php amazon-s3_推荐亚马逊电影-一种协作方法
php amazon-s3
Item-based collaborative and User-based collaborative approach for recommendation system with simple coding.
推荐系统的基于项目的协作和基于用户的协作方法,编码简单。
推荐系统概述 (Overview of Recommendation System)
There are many methods of recommendation system where each of them serve for different purposes. My previous article is talking about the simple and content-based recommendation. These recommendations are non-personalised recommenders, but that doesn’t mean they are less useful when compare to the other. These method are very popular for recommending top music of the week and recommending music of similar genre.
推荐系统的方法很多,每种方法都有不同的用途。 我的上一篇文章讨论的是基于内容的简单推荐。 这些推荐是非个性化的推荐者,但这并不意味着它们与其他推荐相比没有太大用处。 这些方法在推荐本周热门音乐和推荐类似流派的音乐时非常流行。
In this article, it will focus on collaborative filtering method. This method considers your taste in comparison to people/items that are in similar. Then, it recommends a list of items based on consumption similarity and suggest what you probably interested. These method only focus on calculating the rating.
在本文中,它将重点介绍协作过滤方法。 与相似的人/物品相比,此方法考虑了您的口味。 然后,它根据消费相似性推荐商品清单,并建议您可能感兴趣的商品。 这些方法仅专注于计算等级 。
There are two main filtering for this method: item-based filtering and user-based filtering. Item-based filtering will suggest items that are similar to what you have already liked. User-based filtering will suggest items that people similar to you have liked but you have not yet consumed.
此方法主要有两种过滤:基于项目的过滤和基于用户的过滤。 基于项目的过滤将建议与您喜欢的项目相似的项目。 基于用户的过滤将建议与您相似的人喜欢但尚未消耗的物品。
With the Amazon movie data, we will apply item-based filtering and user-based filtering recommendation methods to analyze similar items to be recommend and identify users that have similar taste.
借助Amazon电影数据 ,我们将应用基于项目的过滤和基于用户的过滤推荐方法来分析要推荐的相似项目并识别具有相似品味的用户。
分析概述 (Analysis Overview)
For both item-based filtering and user-based filtering recommendation, we need to clean data and prepare them into matrix so that it can be used for analysis. All ratings need to be in numbers and normalized and cosine similarity will be used to calculate items/users similarity.
对于基于项目的过滤和基于用户的过滤建议,我们都需要清理数据并将它们准备成矩阵,以便可以将其用于分析。 所有等级都必须以数字表示并进行归一化,余弦相似度将用于计算项目/用户相似度。
资料总览 (Data Overview)
There are 4,848 users with a total of 206 movies in the dataset.
数据集中有4848位用户,总共206部电影。
实作 (Implementation)
Now, lets import all tools that we are going to use for the analysis, put data into DataFrame, and clean them.
现在,让我们导入我们将用于分析的所有工具,将数据放入DataFrame并清理它们。
import pandas as pdimport numpy as npimport scipy as spfrom scipy import sparse from sklearn.metrics.pairwise import cosine_similarityamazon = pd.read_csv('.../Amazon.csv')amazon.info()amazon.head()


Then, we need to rearrange data into matrix format where we will set index for the rows as user_id and index for the column as name.
然后,我们需要将数据重新排列为矩阵格式,在该格式中,将行的索引设置为user_id,将列的索引设置为name。
amazon = amazon.melt(id_vars=['user_id'], var_name='name', value_name='rating')amazon_pivot = amazon.pivot_table(index=['user_id'], columns=['name'], values='rating')amazon_pivot.head()

From here, we need to normalized the rating values so that value range are closer to one and another. Then, turn the NaN values into 0 and select only those users who at least rate one movie.
从这里开始,我们需要对评级值进行归一化,以使值范围彼此接近。 然后,将NaN值设置为0,然后仅选择至少对一部电影评分的用户。
amazon_normalized = amazon_pivot.apply(lambda x: (x-np.min(x))/(np.max(x)-np.min(x)), axis=1)amazon_normalized.fillna(0, inplace=True)amazon_normalized = amazon_normalized.Tamazon_normalized = amazon_normalized.loc[:, (amazon_normalized !=0).any(axis=0)]

