学用NHibernate(一)
使用Nunit测试一个使用NHibernate的项目,被测试的项目为Job.Personal.Core,测试项目为Job.Personal.Tests。
配置:
1、在主项目中添加对NHibernate.dll的引用,这样同时会关联到Castle.DynamicProxy.dll HashCodeProvider.dll Iesi.Collections.dll 如果需要记录异常日志的则再引用log4net.dll
2、在测试项目中添加对NHibernate.dll的引用。
3、因为测试项目是使用Nunit进行测试,同时这是一个组件项目,所以配置文件要存放在bin/Debug中,命名为:Job.Personal.Tests.dll.config
4、为定位hbm.xml文件,把对该xml文件的生成操作修改为嵌入的资源。
5、编辑Job.Personal.Tests.dll.config配置文件。
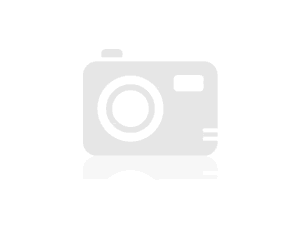
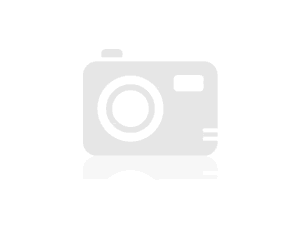
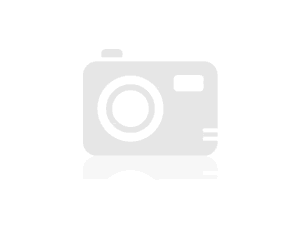
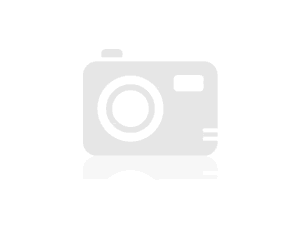
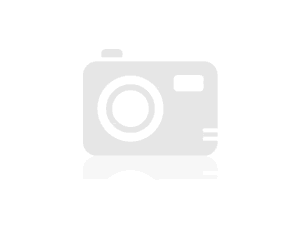
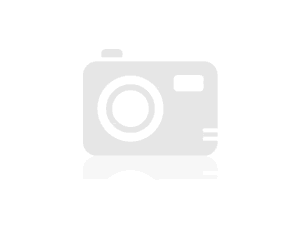
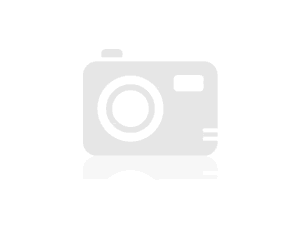
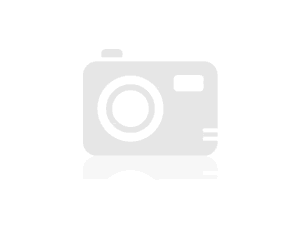
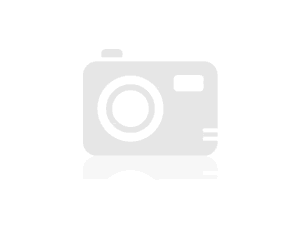
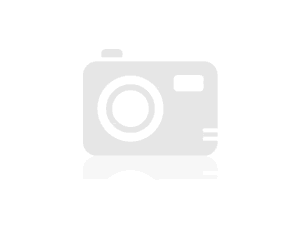
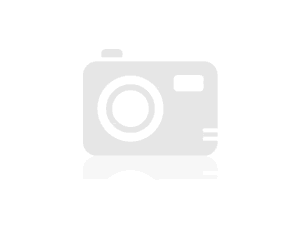
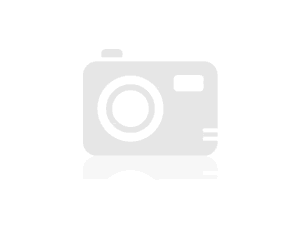
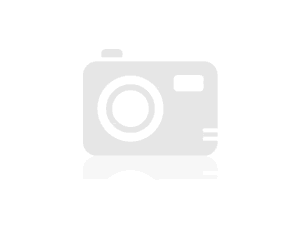
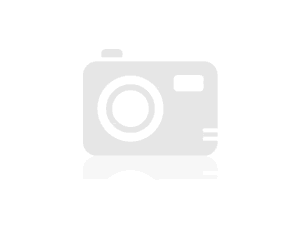
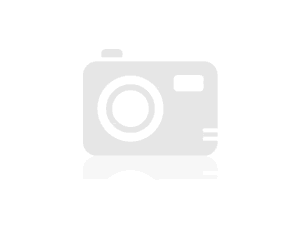
6、编辑MyJob.hbm.xml
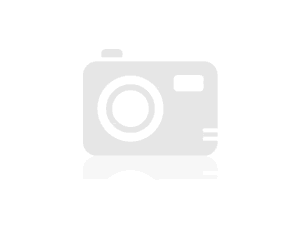
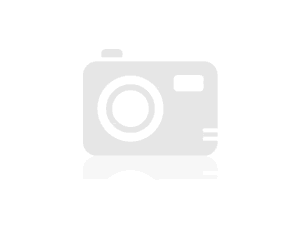
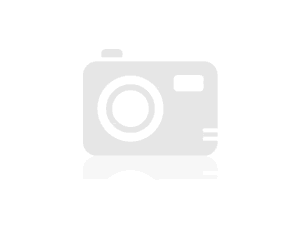
