geometry-api-java 学习笔记(八)分割Intersection
一个简单的例子
Let's look at a couple of simple examples. In the following images, the blue geometry is the intersectorand the green geometries are the input geometries. The intersector is paired with each of the inputgeometries to output the red geometries.
![]() |
![]() |
![]() |
![]() |
Intersector and Inputs | Dimension mask = -1 | Dimension mask = 3 | Dimension mask = 6 |
调用Intersection 操作
这里有2种方法:
There are two ways to call the Intersection operator as there are two execute
methods. Oneof the execute
methods doesn't have a mask
parameter. By calling this method,we are essentially setting the dimension mask equal to -1. Recall that this implies that the returned geometry willbe the lowest dimension of the intersecting pair.
If we are not using a dimension mask, the call looks like this:
GeometryCursor outputGeoms = OperatorIntersection.local().execute(inputGeoms, intersector, spatialRef, null);
If we are using a dimension mask, the call looks like this:
GeometryCursor outputGeoms = OperatorIntersection.local().execute(inputGeoms, intersector, spatialRef, null, mask);
where inputGeoms
and intersector
are of type GeometryCursor
.
Then to retrieve the intersected geometries from the cursor, our code will look something like this:
Geometry geometry = null;while ((geometry = outputGeoms.next()) != null) {... do something }
Understanding the output
The number of output geometries for each input pair depends on the dimension mask. If the dimension mask isequal to -1, then there will be one output geometry for each input pair. If the dimension mask is greater than0, then for each input pair there will be the same number of output geometries as is specified in the dimensionmask. Some of the output geometries may be empty.
To better understand the output, we will look at the output generated by the application shown below.The application creates the geometries we looked at previously and calls intersection with variousdimension masks. It prints out the JSON format of the output geometries.
package geometryapp;import com.esri.core.geometry.Geometry; import com.esri.core.geometry.GeometryCursor; import com.esri.core.geometry.OperatorExportToJson; import com.esri.core.geometry.OperatorIntersection; import com.esri.core.geometry.Polygon; import com.esri.core.geometry.Polyline; import com.esri.core.geometry.SimpleGeometryCursor; import com.esri.core.geometry.SpatialReference; import java.util.ArrayList;/** This program creates an intersector polygon, two input polylines and two input* polygons. It then calls the Intersection operator with three different* dimension masks. Each time the Intersection operator is called, it pairs up* the intersector polygon with each of the input geometries. For each pair the* dimension of the output is determined by the given dimension mask.* -1 => lowest dimension of input pair* 3 => output multipoints (1) and polylines (2) (1 + 2 = 3)* 6 => polylines (2) and polygons (4) (2 + 4 = 6)*/public class IntersectionApp {public static void main(String[] args) {// Create intersector.double coords[][] = {{0,4},{2,10},{8,12},{12,6},{10,2},{4,0}};Polygon poly = createPolygon(coords);// Create the input polylines.ArrayList<Geometry> geomList = createAllInputs();// Create a spatial reference object for GCS_WGS_1984.SpatialReference sr = SpatialReference.create(4326);System.out.println("Intersector");printJSONGeometry(sr, poly);System.out.println("Input Geometries");for (Geometry geom : geomList) {printJSONGeometry(sr, geom);}// Let's try it with different dimension masks.int mask[] = {-1, 3, 6};for (int i = 0; i < 3; i++) {SimpleGeometryCursor inGeoms = new SimpleGeometryCursor(geomList);SimpleGeometryCursor intersector = new SimpleGeometryCursor(poly);GeometryCursor outGeoms = OperatorIntersection.local().execute(inGeoms, intersector, sr, null, mask[i]);System.out.println("*******Dim mask: " + Integer.toString(mask[i]) + "*******");// Get the geometries from the cursor and print them in JSON format.Geometry geom = null;while((geom = outGeoms.next()) != null) {printJSONGeometry(sr, geom);}}}public static ArrayList<Geometry> createAllInputs() {// Create a list of input geometries.ArrayList<Geometry> geomList = new ArrayList<Geometry>(4);// Polyline 1double coords[][] = {{1,15},{3.5,10.5},{6.5,11.5},{18,11.5}};geomList.add(createPolyline(coords));// Polyline 2double coords2[][] = {{-2,10},{1,7}};geomList.add(createPolyline(coords2));// Polygon 3double coords3[][] = {{8,8},{10,10},{14,10},{16,8},{16,4},{14,2},{10,2},{8,4}};geomList.add(createPolygon(coords3));// Polygon 4double coords4[][] = {{1,3},{3,1},{0,0}};geomList.add(createPolygon(coords4));return geomList;}public static Polyline createPolyline(double[][] pts) {Polyline line = new Polyline();line.startPath(pts[0][0], pts[0][1]);for (int i = 1; i < pts.length; i++)line.lineTo(pts[i][0], pts[i][1]);return line;}public static Polygon createPolygon(double[][] pts) {Polygon poly = new Polygon();poly.startPath(pts[0][0], pts[0][1]);for (int i = 1; i < pts.length; i++)poly.lineTo(pts[i][0], pts[i][1]);return poly;}public static void printJSONGeometry(SpatialReference spatialRef, Geometry geometry) {System.out.println("Type: " + geometry.getType());// Export the geometry to JSON format to print it out.String jsonString = OperatorExportToJson.local().execute(spatialRef, geometry);System.out.println(jsonString);System.out.println();} }
Now let's look at the output a bit at a time.
