wordpress 数据库_在WordPress中使用数据库
wordpress 数据库
Out of the box, WordPress provides tons of functions that can be used to interact with the database. In most cases, the WP_Query
class and related functions such as wp_insert_post
, update_post_meta
, get_posts
will be sufficient to get the job done. However, there will be times that we’re required to do something that is not provided by WordPress natively, especially when we need to deal with custom tables.
WordPress开箱即用,提供了大量可用于与数据库进行交互的功能。 在大多数情况下, WP_Query
类和相关功能(例如wp_insert_post
, update_post_meta
, get_posts
足以完成工作。 但是,有时候我们需要做一些WordPress本身不提供的事情,尤其是当我们需要处理自定义表时。
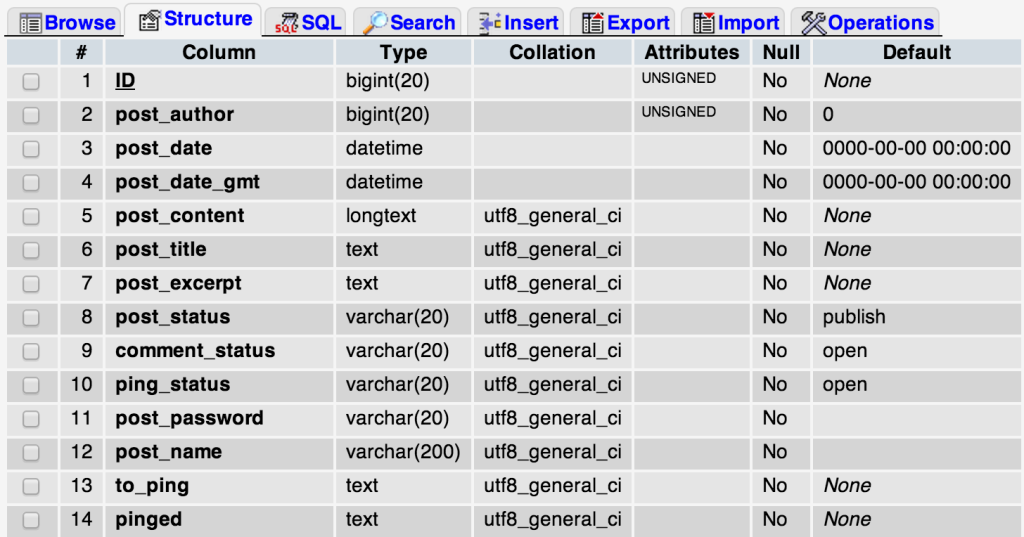
In this tutorial, we’ll walk through the most important class to deal with databases in WordPress – wpdb
, including a few tips and tricks that can be implemented in our development workflow later on. We’ll also touch on dbDelta
that can be used to create custom tables in our plugin. We won’t cover the basics of creating your original WordPress database in this tutorial, but feel free to check out this tutorial on creating databases from cPanel
在本教程中,我们将遍历最重要的类以处理WordPress中的数据库– wpdb
,其中包括一些可以在稍后的开发工作流程中实现的技巧。 我们还将触摸dbDelta
,它可用于在插件中创建自定义表。 在本教程中,我们将不介绍创建原始WordPress数据库的基础知识,但是请随时查看有关从cPanel创建数据库的本教程。
使用wpdb
类 (Working with the wpdb
Class)
wpdb
is perhaps the single most important class that we use when we need to deal with database directly. It is based on the ezSQL
class written by Justin Vincent, adapted to work with WordPress.
wpdb
可能是我们需要直接处理数据库时使用的最重要的单个类。 它基于Justin Vincent编写的ezSQL
类,适用于WordPress。
The basic methods and properties of the wpdb
class is already well explained on the WordPress Codex page so there’s no point to reiterate them here. Instead, we’re going to go through a few common mistakes that WordPress developers can make, how to rectify them, as well as best practices that can be applied when using the wpdb
class.