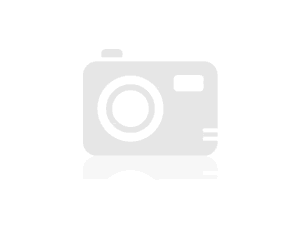
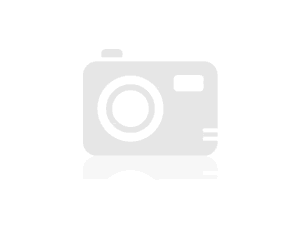
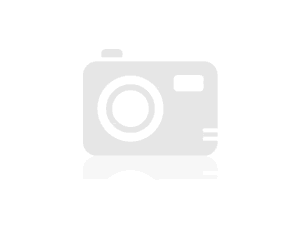
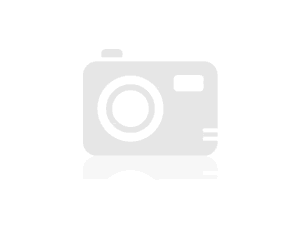
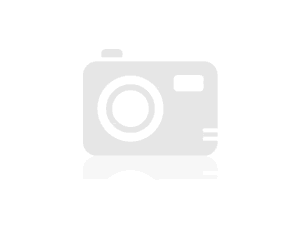
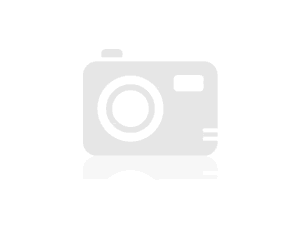
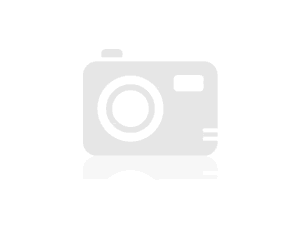
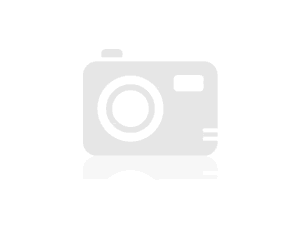
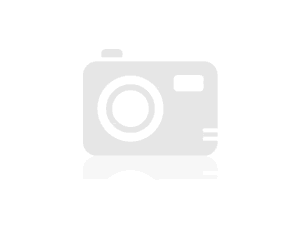
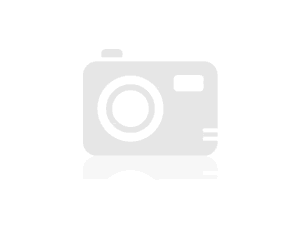
注意:主键的类名必须为ID,如果主键是由数据库自动生成的,则使用<generator class="native" />声明,而不是用<generator class="assigned" />
7、如果使用编辑hbm.xml时使用智能感知,则拷入nhibernate-configuration-2.0.xsd nhibernate-generic.xsd nhibernate-mapping-2.0.xsd文件到hbm.xml所在目录。
8、对于由hbm.xml生成业务对象的操作,则能通过NHibernateContrib,这个在以后的随笔中讲解。现在我们就有了一个对应于hbm.xml的业务对象了。
9、为操作的封装性,这里我提取了Cuyahoga项目对Session的操作,如下:
SessionFactory.cs
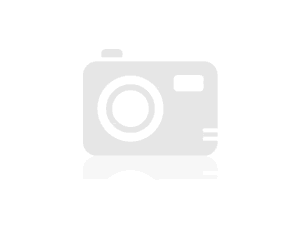
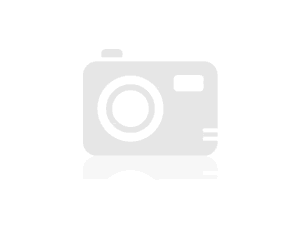
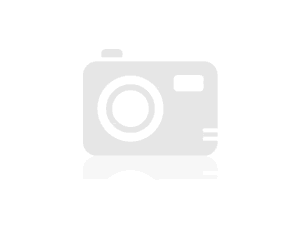
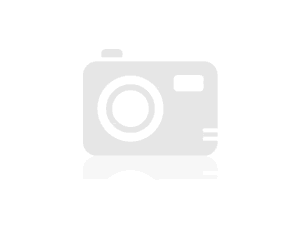
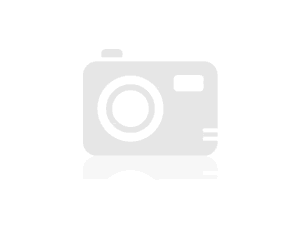
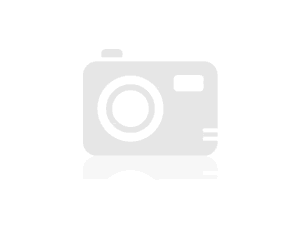
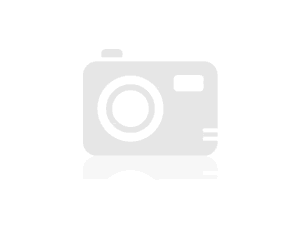
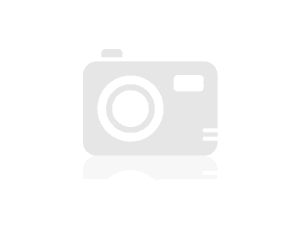
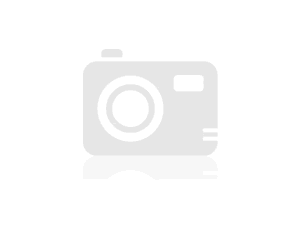


