Enterprise Library 2.0 技巧(4):如何用编程的方法来配置Logging Application Block
在本系列的技巧(1)和技巧(2)中分别介绍了使用外部配置文件,使用数据库记录配置信息两种方法,不知道大家有没有想过不使用任何配置文件,也不使用数据库而直接用编程的方法来实现呢?本文将会展示如何使用编程的方法来配置Logging Application Block。首先我们需要了解一下Logging Application Block中比较重要的几个对象:
1.LogFormatter
格式化对象,LogFormatter有TextFormatter和BinaryFormatter两种,多数情况下我们会使用TextFormatter,它通过一个Template来创建,一个完整的Template格式如下:
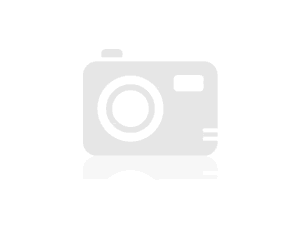
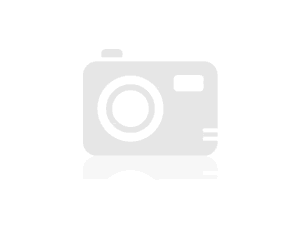
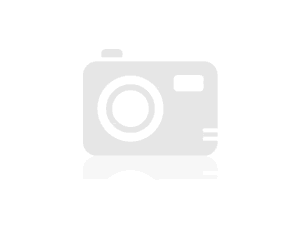
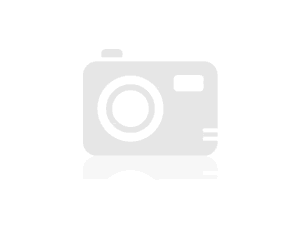
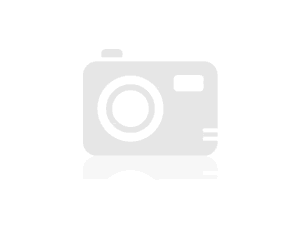
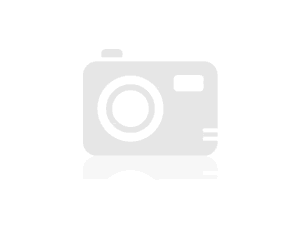
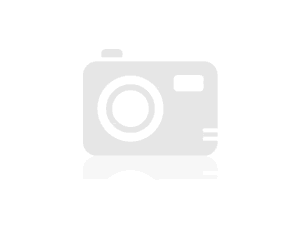
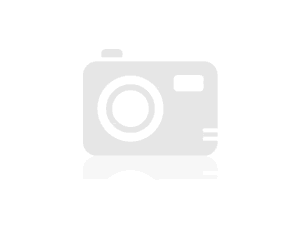
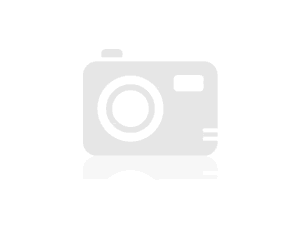
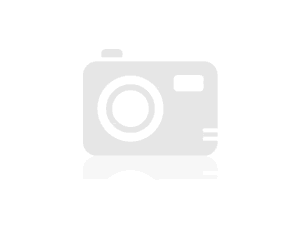
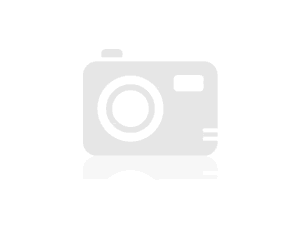
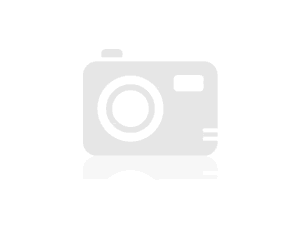
