Ftp上传类(FtpClient)
网上找的,学习下
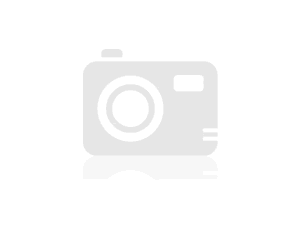
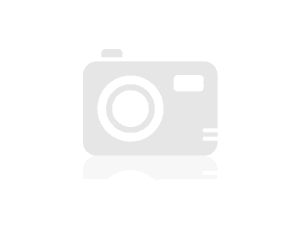
using System;
using System.Net;
using System.IO;
using System.Text;
using System.Net.Sockets;
using System.Diagnostics;
using System.Runtime.Remoting;
using System.Runtime.Remoting.Messaging;
using System.Threading;
/**
* FTP Client library in C#
* Author: Jaimon Mathew
* mailto:jaimonmathew@rediffmail.com
* http://www.csharphelp.com/archives/archive9.html
*
* Addapted for use by Dan Glass 07/03/03
*/
namespace Main08
{
public class FtpClient
{
public class FtpException : Exception
{
public FtpException(string message)
: base(message)
{
}
public FtpException(string message, Exception innerException)
: base(message, innerException)
{
}
}
private static int BUFFER_SIZE = 512;
private static Encoding ASCII = Encoding.Default;
private bool verboseDebugging = false;
// defaults
private string server = "localhost";
private string remotePath = ".";
private string username = "anonymous";
private string password = "anonymous@anonymous.net";
private string message = null;
private string result = null;
private int port = 21;
private int bytes = 0;
private int resultCode = 0;
private bool loggedin = false;
private bool binMode = false;
private Byte[] buffer = new Byte[BUFFER_SIZE];
private Socket clientSocket = null;
private int timeoutSeconds = 10;
/// <summary>
/// Default contructor
/// </summary>
public FtpClient()
{
}
/// <summary>
///
/// </summary>
/// <param name="server"></param>
/// <param name="username"></param>
/// <param name="password"></param>
public FtpClient(string server, string username, string password)
{
this.server = server;
if (username.Length > 0)
this.username = username;
if (password.Length > 0)
this.password = password;
}
/// <summary>
///
/// </summary>
/// <param name="server"></param>
/// <param name="username"></param>
/// <param name="password"></param>
/// <param name="timeoutSeconds"></param>
/// <param name="port"></param>
public FtpClient(string server, string username, string password, int timeoutSeconds, int port)
{
this.server = server;
this.username = username;
this.password = password;
this.timeoutSeconds = timeoutSeconds;
this.port = port;
}
/// <summary>
/// Display all communications to the debug log
/// </summary>
public bool VerboseDebugging
{
get
{
return this.verboseDebugging;
}
set
{
this.verboseDebugging = value;
}
}
/// <summary>
/// Remote server port. Typically TCP 21
/// </summary>
public int Port
{
get
{
return this.port;
}
set
{
this.port = value;
}
}
/// <summary>
/// Timeout waiting for a response from server, in seconds.
/// </summary>
public int Timeout
{
get
{
return this.timeoutSeconds;
}
set
{
this.timeoutSeconds = value;
}
}
/// <summary>
/// Gets and Sets the name of the FTP server.
/// </summary>
/// <returns></returns>
public string Server
{
get
{
return this.server;
}
set
{
this.server = value;
}
}
/// <summary>
/// Gets and Sets the port number.
/// </summary>
/// <returns></returns>
public int RemotePort
{
get
{
return this.port;
}
set
{
this.port = value;
}
}
/// <summary>
/// GetS and Sets the remote directory.
/// </summary>
public string RemotePath
{
get
{
return this.remotePath;
}
set
{
this.remotePath = value;
}
}
/// <summary>
/// Gets and Sets the username.
/// </summary>
public string Username
{
get
{
return this.username;
}
set
{
this.username = value;
}
}
/// <summary>
/// Gets and Set the password.
/// </summary>
public string Password
{
get
{
return this.password;
}
set
{
this.password = value;
}
}
/// <summary>
/// If the value of mode is true, set binary mode for downloads, else, Ascii mode.
/// </summary>
public bool BinaryMode
{
get
{
return this.binMode;
}
set
{
if (this.binMode == value)
return;
if (value)
sendCommand("TYPE I");
else
sendCommand("TYPE A");
if (this.resultCode != 200)
throw new FtpException(result.Substring(4));
}
}
/// <summary>
/// Login to the remote server.
