centos 开发套件_替代的Laravel套件开发工作流程
centos 开发套件
This article was peer reviewed by Francesco Malatesta. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
本文由Francesco Malatesta进行同行评审。 感谢所有SitePoint的同行评审人员使SitePoint内容达到最佳状态!
Every framework gives developers a way to extend the system using packages / extensions. We can generally hook in our logic at any point where we want to provide specific functionality, and Laravel is no exception! Following the article of my fellow author Francesco Malatesta about his Laravel package development workflow, I noticed that mine is a bit different and I wanted to share it with you!
每个框架都为开发人员提供了使用包/扩展来扩展系统的方法。 通常,我们可以在希望提供特定功能的任何地方加入我们的逻辑,Laravel也不例外! 下面的文章我的同胞作家弗朗西斯马爱德他Laravel包的开发流程,我注意到我的是一个有点不同,我想与大家分享吧!
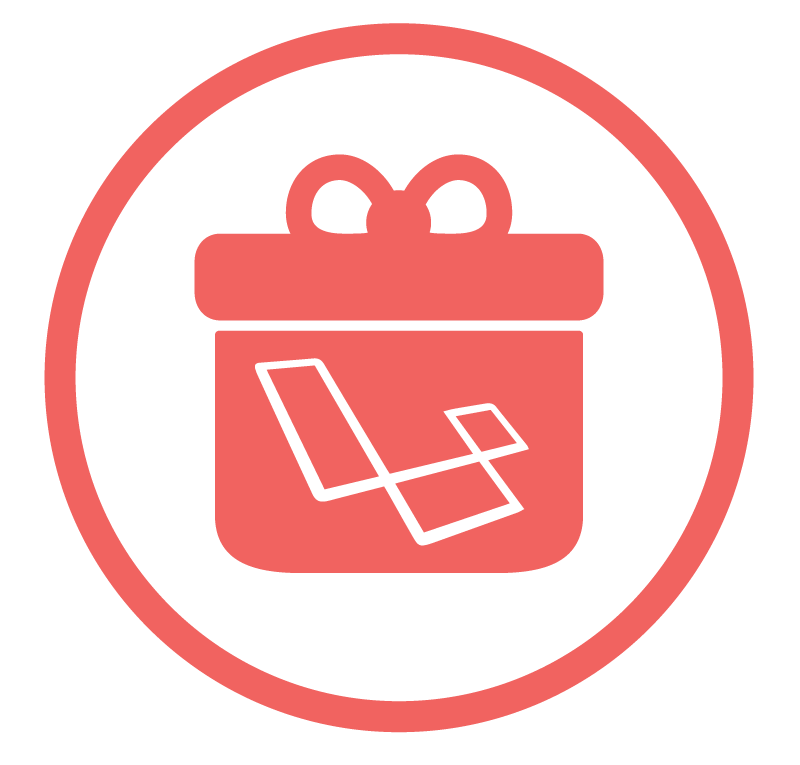
演示包 (Demo Package)
As a demo package, we’re going to build a Laravel two factor authentication package for this article. The package will handle the user authentication with a verification code being sent through a medium like Nexmo, Twilio, etc. You can check out the final package here.
作为一个演示程序包,我们要建立一个Laravel双因素身份验证软件包这一文章 。 该软件包将通过通过Nexmo , Twilio等介质发送的验证码来处理用户身份验证。您可以在此处查看最终软件包。
设置存储库 (Setting up the Repository)
Before we start the development, we need to create a new repository inside GitHub so we can push/pull from it through the development phase.
在开始开发之前,我们需要在GitHub内创建一个新的存储库,以便我们可以在开发阶段将其推入/拉出。
Composer supports a repositories
key inside the composer.json
file. We can use this to specify our custom packages that don’t yet exist on Packagist.
Composer在composer.json
文件中支持repositories
密钥。 我们可以使用它来指定Packagist尚不存在的自定义软件包。
{
// ....
"repositories": [
{
"type": "vcs",
"url": "https://github.com/Whyounes/laravel-two-factor-auth-demo"
}
],
// ....
}
Now, we can require our package as usual.
现在,我们可以照常要求包装。
{
// ....
"require": {
"php": ">=5.6.4",
"laravel/framework": "5.4.*",
"laravel/tinker": "~1.0",
"Whyounes/laravel-two-factor-auth-demo": "dev-master"
},
// ....
}
We can specify a branch or a version number. In case we want to use a branch, it should be prefixed with dev-
. You can read more about configuring repositories on the Packagist website.