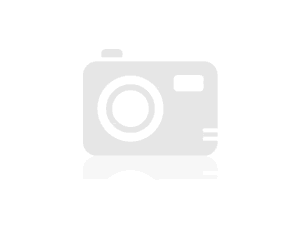
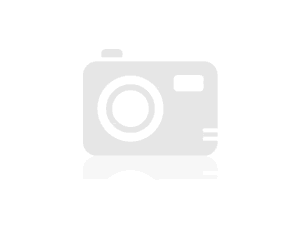
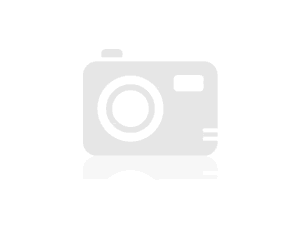
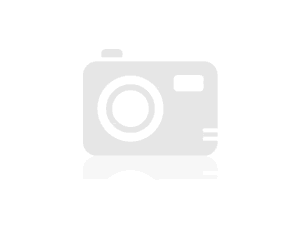
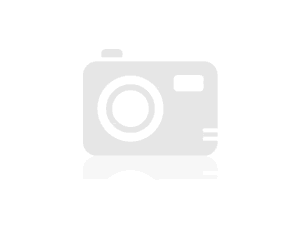
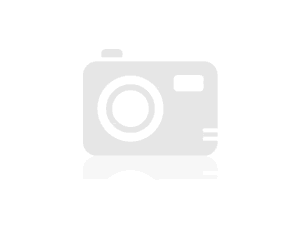
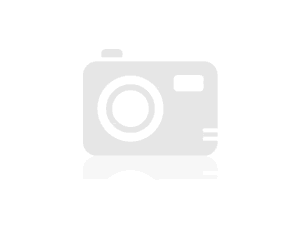
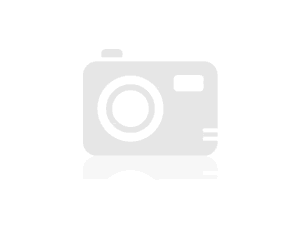
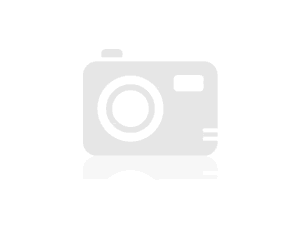
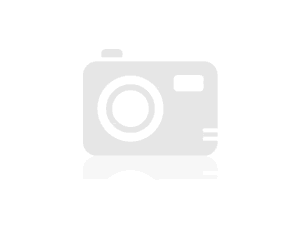
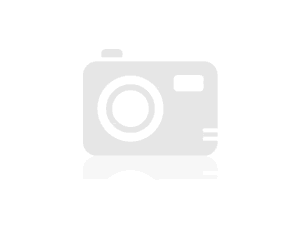
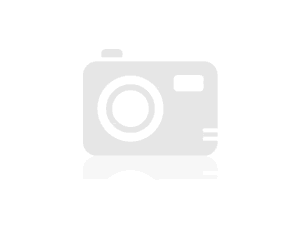
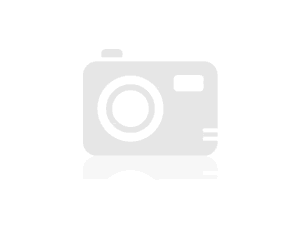
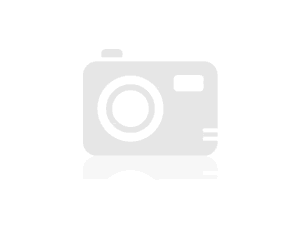
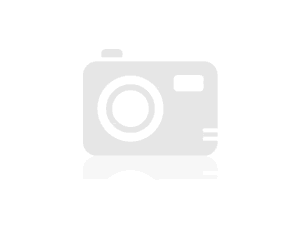
这里就不具体解释每一项的含义,大家可以参考有关文档,示例代码:
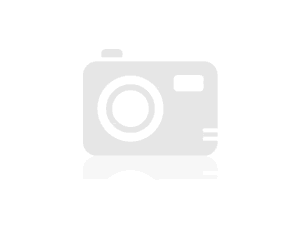
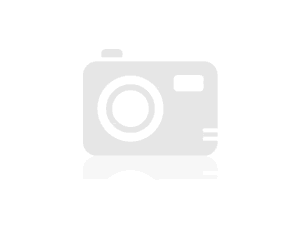
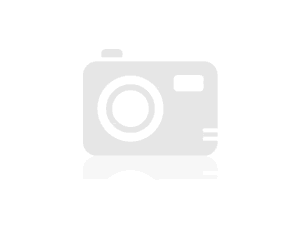
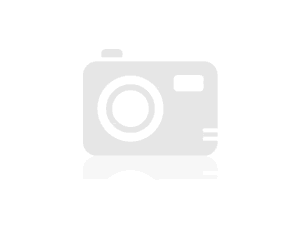
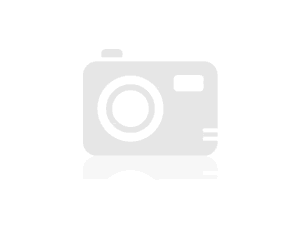