/// </summary>
public void Login()
{
if (this.loggedin)
this.Close();
Debug.WriteLine("Opening connection to " + this.server, "FtpClient");
IPAddress addr = null;
IPEndPoint ep = null;
try
{
this.clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
addr = Dns.GetHostEntry(this.server).AddressList[0];
ep = new IPEndPoint(addr, this.port);
this.clientSocket.Connect(ep);
}
catch (Exception ex)
{
// doubtfull
if (this.clientSocket != null && this.clientSocket.Connected)
this.clientSocket.Close();
throw new FtpException("Couldn't connect to remote server", ex);
}
this.readResponse();
if (this.resultCode != 220)
{
this.Close();
throw new FtpException(this.result.Substring(4));
}
this.sendCommand("USER " + username);
if (!(this.resultCode == 331 || this.resultCode == 230))
{
this.cleanup();
throw new FtpException(this.result.Substring(4));
}
if (this.resultCode != 230)
{
this.sendCommand("PASS " + password);
if (!(this.resultCode == 230 || this.resultCode == 202))
{
this.cleanup();
throw new FtpException(this.result.Substring(0, 3));//
}
}
this.loggedin = true;
Debug.WriteLine("Connected to " + this.server, "FtpClient");
this.ChangeDir(this.remotePath);
}
/// <summary>
/// Close the FTP connection.
/// </summary>
public void Close()
{
Debug.WriteLine("Closing connection to " + this.server, "FtpClient");
if (this.clientSocket != null)
{
this.sendCommand("QUIT");
}
this.cleanup();
}
/// <summary>
/// Return a string array containing the remote directory's file list.
/// </summary>
/// <returns></returns>
public string[] GetFileList()
{
return this.GetFileList("*.*");
}
public bool DirectoryExist(string RomtePathName)
{
try
{
ChangeDir(RomtePathName);
return true;
}
catch
{
MakeDir(RomtePathName);
ChangeDir(RomtePathName);
return true;
}
}
private string[] ListDirectories(string[] msg)
{
string s = "";
foreach (string f in msg)
{
if (f.Length > 0)
{
if (f.ToUpper().Trim()[0] == 'D' && f.ToUpper().IndexOf("<DIR>") < 0)
{
s += f.Trim() + "\n";
}
}
}
return s.Replace("\r", "").Split('\n');
}
public string[] GetDirectory()
{
if (!this.loggedin)
this.Login();
Socket cSocket = createDataSocket();
sendCommand("LIST");
if (this.resultCode == 226)
this.readLine();
if (!(this.resultCode == 150 || this.resultCode == 125))
throw new FtpException(this.result.Substring(4));
this.readLine();
this.message = "";
DateTime timeout = DateTime.Now.AddSeconds(this.timeoutSeconds);
while (timeout > DateTime.Now)
{
int bytes = cSocket.Receive(buffer, buffer.Length, 0);
this.message += ASCII.GetString(buffer, 0, bytes);
if (bytes < this.buffer.Length)
break;
}
string[] msg = this.message.Replace("\r", "").Split('\n');
cSocket.Close();
return ListDirectories(msg);
}
/*/// <summary>
/// Return a string array containing the remote directory's file list.
/// </summary>
/// <param name="mask"></param>
/// <returns></returns>
public string[] GetFileList(string mask)
{
if ( !this.loggedin ) this.Login();
Socket cSocket = createDataSocket();
this.sendCommand("NLST " + mask);
if(!(this.resultCode == 150 || this.resultCode == 125)) throw new FtpException(this.result.Substring(4));
this.message = "";
DateTime timeout = DateTime.Now.AddSeconds(this.timeoutSeconds);
while( timeout > DateTime.Now )
{
int bytes = cSocket.Receive(buffer, buffer.Length, 0);
this.message += ASCII.GetString(buffer, 0, bytes);
if ( bytes < this.buffer.Length ) break;
}
string[] msg = this.message.Replace("\r","").Split('\n');
cSocket.Close();
if ( this.message.IndexOf( "No such file or directory" ) != -1 )
msg = new string[]{};
this.readResponse();
if ( this.resultCode != 226 )
msg = new string[]{};
// throw new FtpException(result.Substring(4));
return msg;
}
*/
/// <summary>
/// Return a string array containing the remote directory's file list.