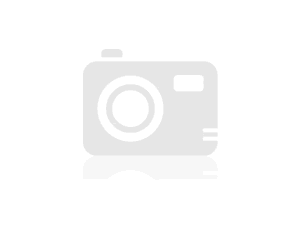




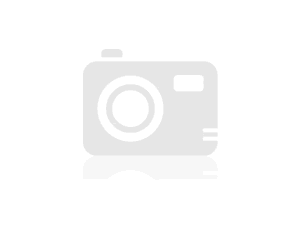








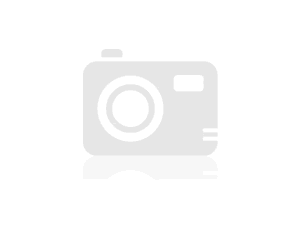






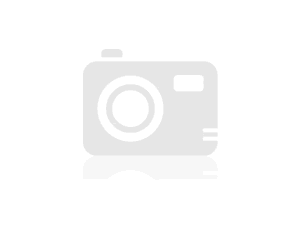





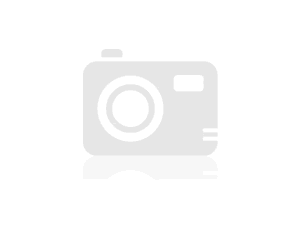




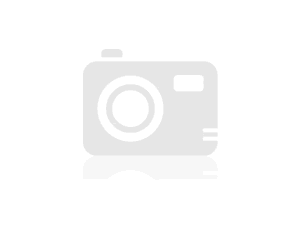





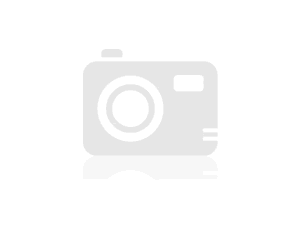




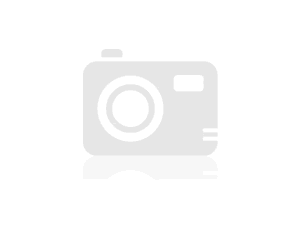





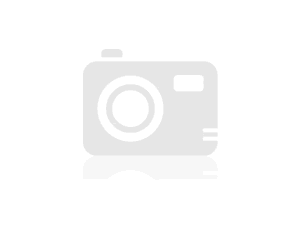




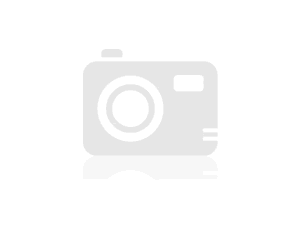



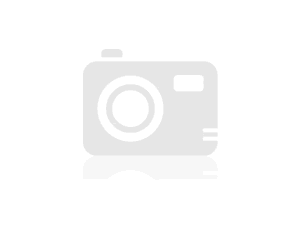








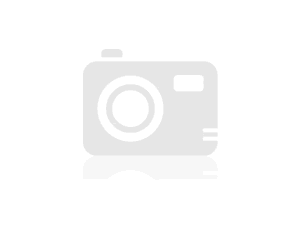




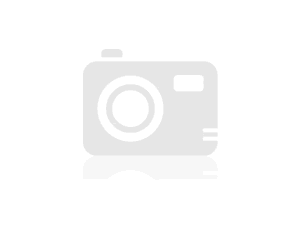




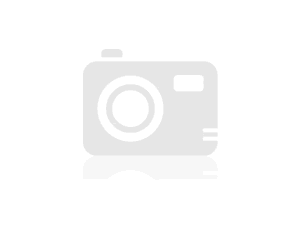







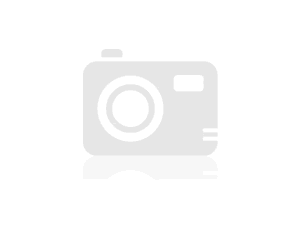