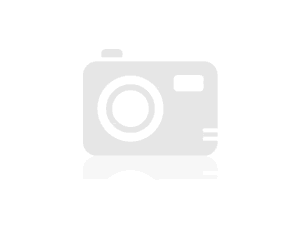
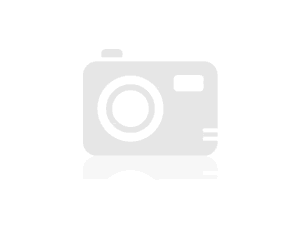
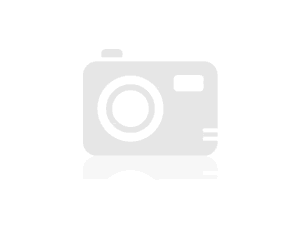
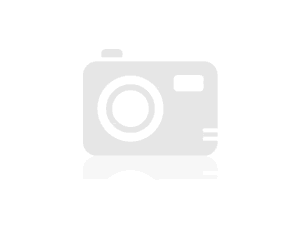
2.TraceListener
TraceListener提供了日志记录服务,它指定的是日志将被记录到何处,数据库中或者是文本文件,Enterprise Library提供了7种
TraceListener:Database TraceListener、Email TraceListener、Flat File TraceListener、Formatter Event Log TraceListener、Msmq TraceListener、System Diagnostics TraceListener、WMI Trace Listener。每一种TraceListener都需要一个LogFormatter来对记录的信息进行格式化,例如创建一个FlatFileTraceListener实例:
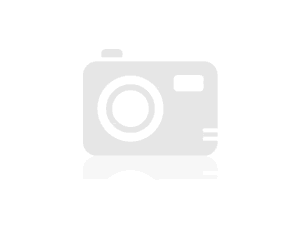
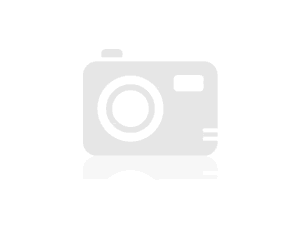
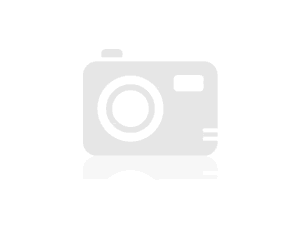
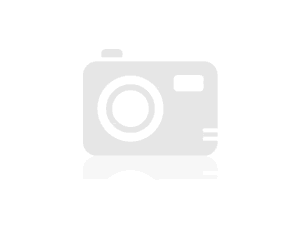
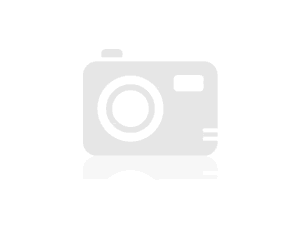
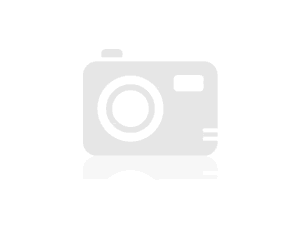
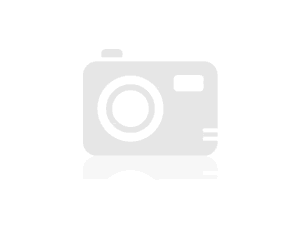
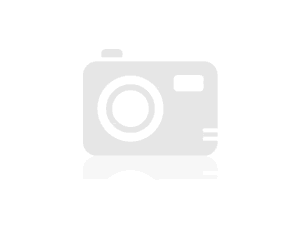
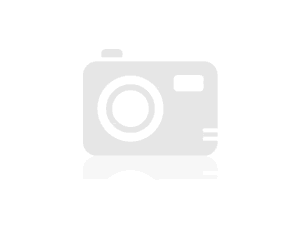
