使用python学线性代数_最简单的神经网络简介| 使用Python的线性代数
使用python学线性代数
A neural network is a powerful tool often utilized in Machine Learning because neural networks are fundamentally very mathematical. We will use our basics of Linear Algebra and NumPy to understand the foundation of Machine Learning using Neural Networks. Our article is a showcase of the application of Linear Algebra and, Python provides a wide set of libraries that help to build our motivation of using Python for machine learning.
神经网络是机器学习中经常使用的强大工具,因为神经网络从根本上说是非常数学的。 我们将使用线性代数和NumPy的基础知识来理解使用神经网络进行机器学习的基础。 我们的文章展示了线性代数的应用,Python提供了广泛的库,有助于建立我们使用Python进行机器学习的动机。
The figure is showing the simplest neural network of two input nodes and one output node.
该图显示了具有两个输入节点和一个输出节点的最简单的神经网络。
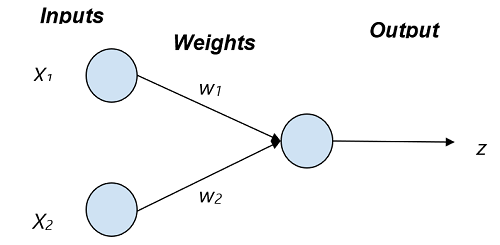
Simplest Neural Network: 2 Input - 1 Output Node
最简单的神经网络:2输入-1输出节点
Input to the neural network is X1 and X2 and their corresponding weights are w1 and w2 respectively. The output z is a tangent hyperbolic function for decision making which have input as sum of products of Input and Weight. Mathematically,
输入到神经网络的是X 1和X 2 ,它们相应的权重分别是w 1和w 2 。 输出z是用于决策的正切双曲函数,其输入为输入与权重的乘积之和。 数学上
z = tanh(X1w1 + X2w2)
Where, tanh() is an tangent hyperbolic function because it is one of the most used decision making functions.
其中, tanh()是切线双曲函数,因为它是最常用的决策函数之一。
So for drawing this mathematical network in a python code by defining a function neural_network( X, W). Note: The tangent hyperbolic function takes input within range of 0 to 1.
因此,通过定义函数Neuro_network(X,W)以python代码绘制此数学网络。 注意:正切双曲函数的输入范围为0到1。
Parameter(s):
参数:
Vector X = [[X1][X2]] and W = [[w1][w2]]
Return value:
返回值:
A value ranging between 0 and 1, as a prediction of the neural network based on the inputs.
一个介于0到1之间的值,作为基于输入的神经网络的预测。
Application:
应用:
Machine Learning
机器学习
Computer Vision
计算机视觉
Data Analysis
数据分析
Fintech
金融科技
# Linear Algebra and Neural Network
# Linear Algebra Learning Sequence
# Simplest Neural Network for 2 input 1 output node
import numpy as np
# Use of np.array() to define an Input Vector
V = np.array([.323,.432])
print("The Vector A : ",V)
# defining Weight Vector
VV = np.array([.3,.63,])
print("\nThe Vector B : ",VV)
# defining a neural network for predicting an
# output value
def neural_network(inputs, weights):
wT = np.transpose(weights)
elpro = wT.dot(inputs)
# Tangent Hyperbolic Function for Decision Making
out = np.tanh(elpro)
return out
outputi = neural_network(V,VV)
# printing the expected output
print("Expected Output of the given Input data and their respective Weight : ", outputi)
Output:
输出:
The Vector A : [0.323 0.432]
The Vector B : [0.3 0.63]
Expected Output of the given Input data and their respective Weight : 0.35316923056117167
翻译自: https://www.includehelp.com/python/introduction-to-simplest-neural-network.aspx
使用python学线性代数
使用python学线性代数_最简单的神经网络简介| 使用Python的线性代数相关推荐
- 扇贝python学完_爬虫:爬取扇贝上python常用单词,减少登陆和贝壳的繁琐
import requests import re file = open("vocabulary.doc", "w", encoding="utf- ...
