百度charts_NBA Shot Charts:更新
百度charts
For some reason I recently got it in my head that I wanted to go back and create more NBA shot charts. My previous shotcharts used colored circles to depict the frequency and effectiveness of shots at different locations. This is an extremely efficient method of representing shooting profiles, but I thought it would be fun to create shot charts that represent a player’s shooting profile continously across the court rather than in discrete hexagons.
由于某种原因,我最近想起要创建更多的NBA投篮记录。 我以前的弹图使用彩色圆圈描绘了不同位置的拍摄频率和效果。 这是表示射击轮廓的一种非常有效的方法,但是我认为创建一个能够在球场上连续而不是离散的六边形来表示球员的射击轮廓的射击图表会很有趣。
By depicting the shooting data continously, I lose the ability to represent one dimenion – I can no longer use the size of circles to depict shot frequency at a location. Nonetheless, I thought it would be fun to create these charts.
通过连续描绘拍摄数据,我失去了表示一个维度的能力–我再也无法使用圆圈的大小来描绘某个位置的拍摄频率。 尽管如此,我认为创建这些图表会很有趣。
I explain how to create them below. I’ve also included the ability to compare a player’s shooting performance to the league average.
我在下面解释如何创建它们。 我还包括了将球员的投篮表现与联盟平均水平进行比较的功能。
In my previous shot charts, I query nba.com’s API when creating a players shot chart, but querying nba.com’s API for every shot taken in 2015-16 takes a little while (for computing league average), so I’ve uploaded this data to my github and call the league data as a file rather than querying nba.com API.
在我以前的击球图中,我在创建球员击球图时查询nba.com的API,但是对于2015-16赛季的每一次击球,查询nba.com的API都需要花费一些时间(用于计算联盟平均水平),因此我已经上传了此数据数据发送到我的github,然后将联赛数据作为文件调用,而不是查询nba.com API。
This code is also available as a jupyter notebook on my github.
此代码也可以在我的github上作为jupyter笔记本使用。
|
Here, I create a function for querying shooting data from NBA.com’s API. This is the same function I used in my previous post regarding shot charts.
在这里,我创建了一个用于从NBA.com的API查询射击数据的函数。 这与我以前的关于击球图表的帖子中使用的功能相同。
You can find a player’s ID number by going to the players nba.com page and looking at the page address. There is a python library that you can use for querying player IDs (and other data from the nba.com API), but I’ve found this library to be a little shaky.
您可以转到播放器nba.com页面并查看页面地址来找到播放器的ID号。 有一个python库可用于查询播放器ID(以及nba.com API中的其他数据),但是我发现该库有些不稳定。
|
Create a function for drawing the nba court. This function was taken directly from Savvas Tjortjoglou’s post on shot charts.
创建一个用于绘制nba球场的函数。 该功能直接取自Savvas Tjortjoglou在击球图表上的帖子 。
|
Write a function for acquiring each player’s picture. This isn’t essential, but it makes things look nicer. This function takes a playerID number and the amount to zoom in on an image as the inputs. It by default places the image at the location 500,500.
编写获取每个玩家照片的功能。 这不是必需的,但可以使事情看起来更好。 此功能将玩家ID号和放大图像的数量作为输入。 默认情况下,它将图像放置在500,500的位置。
|
Here is where things get a little complicated. Below I write a function that divides the shooting data into a 25×25 matrix. Each shot taken within the xy coordinates encompassed by a given bin counts towards the shot count in that bin. In this way, the method I am using here is very similar to my previous hexbins (circles). So the difference just comes down to I present the data rather than how I preprocess it.
这是事情变得有些复杂的地方。 在下面,我写了一个函数,将拍摄数据分为25×25矩阵。 给定仓位所包含的xy坐标内拍摄的每个镜头都计入该仓位中的镜头数量。 这样,我在这里使用的方法与我以前的六边形(圆圈)非常相似。 因此,区别只在于我呈现数据而不是预处理数据。
This function takes a dataframe with a vector of shot locations in the X plane, a vector with shot locations in the Y plane, a vector with shot type (2 pointer or 3 pointer), and a vector with ones for made shots and zeros for missed shots. The function by default bins the data into a 25×25 matrix, but the number of bins is editable. The 25×25 bins are then expanded to encompass a 500×500 space.