我们可以指定分支或版本号。 如果我们要使用分支,则应在其前面加上dev-
。 您可以在Packagist网站上阅读有关配置存储库的更多信息 。
包装骨架 (Package Skeleton)
Since Composer identifies packages using the composer.json
file, we need to create one for our package and push it to the repository.
由于Composer使用composer.json
文件识别软件包,因此我们需要为我们的软件包创建一个并将其推送到存储库。
{
"name": "whyounes/laravel-two-factor-auth",
"type": "library",
"description": "Laravel two factor authentication",
"keywords": [
"auth",
"passwordless"
],
"homepage": "https://github.com/whyounes/laravel-two-factor-auth",
"license": "MIT",
"authors": [
{
"name": "Rafie Younes",
"email": "younes.rafie@gmail.com",
"homepage": "http://younesrafie.com",
"role": "Developer"
}
],
"require": {
"php": "^5.5.9 || ^7.0",
"illuminate/database": "5.1.* || 5.2.* || 5.3.* || 5.4.*",
"illuminate/config": "5.1.* || 5.2.* || 5.3.* || 5.4.*",
"illuminate/events": "5.1.* || 5.2.* || 5.3.* || 5.4.*",
"illuminate/auth": "5.1.* || 5.2.* || 5.3.* || 5.4.*",
"twilio/sdk": "^5.4"
},
"require-dev": {
"phpunit/phpunit": "^4.8 || ^5.0",
"orchestra/testbench": "~3.0",
"mockery/mockery": "~0.9.4"
},
"autoload": {
"psr-4": {
"Whyounes\\TFAuth\\": "src"
},
"classmap": [
"tests"
]
}
}
After running the composer update
command inside the Laravel project’s root folder, we see an error that no tests were found under the tests
folder. This won’t halt the installation process, so we’re fine to continue!
在Laravel项目的根文件夹中运行composer update
命令后,我们看到一个错误,即在tests
文件夹下找不到任何测试。 这不会停止安装过程,因此我们可以继续!
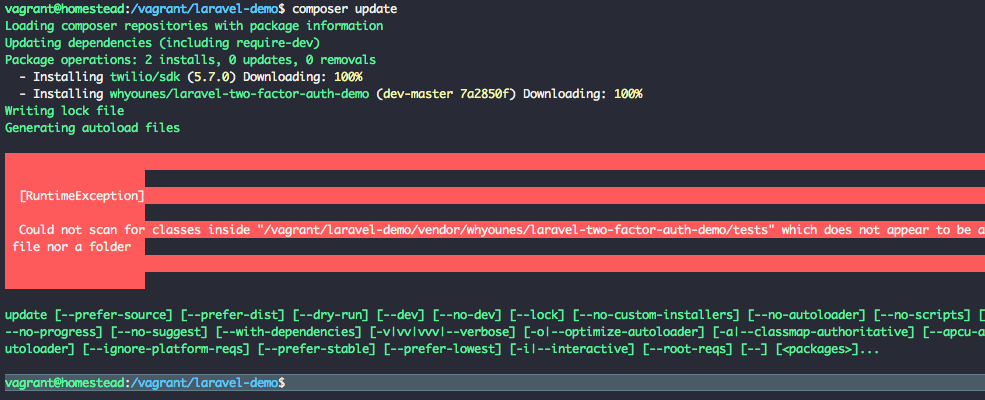
The last part of our package configuration is to link the downloaded package to the GitHub repository.
软件包配置的最后一部分是将下载的软件包链接到GitHub存储库。
// assuming you're inside your project folder
cd vendor/whyounes/laravel-two-factor-auth
git init origin git@github.com:Whyounes/laravel-two-factor-auth.git
git commit -am "Init repo"
git push origin master -f // force push for the first time
包装结构 (Package Structure)
This part is a little bit controversial, we can’t all agree on one single structure. Here’s our package structure:
这部分有一点争议,我们不能在一个单一的结构上达成一致 。 这是我们的包结构:
▶ config/
auth.php
services.php
▶ migrations/
2014_10_1_000000_add_tfa_columns_to_users_table.php
2016_12_1_000000_create_tokens_table.php
▶ resources/
▶ views/
verification_code.blade.php
▶ src/
▶ Contracts/
VerificationCodeSenderInterface.php
▶ Controllers/
TFAController.php
▶ Exceptions/
TFANotEnabledException.php
UserNotFoundException.php
▶ Models/
TFAuthTrait.php
Token.php
▶ Providers/
TwoFAProvider.php
▶ Services/
Twilio.php
▶ tests/
To install the package we use a Laravel provider:
要安装软件包,我们使用Laravel提供程序:
// config/app.php
// ...
