elixir 教程_认识Elixir,Laravel编译资产的方式
elixir 教程
Many thanks to Jad Joubran, Gabriel Zerbib, Anthony Chambers, and Scott Molinari for peer reviewing this post, and thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
非常感谢Jad Joubran , Gabriel Zerbib , Anthony Chambers和Scott Molinari对该文章的同行审阅 ,也感谢所有SitePoint的同行审阅者使SitePoint内容达到最佳状态!
In today’s web applications, we use a wide variety of tools to speed up the development workflow while keeping the code base as lean as possible. However, these tools might slow the process down, as some of them require compilation of the code, which takes time.
在当今的Web应用程序中,我们使用各种各样的工具来加快开发流程,同时保持代码库尽可能的精简。 但是,这些工具可能会减慢该过程的速度,因为其中一些工具需要编译代码,这需要时间。
One such type of tool are preprocessors for CSS and JavaScript. They reduce the amount of code in our stylesheets and JavaScript files, but we still need to compile them to vanilla CSS and JavaScript by running the right compiler. After a while, this will become a boring task and chances of us forgetting to run something will increase.
一种此类工具是CSS和JavaScript的预处理器。 它们减少了样式表和JavaScript文件中的代码量,但是我们仍然需要通过运行正确的编译器将它们编译为原始CSS和JavaScript。 一段时间后,这将成为无聊的任务,而我们忘记运行某些东西的机会将会增加。
Besides preprocessors, there are also many tasks we as developers often have to do, including linting JS files, testing, concatenation, minification, just to name a few.
除预处理程序外,开发人员还经常需要完成许多任务,包括整理JS文件,测试,连接,缩小等。
All this led to the creation of JavaScript-based task runners like Grunt and Gulp. The purpose of these applications is to automate the tasks we need to do repeatedly in our development workflow.
所有这些导致了诸如Grunt和Gulp之类的基于JavaScript的任务运行器的创建。 这些应用程序的目的是使我们在开发工作流程中需要重复执行的任务自动化。
Gulp syntax is clean and easy to use, but there’s always room for doing things the easier way. Laravel 5 introduced Elixir: a Node.js based tool developed by Jeffrey Way, which manages our Gulp tasks the easy way.
Gulp语法很干净而且易于使用,但是总有空间以更简单的方式处理事务。 Laravel 5引入了Elixir :这是由Jeffrey Way开发的基于Node.js的工具,该工具可以轻松管理Gulp任务。
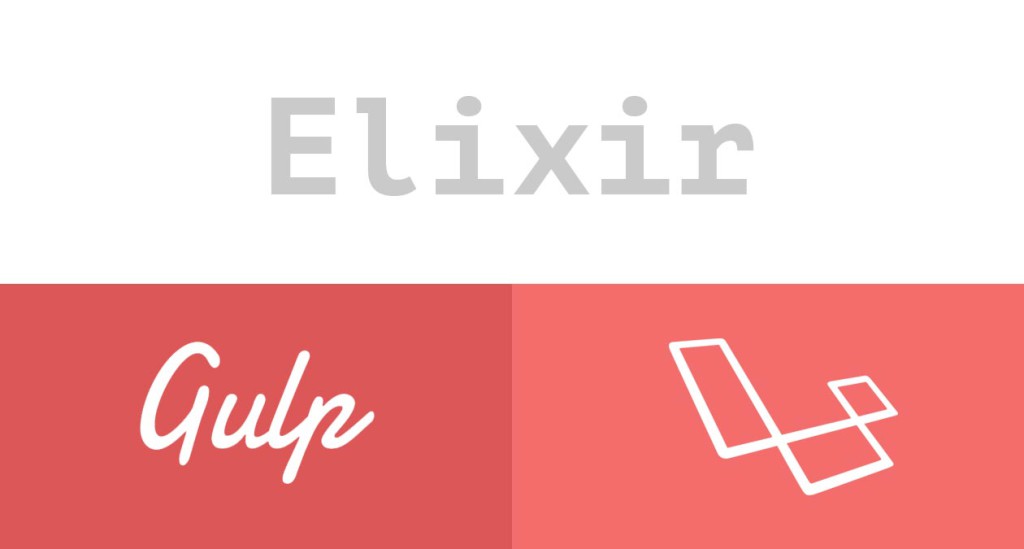
In this tutorial, we will learn how to use Elixir to manage our Gulp tasks with just a few lines of code. This article focuses on asset compilation in the Laravel environment, though we’ll try to cover usage in other environments as well.