此函数获取一个数据帧,该数据帧的X平面上具有射击位置矢量,Y平面上具有射击位置矢量,一个具有射击类型(2指针或3指针)的矢量以及一个带有1的已拍摄镜头和零的向量错过了镜头。 默认情况下,该功能将数据合并到25×25矩阵中,但是合并数是可编辑的。 然后将25×25的箱扩展为包含500×500的空间。
The output is a dictionary containing matrices for shots made, attempted, and points scored in each bin location. The dictionary also has the player’s ID number.
输出是一个字典,其中包含用于在每个仓位中拍摄,尝试和得分的矩阵。 字典中也有玩家的ID号。
|
Below I load the league average data. I also have the code that I used to originally download the data and to preprocess it.
下面我加载联盟平均数据。 我还拥有用于最初下载数据和对其进行预处理的代码。
|
I really like playing with the different color maps, so here is a new color map I created for these shot charts.
我真的很喜欢玩不同的颜色表,因此这里是我为这些拍摄图表创建的新颜色表。
|
Below, I write a function for creating the nba shot charts. The function takes a dictionary with martrices for shots attempted, made, and points scored. The matrices should be 500×500. By default, the shot chart depicts the number of shots taken across locations, but it can also depict the number of shots made, field goal percentage, and point scored across locations.
在下面,我编写了一个用于创建nba击球图的函数。 该功能采用字典,其中包含尝试,做出和得分的矩阵。 矩阵应为500×500。 默认情况下,击球图描述的是不同地点拍摄的照片数量,但它也可以描述不同地点拍摄的照片数量,射门得分百分比和得分。
The function uses a gaussian kernel with standard deviation of 5 to smooth the data (make it look pretty). Again, this is editable. By default the function plots a players raw data, but it will plot how a player compares to league average if the input includes a matrix of league average data.
该函数使用标准偏差为5的高斯核来平滑数据(使其看起来很漂亮)。 同样,这是可编辑的。 默认情况下,该函数绘制球员原始数据,但是如果输入包含联赛平均值数据矩阵,它将绘制玩家与联赛平均值的比较关系。
|
Alright, thats that. Now lets create some plots. I am a t-wolves fan, so I will plot data from Karl Anthony Towns.
好的,就是这样。 现在让我们创建一些图。 我是T型狼迷,所以我将绘制Karl Anthony Towns的数据。
First, here is the default plot – attempts.
首先,这是默认绘图-尝试次数。
|
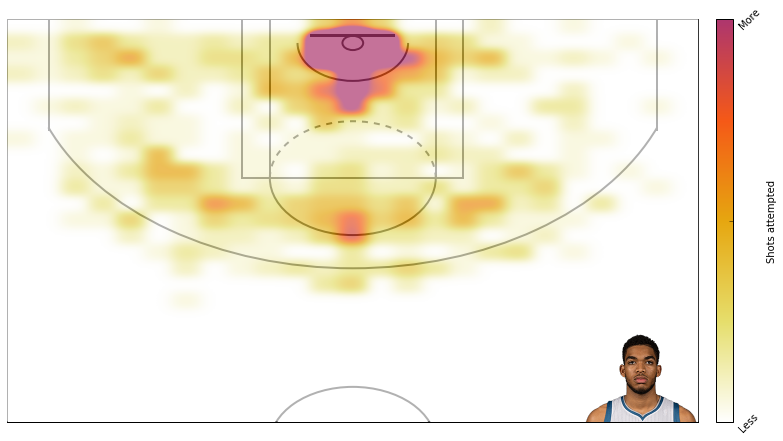
Here’s KAT’s shots made
这是KAT的镜头
|
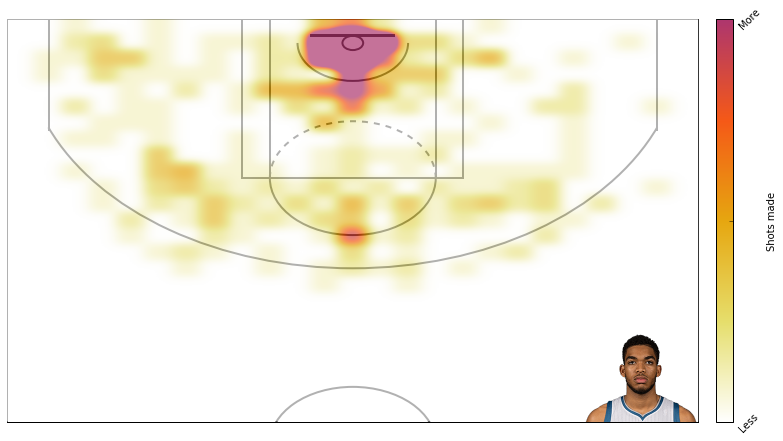
Here’s field goal percentage. I don’t like this one too much. It’s hard to use similar scales for attempts and field goal percentage even though I’m using standard deviations rather than absolute scales.