wpdb
类的基本方法和属性已经在WordPress Codex页面上得到了很好的解释,因此这里没有必要重申它们。 相反,我们将遇到WordPress开发人员可能犯的一些常见错误,如何纠正这些错误以及使用wpdb
类时可以应用的最佳实践。
不要将表名称硬编码到SQL查询中 (Do Not Hardcode Table Names into the SQL Query)
Some developers make the general assumption that the table prefix will be unchanged and use default value of wp_
. A basic example of the wrong way to do this is illustrated in the snippet below:
一些开发人员通常假设表前缀将保持不变,并使用wp_
默认值。 下面的代码段演示了错误方法的一个基本示例:
global $wpdb;
$result = $wpdb->get_results('SELECT * FROM wp_posts LIMIT 10');
This is of course is an over simplification of what a plugin will be actually doing, but this example shows how quickly things can go wrong. What if our users change the table prefix to something else? This can be easily fixed by replacing the wp_
string with actual properties provided by using prefix
.
当然,这是对插件实际操作的过度简化,但是此示例显示了出错的速度有多快。 如果我们的用户将表前缀更改为其他内容怎么办? 通过使用prefix
提供的实际属性替换wp_
字符串,可以轻松解决此问题。
The above code can be made portable by applying the changes as below:
通过应用以下更改,可以使以上代码可移植:
global $wpdb;
$result = $wpdb->get_results('SELECT * FROM ' . $wpdb->prefix . 'posts LIMIT 10');
Even better, if you’re dealing with WordPress’ default tables, you can skip the prefix part and instead, directly address them as properties in wpdb
. Every default WordPress table is represented by a custom property in the wpdb
class with the same name of table without the prefix.
更好的是,如果要处理WordPress的默认表,则可以跳过前缀部分,而直接将其作为wpdb
属性来wpdb
。 每个默认的WordPress表都由wpdb
类中的自定义属性表示,该属性具有与表相同的名称,但没有前缀。
For example, assuming that the table prefix is wp_
:
例如,假设表前缀为wp_
:
$wpdb->posts
will correspond towp_posts
table$wpdb->posts
将对应于wp_posts
表$wpdb->postmeta
will correspond towp_postmeta
table$wpdb->postmeta
将对应于wp_postmeta
表$wpdb->users
will correspond towp_users
table$wpdb->users
将对应于wp_users
表
And so on. The above code can be improved further, since we’re querying the posts table by doing things this way instead:
等等。 上面的代码可以进一步改进,因为我们通过这种方式来查询posts表:
global $wpdb;
$result = $wpdb->get_results('SELECT * FROM ' . $wpdb->posts . ' LIMIT 10');
使用特定的辅助方法进行数据库操作 (Use Specific Helper Methods for Database Operations)
Although the query
method is designed to handle any SQL queries, it’s preferable to use more appropriate helper methods. This is usually provided by methods such as insert
, update
, get_row
and others. Besides that it is more specific to our use cases, it’s also safer as the escaping and other grunt work is taken care of.
尽管query
方法旨在处理任何SQL查询,但最好使用更合适的帮助程序方法。 通常由诸如insert
, update
, get_row
等方法提供。 除了它对于我们的用例更特定之外,它也更安全,因为可以进行转义和其他繁琐的工作。
Let’s take a look at this example:
让我们看一下这个例子:
$global wpdb;
$post_id = $_POST['post_id'];
$meta_key = $_POST['meta_key'];
$meta_value = $_POST['meta_value'];
$wpdb->query("INSERT INTO $wpdb->postmeta
( post_id, meta_key, meta_value )
VALUES ( $post_id, $meta_key, $meta_value )"
);
Apart from the unsafe nature of this snippet, it should run just fine with proper values. However, this snippet can be improved further by using the insert
method instead. The above code can be changed to look like the following:
除了该代码段的不安全特性外,还应使用适当的值来正常运行。 但是,可以改为使用insert
方法来进一步改善此代码段。 上面的代码可以更改为如下所示:
$global wpdb;
$post_id = $_POST['post_id'];
$meta_key = $_POST['meta_key'];
$meta_value = $_POST['meta_value'];
$wpdb->insert(
$wpdb->postmeta,
array(
'post_id' => $_POST['post_id'],
'meta_key' => $_POST['meta_key'],
'meta_value' => $_POST['meta_value']
)
);
If we’re not supplying the format as a third parameter for insert
method, all of the data provided in the second parameter will be escaped as a string. Plus, we can easily know what this code does at a glance, since the method name is clearer.
