php表单验证_用PHP进行表单验证
php表单验证
In this article you’ll construct and validate a simple form using HTML and PHP. The form is created using HTML and validation and processing of the form’s contents is done with PHP. The goal is to teach you some basic HTML form elements and how their data is accessible to you in your PHP scripts.
在本文中,您将使用HTML和PHP构造并验证一个简单的表单。 表单是使用HTML创建的,表单内容的验证和处理是通过PHP完成的。 目的是教您一些基本HTML表单元素以及如何在PHP脚本中访问它们的数据。
The form you’ll create contains a number of inputs: text fields, radio buttons, a multiple-option select list, a checkbox, and a submit button. These inputs will be validated to ensure, for example, that the user has entered their name. Any errors or omissions will be highlighted with a message alongside the relevant field.
您将创建的表单包含许多输入:文本字段,单选按钮,多选项选择列表,复选框和提交按钮。 这些输入将得到验证,以确保例如用户输入了他们的姓名。 任何错误或遗漏将在相关字段旁边以消息突出显示。
HTML表格 (The HTML Form)
First, let’s look at the form. The purpose of the form is to capture user details (name, address, and email) to obtain feedback (on fruit consumption and favorite fruit) and request a brochure.
首先,让我们看一下表格。 该表格的目的是捕获用户详细信息(姓名,地址和电子邮件)以获得反馈(关于水果消费和喜欢的水果)并索取手册。
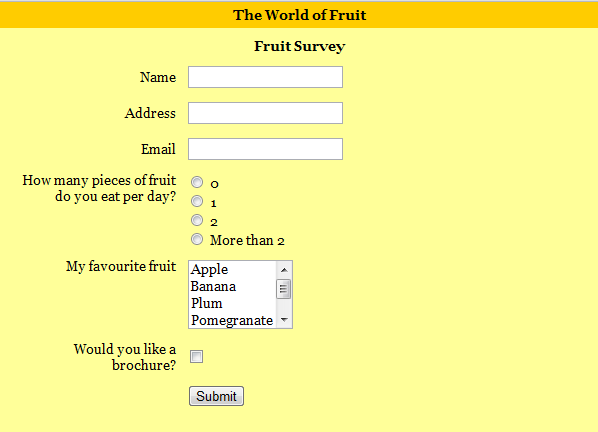
文字栏位 (Text Fields)
The name, address, and email fields are text input
elements and can be coded like this:
名称,地址和电子邮件字段是文本input
元素,可以这样编码:
Name <input type="text" name="name" value="">
Address <input type="text" name="address" value="">
Email <input type="text" name="email" value="">
The HTML input
element can have several attributes, the most important of which are the type
and name
attributes. type="text"
defines these instances as a single-line input field to accept text. The name
attribute is used to specify a name for this value.
HTML input
元素可以具有多个属性,其中最重要的是type
和name
属性。 type="text"
将这些实例定义为接受文本的单行输入字段。 name
属性用于为此值指定名称。
单选按钮 (Radio Buttons)
<input type="radio" name="howMany" value="zero"> 0
<input type="radio" name="howMany" value="one"> 1
<input type="radio" name="howMany" value="two"> 2
<input type="radio" name="howMany" value="twoplus"> More than 2
Here type="radio"
renders the input as a radio button. Notice how each button is assigned the same name. This is because collectively the radio buttons should be treated as a group (the user should select either 0, or 1, or 2, etc.). The value
attribute differs for each button to provide the different selections that can be made.
在这里, type="radio"
将输入呈现为单选按钮。 注意如何为每个按钮分配相同的名称。 这是因为单选按钮应一起视为一个组(用户应选择0或1或2等)。 每个按钮的value
属性都不同,以提供可以进行的不同选择。
选择清单 (Select List)
<select name="favFruit[]" size="4" multiple>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="plum">Plum</option>
<option value="pomegranate">Pomegranate</option>
<option value="strawberry">Strawberry</option>
<option value="watermelon">Watermelon</option>
</select>
In this case, the name
attribute of the select element is given as an array (with square brackets) because multiple choices are allowed (because of the presence of the multiple
attribute). Without multiple
, only one option would be selectable.