/// </summary>
/// <param name="mask"></param>
/// <returns></returns>
public string[] GetFileList(string mask)
{
if (!this.loggedin)
this.Login();
Socket cSocket = createDataSocket();
this.sendCommand("List " + mask);
if (!(this.resultCode == 150 || this.resultCode == 125))
throw new FtpException(this.result.Substring(4));
this.message = "";
DateTime timeout = DateTime.Now.AddSeconds(this.timeoutSeconds);
while (timeout > DateTime.Now)
{
int bytes = cSocket.Receive(buffer, buffer.Length, 0);
this.message += ASCII.GetString(buffer, 0, bytes);
if (bytes < this.buffer.Length)
break;
}
string[] msg = this.message.Replace("\r", "").Split('\n');
cSocket.Close();
if (this.message.IndexOf("No such file or directory") != -1 || this.message == "")
{
msg = new string[] { };
}
//else
// this.sendCommand("List ..");
this.readResponse();
if (this.resultCode != 226)
msg = new string[] { };
// throw new FtpException(result.Substring(4));
return ListFiles(msg);
}
private string[] ListFiles(string[] msg)
{
string s = "";
foreach (string f in msg)
{
if (f.Length > 0)
{
if (f.Trim()[0] != 'd' && f.ToUpper().IndexOf("<DIR>") < 0)
{
s += f + "\n";
}
}
}
return s.Replace("\r", "").Split('\n');
}
/// <summary>
/// Return the size of a file.
/// </summary>
/// <param name="fileName"></param>
/// <returns></returns>
public long GetFileSize(string fileName)
{
if (!this.loggedin)
this.Login();
this.sendCommand("SIZE " + fileName);
long size = 0;
if (this.resultCode == 213)
size = long.Parse(this.result.Substring(4));
else
throw new FtpException(this.result.Substring(4));
return size;
}
/// <summary>
/// Download a file to the Assembly's local directory,
/// keeping the same file name.
/// </summary>
/// <param name="remFileName"></param>
public void Download(string remFileName)
{
this.Download(remFileName, "", false);
}
/// <summary>
/// Download a remote file to the Assembly's local directory,
/// keeping the same file name, and set the resume flag.
/// </summary>
/// <param name="remFileName"></param>
/// <param name="resume"></param>
public void Download(string remFileName, Boolean resume)
{
this.Download(remFileName, "", resume);
}
/// <summary>
/// Download a remote file to a local file name which can include
/// a path. The local file name will be created or overwritten,
/// but the path must exist.
/// </summary>
/// <param name="remFileName"></param>
/// <param name="locFileName"></param>
public void Download(string remFileName, string locFileName)
{
this.Download(remFileName, locFileName, false);
}
/// <summary>
/// Download a remote file to a local file name which can include
/// a path, and set the resume flag. The local file name will be
/// created or overwritten, but the path must exist.
/// </summary>
/// <param name="remFileName"></param>
/// <param name="locFileName"></param>
/// <param name="resume"></param>
public void Download(string remFileName, string locFileName, Boolean resume)
{
if (!this.loggedin)
this.Login();
this.BinaryMode = true;
Debug.WriteLine("Downloading file " + remFileName + " from " + server + "/" + remotePath, "FtpClient");
if (locFileName.Equals(""))
{
locFileName = remFileName;
}
FileStream output = null;
if (!File.Exists(locFileName))
output = File.Create(locFileName);
else
output = new FileStream(locFileName, FileMode.Open);
Socket cSocket = createDataSocket();
long offset = 0;
if (resume)
{
offset = output.Length;
if (offset > 0)
{
this.sendCommand("REST " + offset);
if (this.resultCode != 350)
{
//Server dosnt support resuming
offset = 0;
Debug.WriteLine("Resuming not supported:" + result.Substring(4), "FtpClient");
}
else
{
Debug.WriteLine("Resuming at offset " + offset, "FtpClient");
output.Seek(offset, SeekOrigin.Begin);
}
}
}
this.sendCommand("RETR " + remFileName);
if (this.resultCode != 150 && this.resultCode != 125)
{
throw new FtpException(this.result.Substring(4));
}
DateTime timeout = DateTime.Now.AddSeconds(this.timeoutSeconds);
while (timeout > DateTime.Now)
{
this.bytes = cSocket.Receive(buffer, buffer.Length, 0);
output.Write(this.buffer, 0, this.bytes);
if (this.bytes <= 0)
{
break;
}
}
output.Close();
if (cSocket.Connected)
cSocket.Close();
this.readResponse();
if (this.resultCode != 226 && this.resultCode != 250)
throw new FtpException(this.result.Substring(4));
}
/// <summary>
/// Upload a file.