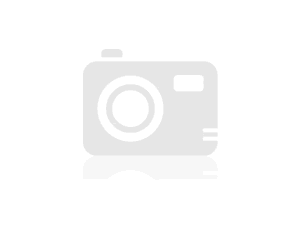






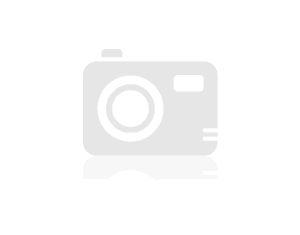
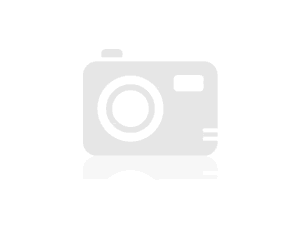
CoreRepository.cs
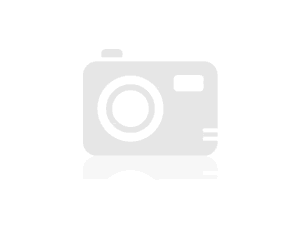
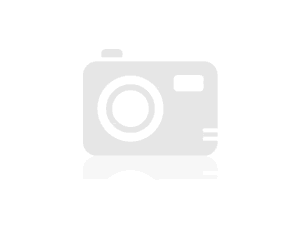
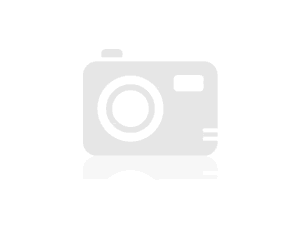
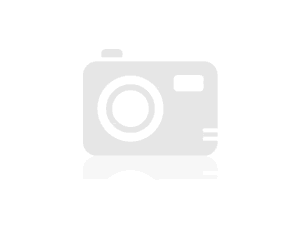
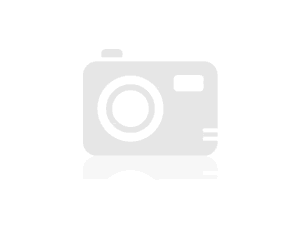
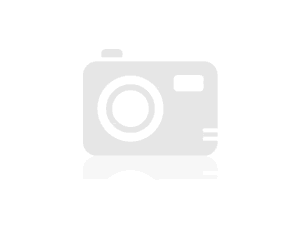
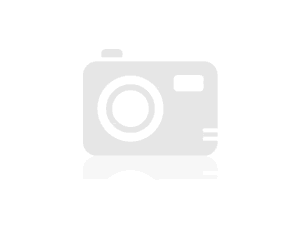
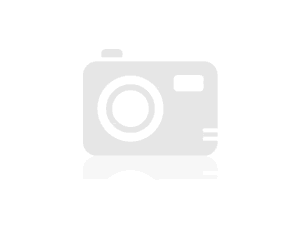
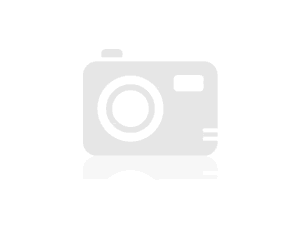
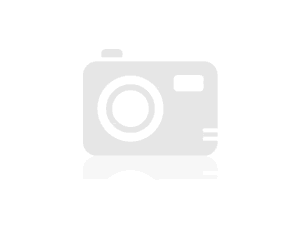
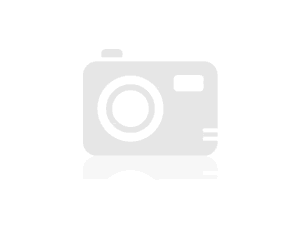
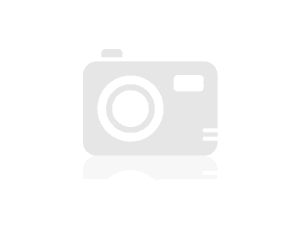
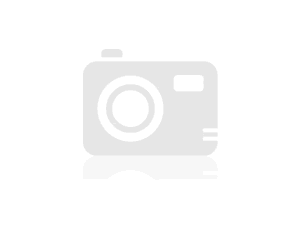


