红黑树如何快速调整到平衡态_快速多态
红黑树如何快速调整到平衡态
进阶主题 (Advanced Topic)
Object-Oriented Programming has ruled the programming world for years. Some programming languages (such as Java) were designed with the native support to what OOP had principled, and some older ones (such as C++) were changed in such a way to add support for OOP lovers. Although during the years there have been new approaches, OOP is still one of the best approaches to use for almost any kind of software project with differnet size and different team skills. Despite all these popularity, this article is not about OOP, it is about walking through together to learn how we can implement Polymorphism using Swift Protocols as well as the traditional OOP approach.
面向对象编程已经统治了编程世界多年。 设计某些编程语言(例如Java)时,要获得对OOP原则性的本机支持,而对某些较旧的语言(例如C ++)进行了更改,以增加对OOP爱好者的支持。 尽管多年来已经有了新的方法,但是OOP仍然是几乎任何一种具有不同网络规模和不同团队技能的软件项目所使用的最佳方法之一。 尽管有如此之多的人气,但本文并不是关于OOP的,而是要逐步学习如何使用Swift协议以及传统的OOP方法来实现多态 。
什么是多态 (What is Polymorphism)
According to Wikipedia
根据维基百科
polymorphism is about provisioning a single interface to entities of different types.
多态性 是关于为不同类型的实体提供单个接口 。
Although it is usually underestimated, it is one of the most powerful concepts in software engineering that lets you define different behaviors for different objects while you are still using a shared interface among them. Don’t worry if it is still vague to you, let’s take a quick recap of how it works.
尽管它通常被低估,但它是软件工程中最强大的概念之一,可让您在仍使用对象之间的共享接口的同时为不同的对象定义不同的行为。 不用担心它是否对您仍然很模糊,让我们快速回顾一下它的工作原理。
如何使用多态 (How to use Polymorphism)
Imagine we are asked to develop a simple zoo application that could contain different types of animals, but for simplicity, let’s just imagine only 3 animals: Lion, Snake, and Eagle. For simplicity, we are asked just to focus on a single behavior for these types, moving
假设我们被要求开发一个简单的动物园应用程序,其中可能包含不同类型的动物,但为简单起见,我们仅假设仅3种动物:狮子,蛇和鹰。 为简单起见,我们被要求只专注于这些类型的单一行为, 移动
The first approach that comes to our mind is to define 3 objects as Lion, Snake, Eagle, and then, define different properties and behaviors for them. Although it doesn’t seem to be a bad design in the first place, we would face issues if we are asked to add more animals with the same categories, such as Pelican or Tiger. What we see is that we are gradually repeating the same behavior for all new animals.
我们想到的第一种方法是将3个对象定义为Lion,Snake,Eagle,然后为它们定义不同的属性和行为。 尽管起初它似乎并不是一个糟糕的设计,但是如果要求我们添加更多具有相同类别的动物,例如鹈鹕或老虎,我们将面临问题。 我们看到的是,我们正在对所有新动物逐渐重复相同的行为。

This is an example where we can gain polymorphism to make a better design.
这是一个示例,我们可以获取多态性以进行更好的设计。
面向对象的方式 (OOP Way)
In OOP, polymorphism is implemented by another OOP principle, Inheritance. All we have to do is to define all objects as the parent type, and initiate them with the child object. Then, calling the parent function will perform the child’s overridden one. Magic!
在OOP中,多态性是通过另一个OOP原则Inheritance实现的 。 我们要做的就是将所有对象定义为父类型,并使用子对象启动它们。 然后,调用父函数将执行子函数的重写函数。 魔法!