/// </summary>
/// <param name="fileName"></param>
public void Upload(string fileName)
{
this.Upload(fileName, false);
}
/// <summary>
/// Upload a file and set the resume flag.
/// </summary>
/// <param name="fileName"></param>
/// <param name="resume"></param>
public void Upload(string fileName, bool resume)
{
if (!this.loggedin)
this.Login();
Socket cSocket = null;
long offset = 0;
if (resume)
{
try
{
this.BinaryMode = true;
offset = GetFileSize(Path.GetFileName(fileName));
}
catch (Exception ex)
{
Debug.WriteLine(ex.Message);
// file not exist
offset = 0;
}
}
// open stream to read file
FileStream input = new FileStream(fileName, FileMode.Open);
if (resume && input.Length < offset)
{
// different file size
Debug.WriteLine("Overwriting " + fileName, "FtpClient");
offset = 0;
}
else if (resume && input.Length == offset)
{
// file done
input.Close();
Debug.WriteLine("Skipping completed " + fileName + " - turn resume off to not detect.", "FtpClient");
return;
}
// dont create untill we know that we need it
cSocket = this.createDataSocket();
if (offset > 0)
{
this.sendCommand("REST " + offset);
if (this.resultCode != 350)
{
Debug.WriteLine("Resuming not supported", "FtpClient");
offset = 0;
}
}
this.sendCommand("STOR " + Path.GetFileName(fileName));
if (this.resultCode != 125 && this.resultCode != 150)
throw new FtpException(result.Substring(4));
if (offset != 0)
{
Debug.WriteLine("Resuming at offset " + offset, "FtpClient");
input.Seek(offset, SeekOrigin.Begin);
}
Debug.WriteLine("Uploading file " + fileName + " to " + remotePath, "FtpClient");
while ((bytes = input.Read(buffer, 0, buffer.Length)) > 0)
{
cSocket.Send(buffer, bytes, 0);
}
input.Close();
if (cSocket.Connected)
{
cSocket.Close();
}
this.readResponse();
if (this.resultCode != 226 && this.resultCode != 250)
throw new FtpException(this.result.Substring(4));
}
/// <summary>
/// Upload a directory and its file contents
/// </summary>
/// <param name="path"></param>
/// <param name="recurse">Whether to recurse sub directories</param>
public void UploadDirectory(string path, bool recurse, string despath)
{
this.UploadDirectory(path, recurse, "*.*", despath);
}
/// <summary>
/// Upload a directory and its file contents
/// </summary>
/// <param name="path"></param>
/// <param name="recurse">Whether to recurse sub directories</param>
/// <param name="mask">Only upload files of the given mask - everything is '*.*'</param>
public void UploadDirectory(string path, bool recurse, string mask, string despath)
{
if (despath != string.Empty)
{
string[] dp = despath.Replace("/", @"\").Split('\\');
foreach (string s in dp)
{
if (s != "")
{
this.MakeDir(s);
this.ChangeDir(s);
}
}
}
string[] dirs = path.Replace("/", @"\").Split('\\');
string rootDir = dirs[dirs.Length - 1];
// make the root dir if it doed not exist
//if ( this.GetFileList(rootDir).Length < 1 )
this.MakeDir(rootDir);
//this.MakeDir(despath);
this.ChangeDir(rootDir);
foreach (string file in Directory.GetFiles(path, mask))
{
//this.Upload(file, true);
this.Upload(file, false);
}
if (recurse)
{
foreach (string directory in Directory.GetDirectories(path))
{
this.UploadDirectory(directory, recurse, mask, "");
}
}
this.ChangeDir("..");
}
/// <summary>
/// Delete a file from the remote FTP server.
/// </summary>
/// <param name="fileName"></param>
public void DeleteFile(string fileName)
{
if (!this.loggedin)
this.Login();
this.sendCommand("DELE " + fileName);
if (this.resultCode != 250)
throw new FtpException(this.result.Substring(4));
Debug.WriteLine("Deleted file " + fileName, "FtpClient");
}
/// <summary>
/// Rename a file on the remote FTP server.
