |
|
Params |
Comma separated value of parameters to the generator
用parameters来为生成器配置参数或者初始化参数. |
|
SequenceName |
Gets or sets the name of the sequence.
当指定主键的生成方式为Sequence时,序列的名称 当指定主键的生成方式为Sequence时,Sequence的名称. |
|
UnsavedValue |
Gets or sets the unsaved value.
设置该实例未保存时的值 |
--------------------------------------------------------------------------------------------------------------------------------------
PrimaryKeyType
--------------------------------------------------------------------------------------------------------------------------------------
Name | Description | |
---|---|---|
Identity |
Use Identity column (auto number) Note: This force an immediate call to the DB when Create() is called
对DB2,MySQL, MS SQL Server, Sybase和HypersonicSQL的内置标识字段提供支持,生成自增的整型 当Create() 方法执行时,便会触发数据库表自增类型字段自增. |
|
Sequence |
Use a sequence
序列 |
|
HiLo |
Use the HiLo algorithm to get the next value
高低位,使用一个高/低位算法来高效的生成Int64, Int32 或者 Int16类型的标识符。 |
|
SeqHiLo |
Use a sequence and a HiLo algorithm - better performance on Oracle
使用序列的高低位,使用一个高/低位算法来高效的生成Int64, Int32 或者 Int16类型的标识符,给定一个数据库序列(sequence)的名字。 |
|
UuidHex |
Use the hex representation of a unique identifier
用一个System.Guid和它的ToString(string format)方法生成字符串类型的标识符。 |
|
UuidString |
Use the string representation of a unique identifier
用一个新的System.Guid产生一个byte[] ,把它转换成字符串。 |
|
Guid |
Generate a Guid for the primary key Note: You should prefer using GuidComb over this value.
用一个新的System.Guid 作为标识符。 |
|
GuidComb |
Generate a Guid in sequence, so it will have better insert performance in the DB.
用Jimmy Nilsso的一个算法产生一个新的System.Guid。 |
|
Native |
Use an identity or sequence if supported by the database, otherwise, use the HiLo algorithm
根据底层数据库的能力选择 identity, sequence 或者 hilo中的一个。默认值。 |
|
Assigned |
The primary key value is always assigned. Note: using this you will lose the ability to call Save(), and will need to call Create() or Update() explicitly.
让应用程序在自己为对象分配一个标示符。 |
|
Foreign |
This is a foreign key to another table
外键 |
|
Counter |
Returns a Int64 constructed from the system time and a counter value.
返回一个INT64类型的系统时间和一个记数器值. |
|
Increment |
Returns a Int64, constructed by counting from the maximum primary key value at startup.
主键最大值加1 |
|
Custom |
A custom generator will be provided. See CustomGenerator
|
--------------------------------------------------------------------------------------------------------------------------------------
PropertyAttribute:
--------------------------------------------------------------------------------------------------------------------------------------
Name | Description | |
---|---|---|
Check |
From NHibernate documentation: create an SQL check constraint on either column or table
约束 |
|
Column |
Gets or sets the column name
设置字段名 |
|
ColumnType |
Gets or sets the type of the column.
设置字段类型 |
|
Formula |
Gets or sets the formula used to calculate this property
一个SQL表达式,定义了这个计算(computed) 属性的值。计算属性没有和它对应的数据库字段。 |
|
Index |
From NHibernate documentation: specifies the name of a (multi-column) index
|
|
Insert |
Set to false to ignore this property when inserting entities of this ActiveRecord class.
表明在用于INSERT的SQL语句中是否包含这个字段。默认为true |
|
Length |
Gets or sets the length of the property (for strings - nvarchar(50) )
字段长度 |
|
NotNull |
Gets or sets a value indicating whether this property allow null.
是否允许为空 |
|
SqlType |
From NHibernate documentation: overrides the default column type
重写默认的字段类型. |
|
Unique |
Gets or sets a value indicating whether this PropertyAttribute is unique.
是否允许重复. |
|
UniqueKey |
From NHibernate documentation: A unique-key attribute can be used to group columns in a single unit key constraint.
|
|
Update |
Set to false to ignore this property when updating entities of this ActiveRecord class.
表明在用于UPDATE的SQL语句中是否包含这个字段。默认为true |
--------------------------------------------------------------------------------------------------------------------------------------
第二部分:源码讲解
ActiveRecordAttribute:
对于ActiveRecordAttribute来说,其中比较重要的参数就是Table了,用它来确定这个实体指向哪个数据库表.不填表示类实体名与数据库表名相同.
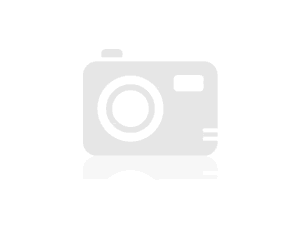
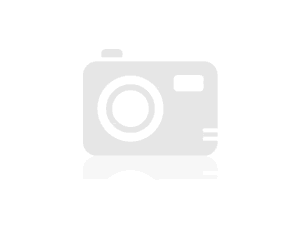
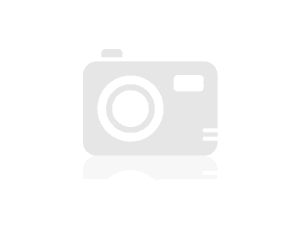
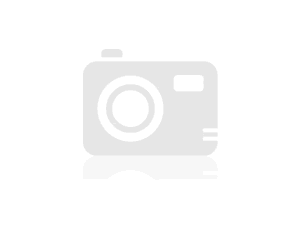