First, we just print the intersector and the input geometries so we can see the coordinates.
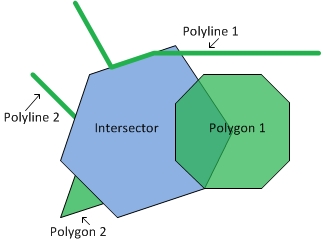
Intersector Type: Polygon {"rings":[[[0.0,4.0],[2.0,10.0],[8.0,12.0],[12.0,6.0],[10.0,2.0],[4.0,0.0],[0.0,4.0]]],"spatialReference":{"wkid":4326}}Input Geometries Type: Polyline {"paths":[[[1.0,15.0],[3.5,10.5],[6.5,11.5],[18.0,11.5]]],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[[[-2.0,10.0],[1.0,7.0]]],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[[[8.0,8.0],[10.0,10.0],[14.0,10.0],[16.0,8.0],[16.0,4.0],[14.0,2.0],[10.0,2.0],[8.0,4.0],[8.0,8.0]]],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[[[1.0,3.0],[3.0,1.0],[0.0,0.0],[1.0,3.0]]],"spatialReference":{"wkid":4326}}
The first dimension mask is equal to -1. Because the intersector is a polygon, if the input is a polygon, thenthe output will be a polygon. If the input is a polyline, then the output will be a polyline.There will be one output for each input geometry.
Notice that the second output polyline is empty. This is because the intersection of the intersector polygon andPolyline 2 is a point, not a polyline. Similarly, the second output polygon is empty because theintersection of the intersector polygon and Polygon 2 is a polyline, not a polygon.
*******Dim mask: -1******* Type: Polyline {"paths":[[[3.5,10.5],[6.5,11.5],[8.333333333333334,11.5]]],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[[[10.0,2.0],[8.0,4.0],[8.0,8.0],[9.6,9.6],[12.0,6.0],[10.0,2.0]]],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[],"spatialReference":{"wkid":4326}}
Next, we use a dimension mask equal to 3. This signifies that we want to find point/multipoint and polylineintersections. Therefore, we will have two output geometries for each input geometry.
Notice that the only multipoint that isn't empty is that which was output as the intersection of the intersectorand Polyline 2.
*******Dim mask: 3******* Type: MultiPoint {"points":[],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[[[3.5,10.5],[6.5,11.5],[8.333333333333334,11.5]]],"spatialReference":{"wkid":4326}}Type: MultiPoint {"points":[[1.0,7.0]],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[],"spatialReference":{"wkid":4326}}Type: MultiPoint {"points":[],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[],"spatialReference":{"wkid":4326}}Type: MultiPoint {"points":[],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[[[3.0,1.0],[0.9999999999999999,3.0]]],"spatialReference":{"wkid":4326}}
Finally, we examine the results when the dimension mask is equal to 6. This time we want intersections thatare polylines and polygons.
*******Dim mask: 6******* Type: Polyline {"paths":[[[3.5,10.5],[6.5,11.5],[8.333333333333334,11.5]]],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[[[10.0,2.0],[8.0,4.0],[8.0,8.0],[9.6,9.6],[12.0,6.0],[10.0,2.0]]],"spatialReference":{"wkid":4326}}Type: Polyline {"paths":[[[3.0,1.0],[0.9999999999999999,3.0]]],"spatialReference":{"wkid":4326}}Type: Polygon {"rings":[],"spatialReference":{"wkid":4326}}
geometry-api-java 学习笔记(八)分割Intersection相关推荐
- java学习笔记(八)----包,jar文件
包 //建立包后同一个文件中的类都属于这个包,所有的类都必须按包名所对应的目录,在硬盘中存放.同一个包中的类在相互调用时,是不用指定包名的. ---在编译时对于下面这个类,用这样的方法 ja ...