/// </summary>
/// <param name="oldFileName"></param>
/// <param name="newFileName"></param>
/// <param name="overwrite">setting to false will throw exception if it exists</param>
public void RenameFile(string oldFileName, string newFileName, bool overwrite)
{
if (!this.loggedin)
this.Login();
this.sendCommand("RNFR " + oldFileName);
if (this.resultCode != 350)
throw new FtpException(this.result.Substring(4));
if (!overwrite && this.GetFileList(newFileName).Length > 0)
throw new FtpException("File already exists");
this.sendCommand("RNTO " + newFileName);
if (this.resultCode != 250)
throw new FtpException(this.result.Substring(4));
Debug.WriteLine("Renamed file " + oldFileName + " to " + newFileName, "FtpClient");
}
/// <summary>
/// Create a directory on the remote FTP server.
/// </summary>
/// <param name="dirName"></param>
public void MakeDir(string dirName)
{
if (!this.loggedin)
this.Login();
this.sendCommand("MKD " + dirName);
string err = result;
if (result.IndexOf("Failed to create") > 0)
{
//文件已经存在
}
if (result.IndexOf("Permission denied") > 0)
{
//没有权限
}
//if ( this.resultCode != 250 && this.resultCode != 257 ) throw new FtpException(this.result.Substring(4));
//Debug.WriteLine( "Created directory " + dirName, "FtpClient" );
}
/// <summary>
/// Delete a directory on the remote FTP server.
/// </summary>
/// <param name="dirName"></param>
public void RemoveDir(string dirName)
{
if (!this.loggedin)
this.Login();
this.sendCommand("RMD " + dirName);
if (this.resultCode != 250)
throw new FtpException(this.result.Substring(4));
Debug.WriteLine("Removed directory " + dirName, "FtpClient");
}
/// <summary>
/// Change the current working directory on the remote FTP server.
/// </summary>
/// <param name="dirName"></param>
public void ChangeDir(string dirName)
{
if (dirName == null || dirName.Equals(".") || dirName.Length == 0)
{
return;
}
if (!this.loggedin)
this.Login();
this.sendCommand("CWD " + dirName);
//Thread.Sleep(7000);
if (this.resultCode == 226)
this.readResponse();
if (this.resultCode != 250)
throw new FtpException(result.Substring(4));
this.sendCommand("PWD");
if (this.resultCode != 257)
throw new FtpException(result.Substring(4));
// gonna have to do better than this.
this.remotePath = this.message.Split('"')[1];
Debug.WriteLine("Current directory is " + this.remotePath, "FtpClient");
}
/// <summary>
///
/// </summary>
private void readResponse()
{
this.message = "";
this.result = this.readLine();
//string rs = this.readLine();
//if (rs != string.Empty && rs.Length > 0)
// result = rs;
if (this.result.Length > 3)
this.resultCode = int.Parse(this.result.Substring(0, 3));
else
this.result = null;
}
/// <summary>
///
/// </summary>
/// <returns></returns>
private string readLine()
{
while (true)
{
this.bytes = clientSocket.Receive(this.buffer, this.buffer.Length, 0);
this.message += ASCII.GetString(this.buffer, 0, this.bytes);
if (this.bytes < this.buffer.Length)
{
break;
}
}
string[] msg = this.message.Split('\n');
if (this.message.Length > 2)
this.message = msg[msg.Length - 2];
else
this.message = msg[0];
if (this.message.Length > 4 && !this.message.Substring(3, 1).Equals(" "))
return this.readLine();
if (this.verboseDebugging)
{
for (int i = 0; i < msg.Length - 1; i++)
{
Debug.Write(msg[i], "FtpClient");
}
}
return message;
}
/// <summary>
///
/// </summary>
/// <param name="command"></param>
private void sendCommand(String command)
{
if (this.verboseDebugging)
Debug.WriteLine(command, "FtpClient");
Byte[] cmdBytes = Encoding.Default.GetBytes((command + "\r\n").ToCharArray());
clientSocket.Send(cmdBytes, cmdBytes.Length, 0);
this.readResponse();
}
/// <summary>
/// when doing data transfers, we need to open another socket for it.