在本教程中,我们将学习如何使用Elixir通过几行代码来管理Gulp任务。 本文重点介绍Laravel环境中的资产编译,尽管我们还将尝试介绍其他环境中的用法。
要求和安装 (Requirements and Installation)
To run Elixir, we’ll need to have the following tools installed on our machine:
要运行Elixir,我们需要在计算机上安装以下工具:
Node.js
Node.js
Gulp
古尔普
Laravel Elixir
拉拉维尔药剂
Node.js (Node.js)
Since Gulp is run inside Node.js, we need to make sure Node.js is installed. If we’re using Homestead Improved, then we’re already good to go, otherwise we have to install Node.js.
由于Gulp是在Node.js内部运行的,因此我们需要确保已安装Node.js。 如果我们正在使用Homestead Improvementd ,那么我们已经很不错了,否则我们必须安装Node.js。
Gulp.js (Gulp.js)
As mentioned earlier, Elixir is a wrapper around Gulp, so we will need to have Gulp running as well.
如前所述,Elixir是Gulp的包装,因此我们也需要运行Gulp。
If it’s not installed yet (if we’re using Homestead Improved, it is), we can install it via npm
:
如果尚未安装(如果我们正在使用Homestead Improvement ,则可以通过npm
进行安装):
npm install --global gulp
拉拉维尔药剂 (Laravel Elixir)
In Laravel’s root directory, there’s a package.json
file which already has laravel-elixir 3.0.0,
as a dependency. To install it, we run npm install
from within that directory.
在Laravel的根目录中,有一个package.json
文件,该文件已经具有laravel-elixir 3.0.0,
作为依赖项。 要安装它,我们从该目录中运行npm install
。
在其他环境中安装Elixir (Installing Elixir in Other Environments)
To install Elixir 3 in environments other than Laravel:
要在Laravel以外的环境中安装Elixir 3:
npm install laravel-elixir --save
开始之前 (Before We Start)
It is useful to know that Elixir assumes that all the source files (.less
, .sass
, .coffee
etc.) are located in ./resources/assets/
. The output files will be copied to the ./public
directory by default:
知道Elixir假定所有源文件( .less
, .sass
, .coffee
等)都位于.sass
, .coffee
./resources/assets/
。 默认情况下,输出文件将被复制到./public
目录:
File type | Base source path | Output path |
---|---|---|
Less | ./resources/assets/less | ./public/css |
Sass | ./resources/assets/sass | ./public/css |
CoffeeScript | ./resources/assets/coffee | ./public/js |
文件类型 | 基本源路径 | 输出路径 |
---|---|---|
减 | ./resources/assets/less | ./public/css |
萨斯 | ./资源/资产/ ass | ./public/css |
CoffeeScript | ./资源/资产/咖啡 | ./public/js |
To create an Elixir task, we need to call the elixir
function in our gulpfile.js
file. This function takes a callback with an object as an argument. This object, called mix
, exposes all the available methods Elixir provides out of the box.
要创建Elixir任务,我们需要在gulpfile.js
文件中调用elixir
函数。 此函数采用以对象为参数的回调。 这个名为mix
对象可以直接使用Elixir提供的所有可用方法。
// ...
elixir(function(mix) {
mix.less('styles.less');
});
If we pass an array of files or a wildcard matching a group of files, all the files will be compiled and concatenated into a single file. The output filename will be app.css
or app.js
for the CSS and JavaScript files respectively.