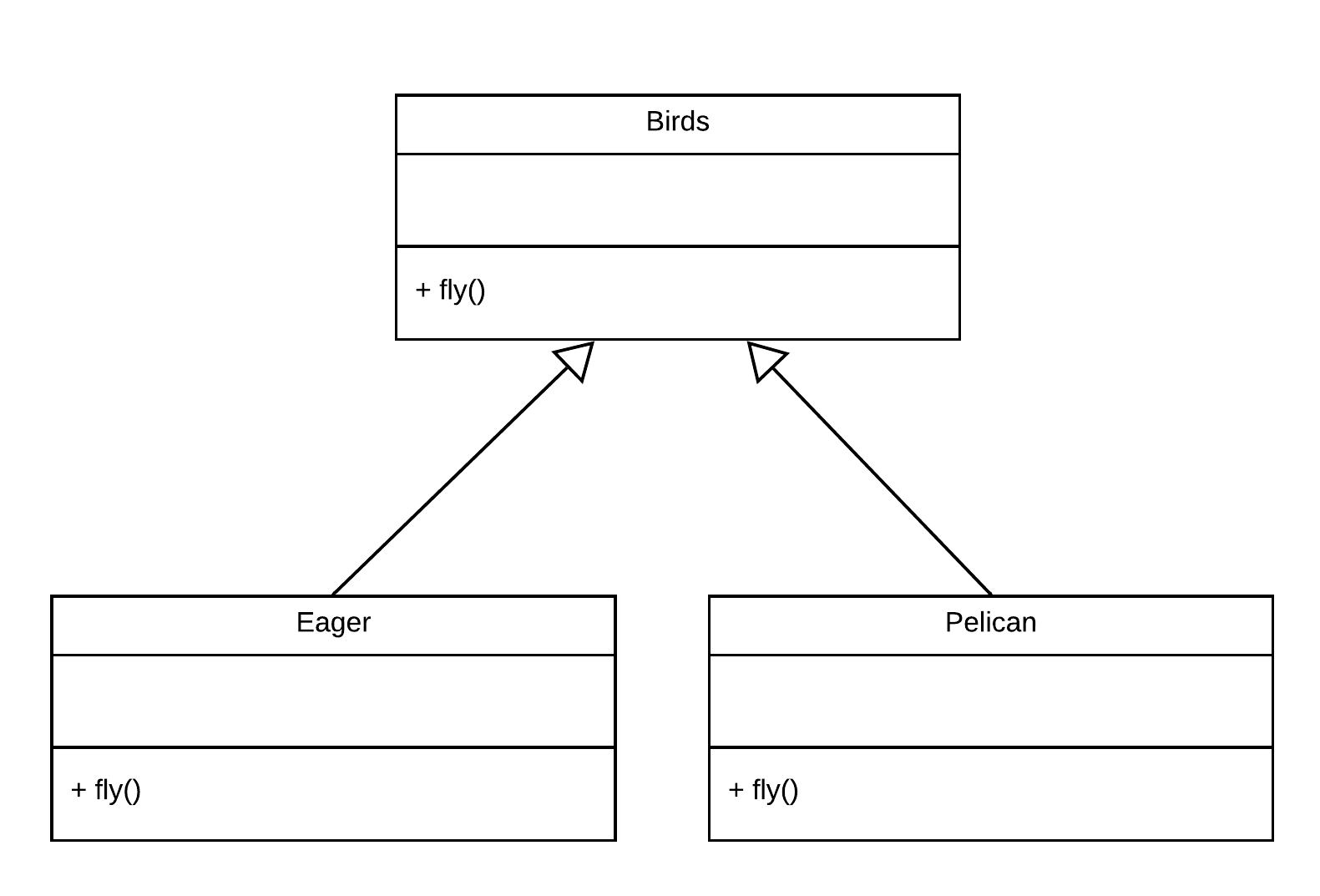
通讯协定 (Protocols)
With the same example in mind, let’s see how can we solve it using Protocols in Swift. Protocols are one of the most powerful Swift concepts making the programming even easier than before. Here, we would learn how they can be useful for the implementation of polymorphism, a Polymorphism without any Inheritances.
考虑到相同的示例,让我们看看如何使用Swift中的Protocols解决它。 协议是最强大的Swift概念之一,使编程比以前更加容易。 在这里,我们将学习它们如何对实现多态性(没有任何继承的多态性)有用。
To solve the design problem in our example, we first need to define proper Protocols. This is probably the most important step in designing your architecture, to decide what you want to design. In our example, we can think about different categories as different protocols.
为了解决示例中的设计问题,我们首先需要定义适当的协议。 这可能是设计体系结构,决定要设计的对象中最重要的步骤 。 在我们的示例中,我们可以将不同的类别视为不同的协议。

再一步 (One more step)
We have a proper polymorphism implemented without any inheritance using just protocols. Now, let’s see how we can go further and make it even better.