- 【Java学习笔记八】包装类和vector
包装类 在Java语言中,每一种基本的数据类型都有相应的对象类型,称为他们基本类型的包装类(包裹类). 字节byte:Byte.短整数型short:Short 标准整数型int:Integer.长整数 ...
- Java学习笔记(八):简单的窗体实现KNN手写体识别(借鉴)
一.KNN手写识别原理 在下图中,要判断绿色圆归属为哪个类(红三角形还是蓝四边形) 如果K=3,因为红三角形占比例为2/3,所以绿色圆归属为红色三角形: 如果K=5,因为蓝四边形比例为3/5,所以绿色 ...
- java学习笔记(二十八)——开发一个小项目(VMeeting3.0)
上篇文章按照较规范的产品需求文档梳理了项目的逻辑,感觉开发起来明晰了很多:挂上一篇文章java学习笔记(二十七)--开发一个小项目(VMeeting2.0)_Biangbangbing的博客-CSDN ...
- 黑马程序员_java自学学习笔记(八)----网络编程
黑马程序员_java自学学习笔记(八)----网络编程 android培训. java培训.期待与您交流! 网络编程对于很多的初学者来说,都是很向往的一种编程技能,但是很多的初学者却因为很长一段时间无 ...
- 《Java学习笔记(第8版)》学习指导
<Java学习笔记(第8版)>学习指导 目录 图书简况 学习指导 第一章 Java平台概论 第二章 从JDK到IDE 第三章 基础语法 第四章 认识对象 第五章 对象封装 第六章 继承与多 ...
- Java学习笔记(原创)
Java学习笔记(原创) 2011-12-01 16:37:00| 分类: Java|举报|字号 订阅 下载LOFTER客户端 基本知识 一. Java基础 1. java语言的特点: ①简单:没有 ...
- java学习笔记13--反射机制与动态代理
本文地址:http://www.cnblogs.com/archimedes/p/java-study-note13.html,转载请注明源地址. Java的反射机制 在Java运行时环境中,对于任意 ...
- Java学习笔记(十)--控制台输入输出
输入输出 一.控制台输入 在程序运行中要获取用户的输入数据来控制程序,我们要使用到 java.util 包中的 Scanner 类.当然 Java 中还可以使用其他的输入方式,但这里主要讲解 Scan ...
- java学习笔记16--I/O流和文件
本文地址:http://www.cnblogs.com/archimedes/p/java-study-note16.html,转载请注明源地址. IO(Input Output)流 IO流用来处理 ...
最新文章
- DrugAI | 抗新型冠状病毒药物榜单解析
- origin+matlab基础绘图
- 用数学方式打开Facebook新Logo,真的和视频号Logo来自同一方程
- NDK 与 JNI 的关系
- 进阶指令——wc指令【作用:统计文件内容信息(包含行数、单词数、字节数)】、date指令【作用:表示操作时间日期(读取、设置)】、cal指令【作用:用来操作日历的】、clear/ctrl + L指令
- 一个判断字符是不是10进制数的函数------isdigit()
- MySQL基础之数据类型介绍
- 针对B2B平台的接口自动化测试系统
- 洛谷 P2596 [ZJOI2006]书架 (splay)
- 云服务器 怎样修改地域,云服务器 怎样修改地域
- 特征工程系列学习(零)引言
- java map 元素个数_Java 小模块之--统计字符串中元素个数
- python3使用requests和requests_toolbelt上传文件
- 【ppt课件制作】Focusky教程 | 如何设置内容全屏显示?
- matlab一元方差分析方法,多元方差分析matlab程序.doc
- 怎么修改电脑的ip地址
- 反射-获取信息详细(转)
- 2021年2月22日 星期一 农历八九 晴
- 站上新风口的区块链:虚火还是实火 到底有多神奇?
- C#dll的生成和使用
热门文章
- QT Creator应用程序开发——QT程序设计基本知识
- python笔记之while循环
- NRF24L01跳频抗信道干扰功能探讨
- 2020年大厂职级薪资一览表
- linux共享文件权限设置,linux – Windows更改Samba文件共享中的文件权限
- linux mysql cron_定时MySQL动作-Linux下用Cron现定时执行脚本
- aem是什么意思_一台400匹的宽体RX7不装转子引擎,那装的是什么?
- blob转成json js_javascript – 文件API – Blob到JSON
- python sklearn 梯度下降法_(四)梯度下降法及其python实现
- 雷蛇灯光配置文件_消费降级?不我只是体验灯光,雷蛇萨诺狼蛛V2开箱