如果我们通过文件或匹配一组文件的通配符的阵列,所有的文件将被编译并连接成一个文件。 输出的文件名是app.css
或app.js
CSS和JavaScript文件分别。
If we pass a single filename to a certain method, it will be compiled to a file of the same name. For instance, if we pass styles.less
, the output filename will be styles.css
.
如果我们将单个文件名传递给某个方法,它将被编译为同名文件。 例如,如果我们传递styles.less
,则输出文件名将为styles.css
。
However, all these defaults can be customized via a method’s arguments or Elixir’s config
object, which we’ll discuss shortly.
但是,所有这些默认值都可以通过方法的参数或Elixir的config
对象进行自定义,我们将在稍后讨论。
让我们看一些动作 (Let’s See Some Actions)
Elixir provides the basic tasks out of the box, including tasks for compilation, concatenation, minification, running test suites, etc.
Elixir提供了开箱即用的基本任务,包括编译,连接,缩小,运行测试套件等任务。
少编译文件 (Compiling Less Files)
To compile Less files, we need to call the less()
method of the mix
object:
要编译更少的文件,我们需要调用mix
对象的less()
方法:
elixir(function(mix) {
mix.less("styles.less");
});
In the above task, Elixir assumes that styles.less
is located under the resources/assets/less
path. After the compilation, it will save the output file as public/css/styles.css
.
在上述任务中,Elixir假定styles.less
位于resources/assets/less
路径下。 编译后,它将输出文件另存为public/css/styles.css
。
The same goes for .sass
files, we will just need to change the method to sass()
like so:
.sass
文件也是如此,我们只需要像下面这样将方法更改为sass()
:
elixir(function(mix) {
mix.sass("styles.sass");
});
Please note that Elixir assumes Sass files are located in resources/assets/sass
by default.
请注意,Elixir假设默认情况下Sass文件位于resources/assets/sass
。
It is good to know that Elixir will take care of the vendor prefixes in our stylesheets as well, so we will only need to write the standard syntax.
很高兴知道Elixir也会在样式表中处理供应商前缀 ,因此我们只需要编写标准语法即可。
编译CoffeeScript文件 (Compiling CoffeeScript Files)
Okay, now let’s look at how CoffeeScript files are compiled:
好的,现在让我们看看如何编译CoffeeScript文件:
elixir(function(mix) {
mix.coffee(['controllers.coffee', 'services.coffee']);
});
Again, Elixir looks inside resources/assets/coffee/
, compiles all the .coffee
files to JavaScript code and copies them to the public/js
directory as app.js
同样,Elixir会查看resources/assets/coffee/
,将所有.coffee
文件编译为JavaScript代码,并将它们复制为app.js
到public/js
目录中
It is as simple as that!
它是如此简单!
更深入 (Going Deeper)
编译多个文件 (Compiling Multiple Files)
Elixir methods like sass()
, less()
, coffee()
all follow the same prototype. We can either pass a single filename, wildcards, an array of filenames or pass no arguments at all.
诸如sass()
, less()
, coffee()
类的Elixir方法都遵循相同的原型。 我们既可以传递单个文件名,通配符,文件名数组,也可以不传递任何参数。
To compile multiple Less files:
要编译多个Less文件:
elixir(function(mix) {
mix.less([
'base.less',
'styles.less',
'custom.less'
]);
});
As a result, the output will be compiled to public/css/app.css
.
结果,输出将被编译为public/css/app.css
。
The same goes for the other methods like sass()
, coffee()
, etc.
其他方法(如sass()
, coffee()
等coffee()
。
不同的源目录和输出目录 (Different Source and Output Directories)
If our source or output paths differ from the default ones, we can change them to be compatible with our directory layout with three approaches.
如果我们的源路径或输出路径与默认路径不同,则可以通过三种方法更改它们以使其与目录布局兼容。
使用参数 (Using Arguments)
All the methods accept a second argument, which defines the base output directory:
所有方法都接受第二个参数,该参数定义了基本输出目录:
elixir(function(mix) {
mix.less([
'base.less',
'styles.less'
'custom.less'
], 'resources/build/css');
});
As a result, the above task will compile the Less files to resources/build/css/app.css
.