在这种情况下,由于允许多个选择(因为存在multiple
属性),因此select元素的name
属性以数组形式(带有方括号)给出。 没有multiple
,则只能选择一个选项。
Each option specified within the select has a distinct value. The value
attribute for each option element is the value that will be submitted to the server, and the text between the opening and closing option
tag is the value the user will see in the menu.
选择中指定的每个选项都有一个不同的值。 每个选项元素的value
属性是将提交给服务器的值,开始和结束option
标签之间的文本是用户将在菜单中看到的值。
选框 (Checkbox)
<input type="checkbox" name="brochure" value="Yes">
The input is presented as a checkbox. Its value will be set to “Yes” if the box has been checked.
输入显示为一个复选框。 如果选中此框,则其值将设置为“是”。
提交按钮 (Submit Button)
<input type="submit" name="submit" value="Submit">
Last but not least there is the submit button. Its value is presented as its label, and clicking on it will trigger the form submission.
最后但并非最不重要的一点是提交按钮。 其值显示为标签,单击它会触发表单提交。
表单元素 (The Form Element)
There are two specific attributes that need to be set in the form tag, action
and method
:
需要在form标记中设置两个特定的属性,即action
和method
:
<form method="POST"
action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
htmlspecialchars()
is used here to convert specific HTML characters to their HTML entity names, e.g. >
will be converted to >
. This prevents the form from breaking if the user submits HTML markup and also is a means of protecting against XSS (Cross Site Scripting) attacks, which attackers will use to try to exploit vulnerabilities in web applications.
htmlspecialchars()
在此处用于将特定HTML字符转换为其HTML实体名称,例如>
将被转换为>
。 这可以防止表单在用户提交HTML标记时中断,并且是防止XSS(跨站点脚本)攻击的一种方法,攻击者将利用该方法尝试利用Web应用程序中的漏洞 。
action
defines where the form contents are sent when the form is submitted; in this case, the value of $_SERVER["PHP_SELF"]
.
action
定义提交表单时将表单内容发送到的位置; 在这种情况下, $_SERVER["PHP_SELF"]
。
$_SERVER
is an array with entries filled in at PHP run time; the PHP_SELF
key contains the filename of the PHP script that is being executed. Using $_SERVER["PHP_SELF"]
is preferable to simply hard-coding a location if you want your form to post back to the same script that generated it since you won’t have to update the code if you change the script’s name.
$_SERVER
是一个数组,其中的条目在PHP运行时填充; PHP_SELF
键包含正在执行PHP脚本的文件名。 如果您希望表单将其回发到生成该脚本的同一脚本,则使用$_SERVER["PHP_SELF"]
比简单地对位置进行硬编码更为可取,因为如果您更改脚本的名称就不必更新代码。
method
determines how the form’s contents is submitted. POST
means that the form’s content is sent as part of the HTTP request’s body. The values are then retrievable in PHP using the $_POST
array.
method
确定表单内容的提交方式。 POST
表示表单的内容作为HTTP请求主体的一部分发送。 然后可以使用$_POST
数组在PHP中检索这些值。
The alternative to POST
is GET
which passes the form’s values as part of the URL. Values sent using GET
are retrievable in PHP using $_GET
. The main difference between the methods POST
and GET
is visibility. There are numerous articles on the web about choosing between them, but my advise is to stick to POST
when using forms unless you have a good reason to pass user data in a viewable URL.
POST
的替代方法是GET
,它将表单的值作为URL的一部分传递。 使用GET
发送的值可以在PHP中使用$_GET
检索。 POST
和GET
方法之间的主要区别是可见性。 网上有很多文章供您选择,但是我的建议是使用表单时坚持使用POST
,除非您有充分的理由在可见URL中传递用户数据。
Remember that when working with HTML forms:
请记住,使用HTML表单时:
All of the form controls must be enclosed within the
form
tags.所有表单控件都必须包含在
form
标签内。- The alignment of text and form controls can be achieved in many ways. CSS is the preferred option for many, but be prepared to see tables used for alignment in older HTML.文本和表单控件的对齐方式可以通过多种方式实现。 CSS是许多人的首选选项,但请准备好查看旧HTML中用于对齐的表。
Not discussed here, but important for accessibility is the
label
element.这里没有讨论,但是对于可访问性很重要的是
label
元素 。
表格处理 (Form Processing)
Now that you’ve defined the form in HTML, let’s identify the two stages the form goes through – when the blank form is loaded, and when the user has completed the form and clicks the submit button. The script needs to be able to differentiate between the two stages so the form behaves properly.
