使用OpenCV在Python中进行人脸和眼睛检测
Modules Used:
使用的模块:
python-opencv(cv2)
python-opencv(cv2)
python-opencv(cv2)
Opencv(Open source computer vision) is a python library that will help us to solve computer vision problems.
Opencv(开源计算机视觉)是一个Python库,可帮助我们解决计算机视觉问题。
Download python-opencv(cv2)
下载python-opencv(cv2)
General Way: pip install python-opencv
通用方式: pip install python-opencv
Pycharm users: Pycharm users can download this module from the project interpreter.
Pycharm用户:Pycharm用户可以从项目解释器下载此模块。
Here, we will detect the face and eyes of the individual. For this, we use the webcam of our system and the XML files to detect the face and eyes. We will detect the face in the frame and after that eyes are in the face so we will enter into the coordinates of the face and will detect the eyes and draw the rectangle on the face and eye detected.
在这里,我们将检测个人的脸和眼睛 。 为此,我们使用系统的网络摄像头和XML文件来检测面部和眼睛。 我们将在帧中检测到面部,然后将眼睛插入面部,因此我们将进入面部坐标并检测眼睛,并在检测到的面部和眼睛上绘制矩形。
Functions related the face and eye detection
与面部和眼睛检测相关的功能
cv2.CascadeClassifier("<xml file for detection>"): This function is for getting the face and eyes extracts, how we will detect them.
cv2.CascadeClassifier(“ <用于检测的xml文件>”):此函数用于获取面部和眼睛的提取物,以及我们将如何检测它们。
cv2.Videocapture(): This is for the Videocapturing through the webcam of our system.
cv2.Videocapture():用于通过我们系统的网络摄像头进行视频捕获。
<face or eye extract>.detectmultiscale(<Gray_scale image>,1.3,5): To detect the the face or eyes in the frame.
<面部或眼睛提取物> .detectmultiscale(<灰度图像>,1.3,5):检测帧中的面部或眼睛。
cv2.rectangle(<frame on which we want the rectangle>,(<starting position>,<ending position>,(<color>),thickness=<thickness of the border>)
cv2.rectangle(<我们要在其上放置矩形的框架>,(<开始位置>,<结束位置>,(<颜色>),厚度= <边框的厚度>)
Note: The detection of the face and eyes will be in grayscale mode.
注意:面部和眼睛的检测将处于灰度模式。
The link to the XML files for the face and eye detection is :
用于面部和眼睛检测的XML文件的链接是:
Face : https://github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/face.xml
人脸: https : //github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/face.xml
Eye: https://github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/eye.xml
眼睛: https : //github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/eye.xml
用于检测人脸和眼睛的Python代码 (Python code to detect face and eyes)
# import the modules
import cv2
# now we have the haarcascades files
# to detect the face and eyes to detect the face
faces=cv2.CascadeClassifier("face.xml")
# to detect the eyes
eyes=cv2.CascadeClassifier("eye.xml")
# capture the frame through webcam
capture=cv2.VideoCapture(0)
# now running the loop for the webcam
while True:
# reading the webcam
ret,frame=capture.read()
# now the face is in the frame
# the detection is done with the gray scale frame
gray_frame=cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY)
face=faces.detectMultiScale(gray_frame,1.3,5)
# now getting into the face and its position
for (x,y,w,h) in face:
# drawing the rectangle on the face
cv2.rectangle(frame,(x,y),(x+w,y+h),(0,0,255),thickness=4)
# now the eyes are on the face
# so we have to make the face frame gray
gray_face=gray_frame[y:y+h,x:x+w]
# make the color face also
color_face=frame[y:y+h,x:x+w]
# check the eyes on this face
eye=eyes.detectMultiScale(gray_face,1.3,5)
# get into the eyes with its position
for (a,b,c,d) in eye:
# we have to draw the rectangle on the
# coloured face
cv2.rectangle(color_face,(a,b),(a+c,b+d),(0,255,0),thickness=4)
# show the frame
cv2.imshow("Abhinav's Frame",frame)
if cv2.waitKey(1)==13:
break
# after ending the loop release the frame
capture.release()
cv2.destroyAllWindows()
Output:
输出:
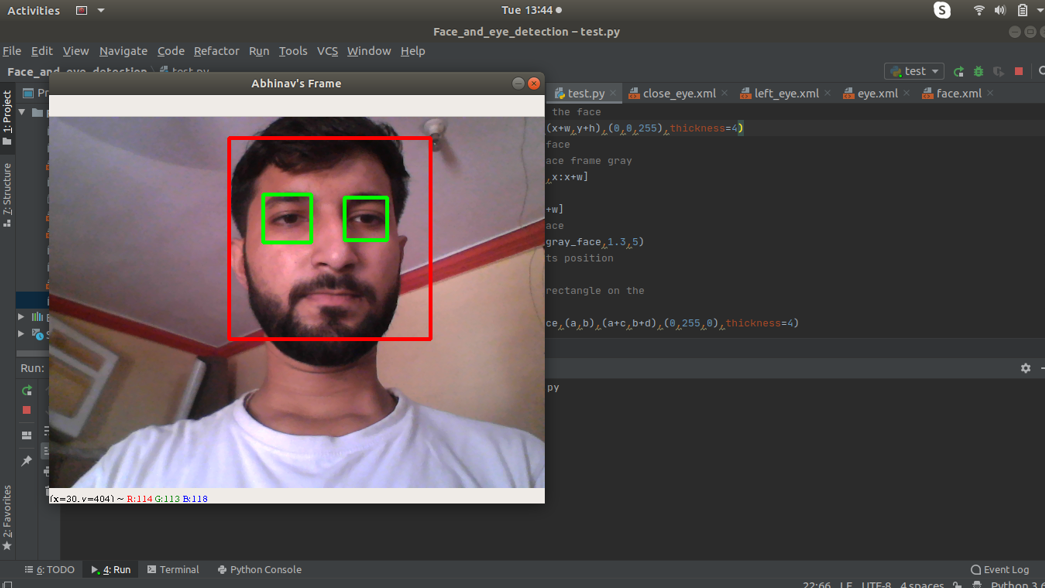
翻译自: https://www.includehelp.com/python/face-and-eye-detection-in-python-using-opencv.aspx
使用OpenCV在Python中进行人脸和眼睛检测相关推荐
- 如何用OpenCV在Python中实现人脸检测
选自towardsdatascience 作者:Maël Fabien 机器之心编译 参与:高璇.张倩.淑婷 本教程将介绍如何使用 OpenCV 和 Dlib 在 Python 中创建和运行人脸检测算 ...
