open cv roi提取_使用pytesseract open cv从扫描的pdf中提取文本
open cv roi提取
The process of extracting information from a digital copy of invoice can be a tricky task. There are various tools that are available in the market that can be used to perform this task. However there are many factors due to which most of the people want to solve this problem using Open Source Libraries.
从发票的数字副本中提取信息的过程可能是一项棘手的任务。 市场上有可以用来执行此任务的各种工具。 但是,由于许多因素,大多数人都希望使用开放源代码库解决此问题。
I came across a similar set of problem a few days back and wanted to share with you all the approach through which I solved this problem. The libraries that I used for developing this solution were pdf2image (for converting PDF to images), OpenCV (for Image pre-processing) and finally PyTesseract for OCR along with Python.
几天前,我遇到了一系列类似的问题,并想与大家分享解决该问题的所有方法。 我用于开发此解决方案的库是pdf2image (用于将PDF转换为图像), OpenCV (用于图像预处理),最后是用于OCR的PyTesseract和Python 。
将PDF转换为图像 (Converting PDF to Image)
pdf2image is a python library which converts PDF to a sequence of PIL Image objects using pdftoppm library. The following command can be used for installing the pdf2image library using pip installation method.
pdf2image是一个python库,可使用pdftoppm库将PDF转换为PIL Image对象序列。 以下命令可用于通过pip安装方法安装pdf2image库。
pip install pdf2image
点安装pdf2image
Note: pdf2image uses Poppler which is a PDF rendering library based on the xpdf-3.0 code base and will not work without it. Please refer to the below resources for downloading and installation instructions for Poppler.
注意:pdf2image使用Poppler ,它是基于xpdf-3.0代码库的PDF渲染库,没有它就无法使用。 请参考以下资源以获取Poppler的下载和安装说明。
https://anaconda.org/conda-forge/poppler
https://anaconda.org/conda-forge/popple r
https://stackoverflow.com/questions/18381713/how-to-install-poppler-on-windows
https://stackoverflow.com/questions/18381713/how-to-install-poppler-on-windows
After installation, any pdf can be converted to images using the below code.
安装后,可以使用以下代码将任何pdf转换为图像。
After converting the PDF to images, the next step is to highlight the regions of the images from which we have to extract the information.
将PDF转换为图像后,下一步是突出显示我们必须从中提取信息的图像区域。
Note: Before marking regions make sure that you have preprocessed the image for improving its quality (DPI ≥ 300, Skewness, Sharpness and Brightness should be adjusted, Thresholding etc.)
注意:在标记区域之前,请确保已对图像进行了预处理以改善其质量(DPI≥300,应调整偏斜度,清晰度和亮度,阈值设置等)。
标记图像区域以进行信息提取 (Marking Regions of Image for Information Extraction)
Here in this step we will mark the regions of the image from where we have to extract the data. After marking those regions with the rectangle, we will crop those regions one by one from the original image before feeding it to the OCR engine.
在此步骤中,我们将标记必须从中提取数据的图像区域。 在用矩形标记了这些区域之后,我们将原始图像一一裁剪掉这些区域,然后再将其提供给OCR引擎。
Most of us would think to this point — why should we mark the regions in an image before doing OCR and not doing it directly?
我们大多数人都会想到这一点–为什么在进行OCR而不直接进行图像处理之前,我们应该在图像中标记区域?
The simple answer to this question is that YOU CAN
这个问题的简单答案是您可以
The only catch to this question is sometimes there are hidden line breaks/ page breaks that are embedded in the document and if this document is passed directly into the OCR engine, the continuity of data breaks automatically (as line breaks are recognized by OCR).
解决此问题的唯一方法是有时在文档中嵌入隐藏的换行符/分页符,并且如果将此文档直接传递到OCR引擎中,则数据的连续性会自动(由于换行符被OCR识别)。
Through this approach, we can get maximum correct results for any given document. In our case we will be trying to extract information from an invoice using the exact same approach.