这是射门得分百分比。 我不太喜欢这个。 即使我使用标准差而不是绝对比例,也很难对尝试和射门得分百分比使用相似的比例。
|
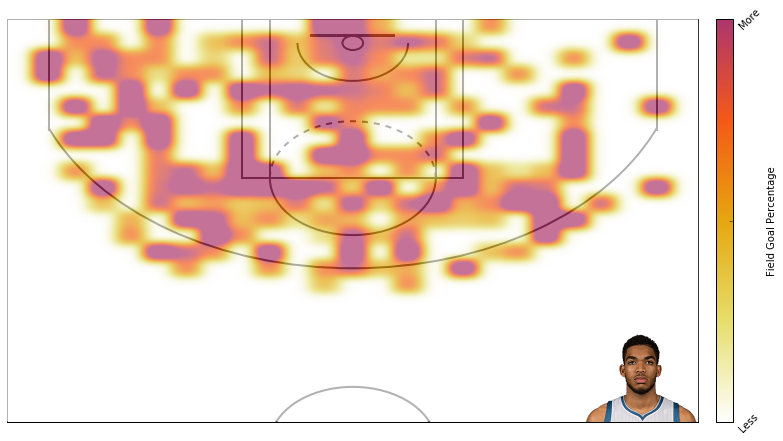
Here’s points across the court.
这是整个球场上的要点。
|
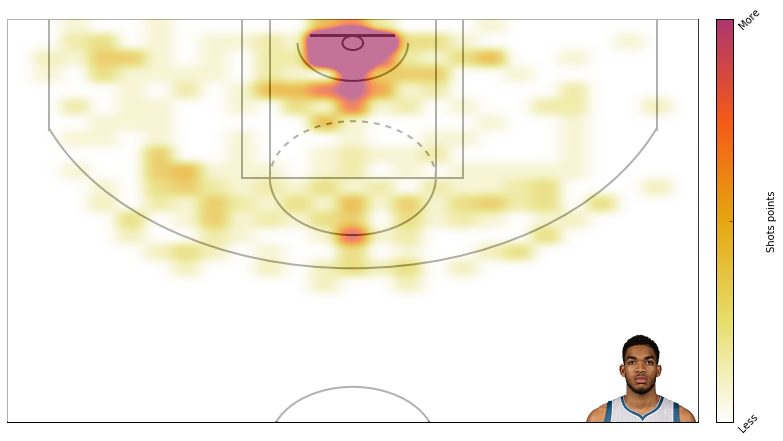
Here’s how KAT’s attempts compare to the league average. You can see the twolve’s midrange heavy offense.