现在,您已经使用HTML定义了表单,让我们确定表单经历的两个阶段-加载空白表单时,以及用户完成表单并单击提交按钮时。 该脚本需要能够在两个阶段之间进行区分,以便表单正常运行。
To determine whether the form has been submitted by the user, either check the request method (an example follows in the next section) or check for the presence of a specific form control (usually the submit button).
要确定用户是否已提交表单,请检查请求方法(下一节中的示例)或检查是否存在特定的表单控件(通常是“提交”按钮)。
Whichever method you use, if the form has been submitted, it should be validated. If it has not been submitted, bypass the validation and display a blank form.
无论使用哪种方法,如果已提交表单,都应进行验证。 如果尚未提交,请跳过验证并显示空白表格。
捕获表单内容 (Capturing the Form Contents)
So far, we have a largely HTML page which defines the form; the only PHP so far is to specify the form’s action value. We need to add code which will pick up the form data and allow us to check it and create error messages if needed.
到目前为止,我们已经有了一个很大HTML页面来定义表单。 到目前为止,唯一PHP是指定表单的操作值。 我们需要添加代码以提取表单数据,并允许我们检查它并在需要时创建错误消息。
At the start of the script, before any HTML, you can check whether the form has been submitted using $_SERVER["REQUEST_METHOD"]
. If the REQUEST_METHOD
is POST
, then you know the script has been submitted.
在脚本开始之前,在任何HTML之前,您都可以使用$_SERVER["REQUEST_METHOD"]
检查表单是否已提交。 如果REQUEST_METHOD
是POST
,那么您知道脚本已提交。
Detecting a form submission is a little bit trickier when you’re using GET
, because just requesting the page to view the form will probably happen as a GET
request as well. One common work around is provide a name attribute to the submit button, and check whether it is set in the $_GET
array with isset()
. For example:
当您使用GET
,检测表单提交会有些棘手,因为仅请求页面查看表单也可能会作为GET
请求发生。 一种常见的解决方法是为Submit按钮提供name属性,并使用isset()
检查是否在$_GET
数组中设置了它。 例如:
<input type="submit" name="submit" value="Submit">
<?php
if (isset($_GET["submit"])) {
// process the form contents...
}
验证表单内容 (Validating the Form Contents)
When the form is submitted, the following entries will be stored in the $_POST
array (or $_GET
array depending on the form’s method
attribute). The values in the left-hand column are taken from the control’s name
attribute, and I’ve also marked whether or not the field is a required field for validation purposes.
提交表单后,以下条目将存储在$_POST
数组(或$_GET
数组,具体取决于表单的method
属性)中。 左侧列中的值取自控件的name
属性,并且我还标记了该字段是否是用于验证目的的必填字段。
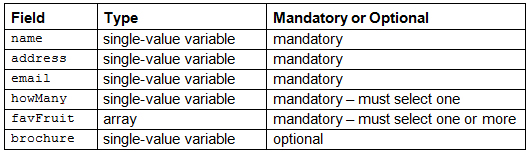
If the user does not comply with these rules, an error message will be displayed. Any fields already completed will be left unchanged, allowing the user to simply adjust her input and re-submit the form without having to enter all of the data again. See example below.