结果,上述任务会将Less文件编译为resources/build/css/app.css
。
To change the output filename, we can include the filename in the argument:
要更改输出文件名 ,我们可以在参数中包含文件名:
elixir(function(mix) {
mix.less([
'base.less',
'styles.less'
'custom.less'
], 'resources/build/css/styles.css');
});
提供完整路径 (Providing Full Paths)
besides customizing the base source and output paths, we can pass the file’s full path prefixed with ./
. If the given path is prefixed with a period, Elixir will assume that the user wants to start at the project’s root directory.
除了自定义基本的源和输出路径外,我们还可以传递以./
为前缀的文件完整路径。 如果给定路径的前缀为句点,则Elixir将假定用户要从项目的根目录开始。
elixir(function(mix) {
mix.less(['./resources/assets/src/less/base.less']);
});
使用配置对象 (Using the Config Object)
The preferred way of customizing the default base source and output paths is to change css.output
and js.output
settings in Elixir’s config
object. We’ll discuss that in the Configuring Elixir section.
自定义默认基本源和输出路径的首选方法是更改Elixir的config
对象中的css.output
和js.output
设置。 我们将在“ 配置Elixir”部分中进行讨论。
串联文件 (Concatenating Files)
Elixir provides several methods for concatenation of stylesheets and JavaScript files, using scripts()
for JavaScript and styles()
for CSS files.
Elixir提供了多种样式表和JavaScript文件的连接方法,JavaScript的scripts()
和CSS文件的styles()
。
elixir(function(mix) {
mix.styles(['base.css', 'customized.css'])
.scripts(['app.js', 'code.js']);
});
连接的基本源和输出路径 (Base Source and Output Paths For Concatenation)
Methods scripts()
and styles()
assume the source files are located in public/js
and resources/assets/css
by default. After compilation, the concatenated version will be copied to public/js
and public/css
:
方法scripts()
和styles()
假定默认情况下源文件位于public/js
和resources/assets/css
。 编译后,串联的版本将被复制到public/js
和public/css
:
Type | Base source path |
---|---|
CSS | ./resources/assets/css |
JavaScript | ./public/js |
类型 | 基本源路径 |
---|---|
CSS | ./资源/资产/ css |
JavaScript | ./public/js |
These two methods accept a second and third argument as well, for the base output path and for the base source path.
对于基本输出路径和基本源路径,这两种方法也接受第二个和第三个参数。
基本输出路径 (Base Output Path)
To change the output path, we can do:
要更改输出路径,我们可以执行以下操作:
elixir(function(mix) {
mix.styles(['base.css', 'customized.css'],
'public/build/css')
.scripts(['app.js', 'code.js'],
'public/build/js')
});
By default, the concatenation output filename is all.css
for CSS and all.js
for JavaScript files. We can change this by including the filename in the argument:
默认情况下,串联的输出文件名是all.css
对CSS和all.js
JavaScript文件。 我们可以通过在参数中包含文件名来更改此设置:
elixir(function(mix) {
mix.styles(['base.css', 'customized.css'],
'public/build/css/application.css')
.scripts(['app.js', 'code.js'],
'public/build/js/application.js');
});
As a result, the output will be saved as application.js
and application.css
.
结果,输出将另存为application.js
和application.css
。
基本源路径 (Base Source Path)
We can change the base source path via the third argument:
我们可以通过第三个参数更改基本源路径:
elixir(function(mix) {
mix.styles(['base.css', 'customized.css'],
'public/build/css/application.css',
'public/css')
.scripts(['app.js', 'code.js'],
'public/build/js/application.js',
'public/js');
});
串联所有文件 (Concatenating All Files)
If we need to concatenate all files in a certain directory, we can use scriptsIn()
and stylesIn()
shortcuts:
如果需要连接某个目录中的所有文件,可以使用scriptsIn()
和stylesIn()
快捷方式:
elixir(function(mix) {
mix.scriptsIn('public/js')
.stylesIn('public/css')
});
玉到剑汇编 (Jade to Blade Compilation)
This Section covers the compilation of Jade files to Blade templates.
