POJ1392: Ouroboros Snake 题解
Description
Ouroboros is a mythical snake from ancient Egypt. It has its tail in its mouth and continously devours itself.
The Ouroboros numbers are binary numbers of 2^n bits that have the property of “generating” the whole set of numbers from 0 to 2^n - 1. The generation works as follows: given an Ouroboros number, we place its 2^n bits wrapped in a circle. Then, we can take 2^n groups of n bits starting each time with the next bit in the circle. Such circles are called Ouroboros circles for the number n. We will work only with the smallest Ouroboros number for each n.
Example: for n = 2, there are only four Ouroboros numbers. These are 0011;0110;1100; and 1001. In this case, the smallest one is 0011. Here is the Ouroboros circle for 0011:
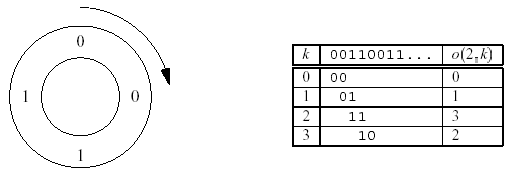
The table describes the function o(n;k) which calculates the k-th number in the Ouroboros circle of the smallest Ouroboros number of size n. This function is what your program should compute.
Input
The input consists of several test cases. For each test case, there will be a line containing two integers n and k (1<=n<=15; 0<=k<2^n). The end of the input file is indicated by a line containing two zeros. Don抰 process that line.
Output
For each test case, output o(n;k) on a line by itself.
Sample Input
2 0
2 1
2 2
2 3
0 0
Sample Output
0
1
3
2
刚开始想如果把每个数看做一个点,把能互相转移的点之间连一条有向边,那么就要求字典序最小的哈密尔顿回路,这是一个NPC,要想办法换成欧拉回路
如果我们把所有的长度为n-1的后缀看做点,能相互转移的后缀之间连边,那么这些边代表的就是所有的数,同时还有新加的数位是0还是1,这样就转化成欧拉回路了
这个图肯定是有解的,为了使字典序最小,肯定从0出发,用Fluery算法求欧拉回路的时候,每次挑字典序小的先走,这样最后的路径reverse一下就是字典序最小的,这个感觉挺对的,大概就是那些0肯定会被放在尽可能后面,reverse完了就到前面了
#include <cstdio>
#include <iostream>
#include <cstring>
#include <string>
#include <cstdlib>
#include <utility>
#include <cctype>
#include <bitset>
#include <sstream>
#include <cmath>
#include <iomanip>
#include <functional>
#include <algorithm>
#include <vector>
#include <set>
#include <map>
#include <stack>
#include <queue>
#include <deque>
#include <list>
using namespace std;#define LL long long
#define LB long double
#define ull unsigned long long
#define x first
#define y second
#define pb push_back
#define pf push_front
#define mp make_pair
#define Pair pair<int,int>
#define pLL pair<LL,LL>
#define pii pair<double,double>const int INF=2e9;
const LL LINF=2e16;
const int magic=348;
const int MOD=1e9+7;
const double eps=1e-10;
const double pi=acos(-1);inline int getint()
{bool f;char ch;int res;while (!isdigit(ch=getchar()) && ch!='-') {}if (ch=='-') f=false,res=0; else f=true,res=ch-'0';while (isdigit(ch=getchar())) res=res*10+ch-'0';return f?res:-res;
}const int MAXN=1e5;int n,k;
int to[2][MAXN+48];bool used[2][MAXN+48];
int path[MAXN*2+48],tot=0;inline void dfs(int cur)
{if (!used[0][cur]){used[0][cur]=true;dfs(to[0][cur]);path[++tot]=0;}if (!used[1][cur]){used[1][cur]=true;dfs(to[1][cur]);path[++tot]=1;}
}int main ()
{int i,Mask,toMask;while (scanf("%d%d",&n,&k) && !(!n && !k)){for (Mask=0;Mask<=(1<<(n-1))-1;Mask++)for (i=0;i<=1;i++){toMask=(Mask<<1);toMask|=i;toMask&=((1<<(n-1))-1);to[i][Mask]=toMask;used[i][Mask]=false;}for (i=0;i<=n-2;i++) path[i]=0;tot=n-2;dfs(0);reverse(path+n-1,path+tot+1);memcpy(path+tot+1,path,(tot+1)*sizeof(int));int ans=0;for (i=k;i<=k+n-1;i++) ans<<=1,ans|=path[i];printf("%d\n",ans);}return 0;
}
POJ1392: Ouroboros Snake 题解相关推荐
- 图论--欧拉路,欧拉回路(小结)
在题目中在慢慢细说概念 1.HDU - 3018 Ant Trip 题目大意:又N个村庄,M条道路.问须要走几次才干将全部的路遍历 解题思路:这题问的是有关欧拉路的判定 欧拉路就是每条边仅仅能走一次, ...