We nearly there. Now we need to put them into sparse matrix.
我们快到了。 现在我们需要将它们放入稀疏矩阵。
amazon_sparse = sp.sparse.csr_matrix(amazon_normalized.values)
Lets look at item-based filtering recommendation.
让我们看一下基于项目的过滤建议 。
item_similarity = cosine_similarity(amazon_sparse)item_sim_df = pd.DataFrame(item_similarity, index=amazon_normalized.index, columns=amazon_normalized.index)item_sim_df.head()

All the columns and rows are now become each of the movie and it is ready for the recommendation calculation.
现在,所有的列和行都成为电影的每一个,并且可以进行推荐计算了。
def top_movie(movie_name): for item in item_sim_df.sort_values(by = movie_name, ascending = False).index[1:11]: print('Similar movie:{}'.format(item))top_movie("Movie102")

These are the movies that are similar to Movie102.
这些是与Movie102类似的电影。
Lets look at user-based filtering recommendation. Who has similar taste to me?
让我们看一下基于用户的过滤推荐 。 谁有和我相似的品味?
user_similarity = cosine_similarity(amazon_sparse.T)user_sim_df = pd.DataFrame(user_similarity, index = amazon_normalized.columns, columns = amazon_normalized.columns)user_sim_df.head()

def top_users(user): sim_values = user_sim_df.sort_values(by=user, ascending=False).loc[:,user].tolist()[1:11] sim_users = user_sim_df.sort_values(by=user, ascending=False).index[1:11] zipped = zip(sim_users, sim_values) for user, sim in zipped: print('User #{0}, Similarity value: {1:.2f}'.format(user, sim))top_users('A140XH16IKR4B0')
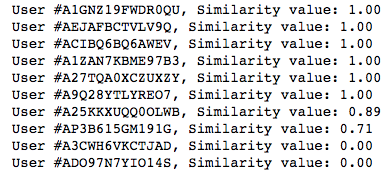
These are the examples on how to implement the item-based and user-based filtering recommendation system. Some of the code are from https://www.kaggle.com/ajmichelutti/collaborative-filtering-on-anime-data
这些是有关如何实施基于项目和基于用户的过滤推荐系统的示例。 一些代码来自https://www.kaggle.com/ajmichelutti/collaborative-filtering-on-anime-data
Hope that you enjoy!
希望你喜欢!
翻译自: https://medium.com/analytics-vidhya/recommend-amazon-movie-a-collaborative-approach-9b3db8f48ad6
php amazon-s3
http://www.taodudu.cc/news/show-997448.html
相关文章:
- 简述yolo1-yolo3_使用YOLO框架进行对象检测的综合指南-第一部分
- 数据库:存储过程_数据科学过程:摘要
- cnn对网络数据预处理_CNN中的数据预处理和网络构建
- 消解原理推理_什么是推理统计中的Z检验及其工作原理?
- 大学生信息安全_给大学生的信息
- 特斯拉最安全的车_特斯拉现在是最受欢迎的租车选择
- ml dl el学习_DeepChem —在生命科学和化学信息学中使用ML和DL的框架
- 用户参与度与活跃度的区别_用户参与度突然下降
- 数据草拟:使您的团队热爱数据的研讨会
- c++ 时间序列工具包_我的时间序列工具包
- adobe 书签怎么设置_让我们设置一些规则…没有Adobe Analytics处理规则