本节介绍了将Jade文件编译为Blade模板的过程。
Elixir doesn’t support Jade to Blade compilation out of the box. However, this is possible by using an npm
package called laravel-elixir-jade
. It compiles jade
files into .blade.php
files.
Elixir不支持开箱即用的Jade to Blade编译。 但是,可以使用名为laravel-elixir-jade
的npm
软件包laravel-elixir-jade
。 它将jade
文件编译成.blade.php
文件。
To install laravel-elixir-jade
:
要安装laravel-elixir-jade
:
npm install laravel-elixir-jade@0.1.8 --save-dev
As a result, laravel-elixir-jade
will be installed and added to devDependencies
inside our package.json
file.
结果,将安装laravel-elixir-jade
并将其添加到package.json
文件内的devDependencies
。
Elixir assumes jade
files are located in resources/jade
, and it will copy the output files to resources/views
.
Elixir假定jade
文件位于resources/jade
,并将输出文件复制到resources/views
。
To compile the .jade
files, we use the jade
method:
要编译.jade
文件,我们使用jade
方法:
var elixir = require('laravel-elixir');
require('laravel-elixir-jade');
elixir(function(mix) {
mix.jade({
search: '**/*.jade'
});
});
jade()
expects an object containing all possible options:
jade()
需要一个包含所有可能选项的对象:
{
baseDir: './resources',
blade: true,
dest: '/views/',
pretty: true,
search: '**/*.jade',
src: '/jade/'
}
All options are self explanatory: baseDir
, dest
, search
and src
define the source and output directories.
所有选项都是不言自明的: baseDir
, dest
, search
和src
定义源目录和输出目录。
pretty
instructs Elixir to preserve all the line breaks and indentations. It is true
by default.
pretty
指示Elixir保留所有换行符和缩进。 默认情况下为true
。
By setting blade
to false
, we instruct Elixir to compile to .php
files, instead of .blade.php
files.
通过将blade
设置为false
,我们指示Elixir编译为.php
文件,而不是.blade.php
文件。
There’s also a helpful collection of jade mixins developed by @franzose.
还有一个有用的集合,由@franzose开发的玉混合 。
文件版本控制 (File Versioning)
Elixir also provides a versioning feature, which appends a unique hash to the file names. This will prevent the browser from loading the asset files from its cache. In the past, we had to append a version number to the file name in the form of a query string(?v=2), but not anymore!
Elixir还提供了版本控制功能,该功能将唯一的哈希值附加到文件名中。 这将阻止浏览器从其缓存加载资产文件。 过去,我们必须以查询字符串(?v = 2)的形式在文件名后附加版本号,但是现在不再可用!
By using Elixir’s version()
method, things are a whole lot easier now. The only thing we need to pass to the version()
method is the full path to the file:
通过使用Elixir的version()
方法,现在事情变得容易得多。 我们唯一需要传递给version()
方法的是文件的完整路径:
elixir(function(mix) {
mix.version("./public/build/js/all.css");
});
// We can also pass an array to the version method.
elixir(function(mix) {
mix.version(['./public/build/js/all.js',
'./public/build/js/code.js']);
});
As a result, the file name will become something like: styles.all-16d570a7.css
. Remembering this name is another problem, though. The good thing is, we don’t have to deal with this on our own, as Laravel has already taken care of this for us.
结果,文件名将变为: styles.all-16d570a7.css
。 记住这个名字是另一个问题。 好处是,我们不必自己处理这个问题,因为Laravel已经为我们解决了这个问题。
Across our Blade views, we can use the elixir()
helper function to refer to the hashed file:
在我们的Blade视图中,我们可以使用elixir()
帮助函数来引用哈希文件:
@block('stylesheets')
<link rel="stylesheet" type="text/css" href="{{ elixir("styles.all.css") }}">
@endblock
We only need to pass the original filename, as we would do without hashing.