'providers' => [
// ...
Whyounes\TFAuth\TwoFAProvider::class,
};
The provider class looks like this:
提供程序类如下所示:
// src/Providers/TwoFAProvider.php
namespace Whyounes\TFAuth;
use Illuminate\Auth\Events\Authenticated;
use Illuminate\Routing\Router;
use Illuminate\Support\Facades\App;
use Illuminate\Support\Facades\Event;
use Illuminate\Support\ServiceProvider;
use Twilio\Rest\Client;
use Whyounes\TFAuth\Contracts\VerificationCodeSenderInterface;
use Whyounes\TFAuth\Services\Twilio;
/**
* Class TwoFAProvider
*
* @package Whyounes\TFAuth
*/
class TwoFAProvider extends ServiceProvider
{
public function boot()
{
$this->loadMigrationsFrom(__DIR__ . '/../../migrations');
$this->mergeConfigFrom(
__DIR__ . '/../../config/auth.php', 'auth'
);
$this->mergeConfigFrom(
__DIR__ . '/../../config/services.php', 'services'
);
$this->loadViewsFrom(__DIR__.'/resources/views', 'tfa');
$this->publishes([
__DIR__.'/../../resources/views' => resource_path('views/vendor/tfa'),
]);
}
public function register()
{
$this->registerBindings();
$this->registerEvents();
$this->registerRoutes();
}
public function registerBindings()
{
$this->app->singleton(Client::class, function () {
return new Client(config('services.twilio.sid'), config('services.twilio.token'));
});
$this->app->bind(VerificationCodeSenderInterface::class, Twilio::class);
}
public function registerEvents()
{
// Delete user tokens after login
if (config('auth.delete_verification_code_after_auth', false) === true) {
Event::listen(
Authenticated::class,
function (Authenticated $event) {
$event->user->tokens()
->delete();
}
);
}
}
public function registerRoutes()
{
/** @var $router Router */
$router = App::make("router");
$router->get("/tfa/services/twilio/say/{text}", ["as" => "tfa.services.twilio.say", "uses" => function ($text) {
$response = "<Response><Say>" . $text . "</Say></Response>";
return $response;
}]);
}
}
Let’s go through every method here:
让我们在这里浏览每种方法:
The
boot
method will be publishing our package assets (config, migrations, views).boot
方法将发布我们的程序包资产(配置,迁移,视图)。The
registerBindings
method binds theVerificationCodeSenderInterface
to a specific implementation. The package uses the Twilio service as a default, so we create asrc/Services/Twilio
class which implements our interface and defines the necessary methods.registerBindings
方法将VerificationCodeSenderInterface
绑定到特定的实现。 该软件包默认使用Twilio服务,因此我们创建了一个src/Services/Twilio
类,该类实现了我们的接口并定义了必要的方法。
// src/Services/Twilio.php
namespace Whyounes\TFAuth\Services;
use Twilio\Rest\Client;
use Whyounes\TFAuth\Contracts\VerificationCodeSenderInterface;
class Twilio implements VerificationCodeSenderInterface
{
/**
* Twilio client
*
* @var Client
*/
protected $client;
/**
* Phone number to send from
*
* @var string
*/
protected $number;
public function __construct(Client $client)
{
$this->client = $client;
$this->number = config("services.twilio.number");
}
public function sendCodeViaSMS($code, $phone, $message = "Your verification code is %s")
{
try {
$this->client->messages->create($phone, [
"from" => $this->number,
"body" => sprintf($message, $code)
]);
} catch (\Exception $ex) {
return false;
}
return true;
}
public function sendCodeViaPhone($code, $phone, $message = "Your verification code is %s")
{
try {
$this->client->account->calls->create(
$phone,
$this->number,
["url" => route('tfa.services.twilio.say', ["text" => sprintf($message, $code)])]
);
} catch (\Exception $ex) {
return false;
}
return true;
}
// getters and setter
}
The
registerEvents
method will delete the previously used token upon authentication if the config value is set totrue
.如果config值设置为
true
,则registerEvents
方法将在身份验证后删除以前使用的令牌。The
registerRoutes
method adds a route for sending a verification code via a phone call.registerRoutes
方法添加了用于通过电话发送验证码的路由。
If, for example, we wanted to switch from Twilio to Nexmo, we only need to create a new class that implements the VerificationCodeSenderInterface
and bind it to the container.