/// </summary>
/// <returns>Connected socket</returns>
private Socket createDataSocket()
{
this.sendCommand("PASV");
if (this.resultCode == 226)
this.readLine();
if (this.resultCode != 227)
throw new FtpException(this.result.Substring(4));
int index1 = this.result.IndexOf('(');
int index2 = this.result.IndexOf(')');
string ipData = this.result.Substring(index1 + 1, index2 - index1 - 1);
int[] parts = new int[6];
int len = ipData.Length;
int partCount = 0;
string buf = "";
for (int i = 0; i < len && partCount <= 6; i++)
{
char ch = char.Parse(ipData.Substring(i, 1));
if (char.IsDigit(ch))
buf += ch;
else if (ch != ',')
throw new FtpException("Malformed PASV result: " + result);
if (ch == ',' || i + 1 == len)
{
try
{
parts[partCount++] = int.Parse(buf);
buf = "";
}
catch (Exception ex)
{
throw new FtpException("Malformed PASV result (not supported?): " + this.result, ex);
}
}
}
string ipAddress = parts[0] + "." + parts[1] + "." + parts[2] + "." + parts[3];
int port = (parts[4] << 8) + parts[5];
Socket socket = null;
IPEndPoint ep = null;
try
{
socket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
ep = new IPEndPoint(Dns.GetHostEntry(this.server).AddressList[0], port);
socket.Connect(ep);
}
catch (Exception ex)
{
// doubtfull.
if (socket != null && socket.Connected)
socket.Close();
throw new FtpException("Can't connect to remote server", ex);
}
return socket;
}
/// <summary>
/// Always release those sockets.
/// </summary>
private void cleanup()
{
if (this.clientSocket != null)
{
this.clientSocket.Close();
this.clientSocket = null;
}
this.loggedin = false;
}
/// <summary>
/// Destuctor
/// </summary>
~FtpClient()
{
this.cleanup();
}
/**************************************************************************************************************/
#region Async methods (auto generated)
/*
WinInetApi.FtpClient ftp = new WinInetApi.FtpClient();
MethodInfo[] methods = ftp.GetType().GetMethods(BindingFlags.DeclaredOnly|BindingFlags.Instance|BindingFlags.Public);
foreach ( MethodInfo method in methods )
{
string param = "";
string values = "";
foreach ( ParameterInfo i in method.GetParameters() )
{
param += i.ParameterType.Name + " " + i.Name + ",";
values += i.Name + ",";
}
Debug.WriteLine("private delegate " + method.ReturnType.Name + " " + method.Name + "Callback(" + param.TrimEnd(',') + ");");
Debug.WriteLine("public System.IAsyncResult Begin" + method.Name + "( " + param + " System.AsyncCallback callback )");
Debug.WriteLine("{");
Debug.WriteLine("" + method.Name + "Callback ftpCallback = new " + method.Name + "Callback(" + values + " this." + method.Name + ");");
Debug.WriteLine("return ftpCallback.BeginInvoke(callback, null);");
Debug.WriteLine("}");
Debug.WriteLine("public void End" + method.Name + "(System.IAsyncResult asyncResult)");
Debug.WriteLine("{");
Debug.WriteLine(method.Name + "Callback fc = (" + method.Name + "Callback) ((AsyncResult)asyncResult).AsyncDelegate;");
Debug.WriteLine("fc.EndInvoke(asyncResult);");
Debug.WriteLine("}");
//Debug.WriteLine(method);
}
*/
private delegate void LoginCallback();
public System.IAsyncResult BeginLogin(System.AsyncCallback callback)
{
LoginCallback ftpCallback = new LoginCallback(this.Login);
return ftpCallback.BeginInvoke(callback, null);
}
private delegate void CloseCallback();
public System.IAsyncResult BeginClose(System.AsyncCallback callback)
{
CloseCallback ftpCallback = new CloseCallback(this.Close);
return ftpCallback.BeginInvoke(callback, null);
}
private delegate String[] GetFileListCallback();
public System.IAsyncResult BeginGetFileList(System.AsyncCallback callback)
{
GetFileListCallback ftpCallback = new GetFileListCallback(this.GetFileList);
return ftpCallback.BeginInvoke(callback, null);
}
private delegate String[] GetFileListMaskCallback(String mask);
public System.IAsyncResult BeginGetFileList(String mask, System.AsyncCallback callback)
{
GetFileListMaskCallback ftpCallback = new GetFileListMaskCallback(this.GetFileList);
return ftpCallback.BeginInvoke(mask, callback, null);
}
private delegate Int64 GetFileSizeCallback(String fileName);
public System.IAsyncResult BeginGetFileSize(String fileName, System.AsyncCallback callback)
{
GetFileSizeCallback ftpCallback = new GetFileSizeCallback(this.GetFileSize);
return ftpCallback.BeginInvoke(fileName, callback, null);
}
private delegate void DownloadCallback(String remFileName);
public System.IAsyncResult BeginDownload(String remFileName, System.AsyncCallback callback)
{