通过这种方法,我们可以获得任何给定文档的最大正确结果。 在我们的案例中,我们将尝试使用完全相同的方法从发票中提取信息。
The below code can be used for marking the regions of interest in the image and getting their respective co-ordinates.
以下代码可用于标记图像中的感兴趣区域并获取它们各自的坐标。
# use this command to install open cv2
# pip install opencv-python# use this command to install PIL
# pip install Pillowimport cv2
from PIL import Imagedef mark_region(imagE_path):im = cv2.imread(image_path)gray = cv2.cvtColor(im, cv2.COLOR_BGR2GRAY)blur = cv2.GaussianBlur(gray, (9,9), 0)thresh = cv2.adaptiveThreshold(blur,255,cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY_INV,11,30)# Dilate to combine adjacent text contourskernel = cv2.getStructuringElement(cv2.MORPH_RECT, (9,9))dilate = cv2.dilate(thresh, kernel, iterations=4)# Find contours, highlight text areas, and extract ROIscnts = cv2.findContours(dilate, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)cnts = cnts[0] if len(cnts) == 2 else cnts[1]line_items_coordinates = []for c in cnts:area = cv2.contourArea(c)x,y,w,h = cv2.boundingRect(c)if y >= 600 and x <= 1000:if area > 10000:image = cv2.rectangle(im, (x,y), (2200, y+h), color=(255,0,255), thickness=3)line_items_coordinates.append([(x,y), (2200, y+h)])if y >= 2400 and x<= 2000:image = cv2.rectangle(im, (x,y), (2200, y+h), color=(255,0,255), thickness=3)line_items_coordinates.append([(x,y), (2200, y+h)])return image, line_items_coordinates
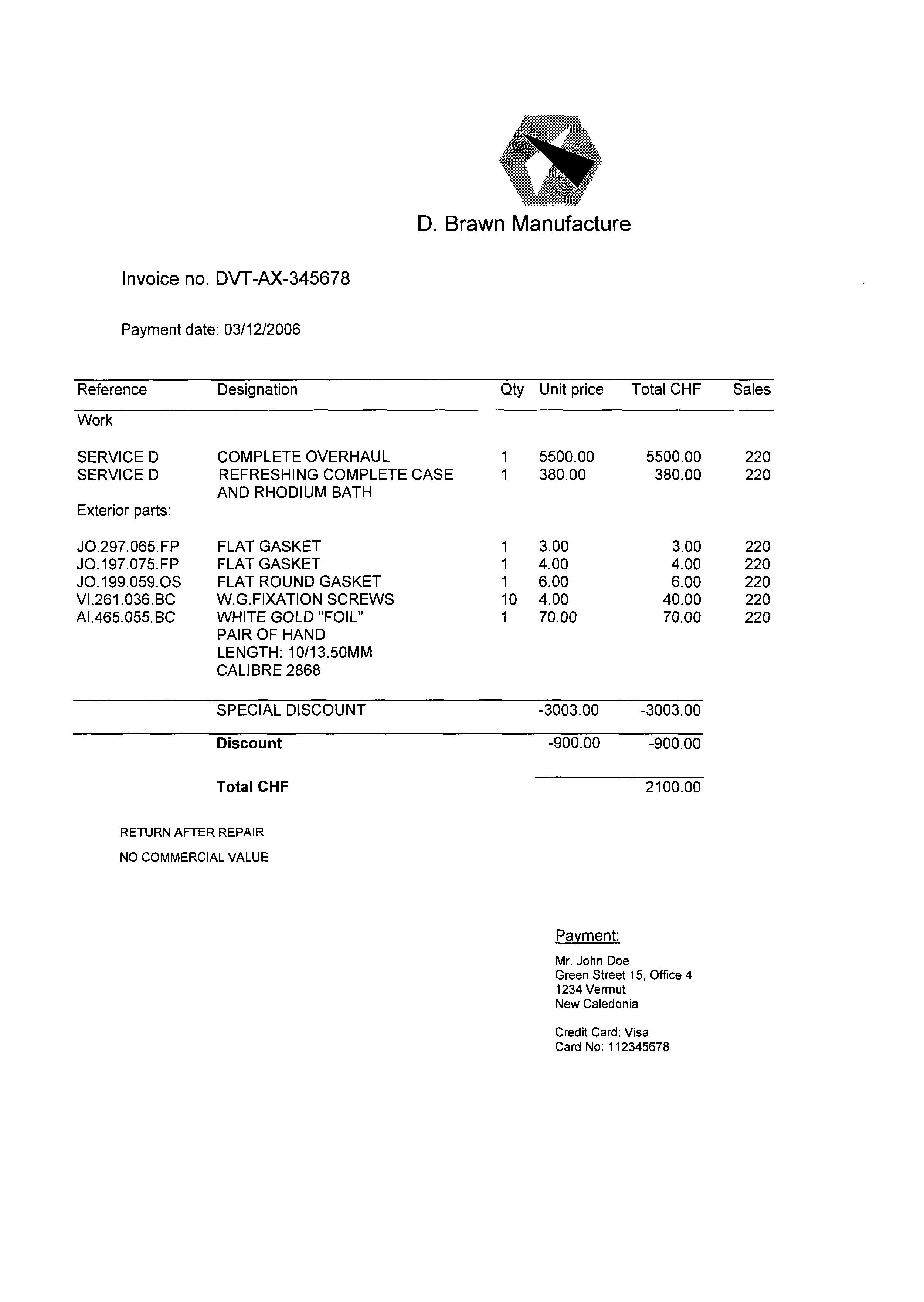

将OCR应用于图像 (Applying OCR to the Image)
Once we have marked the regions of interest (along with the respective coordinates) we can simply crop the original image for the particular region and pass it through pytesseract to get the results.
一旦我们标记了感兴趣的区域(以及相应的坐标),我们就可以裁剪特定区域的原始图像,并将其通过pytesseract以获得结果。
For those who are new to Python and OCR, pytesseract can be an overwhelming word. According to its official website -