例如,如果我们想从Twilio切换到Nexmo,我们只需要创建一个新类来实现VerificationCodeSenderInterface
并将其绑定到容器即可。
Finally, we add a trait to the User
model class:
最后,我们向User
模型类添加一个特征:
// app/User.php
class User extends Authenticatable
{
use \Whyounes\TFAuth\Models\TFAuthTrait;
// ...
}
测验 (Tests)
Tests are mandatory before releasing any code publicly, not just for Laravel. DO NOT use any packages that don’t have tests. For Laravel, you may use the orchestra/testbench
package to help you out, and the documentation provides a really nice flow for testing Laravel components like Storage
, Eloquent
, etc.
在公开发布任何代码之前(不仅限于Laravel),必须进行测试。 不要使用任何没有测试的软件包。 对于Laravel,您可以使用orchestra/testbench
方案,帮助你,和文档提供了用于测试Laravel组件就像一个非常好的流动Storage
, Eloquent
等
For our package, we have tests for our controller, model and service classes. Check the repository for more details about our tests.
对于我们的程序包,我们对控制器,模型和服务类进行了测试。 检查存储库以获取有关我们测试的更多详细信息。
标记 (Tagging)
We can’t keep just a master branch for our package. We need to tag a first version to start with. This depends on how far along are we in the feature development stage, and what the goal is here!
我们不能只为包保留master分支。 我们需要标记一个开始的版本。 这取决于我们在功能开发阶段的进展情况以及目标是什么!
Since our package is not that complicated and doesn’t have a lot of features to build, we can tag it as a first stable version (1.0.0
). You can check the Git documentation website for more details about tagging, and here’s the list of commands for adding a new tag to our repository:
由于我们的软件包不是那么复杂,并且没有很多功能要构建,因此我们可以将其标记为第一个稳定版本( 1.0.0
)。 您可以访问Git文档网站以获取有关标记的更多详细信息,这是用于将新标记添加到我们的存储库的命令列表:
git tag
git tag -a v1.0.0 -m "First stable version"
git push origin --tags
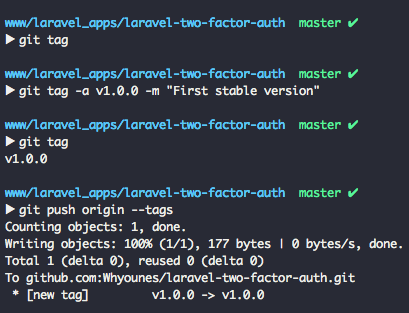
We should now see the tag on GitHub under the branch select box:
现在,我们应该在分支选择框下的GitHub上看到标签:
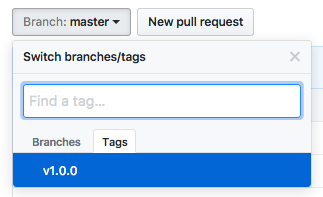
持续集成 (Continuous Integration)
Continuous Integration tools help us integrate new parts into our existing code and send a notification about any infringements. It also gives us a badge (image with a link) for the status of the code. For this package, we use TravisCI as our CI tool.
持续集成工具可帮助我们将新零件集成到现有代码中,并发送有关任何侵权的通知。 它还为我们提供了代码状态的标志(带有链接的图像)。 对于此软件包,我们使用TravisCI作为CI工具。
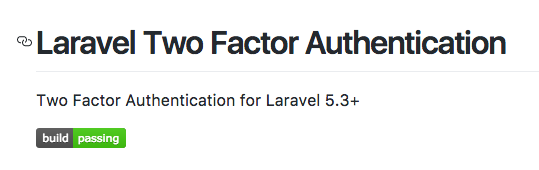
// .travis.yml
language: php
php:
- 5.6
- 7.0
- 7.1
sudo: true
install:
- travis_retry composer install --no-interaction --prefer-source
This config file will be picked up by TravisCI to know how to test the code and what versions to test on. You can read more about how to integrate it here.