如果我们没有将格式作为insert
方法的第三个参数提供,则第二个参数中提供的所有数据都将以字符串形式转义。 另外,由于方法名称更清晰,我们一眼就能知道该代码的作用。
正确调试数据库查询 (Properly Debugging Database Queries)
By default, error reporting is turned off. However, wpdb
provides two methods that can be used to toggle the state of error reporting.
默认情况下,错误报告处于关闭状态。 但是, wpdb
提供了两种可用于切换错误报告状态的方法。
To turn on the error reporting feature, simply run this code.
要打开错误报告功能,只需运行以下代码。
$wpdb->show_errors();
And to turn it off:
并关闭它:
$wpdb->hide_errors();
Another thing to note is that if we’re setting both WP_DEBUG
and WP_DEBUG_DISPLAY
to true
, the show_errors
method will be automatically called. There is another useful method that can be used that deals with errors, namely print_error
:
要注意的另一件事是,如果将WP_DEBUG
和WP_DEBUG_DISPLAY
都设置为true
,则会自动调用show_errors
方法。 可以使用另一种有用的方法来处理错误,即print_error
:
$wpdb->print_error();
As the name suggests, it will only show the error for the most recent query, regardless of the state of error reporting.
顾名思义,它将仅显示最近查询的错误,而不管错误报告的状态如何。
Another neat trick is to enable SAVEQUERIES
in wp-config.php
. This will store all of the database queries that run, times taken, and where it’s originally called from into a property called queries
in the wpdb
class.
另一个巧妙的技巧是在wp-config.php
启用SAVEQUERIES
。 这将存储所有的数据库查询该运行,采取倍,并在它最初是从一个叫做属性调用queries
在wpdb
类。
To retrieve this data, we can do the following:
要检索此数据,我们可以执行以下操作:
print_r( $wpdb->queries );
Note that this will have a performance impact on our site, so use it only when necessary.
请注意,这将对我们的网站产生性能影响,因此仅在必要时使用它。
Most of the time, these functions will be enough to debug what is going wrong with our code. However, for more extensive debugging and reporting, there’s always the Query Monitor plugin that can help debugging more than database queries.
大多数时候,这些功能足以调试我们的代码出了什么问题。 但是,对于更广泛的调试和报告,总有Query Monitor插件可以比数据库查询更多地帮助调试。
确保查询免受潜在攻击 (Securing Queries Against Potential Attacks)
To completely secure our code from SQL injection, wpdb
also provides another helpful method called prepare
that will take a string of an SQL statement and data that needs to be escaped. This is relevant whenever we’re dealing with methods like query
or get_results
.
为了完全保护我们的代码免于SQL注入, wpdb
还提供了另一个有用的方法,称为prepare
,它将采用SQL语句的字符串和需要转义的数据。 每当我们处理诸如query
或get_results
类的方法时,这都是相关的。
$wpdb->prepare( $sql, $format... );
The prepare
method supports both syntax of sprintf
and vsprintf
. The first parameter, $sql
is an SQL statement that’s filled with placeholders. These placeholders can exist in three different formats:
prepare
方法同时支持sprintf
和vsprintf
语法。 第一个参数$sql
是一个占位符填充SQL语句。 这些占位符可以以三种不同的格式存在:
%s
for string字符串的
%s
%d
for integer%d
代表整数%f
for float%f
表示浮动
$format
can be a series of parameters for sprintf
like syntax, or an array of parameters that will be used to replace the placeholder in $sql
. The method will return the SQL with properly escaped data.
$format
可以是sprintf
的一系列参数,例如语法,或者是将用于替换$sql
的占位符的参数数组。 该方法将返回带有正确转义数据SQL。
Let’s take a look at how we can achieve the process of deleting meta_key
in wp_postmeta
for a specific post ID:
让我们来看看如何实现删除的过程meta_key
在wp_postmeta
特定文章ID:
$global wpdb;
$post_id = $_POST['post_id'];
$key = $_POST['meta_key'];
$wpdb->query(
"DELETE FROM $wpdb->postmeta
WHERE post_id = $post_id
AND meta_key = $key"
);
Note that this is not the recommended way to delete a record in the database using wpdb
. It’s because we’re leaving the code open to SQL injection, since the user input is not properly escaped and used directly in the DELETE
statement.