如果用户不遵守这些规则,将显示一条错误消息。 任何已经完成的字段都将保持不变,从而使用户可以简单地调整其输入并重新提交表单,而不必再次输入所有数据。 请参见下面的示例。
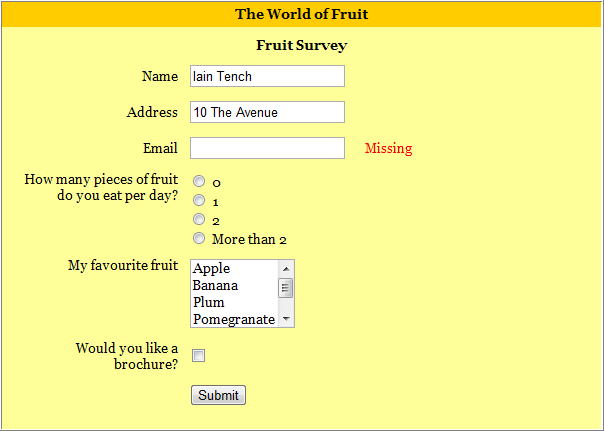
Let’s look at the PHP required for validating the form. All of this code would be placed towards the top of the page before the HTML for the form:
让我们看一下验证表单所需PHP。 所有这些代码都将放置在表单HTML之前的页面顶部:
<?php
// define variables and initialize with empty values
$nameErr = $addrErr = $emailErr = $howManyErr = $favFruitErr = "";
$name = $address = $email = $howMany = "";
$favFruit = array();
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (empty($_POST["name"])) {
$nameErr = "Missing";
}
else {
$name = $_POST["name"];
}
if (empty($_POST["address"])) {
$addrErr = "Missing";
}
else {
$address = $_POST["address"];
}
if (empty($_POST["email"])) {
$emailErr = "Missing";
}
else {
$email = $_POST["email"];
}
if (!isset($_POST["howMany"])) {
$howManyErr = "You must select 1 option";
}
else {
$howMany = $_POST["howMany"];
}
if (empty($_POST["favFruit"])) {
$favFruitErr = "You must select 1 or more";
}
else {
$favFruit = $_POST["favFruit"];
}
}
// the HTML code starts here
The code starts by creating and initializing the variables used in the validation process:
代码首先创建并初始化验证过程中使用的变量:
- A variable for each of the text fields and the favourite fruit array每个文本字段和喜欢的水果数组的变量
- Error message variables for each field每个字段的错误消息变量
The exception is the brochure checkbox which does not need to be validated as it is an optional field.
手册复选框是个例外,由于它是一个可选字段,因此无需进行验证。
Next determine whether the form has been submitted by checking how the page was requested. If the form has just been requested, then the if
statement will be bypassed. The function empty()
checks the text fields for name, address, and email, and the array for favorite fruit. isset()
checks whether a radio button is set for the fruit count. If any are empty, a suitable error message is assigned to the error variable.
接下来,通过检查页面的请求方式来确定是否已提交表单。 如果刚刚请求了表单,则将跳过if
语句。 函数empty()
检查文本字段的名称,地址和电子邮件,并检查数组中是否有喜欢的水果。 isset()
检查是否为水果计数设置了单选按钮。 如果为空,则会将适当的错误消息分配给错误变量。
In the example here I am checking the $_POST
array because the form’s method is POST
. If method was GET
, then I would check the $_GET
array for the input values.
在这里的示例中,我正在检查$_POST
数组,因为表单的方法是POST
。 如果method是GET
,那么我将检查$_GET
数组中的输入值。
Having run these checks, the code would continue with the HTML for the page. PHP needs to be incorporated into the HTML for each element:
运行这些检查后,代码将继续使用页面HTML。 需要将每个元素PHP合并到HTML中:
- To print the value submitted in the text fields so the user does not have to retype it. The value will of course be blank if the form has just been loaded and not submitted.要打印在文本字段中提交的值,以便用户不必重新键入它。 如果表单刚刚被加载而未提交,则该值当然将为空白。
- To print any error messages. Again these will be blank if the form has just been loaded or if there is no error for the field.打印任何错误消息。 同样,如果刚刚加载表单或该字段没有错误,则这些字段将为空白。
Here is the HTML definition of one of the input fields:
这是输入字段之一HTML定义:
<input type="text" name="name" value="">
And here is the same code amended to include the first point specified above:
这是修改为包含上面指定的第一点的相同代码:
<input type="text" name="name"
value="<?php echo htmlspecialchars($name);?>">
And then again to address the second point above:
然后再次解决以上第二点:
<input type="text" name="name"
value="<?php echo htmlspecialchars($name);?>">
<span class="error"><?php echo $nameErr;?></span>
htmlspecialchars()
is used here for the same reason it was used earlier with PHP_SELF
– to protect against XSS attacks.
此处使用htmlspecialchars()
的原因与先前与PHP_SELF
一起使用的原因相同-可以防止XSS攻击。
The use of the class error
provides a hook with which you can style the error message using CSS.
使用类error
提供了一个挂钩,您可以使用该挂钩使用CSS设置错误消息的样式。
.error {
color: #FF0000;
}
扩展表格的可用性 (Extending the Form’s Usability)
With a little more effort, you can also remember the choices the user made from the radio buttons and select.