我们仅使用协议就实现了适当的多态性,而没有任何继承。 现在,让我们看看如何进一步发展并使其变得更好。
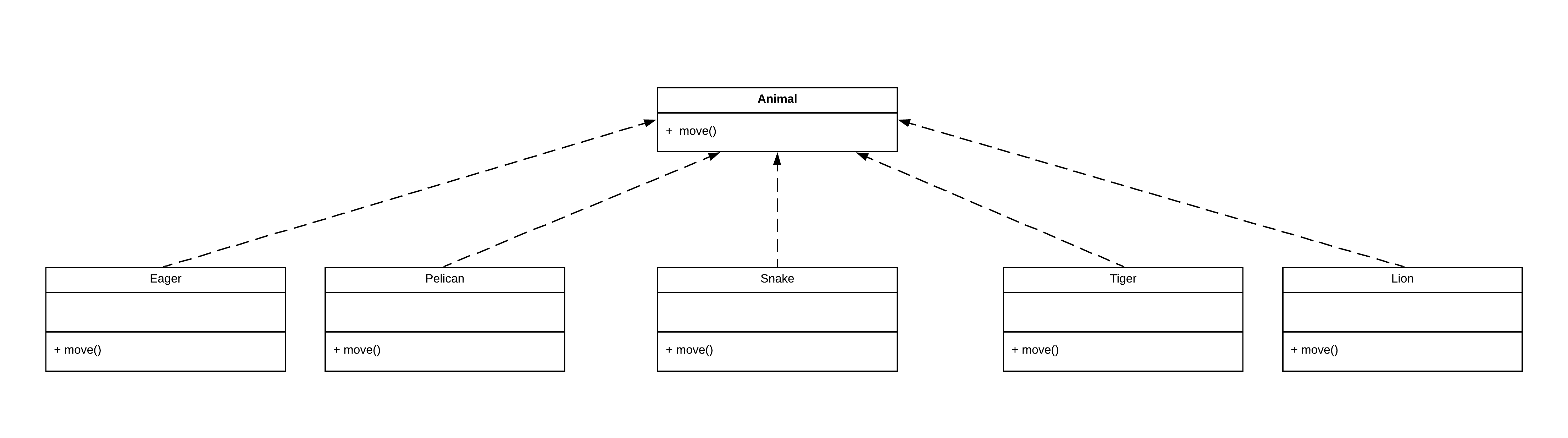
POP还是OOP? (POP or OOP?)
So far we have seen how to implement polymorphism with Object-Oriented and Protocol-Oriented approaches. But which one would you prefer? What are the advantages of each one?
到目前为止,我们已经看到了如何使用面向对象和面向协议的方法来实现多态。 但是您想要哪一个呢? 每个人都有哪些优势?
While polymorphism is an OOP principle, I’d personally prefer to use Protocols to implement it, especially, for large scale projects. Let’s take a quick look at a more complex level of our current zoo project.
尽管多态性是OOP原则,但我个人更喜欢使用协议来实现它,尤其是对于大型项目。 让我们快速看一下当前动物园项目的更复杂层次。
Imagine that now we are asked to add specific behaviors to different animal categories that have nothing in common with any other categories. For example, we now know that Big Cats are naturally attackers, so we want to have a record of their aggressive reactions. This behavior is shared between all Big Cats, but it is not true for other animals such as Birds.
想象一下,现在我们被要求向与其他类别没有共同点的不同动物类别添加特定的行为。 例如,我们现在知道大猫是天生的攻击者,因此我们想要记录它们的攻击性React。 所有大型猫科动物都有这种行为,但其他动物(例如鸟类)却并非如此。
Now we have to decide which approach we would rather to use.
现在,我们必须决定要使用哪种方法。
面向对象 (OOP)
With OOP, we need to come up with a layered inheritance design that reflects our real-world problem. In this case, we need to add one more parent objects that contains the functions we are asked for. Since it is not possible to have multiple inheritances in most modern OOP languages, the middle parent also has to inherit from another parent. Since this could be kind of confusing now, let’s check the code.
使用OOP,我们需要提出一个分层的继承设计,以反映我们的实际问题。 在这种情况下,我们需要再添加一个包含我们要求的功能的父对象。 由于在大多数现代OOP语言中不可能有多个继承,因此中间父级也必须从另一个父级继承。 由于这现在可能会造成混淆,因此让我们检查一下代码。

流行音乐 (POP)
With Protocols, you don’t need to have any inheritance (although you can have inheritance for your Protocols). Any objects in Swift can conform to multiple Protocols at the same time, which means it can hold different behaviors at the same time without any additional inheritance. Check the code below to get the idea.