这是KAT的尝试与联盟平均值进行比较的方式。 您可以看到Twolve的中距离进攻。
|
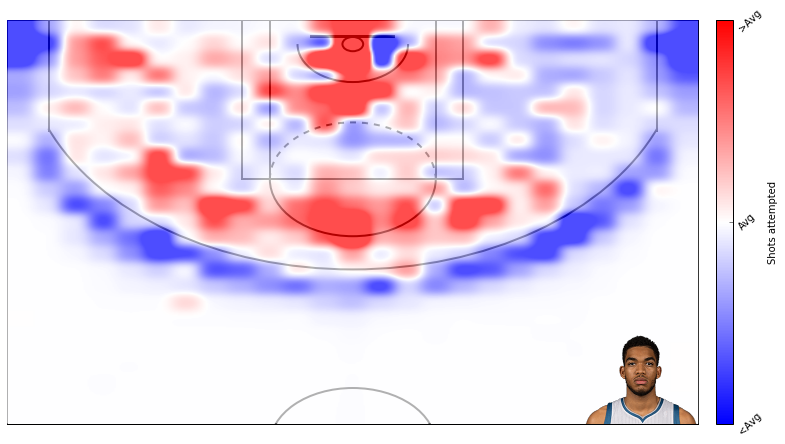
How KAT’s shots made compares to league average.
KAT的投篮方式与联盟平均水平相比。
|
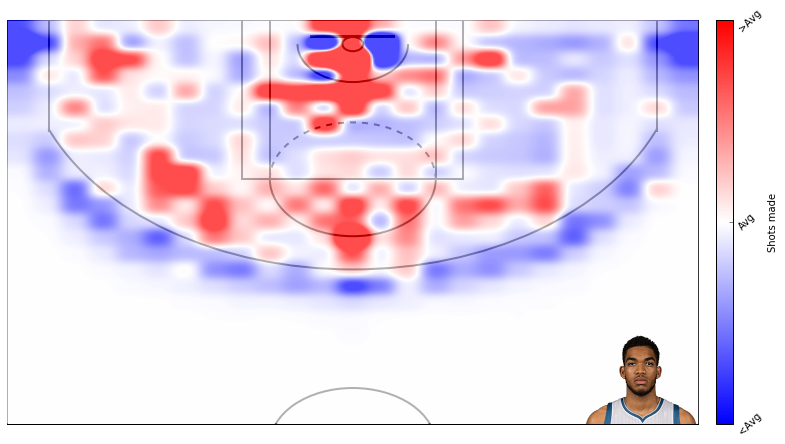
How KAT’s field goal percentage compares to league average. Again, the scale on these is not too good.
KAT的投篮命中率与联盟平均值的比较。 同样,这些方面的规模还不太好。
|
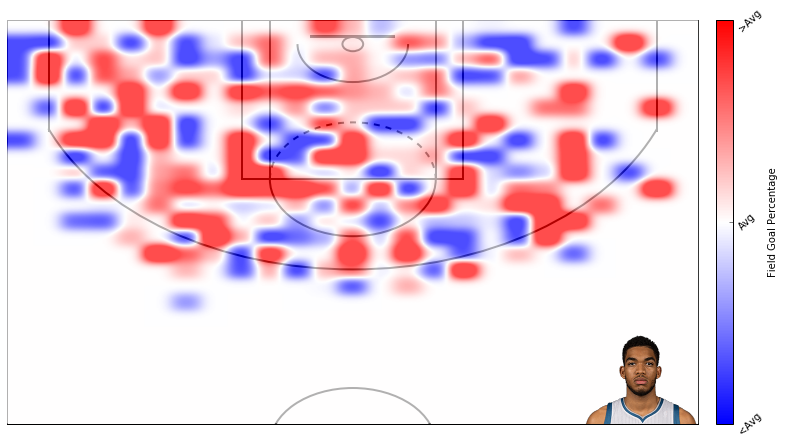
And here is how KAT’s points compare to league average.
这是KAT的积分与联盟平均值的比较。
|
翻译自: https://www.pybloggers.com/2016/05/nba-shot-charts-updated/
百度charts
百度charts_NBA Shot Charts:更新相关推荐
- 64位百度云 catia v6_逃狱兄弟百度云资源已更新,1080P高清资源分享64
进最后阅读原文获取免费资源在线观看 曾经属于香港电影的时代,随着内地电影业迅猛的发展,逐步的落寞了.逃狱兄弟百度云资源已更新,1080P高清资源分享64 但是属于香港电影的情怀依旧难以让人割舍.逃狱兄 ...
- 百度首页快照不更新,内页收录正常是什么原因
百度首页快照不更新,内页收录正常是什么原因 百度首页快照不更新是站长非常头疼的问题,为什么不更新呢? 第一.网站标题.网站关键字.网站描述频繁修改,百度蜘蛛感觉你还没准备好,所以你的网 ...