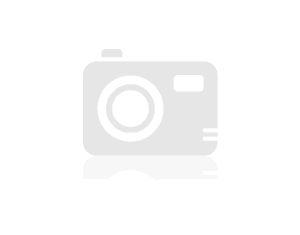
PrimaryKeyAttribute:
对于PrimaryKeyAttribute来说,顾名思义此特性是为了设置主键而设计的,其中PrimaryKeyType为大家提供了多种类型可以应付各种情况,详见上面表格.
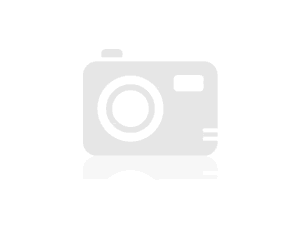
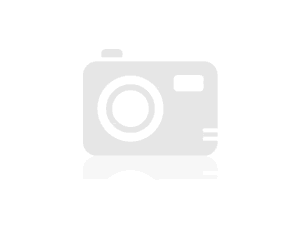
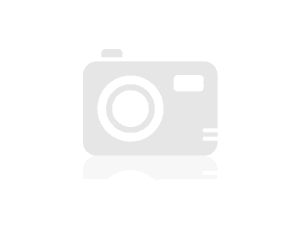
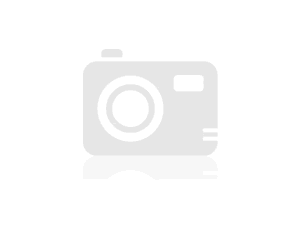


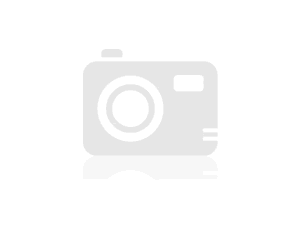


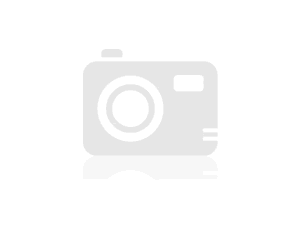

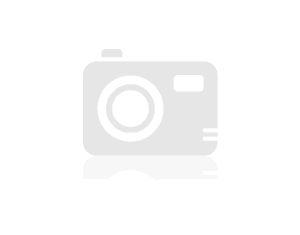
PropertyAttribute:
对于PropertyAttribute来说Column参数相对重要,用它来确定属性对应数据库表中哪个字段,如果为空则字段名与属性名相同.
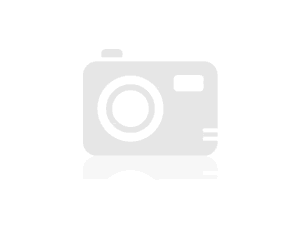
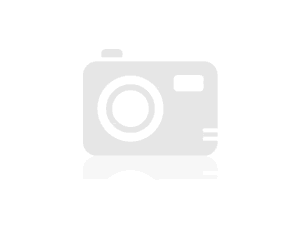
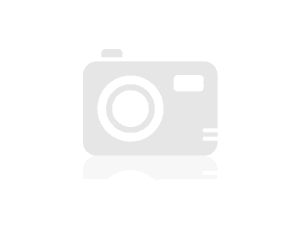
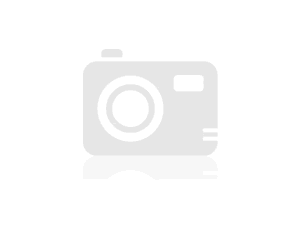