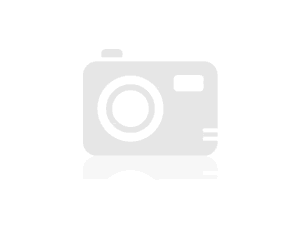
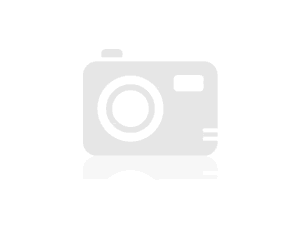
这里的formatter就是在上面创建的TextFormatter对象。
3.LogSource
LogSource其实就是TraceListener的集合,Enterprise Library允许针对不同的日志信息记录到不同地方,因此可以在LogSource中加入多个TraceListener:
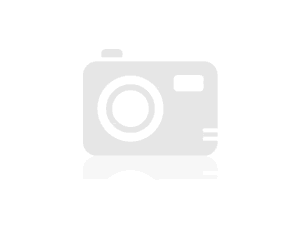
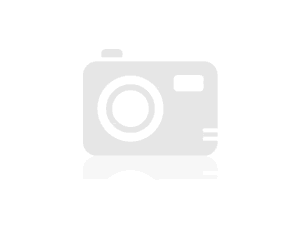
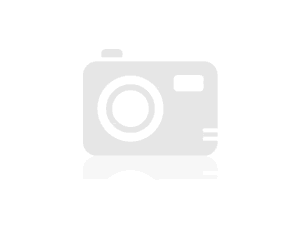
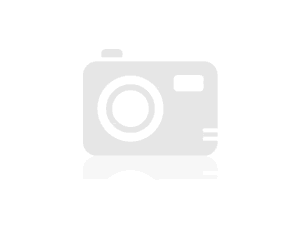
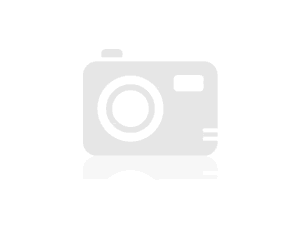
4.LogFilter
过滤器,对日志信息进行过滤,Enterprise Library默认提供了三种过滤器,用户也可以定义自己的过滤器,示例代码:
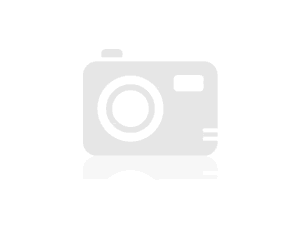
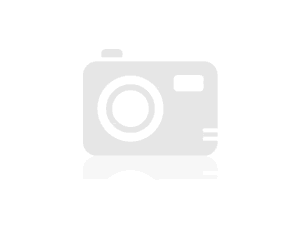
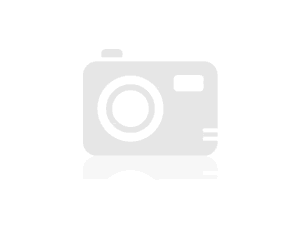
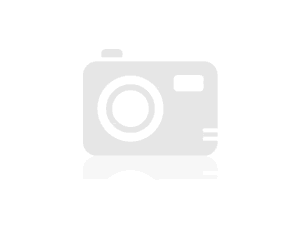
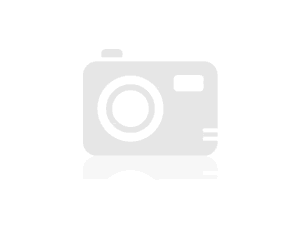
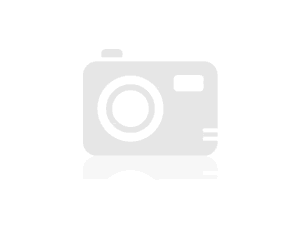
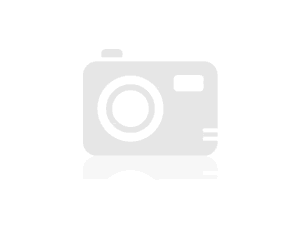
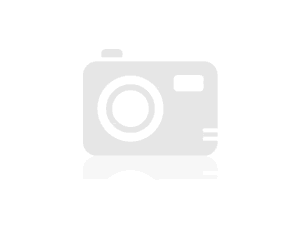
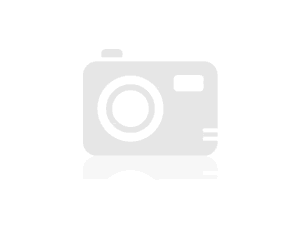