- 【HDOJ图论题集】【转】
1 =============================以下是最小生成树+并查集====================================== 2 [HDU] 3 1213 How ...
- 一系列图论问题[转]
=============================以下是最小生成树+并查集====================================== [HDU] 1213 How Many ...
- 【转载】图论 500题——主要为hdu/poj/zoj
转自--http://blog.csdn.net/qwe20060514/article/details/8112550 =============================以下是最小生成树+并 ...
- kk_想要学习的知识
2018/4/27 计算几何 一.简介 计算几何属于ACM算法中比较冷门的分类,在省赛中只在前几年考察过,这两年还没有考过,而且和高精度计算一样,遇到题目主要靠套模板,因此对题意的理解至关重要,而且往 ...
- POJ前面的题目算法思路【转】
1000 A+B Problem 送分题 49% 2005-5-7 1001 Exponentiation 高精度 85% 2005-5-7 1002 487-3279 n/a 90% 2005-5- ...
- poj题目详细分类及算法推荐题目
DP: 1011 NTA 简单题 1013 Great Equipment 简单题 1024 Calendar Game 简单题 ...
- ACM POJ 题目分类(完整整理版本)
DP: 1011 NTA 简单题 1013 Great Equipment 简单题 1024 Calendar Game 简单题 ...
- POJ ZOJ题目分类
POJ,ZOJ题目分类(多篇整合版,分类很细致,全面) 标签: 题目分类POJ整理 2015-04-18 14:44 1672人阅读 评论(0) 收藏 举报 本文章已收录于: 分类: ACM资料(5) ...
- POJ,ZOJ题目分类(多篇整合版,分类很细致,全面)
水题: 3299,2159,2739,1083,2262,1503,3006,2255,3094 初级: 一.基本算法: (1)枚举 (1753,2965) (2)贪心(13 ...
最新文章
- 高斯拟合原理_看得见的高斯过程:这是一份直观的入门解读
- 一行代码都不用写,教你如何快速搭建Github博客!!!
- xdm,把我大学四年能用到的软件都分享给你。
- dede织梦5.7,后台采集数据导入,空文章过滤.
- flex的enter_frame事件详解
- php图像无法显示,php – 无法显示图像,因为它包含错误[图像生成器]
- 三星宣布华大九天成为其晶圆代工生态系统SAFE EDA合作伙伴
- mat分析dump分析_使用Eclipse Memory Analyzer Tool(MAT)分析线上故障(一)
- Atitit 提升稳定性 错误处理 全局错误捕获 1.2. 可以uncaughtException来全局捕获未捕获的Error, 使用uncaughtException	2 1.2.1. 使用 t
- jquery weui 显示loading
- docker视频教程 百度云网盘
- Ubuntu上安装Chrome浏览器
- 使用ntsd命令强制性杀掉进程[微软未开公的密秘]
- mysql时区重启后失效_mysql时区问题
- 在office2010的ppt中加入音乐
- LaTex练习日记02 —— 字体设置
- API-fox 接口神器
- 西安交通大学第14周大计基
- 人工智能安防初创公司澎思科技宣布完成千万级天使轮融资 洪泰基金领投
- c语言解析cron文件,Cron(表达式)详解
热门文章
- linux mv中途进程断掉,shell入门
- c语言判断奇偶数的函数,c语言高手进,尽量多做点13. 定义一个函数even(),判断一个整数是否是偶数。如果是偶数返回1,否则返回0。(要求包...
- 巨坑:transport.TransportException:Cannot execute request on any known server
- pycharm虚拟环境 更换interpreter
- 学习利用ce修改游戏生命参数
- 服务器做虚拟网吧,一种基于游戏的虚拟网吧实现方法
- brew upgrade出现It seems there is already an App at的解决方法
- docker安装oracle
- 和生活一起理解51单片机① 如何入门学习单片机
- HTML与Java组合使用_【自学java笔记#第五十四天#】javaweb day02 html和css的组合使用...