puppeteer api_使用Node.js和Puppeteer API生成PDF文件
puppeteer api
Puppeteer is a Node library developed by Google and provides a high-level API for developers.
Puppeteer是Google开发的Node库,并为开发人员提供了高级API。
With Node.js already up and running, we will install puppeteer via NPM (node package manager).
在Node.js已经启动并运行的情况下,我们将通过NPM (节点程序包管理器) 安装puppeteer 。
Note: You should have Node.js installed in your PC.
注意:您应该在PC中安装Node.js。
To get started, let's install puppeteer:
首先,让我们安装puppeteer:
Open the command prompt and type npm i puppeteer or npm install puppeteer
打开命令提示符,然后键入npm i puppeteer或npm install puppeteer
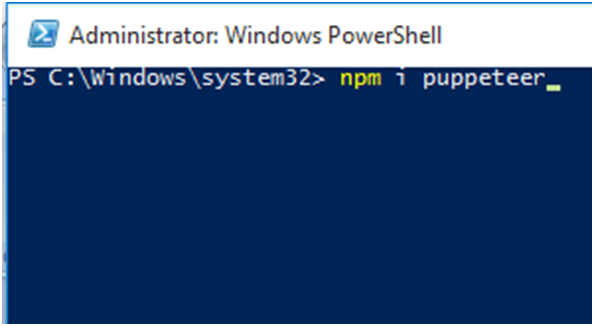
"npm" will download and install the puppeteer library together with other dependencies and Chromium.
“ npm”将下载并安装puppeteer库以及其他依赖项和Chromium。
Open a text editor and type the following code and save it with the file name as app.js
打开文本编辑器,然后输入以下代码,并将其保存为文件名app.js。
// Include puppeteer module
const puppeteer = require ('puppeteer');
// file system node js module.
const fs = require ('fs');
(async function () {try {
// launch puppeteer API
const browser = await puppeteer.launch();
const page = await browser.newPage();
// content of PDF file
await page.setContent ('WELCOME TO GOOGLE PUPPETEER API');
await page.emulateMedia ('screen');
await page.pdf ({// name of your pdf file in directory
path: 'testpdf.pdf',
// specifies the format
format: 'A4',
// print background property
printBackground: true
});
// console message when conversion is complete!
console.log ('done');
await browser.close();
process.exit();
} catch (e) {console.log ('our error', e);
}
} ) () ;
The file should be saved in your Node.js directory.
该文件应保存在您的Node.js目录中。
From the code above, we first of all include the puppeteer module and the file system module. The puppeteer API is then launched and it creates a new A4 page with file name test.pdf.
从上面的代码,我们首先包括puppeteer模块和file system模块 。 然后启动puppeteer API,它将创建一个文件名为test.pdf的新A4页面 。
Run the code by initiating it in the command prompt like a regular Node.js file.
通过像常规Node.js文件一样在命令提示符下启动代码来运行代码。
Following our code, done will be printed out when the conversion is complete.
按照我们的代码,转换完成后将打印完成。

The Output pdf file is then stored in the default Node.js directory with name test.pdf.
然后,输出pdf文件存储在默认的Node.js目录中,名称为test.pdf 。

Output PDF file...
输出PDF文件...
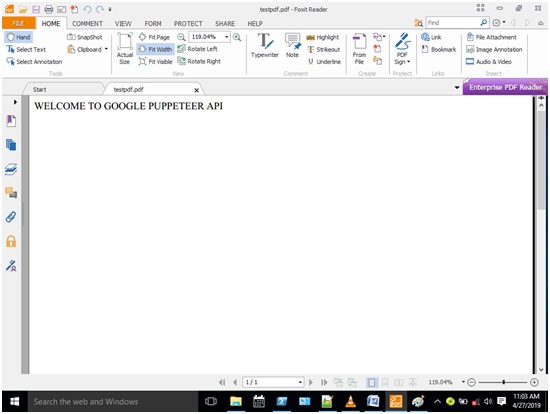
翻译自: https://www.includehelp.com/node-js/generate-pdf-file-using-node-js-and-puppeteer-api.aspx
puppeteer api
puppeteer api_使用Node.js和Puppeteer API生成PDF文件相关推荐
- puppeteer api_使用Node.js和puppeteer API从URL创建PDF文件
puppeteer api We will continue using Node.js and puppeteer which is a node library. As we saw in our ...