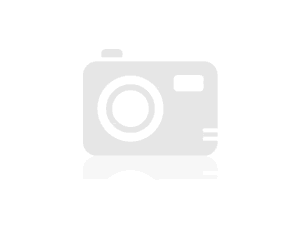
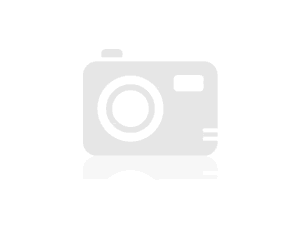
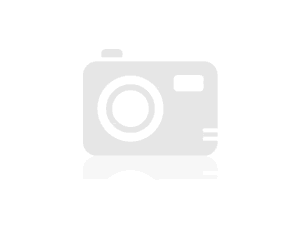
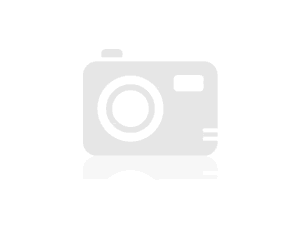
了解了这四个对象,其实我们就已经知道了该如何去用编程的方法配置Logging Application Block,下面给出一个简单的例子,先写一个MyLogger静态类:
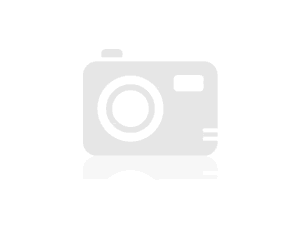
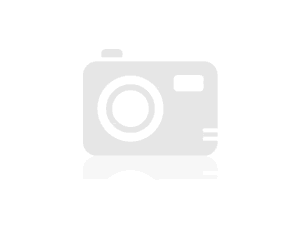
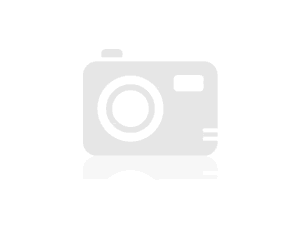
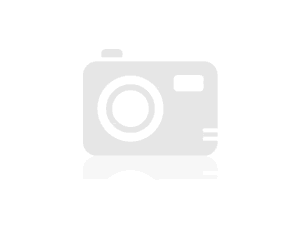
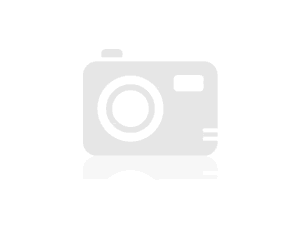
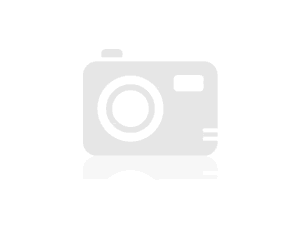
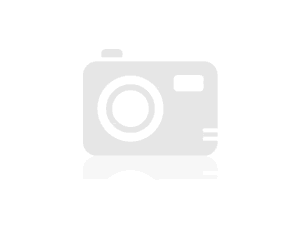
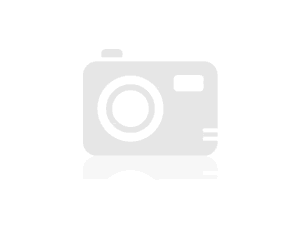
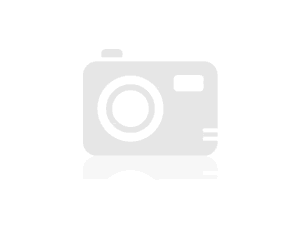
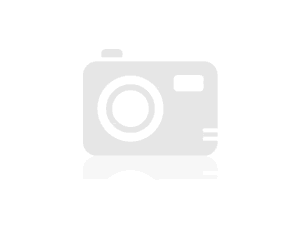
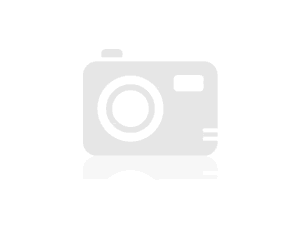
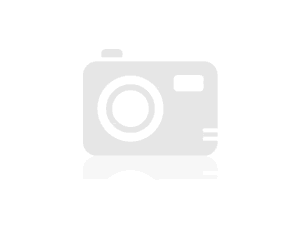
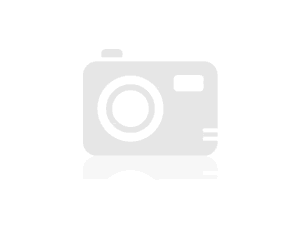
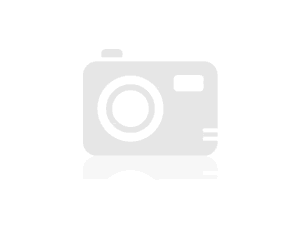
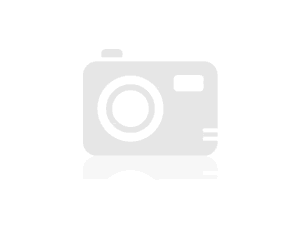