对于那些不熟悉Python和OCR的人,pytesseract可能是一个压倒性的词。 根据其官方网站-
Python-tesseract is a wrapper for Google’s Tesseract-OCR Engine. It is also useful as a stand-alone invocation script to tesseract, as it can read all image types supported by the Pillow and Leptonica imaging libraries, including jpeg, png, gif, bmp, tiff, and others. Additionally, if used as a script, Python-tesseract will print the recognized text instead of writing it to a file.
Python-tesseract是Google Tesseract-OCR Engine的包装。 它也可以用作tesseract的独立调用脚本,因为它可以读取Pillow和Leptonica图像库支持的所有图像类型,包括jpeg,png,gif,bmp,tiff等。 此外,如果将Python-tesseract用作脚本,它将打印识别的文本,而不是将其写入文件。
Also, if you want to play around with the configuration parameters of pytesseract, I would recommend to go through the below links first.
另外,如果您想使用pytesseract的配置参数,我建议您先浏览以下链接。
The following code can be used to perform this task.
以下代码可用于执行此任务。
import pytesseract
pytesseract.pytesseract.tesseract_cmd = r'C:\Users\Akash.Chauhan1\AppData\Local\Tesseract-OCR\tesseract.exe'# load the original image
image = cv2.imread('Original_Image.jpg')# get co-ordinates to crop the image
c = line_items_coordinates[1]# cropping image img = image[y0:y1, x0:x1]
img = image[c[0][1]:c[1][1], c[0][0]:c[1][0]] plt.figure(figsize=(10,10))
plt.imshow(img)# convert the image to black and white for better OCR
ret,thresh1 = cv2.threshold(img,120,255,cv2.THRESH_BINARY)# pytesseract image to string to get results
text = str(pytesseract.image_to_string(thresh1, config='--psm 6'))
print(text)

Output from OCR:
OCR的输出:
Payment:Mr. John DoeGreen Street 15, Office 41234 VermutNew Caledonia

Output from OCR
OCR的输出
COMPLETE OVERHAUL 1 5500.00 5500.00 220REFRESHING COMPLETE CASE 1 380.00 380.00 220AND RHODIUM BATH
As you can see, the accuracy of our output is 100%.