稍加努力,您还可以记住用户从单选按钮所做的选择并进行选择。
<input type="radio" name="howMany"
<?php if (isset($howMany) && $howMany == "zero") echo "checked"; ?>
value="zero"> 0
<input type="radio" name="howMany"
<?php if (isset($howMany) && $howMany == "one") echo "checked"; ?>
value="one"> 1
<input type="radio" name="howMany"
<?php if (isset($howMany) && $howMany == "two") echo "checked"; ?>
value="two"> 2
<input type="radio" name="howMany"
<?php if (isset($howMany) && $howMany == "twoplus") echo "checked"; ?>
value="twoplus"> More than 2
Code has been added to check whether the variable associated with the radio button has been defined within the validation section. If so, then checked
is outputted as part of the element’s HTML.
已添加代码以检查与单选按钮关联的变量是否已在验证部分中定义。 如果是这样,则将checked
作为元素HTML的一部分输出。
Now to address the select menu. It is actually a better idea to restructure the code as a loop than to blindly add code to check each option manually. The loop would generate the HTML for the options on the fly, and the check if the option has been selected or not can be incorporated into it.
现在来解决选择菜单。 与盲目添加代码以手动检查每个选项相比,将代码重组为循环实际上是一个更好的主意。 该循环将动态生成选项HTML,并可以将选项是否已选中的检查合并到其中。
<select name="favFruit[]" size="4" multiple="">
<?php
$options = array("apple", "banana", "plum", "pomegranate", "strawberry", "watermelon");
foreach ($options as $option) {
echo '<option value="' . $option . '"';
if (in_array($option, $favFruit)) {
echo " selected";
}
echo ">" . ucfirst($option) . "</option>";
}
?>
</select>
If you are not yet familiar with PHP loops, you may want to read my earlier article, Learning Loops.
如果您还不熟悉PHP循环,则可能需要阅读我以前的文章Learning Loops 。
Note that the difference here is the use of the attribute selected
; radio buttons use checked
and select options use selected
. It’s just one of those minor inconsistencies we have to live with.
注意这里的区别是使用selected
属性; 单选按钮使用已checked
,选择选项使用selected
。 这只是我们必须忍受的那些微小矛盾之一。
One topic which I have not addressed and is outside the scope of this article is what you would do with the data after validation is successful. There are a number of possibilities, including saving it in a database or emailing the data to yourself. Whichever you choose, you need to be sure:
验证成功后,您将如何处理数据,这是我尚未解决且不在本文讨论范围之内的一个主题。 有很多可能性,包括将其保存在数据库中或通过电子邮件将数据发送给您自己。 无论选择哪种方式,都需要确保:
- The form has been submitted by the user该表格已由用户提交
- The data entered by the user is error-free用户输入的数据没有错误
摘要 (Summary)
Validation is essential, particularly if you are going to save the data in a database – remember the old saying, GIGO (Garbage In, Garbage Out), and you won’t go far wrong. In this article you have learned how to create a simple HTML form and validate it with PHP. Along the way a number of techniques have been used to re-display user input and display error messages. For further information on $_SERVER
, $_POST
, and $_GET
, read the article Introducing Superglobals and of course the PHP documentation.
验证是必不可少的,特别是如果您要将数据保存在数据库中–请记住俗语GIGO(垃圾输入,垃圾输出),这不会出错。 在本文中,您学习了如何创建简单HTML表单并使用PHP对其进行验证。 在此过程中,已经使用了许多技术来重新显示用户输入并显示错误消息。 有关$_SERVER
, $_POST
和$_GET
更多信息,请阅读介绍Superglobals的文章以及PHP文档 。
And if you enjoyed reading this post, you’ll love Learnable; the place to learn fresh skills and techniques from the masters. Members get instant access to all of SitePoint’s ebooks and interactive online courses, like Jump Start PHP.
并且,如果您喜欢阅读这篇文章,您会喜欢Learnable的 ; 向大师学习新鲜技能的地方。 会员可以立即访问所有SitePoint的电子书和交互式在线课程,例如Jump Start PHP 。
Comments on this article are closed. Have a question about PHP? Why not ask it on our forums?
本文的评论已关闭。 对PHP有疑问吗? 为什么不在我们的论坛上提问呢?