请注意,这不是使用wpdb
删除数据库中记录的推荐方法。 这是因为我们没有对SQL注入开放代码,因为用户输入没有正确转义并直接在DELETE
语句中使用。
However, this can be easily fixed! We simply introduce the prepare
method before doing the actual query, so that the generated SQL is safe to use. This can be illustrated in the snippet below:
但是,这很容易解决! 我们仅在进行实际查询之前简单介绍一下prepare
方法,以便可以安全地使用生成SQL。 可以在下面的代码段中进行说明:
$global wpdb;
$post_id = $_POST['post_id'];
$key = $_POST['meta_key'];
$wpdb->query(
$wpdb->prepare(
"DELETE FROM $wpdb->postmeta
WHERE post_id = %d
AND meta_key = %s",
$post_id,
$key
)
);
连接到单独的数据库 (Connecting to Separate Databases)
By default, the $wpdb
variable is an instance of the wpdb
class that connects to the WordPress database defined in wp-config.php
. If we want to interact with other databases, we can instantiate another instance of wpdb
class. This benefits us greatly, because methods like insert
, update
and get_results
are available.
默认情况下, $wpdb
变量是wpdb
类的实例,该类连接到wp-config.php
定义的WordPress数据库。 如果要与其他数据库进行交互,可以实例化wpdb
类的另一个实例。 这使我们受益匪浅,因为可以使用诸如insert
, update
和get_results
类的方法。
The wpdb
class accepts four parameters in construct, which are username, password, database name and database host, in that order. Here’s an example:
wpdb
类在构造中接受四个参数,分别是用户名,密码,数据库名称和数据库主机。 这是一个例子:
$mydb = new wpdb( 'username', 'password', 'my_database', 'localhost' );
// At this point, $mydb has access to the database and all methods
// can be used as usual
// Example query
$mydb->query('DELETE FROM external_table WHERE id = 1');
If we’re using the same username, password and database host, but only need to change the selected database, there’s a handy method called select
on the global $wpdb
variable. This is achieved internally by using the mysql_select_db
/mysqli_select_db
function.
如果我们使用相同的用户名,密码和数据库主机,但只需要更改选定的数据库,则在全局$wpdb
变量上有一个方便的方法叫做select
。 这是通过使用mysql_select_db
/ mysqli_select_db
函数在内部实现的。
$wpdb->select('my_database');
This is also particularly useful when we want to switch to another WordPress database, but still want to retain the functionality of functions like get_post_custom
and others.
当我们想切换到另一个WordPress数据库,但仍想保留诸如get_post_custom
等功能的功能时,这也特别有用。
使用自定义数据库表 (Using Custom Database Tables)
The WordPress default tables usually suffice to handle most complex operations. Utilizing custom post types with post metadata, and custom taxonomies and term metadata, we can do almost anything without the need of using custom tables.
WordPress默认表通常足以处理大多数复杂的操作。 通过将自定义帖子类型与帖子元数据,自定义分类法和术语元数据结合使用,我们几乎可以执行任何操作,而无需使用自定义表格。
However, custom tables might be useful whenever we want to have finer control over the data our plugin can handle. Benefits of using custom tables include:
但是,每当我们想要对插件可以处理的数据进行更好的控制时,自定义表可能会很有用。 使用自定义表的好处包括:
Total control of the data structure – Not all types of data fits into the structure of a
post
, so when we want to store data that does not make any sense as custom post type, a custom table may be a better option.完全控制数据结构 –并非所有类型的数据都适合
post
的结构,因此,当我们要存储对自定义帖子类型没有意义的数据时,自定义表可能是一个更好的选择。Separation of concerns – Since our data is stored in a custom table, it won’t interfere with the
wp_posts
orwp_postmeta
tables as opposed to if we were using custom post types. Migration of our data to another platform is easier since it is not restricted to how WordPress structures its data.关注点分离 –由于我们的数据存储在自定义表中,因此与我们使用自定义帖子类型相比,它不会干扰
wp_posts
或wp_postmeta
表。 将我们的数据迁移到另一个平台更容易,因为它不仅限于WordPress构造数据的方式。Efficiency – Querying data from our specific table will be definitely much faster than scouring over the
wp_posts
table that also contains data unrelated to our plugin. This is an apparent problem when using custom post types to store lots of metadata that can bloat thewp_postmeta
table.效率 –从我们的特定表中查询数据绝对比在
wp_posts
表中进行查询要快得多,该表还包含与我们的插件无关的数据。 使用自定义帖子类型存储大量可能会使wp_postmeta
表膨胀的元数据时,这是一个明显的问题。
dbDelta进行救援 (dbDelta to the Rescue)
Instead of using wpdb
to create custom database table, it’s recommended using dbDelta to handle all of your initial table creation, as well as table schema updates. It’s reliable, since WordPress core uses this function as well to handle any database schema updates from version to version, if any.