- puppeteer执行js_使用Node.js和Puppeteer与表单和网页进行交互– 1
puppeteer执行js Hi guys! Today let's look at another powerful function of the puppeteer API using Node ...
- node.js入门 - 9.api:http
node一个重要任务是用来创建web服务,接下来我们就学习与此相关的一个重要的api -- http.我们使用http.createServer()创建一个http服务的实例,用来处理来自客户的请求. ...
- Autodesk Forge Viewer与Forge API Node.js客户端SDK的TypeScript声明文件发布!
作为一个凝聚专(jie)业(cao)精(man)神(man)的团队(Autodesk ADN),这大过?年的岂能没有一点表示?!花式红包什么的早已化境,技术分享大家也审low疲劳了,所以我们这就本着M ...
- 有没有办法为Node.js项目自动构建package.json文件
本文翻译自:Is there a way to automatically build the package.json file for Node.js projects Is package.js ...
- 利用PDF.JS插件解决了本地pdf文件在线浏览问题(根据需要隐藏下载功能,只保留打印功能)
利用PDF.JS插件解决了本地pdf文件在线浏览问题(根据需要隐藏下载功能,只保留打印功能) 参考文章: (1)利用PDF.JS插件解决了本地pdf文件在线浏览问题(根据需要隐藏下载功能,只保留打印功 ...
- jspdf与zip.js结合。解决转pdf文件清晰度与文件过大的问题
jspdf与zip.js结合.解决转pdf文件过大的问题 一.问题产生:使用jspdf转换html到pdf清晰度不够 二.问题解决:在jspdf.debug.js源代码中下载部分集成zip.js 一. ...
- 原生JS生成PDF文件、生成pdf功能
js实现生成pdf文件 这里我主要做个记录,之前写的现在忘得差不多了,所以直接上代码 先来HTML的代码,这里因为我用的HkCms框架所以{hkcms:adv name="tctotal&q ...
- 使用pdf.js在web页面展示pdf文件
最近弄的项目中需要在线展示PDF文件,以前用的是Adobe PDF阅读器直接在浏览器端打开的,这要求客户端必须安装这个软件,若是没有安装就不能在线预览了.为了解决这个问题,最终决定用pdf.js来实现 ...
最新文章
- 当Docker遇到Intellij IDEA,再次解放了生产力~
- 演示:GLBP跟踪功能、权值、与不同的负载均衡方式
- android os一直唤醒,Android保持屏幕常亮唤醒状态
- 【小o地图Excel插件版】不止能做图表,还能抓58、大众点评网页数据...
- OpenGL之正背面剔除、深度测试与多边形偏移
- [转]ES6、ES7、ES8、ES9、ES10新特性一览 (个人整理,学习笔记)
- stm32驱动ssd1306配置_STM32 OLED 屏幕 驱动芯片SSD1306 IIC代码
- Makefile中怎么使用Shell if判断
- LeetCode 1261. 在受污染的二叉树中查找元素(树哈希)
- JavaScript 常用代码整理
- 利用python来求解网络的平均路径长度和聚类系数
- android - Unable to add window -- token null is not for an application的解决方案
- 关于Centos Linux系统安装Python的问题
- 线程---同步---快乐影院小案例
- 最简单的省市区三级联动
- The command ‘docker‘ could not be found in this WSL 2 distro.
- 货币银行学重点内容复习
- Matlab 4. Matlab2016 不能保存数据(变量)的解决方法(中文版)-v7.3 switch
- 阿里云服务器云数据库免费体验(Java Web详细实例)
- Altium designer 10安装破解以及出现缺少mfc71.dll文件的情况处理