- 百度网盘偷偷更新,终于实现免费不限速了
给你讲个笑话:百度网盘的下载速度. 作为国内仅剩的几款网盘之一,它的占有率是绝对的No.1.但一直以来,百度网盘限速都是一个老生常谈的问题. 动辄几十KB/s,甚至几KB/s,慢的让人痛不欲生.普通用 ...
- soap 版本可能不匹配: 出现意外的 envelope 命名空间_10M/S!百度网盘偷偷更新,终于实现免费不限速了!...
给你讲个笑话:百度网盘的下载速度. 作为国内仅剩的几款网盘之一,它的占有率是绝对的No.1.但一直以来,百度网盘限速都是一个老生常谈的问题. 动辄几十KB/s,甚至几KB/s,慢的让人痛不欲生.普通用 ...
- 10M/S!百度网盘偷偷更新,终于实现免费不限速了!
来自:扩展迷EXTFANS(ID:infinitydaily).快科技 阅读文本大概需要 5 分钟 给你讲个笑话:百度网盘的下载速度. 作为国内仅剩的几款网盘之一,它的占有率是绝对的No.1.但一直以 ...
- 免费空间python_10M/S!百度网盘偷偷更新,终于实现免费不限速了?!
给你讲个笑话:百度网盘的下载速度. 作为国内仅剩的几款网盘之一,它的占有率是绝对的No.1.但一直以来,百度网盘限速都是一个老生常谈的问题. 动辄几十KB/s,甚至几KB/s,慢的让人痛不欲生.普通用 ...
- 红帽 Red Hat Linux相关产品iso镜像下载【百度云】【更新7.7】
红帽 Red Hat Linux相关产品iso镜像下载[百度云][更新7.7] 正式发布 7.7 : RedHat Enterprise Server 7.7 for x86_64: rhel-ser ...
- ddrelease64 黑苹果_[原]红帽 Red Hat Linux相关产品iso镜像下载【百度云】【更新7.7】...
Jul 23 [原]红帽 Red Hat Linux相关产品iso镜像下载[百度云][更新7.7] 引用功能被关闭了. 不为什么,就为了方便搜索,特把红帽EL 5.EL6.EL7.EL8 的各版本整理 ...
- 公司百度百科信息要更新完善,应该怎么修改百度百科词条呢
公司百度百科信息太简单了,想要更新完善,上传更多关于公司简介的内容,应该怎么修改百度百科词条内容呢.洛希爱做百科网提醒大家,所有百度百科词条修改的内容都必须是有依有据,并不是从主观角度想要增添删减任何 ...
最新文章
- 炫酷大屏demo_可视化大屏动态效果
- AMP328音频放大器
- mysql connection闪退重连_玩家排位巅峰赛开局闪退,重连失败,10分钟后一个提示让他懵了...
- Makefile 自动产生依赖
- 异常处理机制——panic 和 recover
- python htmltestrunner报告_为什么python+htmltestrunner生成的测试报告有问题?
- 腾讯开源最大规模多标签图像数据集,刷新行业数据集基准
- ScrollView 里面捕获OnTouchMove事件
- 四、Mysql安装多实例
- 第 7 章 Neutron - 066 - Neutron 网络基本概念
- 图片居中操作 空行 html,word中进行图片居中对齐批量设置的操作技巧
- 悦box(mt7620a)刷padavan固件
- 查看计算机温度指令,如何查看电脑CPU的温度?查看CPU温度的两种方法
- 编写java判断闰年_Java 判断闰年代码实例
- 什么是超图(Hypergraph)网络?
- git 提交代码防止尾行序列LF转为CRLF
- Browserslist: caniuse-lite is outdated. Please run next command npm update caniuse-lite browserslis
- 『Excel』常用五大类函数汇总
- linux中as命令,Linux as 命令 command not found as 命令详解 as 命令未找到 as 命令安装 - CommandNotFound ⚡️ 坑否...
- 【量化LDPC】基于量化技术的LDPC译码算法的研究与matlab仿真