建议不要使用wpdb
创建自定义数据库表,而是建议使用dbDelta来处理所有初始表创建以及表模式更新。 这是可靠的,因为WordPress核心也使用此功能来处理任何版本之间的数据库架构更新(如果有)。
To create a custom table initially on plugin install, we need to hook our function to the register_activation_hook
function. Assuming that our main plugin file is plugin-name.php
inside a plugin-name
directory, we can put this line directly into it:
为了在插件安装时最初创建一个自定义表,我们需要将函数挂钩到register_activation_hook
函数。 假设我们的主插件文件是plugin-name.php
plugin-name
目录中的plugin-name.php
,我们可以直接plugin-name.php
下行放入其中:
register_activation_hook( __FILE__, 'prefix_create_table' );
Next, we need to create the function prefix_create_table
that does the actual table creation on plugin activation. For example, we can create a custom table called my_custom_table
that will be used to store simple customer data such as first name, last name and their email address.
接下来,我们需要创建函数prefix_create_table
,该函数在插件激活时进行实际的表创建。 例如,我们可以创建一个名为my_custom_table
的自定义表,该表将用于存储简单的客户数据,例如名字,姓氏及其电子邮件地址。
function prefix_create_table() {
global $wpdb;
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE my_custom_table (
id mediumint(9) NOT NULL AUTO_INCREMENT,
first_name varchar(55) NOT NULL,
last_name varchar(55) NOT NULL,
email varchar(55) NOT NULL,
UNIQUE KEY id (id)
) $charset_collate;";
if ( ! function_exists('dbDelta') ) {
require_once( ABSPATH . 'wp-admin/includes/upgrade.php' );
}
dbDelta( $sql );
}
To maximize compatibility, we retrieve the database charset collate from wpdb
. Plus, the SQL statement needs to abide by some rules to make sure it works as intended. This is taken directly from the Codex page on Creating tables with plugin:
为了最大化兼容性,我们从wpdb
检索数据库字符集wpdb
。 另外,SQL语句需要遵守一些规则以确保其按预期工作。 直接从使用插件创建表上的Codex页面获取:
- You must put each field on its own line in your SQL statement.您必须在SQL语句中将每个字段放在自己的行上。
- You must have two spaces between the words PRIMARY KEY and the definition of your primary key.在单词PRIMARY KEY和主键定义之间必须有两个空格。
- You must use the key word KEY rather than its synonym INDEX and you must include at least one KEY.您必须使用关键字KEY而不是其同义词INDEX,并且必须至少包含一个KEY。
- You must not use any apostrophes or backticks around field names.您不得在字段名称周围使用任何撇号或反引号。
- Field types must be all lowercase.字段类型必须全部为小写。
- SQL keywords, like CREATE TABLE and UPDATE, must be uppercase.SQL关键字(例如CREATE TABLE和UPDATE)必须为大写。
- You must specify the length of all fields that accept a length parameter. int(11), for example.您必须指定所有接受长度参数的字段的长度。 例如int(11)。
It’s also generally a good idea to store the database version into the options table, so that we can compare them during a plugin update in case our custom table needs updating. In order to do so, we simply add this line right after we create our table using the dbDelta
function:
通常,将数据库版本存储到options表中也是一个好主意,以便我们可以在插件更新期间比较它们,以防自定义表需要更新。 为此,我们只需在使用dbDelta
函数创建表之后立即添加以下行:
add_option( 'prefix_my_plugin_db_version', '1.0' );
更新表架构 (Updating the Table Schema)
Using the same example as above, let’s say during development, we change our mind and we also want to store our customer phone number inside our table. What we can do is trigger a table schema update during our plugin update. Since register_activation_hook
will not be fired during a plugin update, we can hook into the plugin_loaded
action instead, to do our database version checking, and update the table schema if necessary.
