有重复数字的组合问题_带数字重复的组合和问题
有重复数字的组合问题
Description:
描述:
This is a standard interview problem to make some combination of the numbers whose sum equals to a given number using backtracking.
这是一个标准的面试问题,它使用回溯功能将总和等于给定数字的数字进行某种组合。
Problem statement:
问题陈述:
Given a set of positive numbers and a number, your task is to find out the combinations of the numbers from the set whose summation equals to the given number. You can use the numbers repeatedly to make the combination.
给定一组正数和一个数字,您的任务是从集合中找出总和等于给定数字的数字组合。 您可以重复使用数字进行组合。
Input:
Test case T
T no. of N values and corresponding N positive numbers and the Number.
E.g.
3
3
2 3 5
12
3
2 7 5
14
4
1 2 3 4
5
Constrains:
1 <= T <= 500
1 <= N <= 20
1 <= A[i] <= 9
1 <= Number<= 50
Output:
Print all the combination which summation equals to the given number.
Example
例
Input:
N=3
Set[ ]=2 3 5
Number=12
Output:
2 2 2 2 2 2
2 2 2 3 3
2 2 3 5
2 5 5
3 3 3 3
Explanation with example:
举例说明:
Let there is a set S of positive numbers N and a positive number.
设一组正数N和一个正数S。
For pre-requisite, we are recommending you to go to our article combinational sum problem.
对于先决条件,我们建议您转到我们的文章组合总和问题 。
Making some combinations repeatedly using a number in such a way that the summation of that combination results that given number is a problem of combination and we will solve this problem using backtracking approach.
重复使用数字进行一些组合,使得该组合的总和导致给定数字是组合的问题,我们将使用回溯方法解决该问题。
Let,
f(i) = function to insert the isth number into the combinational subset
In this case we will consider two cases to solve the problem,
在这种情况下,我们将考虑两种情况来解决该问题,
We will consider ith element into the part of our combination subset until the sum greater the given number (f(i)).
我们将考虑第 i 个元素进入组合子集的一部分,直到总和大于给定数( f(i) )。
We will not consider ith element into the part of our combination subset (not f(i)).
我们不会在组合子集的一部分( 不是f(i) )中考虑第ith个元素。
And every time we will check the current sum with the number. Each of the time we will count the number of occurrence and the also the combinations.
并且每次我们将用数字检查当前总和。 每次,我们将计算发生的次数以及组合。
Let,
f(i) = function to insert the ith number into the combinational subset
For the input:
对于输入:
S[] = {2, 3, 5}
Number = 12
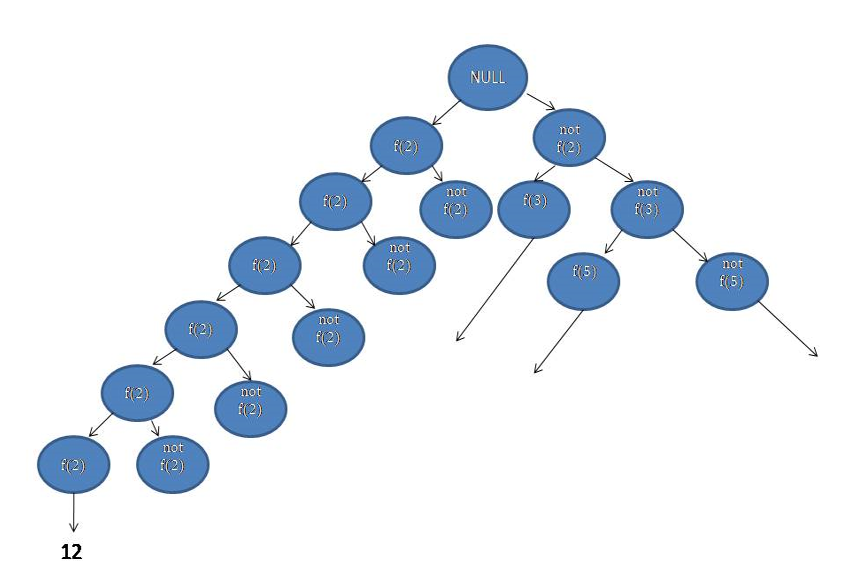
Here from every (not f(2)) there will be two edges (f(3)) and (not f(3)) again from (not f(3)) there will be ( f(5)) and (not f(5)). Here in this case we will discard that edges which have a current sum greater than the given number and make a count to those numbers which are equal to the given number.