Image via Valentyn Volkov / Shuttestock
图片来自Valentyn Volkov / Shuttestock
翻译自: https://www.sitepoint.com/form-validation-with-php/
php表单验证
php表单验证_用PHP进行表单验证相关推荐
- 数据透视表 字段交叉_删除数据透视表的计算字段的宏
数据透视表 字段交叉 Have you ever recorded a macro to remove pivot table calculated fields? Just turn on the ...
- java程序license验证_基于TrueLicense实现产品License验证功能
受朋友所托,需要给产品加上License验证功能,进行试用期授权,在试用期过后,产品不再可用. 通过研究调查,可以利用Truelicense开源框架实现,下面分享一下如何利用Truelicense实现 ...
- 数据结构顺序表的查找_数据结构1|顺序表+链表
数据结构学习笔记1 进度:静态分配顺序表+单链表 参考资料:b站 王道考研+小甲鱼 < 判断一个算法的效率时,函数中的常数和其他次要项常常可以忽略,而更应该关注最高项目.的阶数. 推导大O阶方法 ...
- 2007数据透视表如何删除_删除数据透视表标题中的总和
2007数据透视表如何删除 When you're building a pivot table, if you add fields to the Values area, Excel automa ...
- mysql分库分表按时间_数据库分库分表思路
一. 数据切分 关系型数据库本身比较容易成为系统瓶颈,单机存储容量.连接数.处理能力都有限.当单表的数据量达到1000W或100G以后,由于查询维度较多,即使添加从库.优化索引,做很多操作时性能仍下降 ...
- excel工作表添加目录_长Excel工作表的目录
excel工作表添加目录 In the comments for my post on creating a table of contents in Excel, Eden asked: " ...
- mysql表的类型_浅谈MySQL表类型
MySQL为我们提供了很多表类型供选择,有MyISAM.ISAM.HEAP.BerkeleyDB.InnoDB,MERGE表类型,萝卜白菜各有所爱是不假,可是真正选择何种表类型还是要看业务需要啊,每一 ...
- mysql 两张表合并查询_中级数据分析-多表查询
表的加法 表的联结 联结应用案例 case应用案例 一.表的加法 加法:union 表的加法是把两个表的数据,按行合并在一起. 表的加法,会把两个表里重复的数据删除,只保留一个. 如果想保留重复数据, ...
- mysql表空间满_怎么解决数据库表空间不足
一.数据库表空间不足导致了,插入操作会报出conn.msg = ORA-01653: 表 *******表名字********无法通过 8192 (在表空间 USERS 中) 扩展 ORA-06512 ...
最新文章
- 智慧城市搞圈地卖设备的思路该结束了
- python中itertools模块介绍---03
- givemesomecredit数据_你是如何走上数据分析之路的?
- Nodejs进阶:使用DiffieHellman密钥交换算法
- 《乐在C语言》一2.2 数据类型
- PHP文件系统-文件上传类
- python输入一个整数、输出该整数的所有素数因子_【401】Python 求合数的所有质数因子...
- java堆排序工具包_JAVA 排序工具类
- Aibaba Dubbo 的前世今生以及黑历史 主程序员梁飞 阿里P9(2016年查看)
- 模拟调制与抗噪声性能MATLAB,毕业论文 模拟通信系统抗噪声性能分析
- 九九乘法表打印Python
- 机器学习可以应用在哪些场景
- 凯撒加密,已知偏移量和未知偏移量解密
- 快速分割多个视频,生成每个视频的m3u8
- 传微信要开直播业务:主流社交工具全面杀入直播战场
- 用php做一个相册的程序,搭建自己的相册 50个免费的相册程序(上)
- 上海市区广场、商场、大厦中英文对照大全
- HTML吸引人眼球的网页,超吸引眼球的优秀网站设计欣赏
- [机器学习] 树模型(xgboost,lightgbm)特征重要性原理总结
- html,css学习笔记(第四天)
热门文章
- geetest php,GitHub - lilwil/geetest: Geetest For ThinkPHP5
- java版京东抢购秒杀商品
- Android输入法挤乱布局问题
- JOS学习笔记(八)
- AVplayer的使用详解 完整注释
- iOS开发之弱网测试
- PTA 1015 德才论 (25分) ,满分代码 + 测试点报错解决
- Jarvis OJ 软件密码破解-1
- 一篇感人至深的授权协议
- c语言中错误c2228,error C2228:left of '.Push' must have class/struct/union type