神经网络 代码python_详细使用Python代码和数学构建神经网络— II
神经网络 代码python
机器学习 , 学术 , 教程 (Machine Learning, Scholarly, Tutorial)
Author(s): Pratik Shukla, Roberto Iriondo
作者:Pratik Shukla,Roberto Iriondo
Last updated, June 30, 2020
上次更新时间:2020年6月30日
In the first part of our tutorial on neural networks, we explained the basic concepts about neural networks, from the math behind them to implementing neural networks in Python without any hidden layers. We showed how to make satisfactory predictions even in case scenarios where we did not use any hidden layers. However, there are several limitations to single-layer neural networks.
在神经网络教程的第一部分中,我们解释了有关神经网络的基本概念,从其背后的数学到在Python中没有任何隐藏层的实现神经网络。 我们展示了即使在不使用任何隐藏层的情况下,如何做出令人满意的预测。 但是,单层神经网络存在一些限制。
In this tutorial, we will dive in-depth on the limitations and advantages of using neural networks in machine learning. We will show how to implement neural nets with hidden layers and how these lead to a higher accuracy rate on our predictions, along with implementation samples in Python on Google Colab.
在本教程中,我们将深入探讨在机器学习中使用神经网络的局限性和优势。 我们将展示如何使用隐藏层实现神经网络,以及如何在我们的预测中提高隐藏率,以及Google Colab中Python的实现示例。
指数: (Index:)
Limitations and advantages of neural networks
神经网络的局限性和优势
How to select several neurons in a hidden layer.
如何在隐藏层中选择几个神经元。
The general structure of an artificial neural network (ANN).
人工神经网络(ANN)的一般结构。
Implementation of a multilayer neural network in Python.
在Python中实现多层神经网络。
Comparison with a single-layer neural network.
与单层神经网络的比较。
Non-linearly separable data with a neural network.
具有神经网络的非线性可分离数据。
Conclusion.
结论。
1.神经网络的局限性和优势 (1. Limitations and Advantages of Neural Networks)
单层神经网络的局限性: (Limitations of single-layer neural networks:)
- They can only represent a limited set of functions. If we have been training a model that uses complicated functions (which is the general case), then using a single layer neural network can lead to low accuracy in our prediction rate.它们只能代表一组有限的功能。 如果我们一直在训练使用复杂函数的模型(这是一般情况),那么使用单层神经网络可能会导致我们的预测率准确性降低。
- They can only predict linearly separable data. If we have non-linear data, then training our single-layer neural network will lead to low accuracy in our prediction rate.他们只能预测线性可分离的数据。 如果我们具有非线性数据,那么训练我们的单层神经网络将导致我们的预测率准确性降低。
- Decision boundaries for single-layer neural networks must be in the hyperplane, which means that if our data distributes in 3 dimensions, then our decision boundary must be in 2 dimensions.单层神经网络的决策边界必须在超平面中,这意味着如果我们的数据以3维分布,则我们的决策边界必须以2维分布。

To overcome such limitations, we use hidden layers in our neural networks.
为了克服这些限制,我们在神经网络中使用了隐藏层。
单层神经网络的优点: (Advantages of single-layer neural networks:)
- Single-layer neural networks are easy to set up.单层神经网络很容易建立。
- Single-layer neural networks take less time to train compared to a multi-layer neural network.与多层神经网络相比,单层神经网络花费的时间更少。
- Single-layer neural networks have explicit links to statistical models.单层神经网络与统计模型有明确的链接。
- The outputs in single layer neural networks are weighted sums of inputs. It means that we can interpret the output of a single layer neural network feasibly.单层神经网络中的输出是输入的加权和。 这意味着我们可以切实地解释单层神经网络的输出。
多层神经网络的优点: (Advantages of multilayer neural networks:)
- They construct more extensive networks by considering layers of processing units.他们通过考虑处理单元的层来构建更广泛的网络。
- They can be used to classify non-linearly separable data.它们可用于对非线性可分离数据进行分类。
- Multilayer neural networks are more reliable compared to single-layer neural networks.与单层神经网络相比,多层神经网络更可靠。
2.如何在隐藏层中选择几个神经元? (2. How to select several neurons in a hidden layer?)
There are many methods for determining the correct number of neurons to use in the hidden layer. We will see a few of them here.
有许多方法可以确定要在隐藏层中使用的神经元的正确数量。 我们将在这里看到其中的一些。
- The number of hidden nodes should be less than twice the size of the nodes in the input layer.隐藏节点的数量应小于输入层中节点大小的两倍。
For example: If we have 2 input nodes, then our hidden nodes should be less than 4.
例如:如果我们有2个输入节点,那么我们的隐藏节点应小于4。
a. 2 inputs, 4 hidden nodes:
一个。 2个输入,4个隐藏节点:

b. 2 inputs, 3 hidden nodes:
b。 2个输入,3个隐藏节点:

c. 2 inputs, 2 hidden nodes:
C。 2个输入,2个隐藏节点:

d. 2 inputs, 1 hidden node:
d。 2个输入,1个隐藏节点:

- The number of hidden nodes should be 2/3 the size of input nodes, plus the size of the output node.隐藏节点的数量应为输入节点大小的2/3加上输出节点的大小。
For example: If we have 2 input nodes and 1 output node then the hidden nodes should be = floor(2*2/3 + 1) = 2
例如:如果我们有2个输入节点和1个输出节点,则隐藏节点应为= floor(2 * 2/3 + 1)= 2
a. 2 inputs, 2 hidden nodes:
一个。 2个输入,2个隐藏节点:

- The number of hidden nodes should be between the size of input nodes and output nodes.隐藏节点的数量应在输入节点和输出节点的大小之间。
For example: If we have 3 input nodes and 2 output nodes, then the hidden nodes should be between 2 and 3.
例如:如果我们有3个输入节点和2个输出节点,则隐藏节点应在2到3之间。
a. 3 inputs, 2 hidden nodes, 2 outputs:
一个。 3个输入,2个隐藏节点,2个输出:

b. 3 inputs, 3 hidden nodes, 2 outputs:
b。 3个输入,3个隐藏节点,2个输出:

我们需要多少个体重值? (How many weight values do we need?)
- For a hidden layer: Number of inputs * No. of hidden layer nodes对于隐藏层:输入数量*隐藏层节点数
- For an output layer: Number of hidden layer nodes * No. of outputs对于输出层:隐藏层节点数*输出数量
3.人工神经网络(ANN)的一般结构: (3. The General Structure of an Artificial Neural Network (ANN):)

人工神经网络的概述: (Summarization of an artificial neural network:)
- Take inputs.接受输入。
- Add bias (if required).添加偏见(如果需要)。
- Assign random weights in the hidden layer and the output layer.在隐藏层和输出层中分配随机权重。
- Run the code for training.运行代码进行培训。
- Find the error in prediction.查找预测中的错误。
- Update the weight values of the hidden layer and output layer by gradient descent algorithm.通过梯度下降算法更新隐藏层和输出层的权重值。
- Repeat the training phase with updated weights.使用更新的权重重复训练阶段。
- Make predictions.作出预测。
多层神经网络的执行: (Execution of multilayer neural networks:)
After reading the first article, we saw that we had only 1 phase of execution there. In that phase, we find the updated weight values and rerun the code to achieve minimum error. However, things are a little spicy here. The execution in a multilayer neural network takes place in two-phase. In phase-1, we update the values of weight_output (weight values for output layer), and in phase-2, we update the value of weight_hidden ( weight values for the hidden layer ). Phase-1 is similar to that of a neural network without any hidden layers.
阅读第一篇文章后,我们看到那里只有一个执行阶段。 在该阶段,我们找到更新的权重值,然后重新运行代码以实现最小错误。 但是,这里的东西有点辣。 多层神经网络中的执行分两阶段进行。 在阶段1中,我们更新weight_output的值(输出层的权重值),在阶段2中,我们更新weight_hidden的值(隐藏层的权重值)。 第一阶段类似于没有任何隐藏层的神经网络。
在第一阶段执行: (Execution in phase-1:)
To find the derivative, we are going to use in gradient descent algorithm to update the weight values. Here we are not going to derive the derivatives for those functions we already did in part -1 of neural network.In this phase, our goal is to find the weight values for the output layer. Here we are going to calculate the change in error concerning the change in output weight.
为了找到导数,我们将使用梯度下降算法来更新权重值。 在这里,我们将不为在神经网络的第-1部分中已经完成的那些函数导出导数。在此阶段,我们的目标是找到输出层的权重值。 在这里,我们将计算与输出重量变化有关的误差变化。
We first define some terms we are going to use in these derivatives:
我们首先定义将在这些派生词中使用的一些术语:

a. Finding the first derivative:
一个。 查找一阶导数:

b. Finding the second derivative:
b。 寻找二阶导数:

c. Finding the third derivative:
C。 找到三阶导数:

Notice that we already derived these derivatives in the first part of our tutorial.
注意,我们已经在本教程的第一部分中导出了这些导数。
在第二阶段执行: (Execution in phase-2:)
In phase-1, we find the updated weight for the output layer. In the second phase, we need to find the updated weights for the hidden layer. Hence, find how the change in hidden weight affects the change in error value.
在阶段1中,我们找到了输出层的更新权重。 在第二阶段,我们需要找到隐藏层的更新权重。 因此,发现隐藏权重的变化如何影响误差值的变化。
Represented as:
表示为:
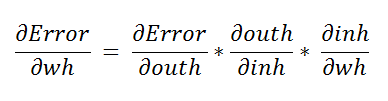
a. Finding the first derivative:
一个。 查找一阶导数:
Here we are going to use the chain rule to find the derivative.
在这里,我们将使用链式规则找到导数。
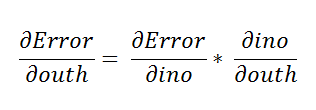
Using the chain rule again.
再次使用链式规则。

The step below is similar to what we did in the first part of our tutorial on neural networks.
下面的步骤类似于我们在神经网络教程的第一部分中所做的步骤。

b. Finding the second derivative:
b。 寻找二阶导数:

c. Finding the third derivative:
C。 找到三阶导数:

4.用Python实现多层神经网络 (4. Implementation of a multilayer neural network in Python)
神经网络 代码python_详细使用Python代码和数学构建神经网络— II相关推荐
- 联邦学习算法介绍-FedAvg详细案例-Python代码获取
联邦学习算法介绍-FedAvg详细案例-Python代码获取 一.联邦学习系统框架 二.联邦平均算法(FedAvg) 三.联邦随梯度下降算法 (FedSGD) 四.差分隐私随联邦梯度下降算法 (DP- ...
- 如何防止你的代码被窃取?Python代码加密方案汇总(带实例验证)
Python代码加密方案汇总 文章目录 Python代码加密方案汇总 需求描述 基础:Python文件格式 `.py` python源代码 `.pyc` 编译得到的字节码文件 `.pyo` 编译优化后 ...
- DeepMind提图像生成的递归神经网络DRAW,158行Python代码复现
作者 | Samuel Noriega 译者 | Freesia 编辑 | 夕颜 出品 | AI科技大本营(ID: rgznai100) [导读]最近,谷歌 DeepMInd 发表论文( DRAW: ...
- python代码编程教学入门,python代码编程火影忍者
python源代码编程软件 编写python源代码的软件.首推的Pycharm. PyCharm用于bai一般IDE具备的功能,比如, 调试.语法高亮.Project管理.du代码跳转.智能提示.自动 ...
- python代码大全p-21行Python代码实现拼写检查器
引入 大家在使用谷歌或者百度搜索时,输入搜索内容时,谷歌总是能提供非常好的拼写检查,比如你输入 speling,谷歌会马上返回 spelling. 下面是用21行python代码实现的一个简易但是具备 ...
- python语言代码片段-有用的Python代码片段
我列出的这些有用的Python代码片段,为我节省了大量的时间,并且我希望他们也能为你节省一些时间.大多数的这些片段出自寻找解决方案,查找博客和StackOverflow解决类似问题的答案.下面所有的代 ...
- python写一个游戏多少代码-使用50行Python代码从零开始实现一个AI平衡小游戏
集智导读: 本文会为大家展示机器学习专家 Mike Shi 如何用 50 行 Python 代码创建一个 AI,使用增强学习技术,玩耍一个保持杆子平衡的小游戏.所用环境为标准的 OpenAI Gym, ...
- python代码需要背吗-python代码运行需要编译吗
有人在讨论 Python 代码是编译执行还是解释执行?这个问题还可以换一种说法: Python 是编译型语言还是解释型语言?回答这个问题 前,我们先弄清楚什么是编译型语言,什么是解释型语言. 所谓编译 ...
- python语言必背代码-让你的Python代码实现类型提示功能
Python是一种动态类型语言,这意味着我们在编写代码的时候更为自由,但是与此同时IDE无法向静态类型语言那样分析代码,及时给我们相应的提示.为了解决这个问题,Python 3.6 新增了几个特性PE ...
- python代码需要背吗-Python代码需要缩进吗
Python则是通过缩进来识别代码块的. 缩进 Python最具特色的是用缩进来标明成块的代码.我下面以if选择结构来举例.if后面跟随条件,如果条件成立,则执行归属于if的一个代码块. 先看C语言的 ...
最新文章
- mysql unsigned zerofill_Mysql中Unsigned和Zerofill数据型的使用(细节也很重要啊)
- Linux命令--pwd
- 改变自己,YT,吵架,和好,感冒,烦,新的项目,旧的垃圾,呵呵呵。。
- 正则表达式(基础、常用)----JavaScript
- php常用的数组函数及功能,PHP 常用数组函数 (1)
- linux系统深度清理上网记录,linux日志清理,云主机磁盘清理经验
- 关于Oracle RAC调整网卡MTU值的问题
- 【ES】es 冻结的索引如何查询
- MyBatis集合Spring(二)之SqlSession
- 系统类配置(六) ubuntu16.04命令行安装Nvidia显卡驱动(操作指令详细注释版)
- 边工作边刷题:70天一遍leetcode: day 45-1
- Redis 禁止使用耗时命令和时间复杂度为O(n)的命令
- JAVA程序修改PDF内容_java 修改pdf
- 一键报警(IP对讲)
- linux iozone测试工具,IOZONE测试工具使用方法
- kubernetes-kube-scheduler进程源码分析
- [NLP]——BPE、WordPiece、Unigram and SentencePiece
- html数独游戏源代码,数独算法及源代码
- 关于SQL_Errno1677导致主从复制中断处理
- 星巴克中国首推全新精品咖啡品类“威士忌桶酿咖啡”
热门文章
- Daily Scrum 12/9/2015
- 如何高效的使用Google
- [译]Java 设计模式之组合
- ubuntu硬盘安装及启动,menu.lst
- thinkphp 文件下载实例 实现以及注意事项
- (原创)c++11改进我们的模式之改进单例模式
- Listview+DataPager分页
- 190123每日一句
- 数据保护条例框架与wik解读 第一章 GDPR 个人数据的控制者和处理者必须采取适当的技术和组织措施以实施数据保护原则。在设计和构建处理个人数据的业务流程时,必须考虑到这些原则,并提供保护数据的
- Atitit 常用技能点体系树 os win linux android 前后端 gui h5 vue js jquery bootstrap cocos2d Jafavx wpf