图片轮播器(swift)
如何实现一个无限循环,无缝衔接的图片轮播器 自己实现一次以后就不用使用轮播器的框架了
能用代码解决的问题就不在这里瞎BB了 O(∩_∩)O
首先先在Carousel文件件夹创建以下几个文件
CarouselFlowLayout.swift
CarouselView.swift
CarouselView.xib
CarsouselCell.swift
CarsouselCell.xib
Extension.swift
//CarouselFlowLayout.swift
import UIKit
class CarouselFlowLayout: UICollectionViewFlowLayout {
override func prepare() {
super.prepare()
itemSize = collectionView?.bounds.size ?? CGSize.zero
scrollDirection = .horizontal
minimumLineSpacing = 0
minimumInteritemSpacing = 0
collectionView?.isPagingEnabled = true
collectionView?.showsVerticalScrollIndicator = false
collectionView?.showsHorizontalScrollIndicator = false
}
}
// CarouselView.swift
//CarouselView.xib
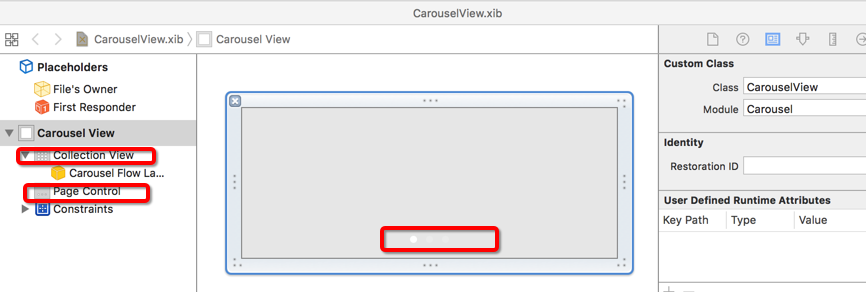
import UIKit
private let kCellID = "CarsouselCellID"
private let kMultiple = 10000
/// 图片轮播器
public class CarouselView: UIView {
/// collectionView
@IBOutlet var collectionView: UICollectionView!
/// 页码指示器
@IBOutlet var pageControl: UIPageControl!
/// 图片地址集合
public var imageURLList: [String]? {
didSet {
collectionView.reloadData()
pageControl.numberOfPages = imageURLList?.count ?? 0
}
}
/// 定时器
var timer: Timer?
public override func awakeFromNib() {
super.awakeFromNib()
// 注册Cell
collectionView.register(UINib(nibName: "CarsouselCell", bundle: Bundle(for: CarsouselCell.self)), forCellWithReuseIdentifier: kCellID)
// 设置数据源
collectionView.dataSource = self
// 设置代理
collectionView.delegate = self
pageControl.hidesForSinglePage = true
}
public override func layoutSubviews() {
super.layoutSubviews()
collectionView.scrollToItem(at: IndexPath(item: (imageURLList?.count ?? 0) * Int(Float(kMultiple) * 0.5), section: 0), at: .left, animated: false)
}
}
// MARK: - 公共方法
extension CarouselView {
/// 获取图片轮播器
///
/// - returns: 图片轮播器对象
public static func carouselView() -> CarouselView{
return Bundle(for: CarouselView.self).loadNibNamed("CarouselView", owner: nil, options: nil)?.first as! CarouselView
}
/// 开始自动滚动
public func start() {
end()
timer = Timer.scheduledTimer(timeInterval: 3.0, target: self, selector: #selector(doTask), userInfo: nil, repeats: true)
RunLoop.main.add(timer!, forMode: .commonModes)
}
/// 停止自动滚动
public func end() {
timer?.invalidate()
timer = nil
}
@objc private func doTask() {
var contentOffset = collectionView.contentOffset
contentOffset.x += collectionView.frame.width
collectionView.setContentOffset(contentOffset, animated: true)
}
}
// MARK: - UICollectionViewDataSource
extension CarouselView: UICollectionViewDataSource {
public func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return (imageURLList?.count ?? 0) * kMultiple
}
public func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: kCellID, for: indexPath) as! CarsouselCell
cell.imageURL = imageURLList?[indexPath.item % (imageURLList?.count ?? 1)]
return cell
}
}
// MARK: - UICollectionViewDelegate
extension CarouselView: UICollectionViewDelegate {
public func scrollViewDidScroll(_ scrollView: UIScrollView) {
let offsetPage = scrollView.contentOffset.x / scrollView.bounds.width
let currentIndex = Int(round(offsetPage))
pageControl.currentPage = currentIndex % (imageURLList?.count ?? 1)
}
public func scrollViewWillBeginDragging(_ scrollView: UIScrollView) {
end()
}
public func scrollViewDidEndDragging(_ scrollView: UIScrollView, willDecelerate decelerate: Bool) {
start()
}
}
// CarsouselCell.swift
//CarsouselCell.xib
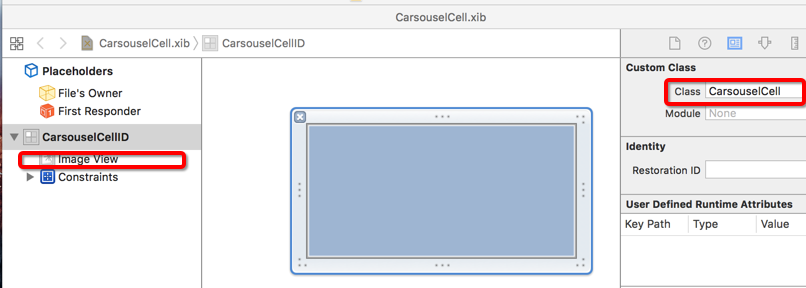
import UIKit
class CarsouselCell: UICollectionViewCell {
/// 图片区域
@IBOutlet var imageView: UIImageView!