使用协议,您不需要任何继承(尽管您可以对协议进行继承)。 Swift中的任何对象都可以同时符合多个协议,这意味着它可以同时具有不同的行为,而无需任何其他继承。 查看下面的代码以了解主意。

结论 (Conclusion)
As you have seen so far, polymorphism is a very powerful concept that lets you design complex relations between objects in a scalable manner. As both OOP and POP offers their own advantages, it is finally up to you, as a software engineer, to choose horizontal growth (POP) or vertical growth (OOP). Personally, I’d rather POP as it is applicable to Swift structs types which are commonly advised over using classes due to performance improvements.
到目前为止,您已经看到,多态是一个非常强大的概念,可让您以可扩展的方式设计对象之间的复杂关系。 由于OOP和POP都有各自的优势,因此最终,作为软件工程师,您需要选择水平增长(POP)或垂直增长(OOP)。 就个人而言,我更喜欢POP,因为它适用于Swift结构结构类型,由于性能改进,通常建议在使用类时使用POP结构。
There are also many articles available to help you to combine different features to gain even better design decisions, where you will end up with a code that is easier to read, maintain, and scale.
也有许多文章可帮助您组合不同的功能以获得更好的设计决策,最终您将获得一个易于阅读,维护和扩展的代码。
翻译自: https://levelup.gitconnected.com/polymorphism-in-swift-b03def92fa26
红黑树如何快速调整到平衡态
http://www.taodudu.cc/news/show-4316159.html
相关文章:
- 属兔2013年蛇年运程
- 【LSTM回归预测】基于matlab布谷鸟算法优化LSTM回归预测【含Matlab源码 2037期】
- list移除元素时报错
- 获取svg内text文本元素的高度、宽度及坐标等信息
- 传奇装备元素属性设置教程
- SparkSql 控制输出文件数量且大小均匀(distribute by rand())
- Hive Distribute by 应用之动态分区小文件过多问题优化
- Python安装distribute包
- 计算机总是蓝屏怎么解决办法,笔记本电脑总蓝屏如何解决_笔记本频繁蓝屏怎么办-win7之家...
- 电脑开机蓝屏怎么解决?3个方法,快速解决电脑蓝屏
- w7电脑蓝屏怎么解决_详解win7电脑蓝屏怎么办
- w7电脑蓝屏怎么解决_教您电脑蓝屏怎么办
- 物联网的云计算、雾计算、边缘计算和MIST计算的基本概念
- unity沙子堆积_unity游戏动态体积雾沙尘暴管理渲染插件Dynamic Fogamp;Mist 6.5 - 素材巷...
- CentOS6 安装mist.io
- Pychram连接mist远程服务器踩坑指南
- 增量式旋转编码器的使用,以arduino为例
- Arduino学习笔记(14)-- Arduino使用增量编码器测速
- 计算机页面不稳定怎么办,电脑网速不稳定怎么解决_电脑网速时快时慢的处理方法...
- 使用weixin4j开发微信JS-SDK微信配置接口实现分享朋友圈
- 使用weixin-java-miniapp实现微信小程序登录接口
- uni-app 开发微信小程序 自动化编译,启动项目
- laravel5实现第三方登录(微信)
- Android Studio超级详细安装教程(AMD)
- ADM打不开/data,或打开后无法导出里面的文件
- 生动化你的表达——DuerOS中的SSML应用
- 三菱CN1接线
- 补题系列 1 最小布线
- P3647 [APIO2014]连珠线
- luogu P3647 [APIO2014] 连珠线
红黑树如何快速调整到平衡态_快速多态相关推荐
- 红黑树和平衡二叉树的区别_一文搞懂红黑树
文章参考 | https://segmentfault.com/a/1190000012728513 前言 当在10亿数据进行不到30次比较就能查找到目标时,不禁感叹编程之魅力! 二叉树 在了解红黑树 ...
- 红黑树和平衡二叉树的区别_面试题精选红黑树(c/c++版本)
红黑树的使用场景非常广泛,比如nginx中用来管理timer.epoll中用红黑树管理事件块(文件描述符).Linux进程调度Completely Fair Scheduler用红黑树管理进程控制块. ...