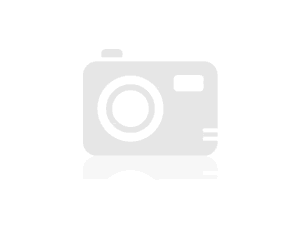
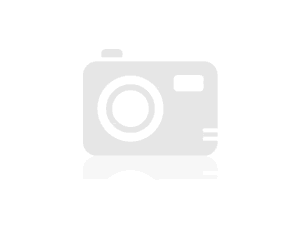
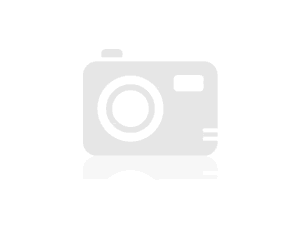
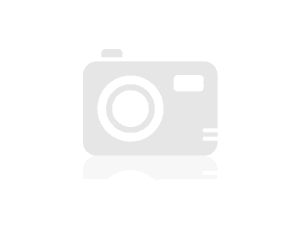
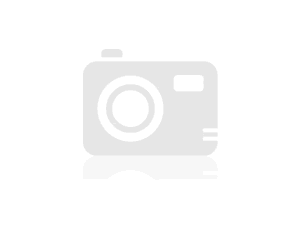






























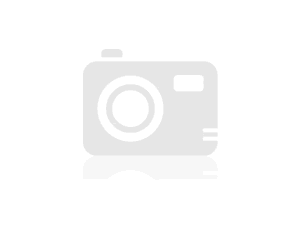












































































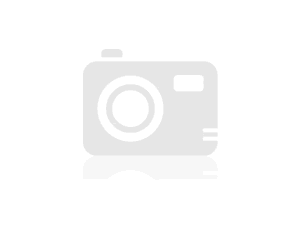










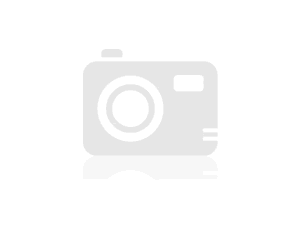







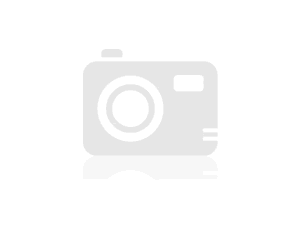












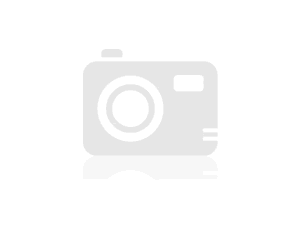
















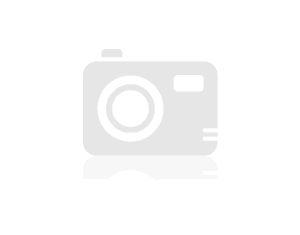
我们再来写一个简单的测试,注意上面的代码中我们过滤掉了Debug类别的日志信息,这样记录到文本文件中的日志信息应该只有My Error一条:
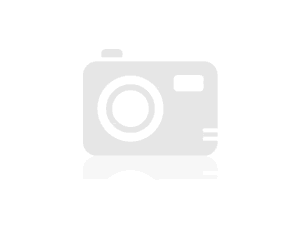
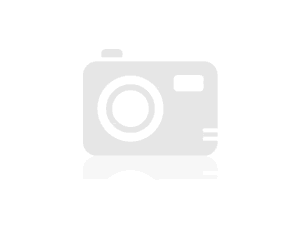
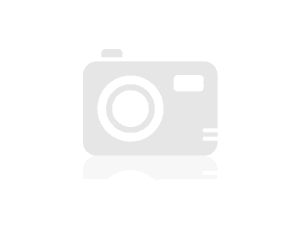
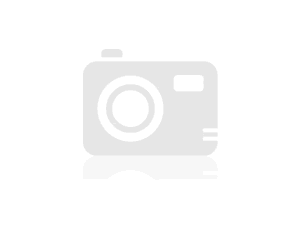