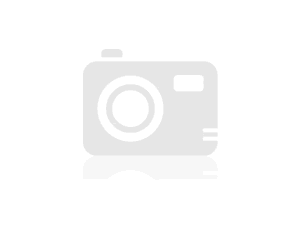




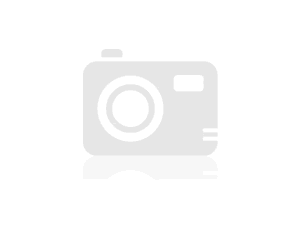







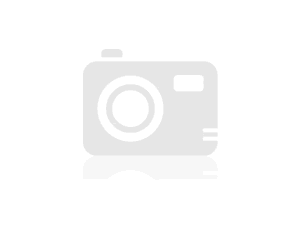




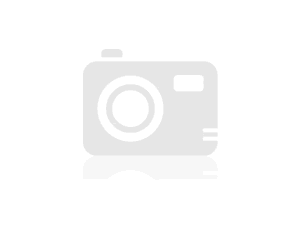


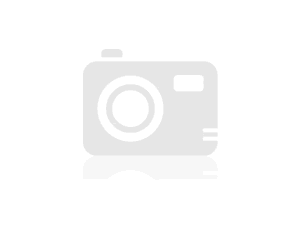





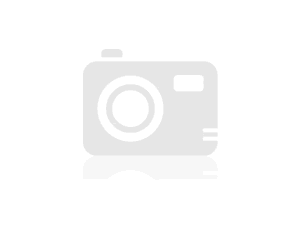




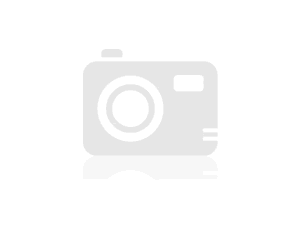





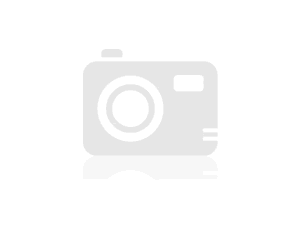




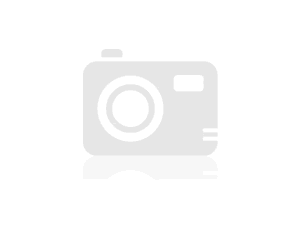






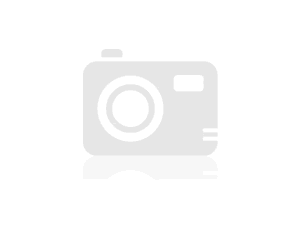




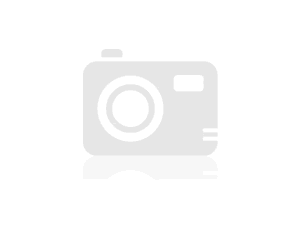



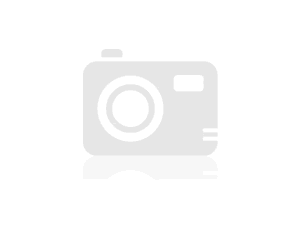





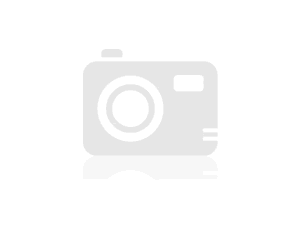






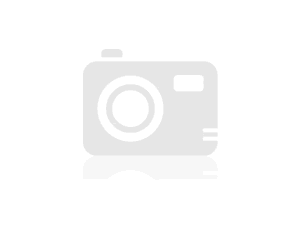




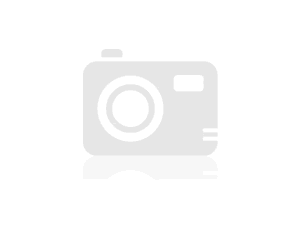



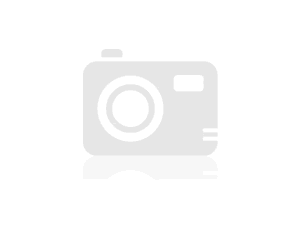






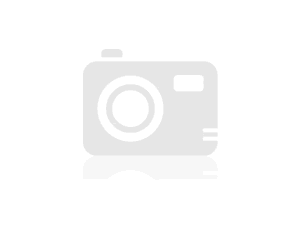




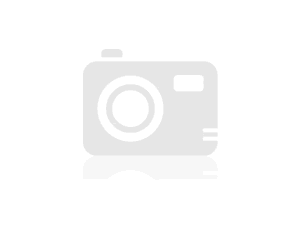



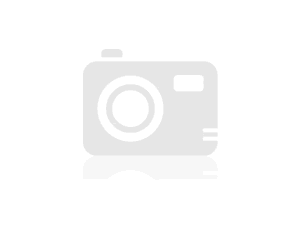



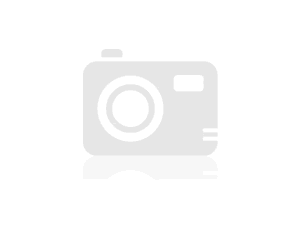








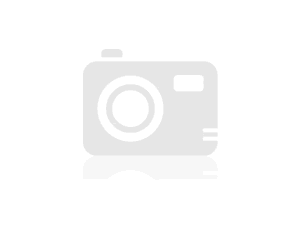



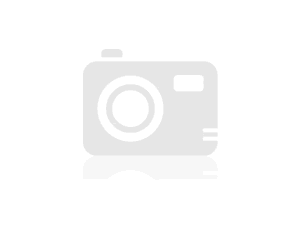







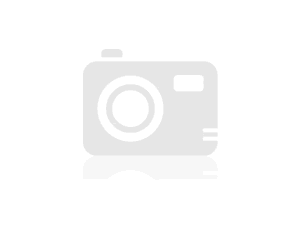



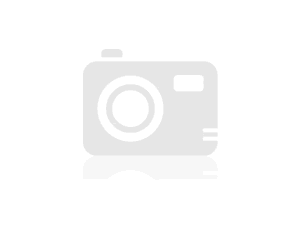