在每个(非f(2))中将有两个边(f(3))和(非f(3))从(非f(3))再有(f(5))和(非f(5)) 。 在这种情况下,我们将丢弃当前总和大于给定数字的那些边,并对等于给定数字的那些数字进行计数。
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
void combination(int* arr, int n, int num, int pos, int curr_sum, vector<vector<int> >& v, vector<int> vi, set<vector<int> >& s)
{if (num < curr_sum)
return;
if (num == curr_sum && s.find(vi) == s.end()) {s.insert(vi);
v.push_back(vi);
return;
}
//go for the next elements for combination
for (int i = pos; i < n; i++) {if (curr_sum + arr[i] <= num) {vi.push_back(arr[i]);
combination(arr, n, num, i, curr_sum + arr[i], v, vi, s);
vi.pop_back();
}
}
}
//print the vector
void print(vector<vector<int> > v)
{for (int i = 0; i < v.size(); i++) {for (int j = 0; j < v[i].size(); j++) {cout << v[i][j] << " ";
}
cout << endl;
}
}
void combinational_sum(int* arr, int n, int num)
{vector<vector<int> > v;
vector<int> vi;
int pos = 0;
int curr_sum = 0;
set<vector<int> > s;
combination(arr, n, num, pos, curr_sum, v, vi, s);
print(v);
}
int main()
{int t;
cout << "Test Case : ";
cin >> t;
while (t--) {int n, num;
cout << "Enter the value of N : ";
cin >> n;
int arr[n];
cout << "Enter the values : ";
for (int i = 0; i < n; i++) {cin >> arr[i];
}
sort(arr, arr + n);
cout << "Enter the number : ";
cin >> num;
combinational_sum(arr, n, num);
}
return 0;
}
Output
输出量
Test Case : 3
Enter the value of N : 3
Enter the values : 2 3 5
Enter the number : 12
2 2 2 2 2 2
2 2 2 3 3
2 2 3 5
2 5 5
3 3 3 3
Enter the value of N : 3
Enter the values : 2 7 5
Enter the number : 14
2 2 2 2 2 2 2
2 2 5 5
2 5 7
7 7
Enter the value of N : 4
Enter the values : 1 2 3 4
Enter the number : 5
1 1 1 1 1
1 1 1 2
1 1 3
1 2 2
1 4
2 3
翻译自: https://www.includehelp.com/icp/combinational-sum-problem-with-repetition-of-digits.aspx
有重复数字的组合问题
有重复数字的组合问题_带数字重复的组合和问题相关推荐
- 定义一个类:实现功能可以返回随机的10个数字,随机的10个字母, 随机的10个字母和数字的组合;字母和数字的范围可以指定,类似(1~100)(A~z)...
#习题2:定义一个类:实现功能可以返回随机的10个数字,随机的10个字母, #随机的10个字母和数字的组合:字母和数字的范围可以指定class RandomString():#随机数选择的范围作为参数 ...
- 怎么将一个数字高低位互换_多彩数字 多彩童年——东城幼儿园玩具研究教学案例...
玩具是儿童的天使,孩子在天使的陪伴下,创造性地进行着自己的游戏活动.在幼儿众多的玩具中怎样甄别一款好玩的玩具,挖掘出玩具的最大教育价值,让他们在和玩具的互动中快乐地学习呢?下面,我们来看看老师们是怎样 ...
- 数字盲打怎么练_会计数字键盘盲打技巧
1 会计 数字键盘盲打技巧 以下是会计数字键盘盲打技巧等等的介绍,希望可以为您提供帮助. 数字小键盘区(又名'辅助键区')位于键盘的右下方,主要用于快速输入数字,并加以编辑处理.它特别适合于经常与数字 ...
- python数字排列组合去重_排列组合-生成集合的所有子集
//一个有N个不重复元素的集合的某个子集,可以用这个N个元素中每个元素在或是不在这个子集中来表示. //把这N个元素一字排开,每个位置可以用1来标识对应位置的元素在子集中,用0来标识这个元素不在子集中 ...
- python数字转拼音输出_将数字拼音转换为带声调的拼音
我有一些python3代码可以做到这一点,它足够小,可以直接放在这里的答案中.在PinyinToneMark = { 0: "aoeiuv\u00fc", 1: "\u0 ...
- java猜数字游戏应用程序_猜数字游戏的Java小程序
/* 猜数字游戏: 1,产生随机数. 2,获取键盘录入. 3,将录入数据变成数字,和随机数比较. 给出提示信息. 4,重复这个过程,如果猜中,程序就结束. 注意:对于输入1~100以外的数字,,以及非 ...
- java将数字替换为空_将数字替换为java中正确位置的单词
实际上我正试图用用户给出的句子中的数字替换为单词.本案例日期格式;例如:我的生日是在16/6/2000,我是 java的新手 – >成为->我的生日是七月十六日,我是java的新手 这是代 ...
- python猜数字统计游戏次数_猜数字游戏(Python)
在Python3中工作.在 我对Python还比较陌生(只有几个星期的知识).在 这个程序给我的提示是写一个随机数游戏,用户必须猜出随机数(1到100之间),如果不正确,就会提示它太低或太高.然后用户 ...
- 分离数字的python编码_把数字拆分成2的幂的和
问题: 任何数都能分解成2的幂,比如 7=1+1+1+1+1+1+1 =1+1+1+1+1+2 =1+1+1+2+2 =1+2+2+2 =1+1+1+4 =1+2+4 共有6种分解方式,设f(n)为任 ...
最新文章
- 独家 | 11步转行数据科学家 (送给数据员/ MIS / BI分析师)
- 【学术相关】如何将半页纸论文写到十页?
- Java开发人员在编程中常见的雷!
- 吴恩达 deeplearning.ai 经典总结:28 张精炼图+思维导图(附下载链接)
- A - Greed CodeForces - 892A(水题)
- a byte of python中文版_面试官问 Python 版 “垃圾回收”机制,我没答上来
- C语言变量声明和定义 - C语言零基础入门教程
- 前端之BOM和DOM
- 正射影像、倾斜摄影测量相关软件汇总
- window双网卡上网
- PS 工具获取:Photoshop CS6超级免安装精简版来临!不到200M!
- 每日一道leetcode(python)347. 前 K 个高频元素
- 【Scratch考级99图】图36-等级考试scratch绘制复杂图形中间带凸点正方形花 少儿编程 scratch画图案例教程
- 吐血总结《Mysql从入门到入魔》,图文并茂
- 最全的数据中心(IDC)机房整体工程介绍
- 六十甲子亡命安葬山向宜忌
- Java面试准备(四)——Java8特性
- 『JavaScript』JS简介
- 罗技计算机配置存入板载内存,板载内存 没有驱动也能自定义_鼠标_键鼠导购-中关村在线...
- 微信小程序连接阿里云物联网平台操控设备(IOT)一