TravisCI将提取此配置文件,以了解如何测试代码以及要测试的版本。 您可以在此处阅读有关如何集成它的更多信息。
结论 (Conclusion)
The development workflow is not that complicated. In fact, we can develop a package without needing to install the Laravel framework; just include the necessary Illuminate
components and test drive your package’s implementation. At the end, you can do an integration test as a demo that the package is working as expected. I hope this helps you create some packages to give back to the community :)
开发工作流程并不那么复杂。 实际上,我们可以开发一个软件包,而无需安装Laravel框架。 仅包括必要的Illuminate
组件并测试驱动程序包的实现。 最后,您可以进行集成测试,以演示该软件包是否按预期工作。 我希望这可以帮助您创建一些可以回馈社区的软件包:)
If you have any questions or comments you can post them below! What’s your package development workflow?
如果您有任何疑问或意见,可以在下面发布! 您的包装开发工作流程是什么?
翻译自: https://www.sitepoint.com/alternative-laravel-package-development-workflow/
centos 开发套件
centos 开发套件_替代的Laravel套件开发工作流程相关推荐
- 华为ai开发套件_使用华为ml套件图像分割构建背景橡皮擦应用
华为ai开发套件 Image segmentation is a widely used term in image processing and computer vision world. It ...
- sdn智能互联系统及开发平台_聊天交友平台系统APP开发
点击上面"蓝字"关注我们! 聊天交友系统开发APP软件平台找[卢经理:186微1316电8035同号], 聊天交友短视频APP开发,聊天交友短视频软件APP开发系统,聊天交友开发A ...
- 6天移动web开发视频教程_针对Web和移动开发人员的完整视频解决方案
6天移动web开发视频教程 This article was originally published on Cloudinary Blog. Thank you for supporting the ...
- 移动应用开发实例_物联网改变移动应用开发的4种方式
图片来源:pixabay.com 来源:物联之家网(iothome.com) 转载请注明来源! 物联网改变了移动应用程序的开发格局.那么,为物联网开发移动应用程序有何不同? 物联网与移动应用程序开发齐 ...
- python人工智能开发语言_哪些编程语言最适合开发人工智能?
这两年,"一只狗"AlphaGo打遍天下棋手无对手,让我们认识了什么叫人工智能.在刚刚过去的IT领袖峰会上,BAT三位大佬都表示看好人工智能的未来发展.今年年初,百度就做了一个大动 ...
- 中南大学杰出校友_杰出PHP社区成员的工作流程是什么?
中南大学杰出校友 Workflow refers to both the process and the tools that are used in this process. Almost eve ...
- javascript控制台_如何使用JavaScript控制台改善工作流程
javascript控制台 by Riccardo Canella 里卡多·卡内拉(Riccardo Canella) 如何使用JavaScript控制台改善工作流程 (How you can imp ...
- 绿色数据中心空调设计 书评_书评:响应式设计工作流程
绿色数据中心空调设计 书评 Responsive design has many challenges. Performance. Tables. Images. Content. Testing. ...
- iMeta | 大连海洋大学傅松哲和根特大学杨倩开发宏基因组测序和流式细胞术相结合的工作流程...
点击蓝字 关注我们 宏基因组测序结合流式细胞术为城市污水中细菌病原体的微生物风险评估提供了新的框架 原文链接DOI: https://doi.org/10.1002/imt2.77 COMMENTAR ...
最新文章
- 【markdown】图片的处理
- 2017-2018-2 20155303『网络对抗技术』Final:Web渗透获取WebShell权限
- Git++ - 有趣的命令
- 非常详尽的 Shiro 架构解析!
- swd脱机烧录器及上位机源码_通用上位机框架HwLib.Automation(C#)
- StringUtils类中 isEmpty() 与 isBlank()的区别
- Android中Activity和task,活动亲和力,启动模式,活动状态以及生命周期,激活钝化
- R 语言学习过程全记录 ~
- android studio 发布版本,Android Studio 4.1 Canary 版本发布
- Java入门系列-26-JDBC
- nyoj 586 疯牛(二分+贪心)
- linux怎么修改bash,Linux操作系统中如何对Bash变量内容修改?
- idea打不了断点红点没对号_你可能不知道的 IDEA 高级调试技巧
- 计算机信息处理工具教案设计,《信息和信息处理工具》教案设计
- php点广告送积分,PHP猜一猜奇偶商城积分促销模式
- 偏微分方程数值解的matlab 实现,偏微分方程数值解的Matlab 实现
- android gms包
- 计算机已锁定的时候鼠标不能用,鼠标驱动正常但是不能用怎么回事_电脑鼠标驱动正常但是不能用怎么解决...
- python 把京东订单,推送到测试环境,提供便利
- echarts 直方图加正态_直方图和正态分布图(只需填入待分析数据_自动分析_自动生成图)...