var imageURL: String? {
didSet{
imageView.sd_setImage(path: imageURL)
}
}
}
// Extension.swift
import UIKit
import SDWebImage
extension UIImageView {
public func sd_setImage(path: String?) {
guard let noNullPath = path as? NSString else { return }
guard let newPath = noNullPath.addingPercentEncoding(withAllowedCharacters: .urlFragmentAllowed) else { return }
self.sd_setImage(with: URL(string: newPath))
}
}
到此图片轮播器的代码已经over了
在项目中如何使用呢?
// ViewController.swift
// Carousel
import UIKit
import Carousel
class ViewController: UIViewController {
lazy var cv: CarouselView = CarouselView.carouselView()
override func viewDidLoad() {
super.viewDidLoad()
cv.frame = CGRect(x: 0, y: 100, width: UIScreen.main.bounds.width, height: 150)
cv.imageURLList = ["http://www.17sucai.com/preview/569922/2016-06-30/轮播/images/3.jpg", "http://www.17sucai.com/preview/569922/2016-06-30/轮播/images/1.jpg"]
view.addSubview(cv)
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
cv.start()
}
}
图片轮播器(swift)相关推荐
- swift:创建滚动视图的图片轮播器
用swift创建图片轮播器和用OC创建的方式是一样的,都主要用到UIScrollView和UIImageview这两个控件,有几张图片,就将滚动视图的内容区域大小设置为每一张图片的大小乘以张数即可.然 ...
- 图片轮播器,relativelayout,外加textview小结
十多个textview,外加三个relativelayout,心烦的是图片轮播器,就环境老玄了,写了三个图片轮播器才成功,直接来代码吧,gogogo. Activity_main.xml <?x ...
- IOS开发基础之图片轮播器-12
IOS开发基础之图片轮播器-12 核心代码 // // ViewController.m // 12-图片轮播器 // // Created by 鲁军 on 2021/2/2. //#import ...
- javascript+css实现走马灯图片轮播器
javascript+css实现图片轮播-走马灯式 该图片轮播器主要是通过改变"图片容器"(即下面html代码中的div class="innerBox")的m ...
- 图片轮播器——javascript
在网页中,图片轮播器用得比较多. 效果图: <!DOCTYPE html> <html><head><meta http-equiv="Conten ...
- Android高级图片滚动控件,编写3D版的图片轮播器
转载请注明出处:http://blog.csdn.net/guolin_blog/article/details/17482089 大家好,好久不见了,最近由于工作特别繁忙,已经有一个多月的时间没写博 ...
- JAVA轮播器_Android 图片轮播器的实现及源码解析
在很多产品,尤其是电商类社区内的网页或者app中,我们经常会看到一个图片轮播墙,一页一页的广告/活动/商品介绍每隔一段时间就切换到下一张.那在安卓中我们该如何实现图片轮播器呢?面对自定义样式.自定义图 ...
- 图片轮播c语言,IOS开发之UIScrollView实现图片轮播器的无限滚动
IOS开发之UIScrollView实现图片轮播器的无限滚动 简介 在现在的一些App中常常见到图片轮播器,一般用于展示广告.新闻等数据,在iOS内并没有现成的控件直接实现这种功能,但是通过UIScr ...
- ios ScrollerView之图片轮播器
ios ScrollerView之图片轮播器 今天项目中用到了图片轮播器,写完之后贴到博客里来记录一下,也方便有兴趣的同学学习 #import "JYHCarouselController. ...
最新文章
- Windows Phone 8初学者开发—第21部分:永久保存Wav音频文件
- python赋值字符串的切片_python基础知识之字符串
- 怎样快速识别 英文地址中包含非英文字符_[论文笔记]端到端的场景文本识别算法--CRNN 论文笔记...
- 你对博客中提到的评分规则有何意见和建议?
- 鲲鹏云实验-Python+Jupyter机器学习基础环境
- Python综合练习:学生信息管理(文件版)
- spring-security过程分析
- yum install ruby出错:error downloading packages
- java 是怎么跨平台运行的
- 详解:Sqoop的介绍
- 【 javascript 】.innerHTML属性定义
- hibernate一对多双向关联中怎么配置list
- 【正点原子STM32连载】 第三十三章 光敏传感器实验 摘自【正点原子】MiniPro STM32H750 开发指南_V1.1
- 【音频处理】使用 Adobe Audition 录制电脑内部声音 ( 启用电脑立体声混音 | Adobe Audition 中设置音频设备 | Adobe Audition 内录 )
- Java学习笔记6——网络编程
- 百度地图API和2D/3D地图的转换
- windows默认共享的打开和关闭
- ligerUI的dialog
- 动态修改esxi虚拟机的CPU和内容
- Windows10 中的字母映射表