- 分类预测回归预测_我们应该如何汇总分类预测?
- 神经网络推理_分析神经网络推理性能的新工具
- 27个机器学习图表翻译_使用机器学习的信息图表信息组织
- 面向Tableau开发人员的Python简要介绍(第4部分)
- 探索感染了COVID-19的动物的数据
- 已知两点坐标拾取怎么操作_已知的操作员学习-第4部分
- lime 模型_使用LIME的糖尿病预测模型解释— OneZeroBlog
- 永无止境_永无止境地死:
- 吴恩达神经网络1-2-2_图神经网络进行药物发现-第1部分
- python 数据框缺失值_Python:处理数据框中的缺失值
- 外星人图像和外星人太空船_卫星图像:来自太空的见解
- 棒棒糖 宏_棒棒糖图表
- nlp自然语言处理_不要被NLP Research淹没
- 时间序列预测 预测时间段_应用时间序列预测:美国住宅
- 经验主义 保守主义_为什么我们需要行动主义-始终如此。
- python机器学习预测_使用Python和机器学习预测未来的股市趋势
- knn 机器学习_机器学习:通过预测意大利葡萄酒的品种来观察KNN的工作方式
- python 实现分步累加_Python网页爬取分步指南
- 用于MLOps的MLflow简介第1部分:Anaconda环境
php amazon-s3_推荐亚马逊电影-一种协作方法相关推荐
- 提高亚马逊关键词搜索排名的方法
亚马逊关键词搜索排名在首页是很重要的,因为客户都是通过关键词搜索产品,如果你的产品通过关键词搜索出来排在了后面几页,那么消费者基本上都不会去查看及购买,因此想要产品能被发现及购买,提高亚马逊关键词的搜 ...
- AWS(Amazon Web Services, 亚马逊网络服务系统)简介
1.简介 亚马逊网络服务系统(英语:Amazon Web Services,简称为AWS),由亚马逊公司所建立的云端运算平台,提供许多远端Web服务.Amazon EC2与Amazon S3都架构在这 ...
- amazon数据集_亚马逊增加了新服务:公共数据集
amazon数据集 Amazon announced the released of a new web service today that aims to facilitate easier ac ...
- 手机连接amazon虚拟机服务器,亚马逊EC2可直接导入VMware虚拟机镜像
亚马逊网络服务(AWS)周五表示,将提供一个新的VMware管理平台插件,方便IT人员将虚拟机镜像转移到AWS云应用中. 亚马逊EC2 VM Import Connector是为VMware vCen ...
- 亚马逊的18种关联因素及防范方式
首先,亚马逊只允许个人或者公司拥有一个账号.无论是电话.地址.邮箱.姓名.银行卡.电脑IP.网卡.浏览器.cookie.路由器.等等因素存在相同,都可能被判定为关联.但是一般的亚马逊老玩家手里不可能只 ...
- 亚马逊6页纸开会方法!
一.什么是亚马逊6页纸? 亚马逊在内部管理实践中,特别是会议管理上,禁止使用 PPT,而是使用一种简洁的「结构化备忘录」,也就是我们熟知的"六页纸". 二.6页纸思路整理 那么&q ...
- php 亚马逊关键字排名,亚马逊提升关键词排名的方法
影响关键词排名的变化有以下四种因素造成: 1.销量 2.转化率:在固定访客数的情况下转化为购买订单的数量,转化率的高低也影响着关键词排名,而不是流量越多就越好 3.产品相关性:亚马逊系统会根据List ...
- 高效提升亚马逊搜索排名的十个方法,赶紧学起来
在亚马逊这个销售大舞台上,你可得掌握一招半式,别让你的产品在搜索结果里默默无闻呀!说到亚马逊搜索排名,可不是开玩笑的,它直接关系着你的产品能不能被客户看到.买到!想让你的货品在搜索结果的前排儿亮相?咱 ...
- 亚马逊关键词上首页的方法有哪些?
关键词上首页一般是指在某个核心关键词搜索条件下的首页48个结果内,自己的产品排在前10的位置.分为两种情况.第一种是广告上首页,通过自动广告积累一定的买家搜索词数据,然后针对出现频率和转化相对较高的核 ...
最新文章
- php 长连接心跳_支持gRPC长链接,深度解读Nacos2.0架构设计及新模型
- Linux的epoll
- 2010.12.14 关于decimal和Numeric类型
- Supervisor重新加载配置启动新的进程
- MAC OS X 10.8 操作远程SSH服务器 + 无密码输入使用SSH服务器
- java与数据结构(4)---java实现双向循环链表
- docker安装Tomcat
- java图像处理,彩色图像转灰度图的几种实现方式
- 给超链接(a标签)加onclick事件
- 使用DispatcherTimer计时器
- 程序员想知道代码是怎样跑起来的
- git 管理 Linux 文件系统
- 备战毕设——JAVA基础(简单的数据类型和语句类型)
- 25.龙贝格求积公式
- 数据结构大作-学生信息管理系统
- 风口之下,隐形正畸还能走多远?
- AutoCAD、Revit、Maya、3dsMax出现许可管理器不起作用
- vlan的端口隔离及端口优化——“道高一尺魔高一丈”
- vue-loader无法解析vue里的stylus的style,外部引入styl文件可以解析,引入VueLoaderPlugin也没用
- Win10开机自动同步时间