DownloadCallback ftpCallback = new DownloadCallback(this.Download);
return ftpCallback.BeginInvoke(remFileName, callback, null);
}
private delegate void DownloadFileNameResumeCallback(String remFileName, Boolean resume);
public System.IAsyncResult BeginDownload(String remFileName, Boolean resume, System.AsyncCallback callback)
{
DownloadFileNameResumeCallback ftpCallback = new DownloadFileNameResumeCallback(this.Download);
return ftpCallback.BeginInvoke(remFileName, resume, callback, null);
}
private delegate void DownloadFileNameFileNameCallback(String remFileName, String locFileName);
public System.IAsyncResult BeginDownload(String remFileName, String locFileName, System.AsyncCallback callback)
{
DownloadFileNameFileNameCallback ftpCallback = new DownloadFileNameFileNameCallback(this.Download);
return ftpCallback.BeginInvoke(remFileName, locFileName, callback, null);
}
private delegate void DownloadFileNameFileNameResumeCallback(String remFileName, String locFileName, Boolean resume);
public System.IAsyncResult BeginDownload(String remFileName, String locFileName, Boolean resume, System.AsyncCallback callback)
{
DownloadFileNameFileNameResumeCallback ftpCallback = new DownloadFileNameFileNameResumeCallback(this.Download);
return ftpCallback.BeginInvoke(remFileName, locFileName, resume, callback, null);
}
private delegate void UploadCallback(String fileName);
public System.IAsyncResult BeginUpload(String fileName, System.AsyncCallback callback)
{
UploadCallback ftpCallback = new UploadCallback(this.Upload);
return ftpCallback.BeginInvoke(fileName, callback, null);
}
private delegate void UploadFileNameResumeCallback(String fileName, Boolean resume);
public System.IAsyncResult BeginUpload(String fileName, Boolean resume, System.AsyncCallback callback)
{
UploadFileNameResumeCallback ftpCallback = new UploadFileNameResumeCallback(this.Upload);
return ftpCallback.BeginInvoke(fileName, resume, callback, null);
}
private delegate void UploadDirectoryCallback(String path, Boolean recurse, String despath);
public System.IAsyncResult BeginUploadDirectory(String path, Boolean recurse, String despath, System.AsyncCallback callback)
{
UploadDirectoryCallback ftpCallback = new UploadDirectoryCallback(this.UploadDirectory);
return ftpCallback.BeginInvoke(path, recurse, despath, callback, null);
}
private delegate void UploadDirectoryPathRecurseMaskCallback(String path, Boolean recurse, String mask, String despath);
public System.IAsyncResult BeginUploadDirectory(String path, Boolean recurse, String mask, String despath, System.AsyncCallback callback)
{
UploadDirectoryPathRecurseMaskCallback ftpCallback = new UploadDirectoryPathRecurseMaskCallback(this.UploadDirectory);
return ftpCallback.BeginInvoke(path, recurse, mask, despath, callback, null);
}
private delegate void DeleteFileCallback(String fileName);
public System.IAsyncResult BeginDeleteFile(String fileName, System.AsyncCallback callback)
{
DeleteFileCallback ftpCallback = new DeleteFileCallback(this.DeleteFile);
return ftpCallback.BeginInvoke(fileName, callback, null);
}
private delegate void RenameFileCallback(String oldFileName, String newFileName, Boolean overwrite);
public System.IAsyncResult BeginRenameFile(String oldFileName, String newFileName, Boolean overwrite, System.AsyncCallback callback)
{
RenameFileCallback ftpCallback = new RenameFileCallback(this.RenameFile);
return ftpCallback.BeginInvoke(oldFileName, newFileName, overwrite, callback, null);
}
private delegate void MakeDirCallback(String dirName);
public System.IAsyncResult BeginMakeDir(String dirName, System.AsyncCallback callback)
{
MakeDirCallback ftpCallback = new MakeDirCallback(this.MakeDir);
return ftpCallback.BeginInvoke(dirName, callback, null);
}
private delegate void RemoveDirCallback(String dirName);
public System.IAsyncResult BeginRemoveDir(String dirName, System.AsyncCallback callback)
{
RemoveDirCallback ftpCallback = new RemoveDirCallback(this.RemoveDir);
return ftpCallback.BeginInvoke(dirName, callback, null);
}
private delegate void ChangeDirCallback(String dirName);
public System.IAsyncResult BeginChangeDir(String dirName, System.AsyncCallback callback)
{
ChangeDirCallback ftpCallback = new ChangeDirCallback(this.ChangeDir);
return ftpCallback.BeginInvoke(dirName, callback, null);
}
#endregion
}
}
转载于:https://www.cnblogs.com/huaibaobao/archive/2009/09/04/1560379.html
Ftp上传类(FtpClient)相关推荐
- C#写的ftp上传类
这个类是我之前在一个什么地方看到的不记得了,最近要用着大文件上传,翻箱倒柜的找出来. view sourceprint? using System; using System.Collections. ...