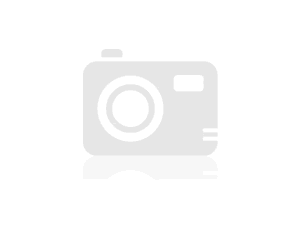






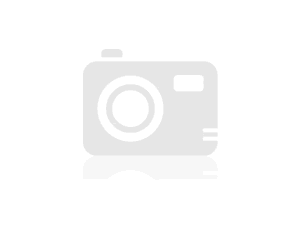








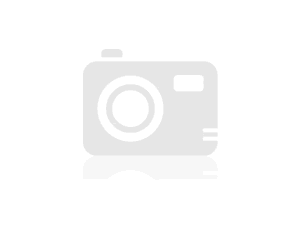



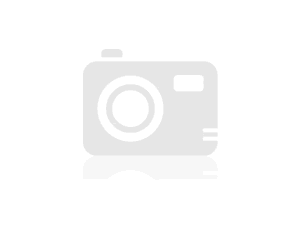



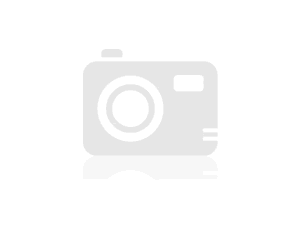





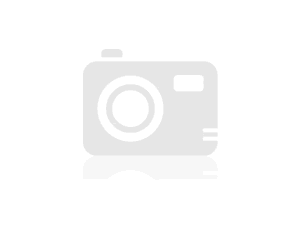






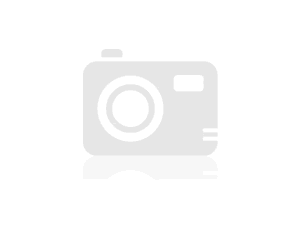






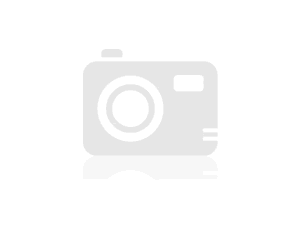






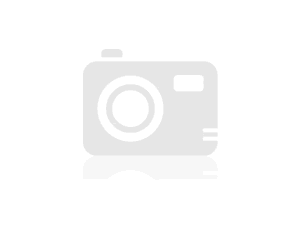





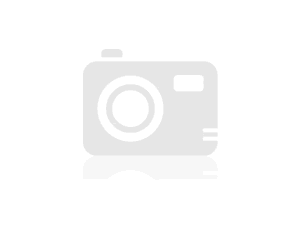









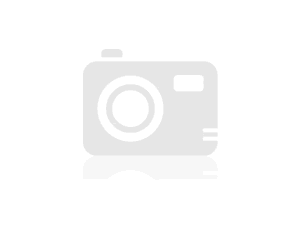




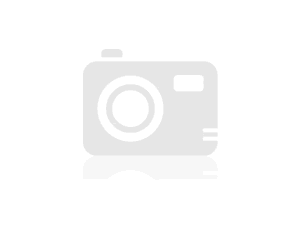



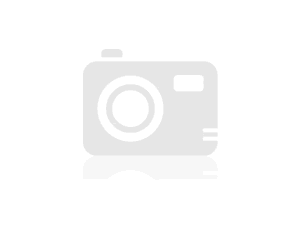








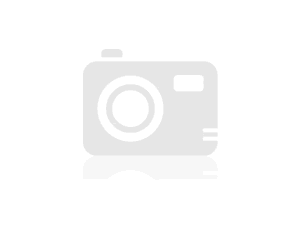





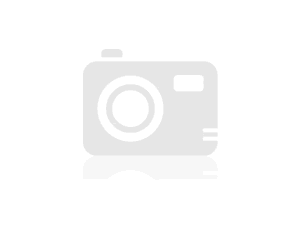




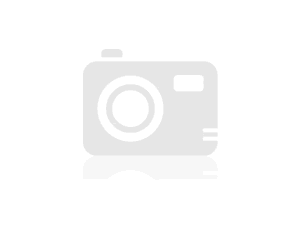





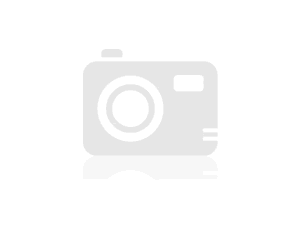








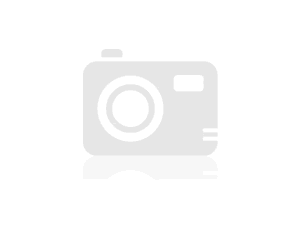







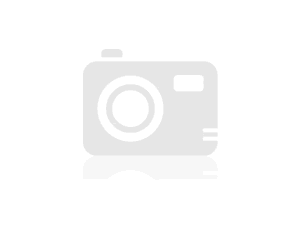





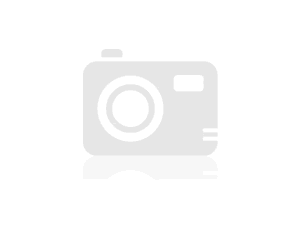




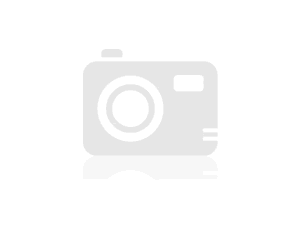






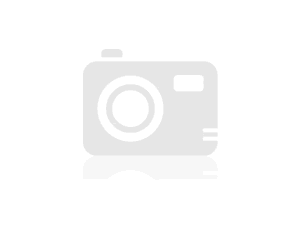







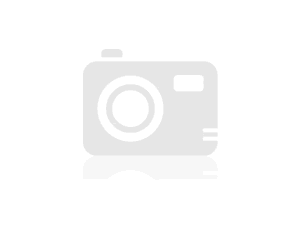






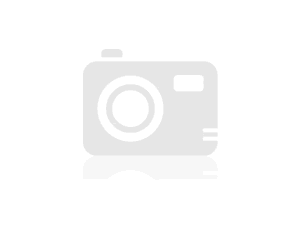