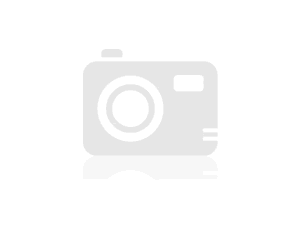


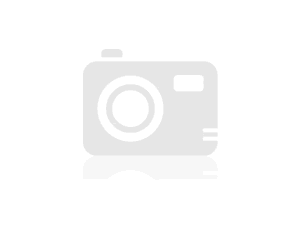

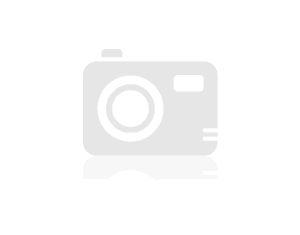
实体类源码下载
最近真是太忙了,SAP一直在我们周围转啊转,终于到了要上线的地步了.
写了这一些,先给大家看看吧,下一篇会在数据的操作上向大家演示Castle.ActiveRecord的奇妙之处,以完成Castle的Castle.ActiveRecord部分,敬请关注.
谢谢Terrylee老师的教程对我的帮助.
QQ:22566547;
MSN:LGUYSS@GMAIL.COM;
SITE:WWW.MOBILEBETA.NET
转载于:https://www.cnblogs.com/lguyss/archive/2008/04/08/1135962.html
Castle.ActiveRecord 学与练[3]相关推荐
- Castle ActiveRecord(一)概述
一.ActiveRecord与Castle ActiveRecord ActiveRecord是<Patterns of Enterprise Application Architecture& ...
- Castle ActiveRecord 泛型应用
Castle ActiveRecord在.Net2.0下支持泛型,这极大的方便了我们创建强类型集合以及对对象的强类型操作.本文引用了Castle站点上泛型的例子来详细介绍如何应用泛型. 另外你需要在这 ...
- Castle.ActiveRecord的嵌套事务处理
嵌套的情况下,怎么处理Castle.ActiveRecord的事务? 今天试了一下,原来还是很简单的,只需要使用Castle.ActiveRecord.TransactionMode.Inherits ...
- Castle ActiveRecord学习实践(1):快速入门指南
摘要:最近几天有时间看了一下Castle,原来它的功能是如此的强大,从数据访问框架到IOC容器,再到WEB框架,基本包括了整个开发过程中的所有东西,看来得好好学习研究一下了,并且打算把自己学习过程的一 ...
- Castle.ActiveRecord的ProxyFactory配置
前后差不多两年没使用过Castle.ActiveRecord做ORM了,也近两年没有关注Castle的版本变化了,最近关注了发现很多地方都改变了.今天在一个现有的小项目中尝试添加Castle.Acti ...
- 使用 Castle ActiveRecord
使用 Castle ActiveRecord: 由 database (测试于SQL 2005) 直接使用 AR 的 Generator 产生 mapping class (C#/BV.Net) 法: ...
- castle activerecord mysql_Castle ActiveRecord配置中需要注意的地方
关于Castle 的开发可参考李会军老师的Castle 开发系列文章,里面有关于ActiveRecord学习实践系列和Castle IOC容器系列两个部分,是比较好的教程. 这里主要说明在Castle ...
- Castle ActiveRecord学习实践(2):构建配置信息
摘要:ActiveRecord在底层封装了NHibernate,在框架启动时需要指定相关的配置信息,那么我们需要配置些什么?又该如何去配置呢?本文将会介绍在ActiveRecord中构建配置信息. 主 ...
- Castle ActiveRecord学习实践(8)HQL查询
本篇来了解下Castle ActiveRecord hql 查询语句. 博客园中讲解Castle ActiveRecord 的文章已经很多了,博主就不自己写了.转载一篇TerryLee大大的文章. 摘 ...
最新文章
- CRichEdit小记
- PHP无法编译undefined reference to `libiconv_open
- Xamarin.Forms教程开发的Xcode的下载安装
- viewDidUnload 和 dealloc 的区别
- Xgboost调参小结
- 阿里巴巴云原生的 2020,注定不凡的一年
- 集成学习(期末复习)
- 百万局对战教AI做人,技术解读FPS游戏中AI如何拟人化
- 在opencv3中实现机器学习之:利用逻辑斯谛回归(logistic regression)分类
- 这场直播,我们把 Apache 顶级项目盛会搬来了!
- import sys是什么意思_学了半天,import 到底在干啥?
- 基于Matlab使用激光雷达从点云到跟踪列表跟踪车辆仿真(附源码)
- 全面讲解 Handler机制原理解析 (小白必看)
- zigbee 协调器与终端通信问题
- 使用List和Map遇到得空指针异常
- CLC龍链:致力于打造基于区快链技术的全球跨境支付生态系统
- SlicePlane的Heading角度与Math.atan2(y,x)的对应转换关系
- Acer 笔记本双硬盘安装Ubuntu18.04.4+Win10双系统
- 手机拍照技巧(一:校园拍摄)
- 回溯法-子集树排序树满m叉树
热门文章
- 景观连接度指数怎么算都是0的解决方案
- 遥感、制图学中各种图的区别
- 8能达到go速度吗 php_相同逻辑的php与golang代码效率对比,最好语言落谁家…
- python100天从新手到大师 pdf_Python100天从新手到大师(Python100Days)
- php post 400,post数据时报错:远程服务器返回错误: (400) 错误的请求。
- 荣耀v10玩flash游戏_“王者荣耀”游戏竟然还能这样玩?(送皮肤)
- VGA、DVI、HDMI区别
- 信安精品课:第7章访问控制技术原理与应用精讲笔记
- kali2020设置root用户登录
- python高级应用程序课程设计_Python高级应用程序设计任务