使用与上面相同的示例,假设在开发过程中,我们改变了主意,并且还希望将客户电话号码存储在表中。 我们可以做的是在插件更新期间触发表架构更新。 由于在插件更新期间不会触发register_activation_hook
,因此我们可以plugin_loaded
而挂接到plugin_loaded
操作中,以进行数据库版本检查,并在必要时更新表模式。
First, we add our custom upgrade function to the plugin_loaded
hook:
首先,我们将自定义升级功能添加到plugin_loaded
挂钩:
add_action( 'plugin_loaded', 'prefix_update_table' );
The actual functions needs to do a few things:
实际功能需要做一些事情:
- We need to get the stored database version.我们需要获取存储的数据库版本。
- Compare them with our current database version.将它们与我们当前的数据库版本进行比较。
If it’s newer, we run the
dbDelta
function again.如果是较新的版本,我们将再次运行
dbDelta
函数。- Finally, we store the updated database version to the option table.最后,我们将更新的数据库版本存储到选项表中。
For the most part, we can actually reuse the prefix_create_table
function like we did above, with some minor modifications:
在大多数情况下,我们实际上可以像上面一样重用prefix_create_table
函数,但需要进行一些小的修改:
function prefix_update_table() {
// Assuming we have our current database version in a global variable
global $prefix_my_db_version;
// If database version is not the same
if ( $prefix_my_db_version != get_option('prefix_my_plugin_db_version' ) {
global $wpdb;
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE my_custom_table (
id mediumint(9) NOT NULL AUTO_INCREMENT,
first_name varchar(55) NOT NULL,
last_name varchar(55) NOT NULL,
phone varchar(32) DEFAULT '' NOT NULL, //new column
email varchar(55) NOT NULL,
UNIQUE KEY id (id)
) $charset_collate;";
if ( ! function_exists('dbDelta') ) {
require_once( ABSPATH . 'wp-admin/includes/upgrade.php' );
}
dbDelta( $sql );
update_option( 'prefix_my_plugin_db_version', $prefix_my_db_version );
}
}
Notice that we do not need to use the ALTER
statement, since dbDelta
will take our SQL statement, compare it against existing tables and make the modification accordingly. Pretty handy!
注意,我们不需要使用ALTER
语句,因为dbDelta
将采用我们SQL语句,将其与现有表进行比较并进行相应的修改。 很方便!
结论 (Conclusion)
WordPress is not limited to creating simple websites, as it’s rapidly moving to a full-fledged application framework. Extending WordPress via custom post types and custom taxonomies should be our main priority. However, when we need finer control of our data, it’s reassuring to know that WordPress itself provides various functions and classes like wpdb
for developers to utilize. This is what makes WordPress a mature solution.
WordPress不仅限于创建简单的网站,还因为它正Swift迁移到成熟的应用程序框架。 通过自定义帖子类型和自定义分类法扩展WordPress应该是我们的首要任务。 但是,当我们需要更好地控制数据时,令人欣慰的是,WordPress本身提供了wpdb
等各种函数和类供开发人员使用。 这就是使WordPress成为成熟解决方案的原因。
翻译自: https://www.sitepoint.com/working-with-databases-in-wordpress/
wordpress 数据库
wordpress 数据库_在WordPress中使用数据库相关推荐
- wamp mysql创建数据库_在wamp中创建数据库
有2种创建方式 1.用phpmyadmin可视化创建 1-1:注意数据库的编码选择"utf8_general_ci"这一栏 1-2:接着创建数据表及其相应字段就很简单了 2.启动m ...
- Mysql生产环境表更新方案_生产环境中,数据库升级维护的最佳解决方案flyway
官网:https://flywaydb.org/ 转载:http://casheen.iteye.com/blog/1749916 1. 引言 想到要管理数据库的版本,是在实际产品中遇到问题后想到的 ...