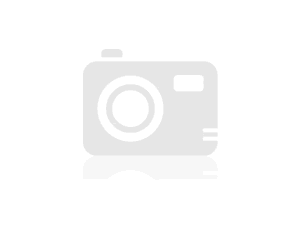






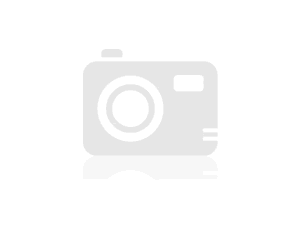







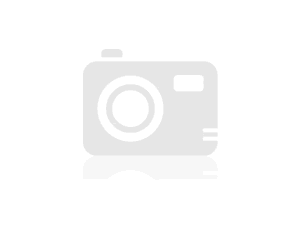





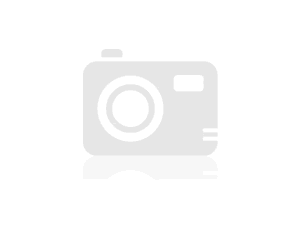





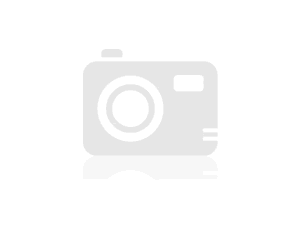





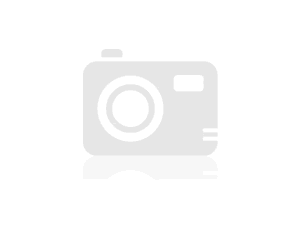






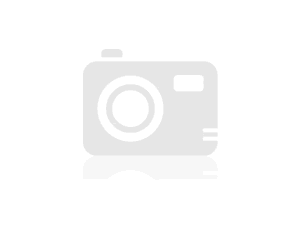






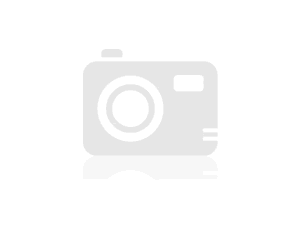






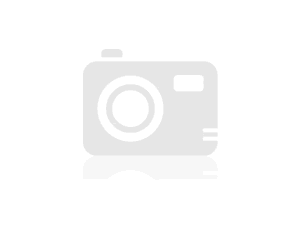





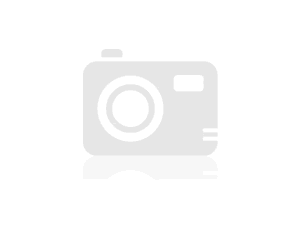








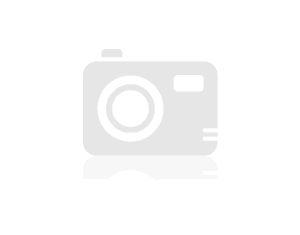
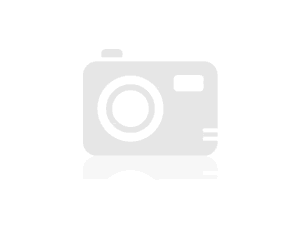
8、在Job.Personal.Tests项目中添加ProxyTest测试类。
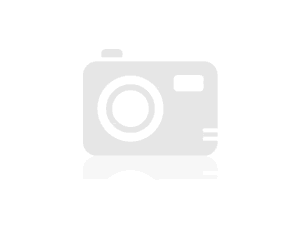
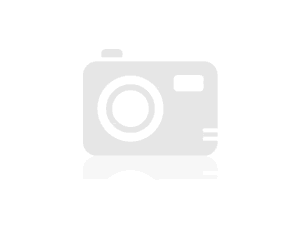
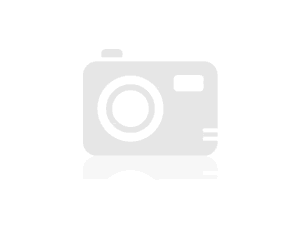
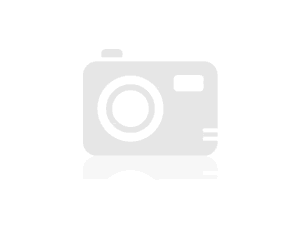
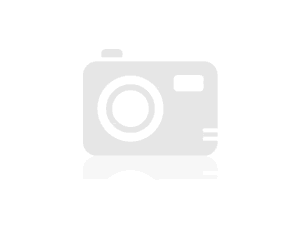
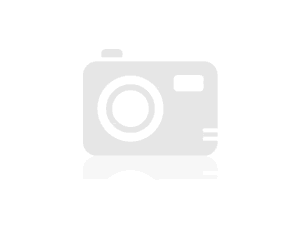
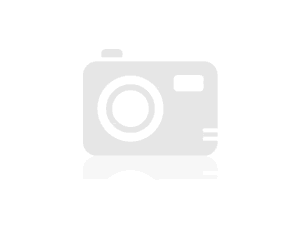
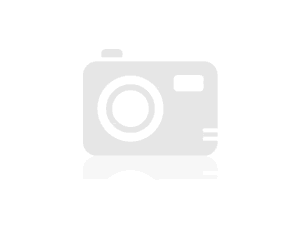
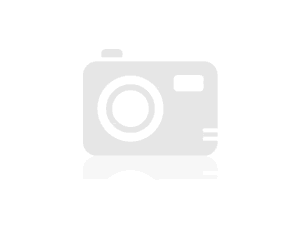
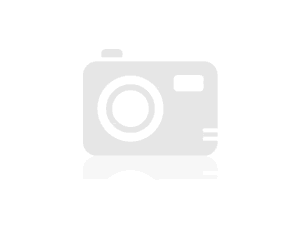
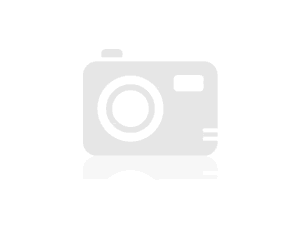
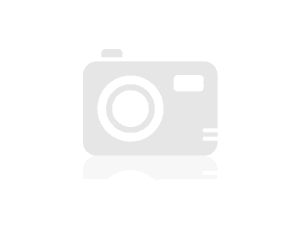
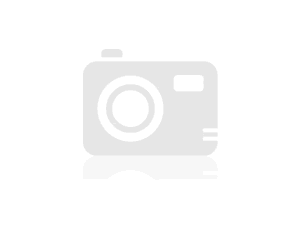