- 【OpenCV图像处理入门学习教程六】基于Python的网络爬虫与OpenCV扩展库中的人脸识别算法比较
OpenCV图像处理入门学习教程系列,上一篇第五篇:基于背景差分法的视频目标运动侦测 一.网络爬虫简介(Python3) 网络爬虫,大家应该不陌生了.接下来援引一些Jack-Cui在专栏<Pyt ...
- 使用网络摄像头在 Python 中进行人脸检测
本教程是Python 中的人脸识别的后续教程,因此请确保您已经阅读了第一篇文章. 正如第一篇文章中提到的,通过网络摄像头从检测图像中的人脸到检测视频中的人脸非常容易--这正是我们将在本文中详细介绍的内 ...
- 调用摄像头使用face_recognition 或 opencv中haar人脸特征实时检测识别人脸、给人脸打马赛克/给人脸贴图
日萌社 人工智能AI:Keras PyTorch MXNet TensorFlow PaddlePaddle 深度学习实战(不定时更新) OpenCV:python调用摄像头同时使用OpenCV中自带 ...
- 颜色空间以及如何在OpenCV和Python中使用它们
It may be the era of deep learning and big data, where complex algorithms analyze images by being sh ...
- Pyhton,OpenCV对象检测之——Haar级联人脸及眼睛检测
Pyhton,OpenCV对象检测之--Haar级联人脸及眼睛检测 1. 效果图 2. 原理 2.1 Haar人脸检测原理 2.2 Haar级联预训练的模型 3. 源码 3.1 图像面部及眼睛检测 3 ...
- 使用OpenCV-python识别图片视频中的人脸和眼睛
文章目录 思路和核心代码 识别图像中的人脸 识别视频中的人脸和眼睛 思路和核心代码 思路如下: 首先需要一个训练好的人脸和眼睛特征数据库,用于识别人脸和眼睛 识别出人脸和眼睛之后,返回所在的区域(一般 ...
- python 人脸检测_借助摄像头在Python中实现人脸检测
Python部落(www.freelycode.com)组织翻译, 禁止转载 本文作者是Shantnu Tiwari--曾多年在C/C++的魔爪中饱受折磨,直到他发现了Python--使用起来感觉如呼 ...
- 科研日志——使用opencv提取视频中的人脸
科研任务需要,将视频中与文字和音频对应的视频帧根据时间戳提取出来,并截取视频帧中的人脸.使用的工具是opencv,步骤如下: 1. 提取视频帧 Sentence标号的含义 数据集中对视频中的每个sen ...
最新文章
- SQLServer之创建非聚集索引
- C语言 矩阵的几种乘法
- 向前欧拉公式 matlab_你可能不知道的MATLAB操作#第三话
- 关于meta name=viewport content=width=device-width, initial-scale=1.0, maximum-scale=1.0, user-sc..
- 互联网企业安全高级指南3.7.2 SDL落地率低的原因
- Linux基础 —— Linux终端命令格式
- Uzi宣布退役:身体条件不允许再继续战斗了!
- ChaiNext:大盘调整,主流币种还未稳住阻力位
- http,tcp的长连接和短连接
- javascript中的||运算符
- 【转贴】二节棍精典棍花动作详解
- 移远BC26模组连接阿里云物联网平台
- 安防摄像头互联网直播方案LiveGBS设计文档
- Failed to send a request to Slack API server: <urlopen error [SSL: CERTIFICATE_VERIFY_FAILED] certif
- 1年2轮融资团队2倍扩张,180人的产研团队如何有序协同?
- 网格化管理服务系统,携同用户创新共进步
- elementUI使用卡槽二次封装table(亲测可用)
- 天下数据支招如何防范域名被劫持
- C/C++ 控制台高级操作(非常详细)
- 怎么复制cmd显示的内容?怎么把外面的东西复制到cmd里面?
热门文章
- python在浏览器运行一片空白_Webdriver启动Firefox浏览器后,页面显示空白
- Mysql保存是事件驱动吗_【CHRIS RICHARDSON 微服务系列】事件驱动的数据管理-5
- 安装python3.9
- JDK源码解析之 java.lang.Long
- 「javaScript-每三位插入一个逗号实现方式」
- PostgreSQL 并行查询概述
- Improved GAN
- 国内远程医疗市场快速增长
- CycleGAN 各种变变变
- onActivityResult()后onresume()