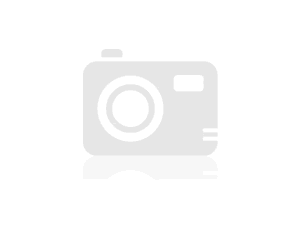








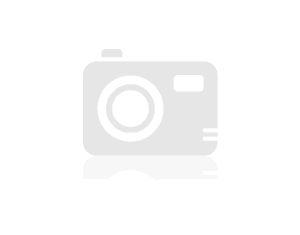
文本文件中输出的结果为:
----------
Timestamp: 2006-7-8 3:45:05
Message: My Error
Category: Error
Machine: RJ-097
----------
输出的结果与我们设想的一致,使用编程的方法配置Logging Application Block简单的就介绍到这里,你也可以使用这种方法来配置其他的应用程序块。不过使用编程的方法来配置,失去了EL的灵活性,要知道EL的根本思想就是配置驱动,但是如果掌握了这些,也许你能够更好的使用EL,在CodeProject上有人写了一篇《Plug-in Manager for Logging - Configure MSEL2 On the fly》,有兴趣的朋友不妨参考一下。
参考:
http://davidhayden.com/blog/dave/archive/2006/02/18/2805.aspx
http://geekswithblogs.net/akraus1/archive/2006/02/16/69784.aspx
转载于:https://www.cnblogs.com/Terrylee/archive/2006/07/08/enterprise_library2_4.html
Enterprise Library 2.0 技巧(4):如何用编程的方法来配置Logging Application Block相关推荐
- Enterprise Library 2.0 技巧(3):记录ASP.NET站点中未处理的异常
这篇文章不能算是Enterprise Library 2.0的一个技巧,只是Logging Application Block的一个简单应用而已,在这里我们使用Logging Application ...