- 用python学编程_用Python学编程
第1部分 引 论 第1章 关于本书 1.1 什么人要学编程 1.2 本书的内容 1.3 为什么选择Python 1.4 如何阅读本书 1.5 本书内容的组织 第2章 学习编程的要求 2.1 关于编程者 ...
- python初中可以学吗_初中学历零基础想转行Python,能否学会?Python难吗?
肯定的回答您:这个是一个技术工种学历不是很重要,完全能学会 其实,很多个程序员都是从不会到会,每种知识也是从基础到复杂,大家都是从零基础开始的,有的学员英语和数字基础也很差,从一点都不了解编码到现在非 ...
- python http服务器_超简单的Python HTTP服务
超如果你急需一个简单的Web Server,但你又不想去下载并安装那些复杂的HTTP服务程序,比如:Apache,ISS等.那么, Python 可能帮助你.使用Python可以完成一个简单的内建 H ...
- python 字符串拼接_面试官让用 3 种 python 方法实现字符串拼接 ?对不起我有8种……...
点击上方 蓝字关注我们 点击上方"印象python",选择"星标"公众号重磅干货,第一时间送达!之前发过很多关于 Python 学习的文章,收到大家不少的好评, ...
- python入门教授_南开大学教授强力推荐的5本Python入门书籍,附电子版
筛选了2年内优秀的python书籍,个别经典的书籍扩展到5年内. python现在的主流版本是3.7(有明显性能提升,强烈推荐) 3.6, 不基于这两个或者更新版本的书,慎重选择.很多库已经不提供py ...
- 阿里云大学python教程下载_阿里大学开放 11 门免费 Python 视频课程
Python 语言近几年越来越火,语言使用率占比节节攀升. 我们知道Python 现在稳居世界编程语言前三名,在 PYPL 语言流行指数上更是稳居第一,可见 python 的适用范围.受众基础.影响力 ...
- 初识python 教学设计_青岛版八年级《初识Python》教学设计.doc
PAGE PAGE 2 第4课 初识Python教学设计 [教学目标] 1.知识与技能:了解python编程语言起源以及应用,知道python编辑器的常用用法,掌握利用python编写程序的一般步骤. ...
- python画图视频_你能分享比较全面的Python视频教程吗?谢谢《用python画图教程视频》...
python好学吗? python相对于C 来说确实是好学的多. python不需要特别关注类型,因此不需要花费太多的时间在变量类型上面.python的语法相比于C 来说,要简单的多. 如何用pyth ...
最新文章
- Keil uVision5 之 C51 与 MDK 共存
- dw java 编码_dW 编辑推荐:Java 8 习惯用语,第 4 部分:提倡使用有帮助的编码方式...
- 使用mpvue开发小程序
- 虚拟主机中,不修改IIS设置,在IIS6下运行MVC架构的网站
- UE4 移动平台游戏开发
- 基于JAVA+SpringBoot+Mybatis+MYSQL的小区物业管理系统
- Alyona and copybooks
- FreeWheel业务系统微服务化过程经验分享
- splice slice
- 文件被误删不需要绝望,EasyRecovery送你时光机
- 记录PHP错误日志 display_errors与log_errors的区别
- [R语言统计]频数表
- 如何制作通讯录vcf_批量信息从表格导入手机“通讯录”
- 计算机文化与社会发展
- android 人脸 动画表情包,天呐 原来动画角色的面部表情是这样做出来的
- JAVA Exception Handing
- Browserslist: caniuse-lite is outdated. Please run next command `npm update caniuse-lite browserslis
- linux中断子系统(基于imx6ul arm32分析)
- 计算机网络(3)--应用层协议--HTTP与HTTPS
- 判断图有无环_21考研有机化学打卡第四题——芳香性判断