如您所见,我们输出的准确性为100%。
So this was all about how you can develop a solution for extracting data from a complex document such as invoices.
因此,这就是如何开发一种解决方案来从发票等复杂文档中提取数据的全部内容。
There are many applications to what OCR can do in term of document intelligence. Using pytesseract, one can extract almost all the data irrespective of the format of the documents (whether its a scanned document or a pdf or a simple jpeg image).
OCR在文件智能方面可以做很多应用。 使用pytesseract,可以提取几乎所有数据,而不管文档的格式如何(无论是扫描的文档还是pdf或简单的jpeg图像)。
Also, since its open source, the overall solution would be flexible as well as not that expensive.
而且,由于它是开源的,所以整体解决方案将是灵活的,而且不会那么昂贵。
翻译自: https://towardsdatascience.com/extracting-text-from-scanned-pdf-using-pytesseract-open-cv-cd670ee38052
open cv roi提取
相关文章:
- 四氧化三铁负载碳纳米管/多壁碳纳米管/磁性纳米粒子 Fe3O4-MNPs/CNTs/MCNTs的制备
- DLPC-PEG-CNTs/MCNTs/AuNPs/MNPs/IONPs 二亚油酰磷脂酰胆碱-聚乙二醇-纳米材料
- Open-cv中由cv2.drawContours(contours_img,cnts,-1,(0,0,255),3) 造成的cv2.error
- 11.20打卡python
- 五. 防护设计学习笔记
- SURGE 浪涌抗扰度
- 助力车主控板0.8KV浪涌的选型及测试
- 浪涌(Surge)抗扰度标准解读
- PyTorch-上分之路(基于Vision Transformer的鸟群分类算法)
- 基于PSO粒子群优化算法的TSP问题最短路径求解matlab仿真
- 【车间调度】鸟群算法求解车间调度问题【含Matlab源码 1395期】
- 路径规划算法:基于鸟群优化的路径规划算法- 附代码
- 【无标题】速度环中限制加速度与加加速度的积分处理
- 飞控学习笔记-姿态角解算(MPU6050 加速度计加陀螺仪)
- SAP 财务固定资产常用BAPI
- 固定资产有收入的报废、无收入的报废 的冲销
- 固定资产报废BAPI
- abap中的BAPI_ASSET_RETIREMENT_POST (固定资产报废BAPI)
- SAP ABAVN固定资产中实现多重资产的批量报废
- [BAPI]固定资产报废-BAPI_ASSET_RETIREMENT_POST
- ABAP 固定资产报废的BAPI
- Web课程总结
- 图像处理与机器视觉 综合课程设计
- python培训课程背景
- Oracle01-课程背景-基本概念-DQL查询语句
- BASIS工作内容
- 知道等级保护测评都有哪些工作内容吗?
- Http | 工作机制
- 安全服务的工作内容
- ppt压缩文件大小,4个压缩教程
open cv roi提取_使用pytesseract open cv从扫描的pdf中提取文本相关推荐
- 怎么从pdf中提取图片?三招告诉你如何从pdf中提取图片
众所周知,PDF的格式对于一些重要文件的保存,以及隐私文件的保护来说都是非常好用的.同时,如果要将PDF格式的文件转换成其它格式的文件也挺方便的.因此,PDF格式在日常办公中具有较高的使用率.那么我们 ...
- linux中将文本中的单词换掉的指令_为什么说从PDF中提取文本是一件困难的事?...
PDF文档处理工作中,总是绕不开对文本提取的需求.很多用户觉得我们PDFlux好用,所以对其中的底层技术也非常感兴趣.也有人为认为,从PDF里抽取文本段落和表格,应该非常简单! 近期,我们会对PDF文 ...