- Enterprise Library 2.0 技巧(1):如何使用外部配置文件
摘要:我们知道在Enterprise Library1.1中对于每一个应用程序块都有一个对应的配置文件,而在Enterprise Library2.0中却把所有的配置信息都放在了应用程序配置文件(Ap ...
- Enterprise Library 3.0 发布
微软今天发布了Enterprise Library 3.0,又有新的东西可以研究了,在Enterprise Library 3.0中包含如下8个应用程序块: l Caching App ...
- Microsoft Enterprise Library 简介与请大家下载Microsoft Enterprise Library 5.0体验微软最新技术应用于企业信息平台
什么是Enterprise Library Enterprise Library是一组应用程序块(Application Block)的集合.他们是可重用的软件组件,被设计用来帮助开发者面对常 ...
- 黄聪:Microsoft Enterprise Library 5.0 系列教程(二) Cryptography Application Block (高级)
原文:黄聪:Microsoft Enterprise Library 5.0 系列教程(二) Cryptography Application Block (高级) 本章介绍的是企业库加密应用程序模块 ...
- Microsoft Enterprise Library 4.0 for Visual Studio 2008
Microsoft Enterprise Library 4.0 for Visual Studio 2008已经发布,可以下载看看 转载于:https://www.cnblogs.com/rover ...
- Enterprise Library 3.0 体验(3):使用配置文件的Validation Application Block
摘要:Enterprise Library 3.0 January 2007 CTP版发布了,这次发布的版对于Validation Application Block有了很大的改进,包括对配置工具的支 ...
- Enterprise Library 3.0 安装过程
微软刚刚发布Enterprise Library 3.0,其更新程度非常大,功能非常强大. 3.0的安装过程却非常复杂,下面是安装过程记录,希望对应用Entlib3.0的人有所借鉴. 相关的介绍资源 ...
- Enterprise Library 5.0发布
Microsoft Enterprise Library 5.0是一套可重用的应用程序块,帮助开发人员进行企业应用开发.包括:Caching Block.Cryptography Block.Data ...
最新文章
- C++ 11 新特性 nullptr 学习
- bat命令 修改ini文件内容_Linux文件内容查看相关命令
- 如何使用阿里云容器服务保障容器的内存资源质量
- DNA repair - HDU 2457(自动机+dp)
- c盘怎么清理到最干净_C盘快满了不敢乱删,该如何清理?这里给你最详细的方法!...
- [Python人工智能] 三.TensorFlow基础之Session、变量、传入值和激励函数
- sql如何遍历几百万的表_SQL Server遍历表中记录的2种方法(使用表变量和游标)
- jbb是什么梗_子水是什么意思,子水命理
- 原子变量 java_Java原子变量详解
- Robot Framework中经常用的第三方库的安装方法
- 《威胁建模:设计和交付更安全的软件》——3.11 小结
- 思维方式-《策略思维》书中的精髓:生活工作中博弈无处不在,理解博弈论、善用策略思维,能帮助我们做出更好的决策。
- kafka 修改分区_kafka分区
- UEditor CKEditor 网页编辑器
- 华硕重装后进入bios_华硕电脑如何进入bios,教您如何华硕进入bios
- 网络管理——直接网络管理规范
- c语言程序设计 超市收银设计,C语言超市收银系统方案
- 二、马尔可夫决策过程与贝尔曼方程
- Excel做 “回归分析”,你可能都没玩儿过!
- 调用接口时报:Required String parameter '' is not present