- Java中怎样创建数据库_在java中怎样创建MySQL数据库列表给个例子 爱问知识人
java中怎样创建MySQL数据库列表 需要使用jdbc访问数据库. 具体步骤如下: 1:加载驱动 ,返回连接 private static final String DRIVER_CLASS = & ...
- pg数据库执行sql文件_在linux中Postgresql数据库如何执行脚本文件
在linux中Postgresql数据库如何恢复数据库?执行sql脚本文件呢?具体步骤如下: 一.恢复数据库备份文件 1.将数据库备份文件xxx.backup用连接工具拷贝到/opt下,这个不固定,位 ...
- mysql 数据拦截器_拦截器中操作数据库
做了个小项目,当初设计的是只有一个模块的用户行为被记录,其他不用记录,昨天突然说,要用户在整个系统的行为都要被记录. 很懵逼,如果把用户行为的记录放在各个模块,可以很精确的记录,但是各个模块都要有更改 ...
- php access数据库_在PHP中使用Access数据库
php access数据库 A previous client of mine had an Access database of products they sell, which they had ...
- linux 备份mysql并上传_实现Linux中Mysql数据库自动备份并上传到远程FTP服务器
这篇文章很有分享价值,因为我们在实际的生产环境中需要将数据库进行自动备份,然后上传到指定的位置,当然也可以像以下文章中所讲的一样,上传到你指定的FTP服务器中,从而实现Mysql数据库自动备份并上传到 ...
- java对数据库的增删改查_在java中对数据库进行增删改查
代码区域: package com.oracle.jdbc.demo1; import java.sql.Connection; import java.sql.DriverManager; impo ...
- mysql 新增从数据库_从零开始学 MySQL - 创建数据库并插入数据
目录 1.实验内容 2.实验知识点 3.开发准备 4.实验总结 1.实验内容 本次课程将介绍 MySQL 新建数据库,新建表,插入数据以及基本数据类型的相关知识.本节实验将创建一个名为 mysql_s ...
最新文章
- Genymotion配置及使用教程(最新最完整版附各部分下载地址)最快模拟器
- 视觉检索:视频多帧排序
- 求正多边形的面积JAVA_第六章第三十六题(几何:正多边形的面积)(Geometry: area of a regular polygon)...
- scrum与第一次teamwork
- Python 之 使用 PIL 库做图像处理
- sts 创建webservice项目_常用的RPC架构---WebService
- HTML5 基础知识
- 地铁系统_北斗授时助力北京地铁地下定位系统
- mysql jdbc 驱动 下载地址官网
- 基于FPGA的1080P 60Hz BT1120接口调试过程记录
- confluent(Confluent Cloud)
- hd4600黑苹果html5死机,HD4600终于可以不花屏了,写下心得。
- 背课文记单词,读课文记单词,读文章记单词;40篇文章搞定3500词;71篇文章突破中考单词;15篇文章贯通四级词汇;15篇文章贯通六级词汇
- 实现表格中的el-switch 开关切换
- 使用Teleport Ultra批量克隆网站,使用Easy CHM合并生成chm文件
- oracle锁资源不够,Oracle解锁,解决“ora00054:资源正忙”错误
- 【STM32F429的DSP教程】第25章 DSP变换运算-快速傅里叶变换原理(FFT)
- 让C语言写图形库时不关闭控制台,GCC编译Win图形程序不显示控制台方法
- 【目标检测】目标检测中常见的评价指标
- Robinson Crusoe chapter 8 A ship arrives at the island
热门文章
- ModelSim-Altera路径找不到或者不正确的解决办法
- 【双拼打字】双拼对照表
- Apache Echarts常用图表之柱状图
- 大学应用计算机应用基础课程介绍,《大学计算机应用基础》课程教学大纲
- Git操作与仓库创建
- Office2007中简繁体转换功能按钮消失解决
- 尊敬的用户您好: 您访问的网站被机房安全管理系统拦截,可能是以下原因造成: 1.您
- STM32踩坑1-SWD下载失败
- NOI模拟(5.11) BJOID2T3 治疗之雨 (bzoj5292)
- PyTorch笔记【1】---A卡安装(RX580)无果后,改为N卡(2070)安装。这年头就不要用CPU了来搞AI了