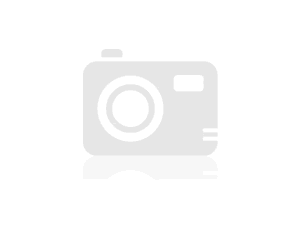


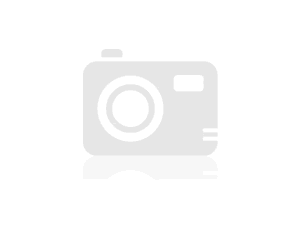





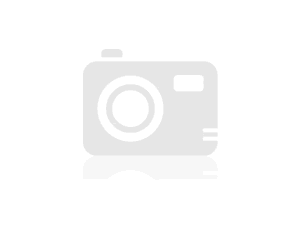












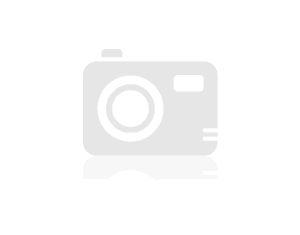
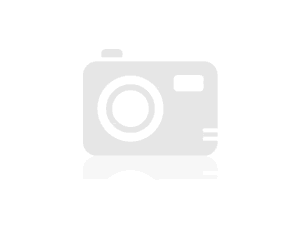
转载于:https://www.cnblogs.com/BillChen/archive/2005/10/21/259127.html
学用NHibernate(一)相关推荐
- 推荐NHibernate新书:NHibernate 3.0 CookBook[附下载]
NHibernate 3.0 CookBook这本书在2010年10月4号出版,出版后NHibernate的Lead:Fabio Maulo赠送我一份免费优惠券,我花了几天时间阅读了这本电子书,以下是 ...
- NHibernate教程
一.NHibernate简介 在今日的企业环境中,把面向对象的软件和关系数据库一起使用可能是相当麻烦.浪费时间的.NHibernate是一个面向.Net环境的对象/关系数据库映射工具.对象/关系数据库 ...
- 合适么?现在学ASP.NET Core入门编程……
现在都快找不到ASP.NET的培训课程了. 知道我要开课做培训,有同学劝我:"憋讲那什么.NET,讲Java,现在这个火!"我说我Java不熟,"唉呀!C#转Java,分 ...
- [原创]Fluent NHibernate之旅
ORM大家都非常熟悉了吧,我相信也有很多朋友正在用自己或者一些公开的框架,而最常用而且强大的,非Hibernate了(Net中为NHibernate),网上的文档非常多,不过在博客园中,介绍NHibe ...
- NHibernate入门hello world
NHibernate入门hello world 现在潮流兴orm,在博客园关于orm的充满每一个角落.所以我也来赶一赶这个潮流,拿起Nhibernate 就开始百度和google.闲话一下,为什么用N ...
- 快速生成NHibernate的映射文件和映射类的利器 —— codesmith软件
1. Codesmith软件简介 (1) Codesmith软件是一种基于模板的代码生成工具,在ORM中,它能帮助我们生成实体类.XML配置文件,从而简化了我们一部分的开发工作,它的使用类似于 ...
- NHibernate学习(转)
本文约定: 1. Nhibernate简写为NHB; 2. 本文例子的开发平台为win2000xp+sp2, sql server2000, Nhibernate0.9.1.0; 3. 使用SQL S ...
- Nhibernate教程2(3)
2) 含有关系的表的情况 含有关系的表指的是像学生这样,除了保存学生的基本信息,还希望把选课信息保存到学生的类中.这样情况下不能用软件来辅助产生对应的类和XML,这是NHibernate中 ...
- Fluent NHibernate之旅
ORM大家都非常熟悉了吧,我相信也有很多朋友正在用自己或者一些公开的框架,而最常用而且强大的,非Hibernate了(Net中为NHibernate),网上的文档非常多,不过在博客园中,介绍NHibe ...
- NHibernate学习--初识NHibernate
要学NHibernate之前先了解一下什么是ORM ORM(对象关系映射 Object Relational Mapping)是一种解决面向对象与关系型数据库之间的不匹配的技术,如图 现 ...
最新文章
- 核爆rpg手机版_好嗨游戏:不玩吃亏 ,20款全世界最佳移动RPG角色扮演游戏(上)...
- 我的Android进阶之旅------解决错误: java.util.regex.PatternSyntaxException: Incorrect Unicode property...
- notepad++主题
- Python之 sklearn:sklearn.preprocessing中的StandardScaler函数的简介及使用方法之详细攻略
- python opencv 窗口循环显示时,如果用鼠标拖动窗口会导致程序暂停(卡住)(不知道为啥。。。)
- 一条SQL更新语句是如何执行的?
- Fiori launchpad里Enter Group name这个tile是怎么配置出来的
- 互联网晚报 | 9月28日 星期二 | 乐视手机宣布回归;小鹏汽车累计交付量突破10万台;苹果售出20亿部iPhone...
- java semaphorewa_Java并发(十五):并发工具类——信号量Semaphore
- epic关于win7报错缺失api-ms-win-downlevel-kernel32-l2-1-0.dll
- 左拥快手右抱抖音,丁磊直播究竟图什么?
- jpa :配置一对多 Error accessing field 错误
- Linux与Windows的区别与比较,及Linux基本命令
- JS--购物车二级联动
- cc链2(小宇特详解)
- Android零基础入门
- CSS实现圆角,三角,五角星,五边形,爱心,12角星,8角星,圆,椭圆,圆圈,八卦等等
- 人机对战的猜拳游戏,用户通过输 入(1.剪刀 2.石头 3.布),机器随机生成(1.剪刀 2.石头 3.布),胜者积分, n 局以后通过积分的多少判定胜负。
- 梳理确定性策略梯度,随机策略梯度,AC,DPG,DDPG之间的联系
- Endnote 软件中的名词解释
热门文章
- labelcontrol 多行_ios – UISegmentedControl中的两行文本
- unittest 简单使用
- Markdown 编写示例
- SeaweedFS上手使用指南
- win7/64位下python2.7、easy_install安装经验
- C++ STL 整理
- 记我的一次重构——希望对新人有所帮助
- C#中的正则表达式 \(([^)]*)\) 详解
- Linux 找不到qt,linux – CMake找不到QtCore
- guava 的重试机制 guava-retrying 使用