- 红黑树 平衡二叉搜索树_红黑树:自我平衡的二叉搜索树,并举例说明
红黑树 平衡二叉搜索树 什么是红黑树? (What is a Red-Black Tree?) Red-Black Tree is a type of self-balancing Binary Se ...
- 常见的数据结构:栈 队列 数组 链表 红黑树——List集合 _ HashSet集合、可变参数 collections集合 Map集合
2021-06-07复习java 一.常见的数据结构 栈(先进后出) 队列 数组 链表 红黑树 二.List集合_介绍&常用方法 ArrayList集合 Linkedlist集合 三.Hash ...
- 红黑树-Java实现
目录 一.定义 二.插入 三.删除 四.全部代码 五.颜色效果 一.定义 红黑树是特殊的平衡二叉树,具有以下特性: 1.根节点的颜色是黑色 2.节点颜色要么是黑色.要么是红色 3.如果一个节点的颜色是 ...
- 红黑树 键值_查找(一)史上最简单清晰的红黑树讲解
http://blog.csdn.net/yang_yulei/article/details/26066409 查找(一) 我们使用符号表这个词来描述一张抽象的表格,我们会将信息(值)存储在其中,然 ...
- C++_泛型编程与标准库(九)——红黑树的使用
C++_泛型编程与标准库(九)--红黑树的使用 参考:<侯捷泛化编程与标准库>.GNU9.3.0,vs2019 图中标红部分为自己的笔记理解 struct _Rb_tree_node_ba ...
- 红黑树分为红和黑有什么好处_彻底搞懂红黑树
红黑树和c++ 虚拟继承内存分布 几乎成了我的死敌,因为完全没用过,所以导致每次看懂了之后都忘了(也许不是真的看懂了,有些关键性的东西没理解透),这次准备把这两个难题(其实也不难)仔细看懂,然后再写一 ...
- java treeset 红黑树_【数据结构】红黑树与跳表-(SortSet)-(TreeMap)-(TreeSet)
SortSet 有序的Set,其实在Java中TreeSet是SortSet的唯一实现类,内部通过TreeMap实现的:而TreeMap是通过红黑树实现的:而在Redis中是通过跳表实现的: Skip ...
最新文章
- isinstance函数和@staticmethod用法
- 零基础学习SVN之(二):CVS与SVN的区别
- else if mybatis 嵌套_mybatis踩坑之——foreach循环嵌套if判断
- php文件名函数,php 获取文件名basename()函数的用法总结
- sql a 表 若包含b表 则a 表 列显示_几道常见的SQL面试题,看你能答对几道?
- 15、iOS开发之duplicate symbols for architecture x86_64错误
- codeforces1455 D. Sequence and Swaps
- 限制会话id服务端不共享_不懂 Zookeeper?看完不懂你打我
- 一份阿里员工的Java问题排查工具单
- python中模块文件的扩展名不一定是py_python模块和python包有什么区别?
- apdu 移动sim_SIM卡APDU指令【转】
- 网络子系统34_网桥设备的传输与接收
- 十个接私活赚外快的网站,你有技术就有钱
- FPGA工程师面试试题集锦41~50
- 开源自己开发的一款宠物小精灵游戏
- linux drwxr-xr-x 什么意思
- 从手机端 H5 制作来看 WEB 动画的术与道
- pyqt5 图像上划线_PyQt5 绘制画 线(鼠标笔)
- java系列之redis基础
- N: 无法安全地用该源进行更新,所以默认禁用该源
热门文章
- Flutter中的多选按钮组件Checkbox
- 新产品、新特性、新生态丨一文回顾openGauss峰会云和恩墨分论坛150分钟的精彩...
- Win7系统服务优化攻略
- 男人的终极品味是选妻
- 理论力学知识要点归纳(一)
- Z3735d android x86,首款搭载Z3735处理器 神秘平板被曝光
- android os 1.5 下载地址,技德Remix OS 1.5发布 适配Android 5.0
- 位段(域)机制——结构体的特殊实现
- 【自然语言处理篇】--Chatterbot聊天机器人
- 计算机网络传输介质的特点,计算机网络基础:数据通信技术之传输介质