- php 如何做ftp传输,php如何实现ftp上传
php实现ftp上传的方法:首先通过"ftp_connect"函数连接FTP服务器 :然后使用username和password登录:最后通过"ftp_put()&quo ...
- 高可用的Spring FTP上传下载工具类(已解决上传过程常见问题)
点击上方"方志朋",选择"设为星标" 回复"666"获取新整理的面试文章 作者:宇的季节 cnblogs.com/chenkeyu/p/80 ...
- ftp上传-下载文件通用工具类,已实测
话不多说直接上代码 package com.springboot.demo.utils;import lombok.extern.slf4j.Slf4j; import org.apache.comm ...
- php vsftpd文件上传类,php ftp文件上传函数(基础版)
php ftp文件上传函数(基础版) 复制代码 代码如下: // 定义变量 $local_file = 'local.zip'; $server_file = 'server.zip'; // 连接F ...
- applet实现大文件ftp上传(一)
由于要用APPLET实现大文件FTP上传下载,从网上搜索了几下,找到很多资料,最后决定采用基于 org.apache.commons.net.ftp包实现FTP上传下载,Net包中的类既提供对协议的底 ...
- Commons net实现 FTP上传下载
最近项目中需要到Ftp文件上传,选择了Commons net.Commons net包中的ftp工具类能够帮助我们轻松实现Ftp方式的文件上传/下载.其中最重要的一个类就是FTPClient类,这个提 ...
- asp.net ftp上传文件到服务器,.net 文件上传到服务器上
详解 Linux 下 SSH 远程文件传输命令 scp 3.将本地文件上传到服务器上 scp-P 2222/home/lnmp0.4.tar.gz root@www.vpser.net:/root/l ...
- java ftp 上传文件 无效_java实现FTP文件上传出现的问题
昨天用JAVA写了一个实现FTP文件上传功能的类(是通过sun.net.ftp包实现的,此包为SUN的私有类包,所以官方没有提供相关API文档),然后进行了简单的测试. 具体类代码如下(此类的部分方法 ...
最新文章
- mysql创建库几种方法_MySQL创建数据库的两种方法
- Samba 系列(九):将 CentOS 7 桌面系统加入到 Samba4 AD 域环境中
- java聊天程序_急需一个用java 语言写的聊天程序
- 苹果“自研”心不死 仍考虑收购英特尔基带业务
- 【PHP学习】—PHP的基本数据类型(二)
- Google Plugin for Eclipse离线下载及安装
- 模板 - 数论 - 整除分块
- php 腾讯短信接口api,腾讯云短信发送功能API-PHP接入
- 计算机的硬盘如何查看,怎样查看电脑硬盘信息 电脑中的硬盘信息
- 使用脚本更改计算机名
- POJ 1375 Intervals
- Windows内存 之 任务管理器
- c语言零错误零警告,C语言 g警告:无符号表达式的比较0始终为false
- 迷你马拉松的微博营销
- Android——Hander+Service,实现后台长期周期性定时任务
- 大牛耗时一年最佳总结,让你的app体验更丝滑!震撼来袭免费下载!
- C++ Vecor 清空内存
- Appstore商店排名前十的威客应用!
- 参加51CTo培训,红帽RHCE认证考试通过啦
- 服装服饰行业SCRM-VIP会员营销解决方案