- Camelot:从pdf中提取表格数据
Camelot:从pdf中提取表格数据 文章目录: 一.Camelot的介绍和安装 1. Camelot介绍 2. Camelot的安装 3. 其他 二.Camelot的使用 1. 快速入门使用 2. ...
- 从 pdf 中提取表格信息、合并、解析、输出数据
从 pdf 中提取表格信息.合并.解析.输出 pdf 格式浅述 word 文档 与 pdf pdf 文档撰写的优点与难点 从 pdf 中抽取表格所在的页 从 pdf 中抽取表格 合并表格.解析表格.生 ...
- pdfparser java_如何使用java从PDF中提取内容?
在Java编程中,如何使用java从PDF中提取内容? 项目的目录结构如下 - Tika的工具包可从以下网址下载:http://tika.apache.org/download.html ,只下载:t ...
- python处理pdf提取指定数据_python从PDF中提取数据的示例
01 前言 数据是数据科学中任何分析的关键,大多数分析中最常用的数据集类型是存储在逗号分隔值(csv)表中的干净数据.然而,由于可移植文档格式(pdf)文件是最常用的文件格式之一,因此每个数据科学家都 ...
- python从字符串中提取数字并转换为相应数据类型_python从PDF中提取数据的示例
01 前言 数据是数据科学中任何分析的关键,大多数分析中最常用的数据集类型是存储在逗号分隔值(csv)表中的干净数据.然而,由于可移植文档格式(pdf)文件是最常用的文件格式之一,因此每个数据科学家都 ...
- PDF编辑方法,怎么从PDF中提取页面
随着大家在工作中使用到PDF文件的情况越来越多,怎么编辑PDF文件也是大家常常谈论的话题,PDF文件的编辑是需要用到PDF编辑器的,那么,在编辑文件的时候,怎么提取PDF文件的页面呢,不会的小伙伴可以 ...
- html 提取pdf,使用PDF.js从PDF中提取文本(2019)
正如标题所说,我正在尝试使用由Mozilla维护的PDF.js从PDF中提取文本.我知道前面关于stackoverflow的问题,但我不知道从哪里开始. 我试着跟着这个 article 这件事我需要帮 ...
最新文章
- python里感叹号什么意思_仪表盘上的感叹号是什么意思
- 张家口以太坊智能合约开发实战pdf_以太坊的再次腾飞,你看得懂么?
- Java设计模式百例(番外) - Java的clone
- 编写你的第一个 Django 应用,第 3 部分
- hdu 1006 Tick and Tick
- Selector 实现原理
- 【更新】深度学习推荐系统
- python自动翻译excel某一列_【python excel实例教程】怎样用Python将excel的某一列生成一个列表?...
- 业界 | 成为CTO之前,我希望有人告诉我这些
- Tensorflow训练mnist数据集源代码解析
- html添加变量参数吗,动态CSS与变量参数? (可能吗?)
- python编写水仙花数
- SQL2008卸载。
- 5G系统中BBU与RRU之间前传接口(CPRI)带宽计算
- 学习的爬虫一点小感悟附上爬取淘宝信息的教程
- ubuntu系统打不开网易云音乐解决办法。
- java 设置纸张大小设置_java page如何设置纸张
- 若依项目环境搭建及使用
- 【Python 爬虫实践】:《战狼2》豆瓣影评分析
- qt textbrowser的边界框怎样改变颜色_专访天使投资人续沛川:用深度思考打破人生边界,拥有张力一生...
热门文章
- “中国IT就业训练营”正式启动 首次校园公开课成功举办
- 高校宿舍预付费用电管理平台
- Android 自定义控件基础:MeasureSpec
- 网页中在线玩拳皇KOF
- 2020-12-31小黄自学文档整理(其实是小白,因为姓黄)
- Pytest 结合 Allure 生成测试报告
- Hackthebox - Previse 靶场实战
- sourceinsight4.0破解教程及下载
- 【GAMES103学习笔记】线性系统(Linear System)及其解法(Linear Sovler)
- tessafe.sys不兼容驱动程序怎么解决?