我们只需要传递原始文件名,就像不进行哈希处理一样。
Since Elixir 3.0, we need to provide the full path to the file. This means we can even use version()
for all the other files inside our project.
从Elixir 3.0开始,我们需要提供文件的完整路径。 这意味着我们甚至可以将version()
用于项目中的所有其他文件。
配置Elixir (Configuring Elixir)
One of the good things about Elixir is that it is configurable via its config object. All the available options exist in node_modules/laravel-elixir/Config.js
. If we open the file, we’ll see that there’s a config
object containing all the settings which define Elixir’s behavior. If we’re using Elixir inside a Laravel environment, we won’t have to change most of these options, as they are already compatible with Laravel’s directory layout.
关于Elixir的优点之一是可以通过其config对象进行配置。 所有可用选项都存在于node_modules/laravel-elixir/Config.js
。 如果打开文件,我们将看到有一个config
对象,其中包含定义Elixir行为的所有设置。 如果我们在Laravel环境中使用Elixir,则无需更改大多数选项,因为它们已经与Laravel的目录布局兼容。
Settings are grouped inside nested objects (according to their usage) within the config
object. For example, settings related to .css
files are defined within the css
object:
设置分组在config
对象内的嵌套对象内(根据其用途)。 例如,与.css
文件相关的设置在css
对象中定义:
// ...
css: {
folder: 'css',
outputFolder: 'css',
autoprefix: {
enabled: true,
// https://www.npmjs.com/package/gulp-autoprefixer#api
options: {
browsers: ['last 2 versions'],
cascade: false
}
}
}
// ...
To see all the available options, please check the Config.js
file.
要查看所有可用选项,请检查Config.js
文件。
To override the default settings, we can access them via the elixir.config
object in gulpfile.js
:
要覆盖默认设置,我们可以通过访问这些elixir.config
在对象gulpfile.js
:
//...
elixir.config.assetsPath = 'resources/assets/src/';
To configure a group of options, we can create an elixir.json
file in our project root directory, and declare an object containing the settings we need to override:
要配置一组选项,我们可以在项目根目录中创建一个elixir.json
文件,并声明一个包含我们需要覆盖的设置的对象:
elixir.json
elixir.json
{
"assetsPath": "resources/assets/src",
"css": {
"outputFolder": "stylesheets"
}
}
Anything we add in this object will override the default settings in the main config
object.
我们在此对象中添加的所有内容都将覆盖主config
对象中的默认设置。
真实的例子 (A Real World Example)
Let’s see the power of Elixir in a real world example. We’ll be managing our assets in a Laravel/Angular project.
让我们在一个真实的示例中查看Elixir的强大功能。 我们将在Laravel / Angular项目中管理资产。
资产目录布局 (Assets Directory Layout)
Imagine that our resources
directory layout is:
假设我们的resources
目录布局为:
.
.
.
resources
├── assets
│ └── src
│ ├── coffee
│ │ ├── app.coffee
│ │ ├── base.coffee
│ │ ├── controllers.coffee
│ │ ├── directives.coffee
│ │ └── services.coffee
│ └── less
│ ├── base.less
│ ├── bootstrap
│ ├── footer.less
│ ├── header.less
│ ├── styles.less
│ ├── typography.less
│ └── Bootstrap
│ └── ...
├── jade
│ ├── dashboard.jade
│ ├── incs
│ │ ├── footer.jade
│ │ └── navbar.jade
│ ├── layouts
│ │ ├── base.jade
│ │ └── master.jade
│ ├── list.jade
│ └── login.jade
.
.
.
All the asset files are located in ./resources/assets/src
.
所有资产文件都位于./resources/assets/src
。
Please note that in the less
directory, we only need to compile styles.less
, because all other .less
files are already imported into it:
请注意,在less
目录中,我们只需要编译styles.less
,因为所有其他.less
文件已经被导入到其中:
/* resources/assets/src/less/styles.less */
@import "bootstrap/bootstrap.less";
@import "base.less";
@import "typography.less";
@import "header.less";
@import "footer.less";
The reason why Bootstrap mixins
and variables are imported into styles.less
is to use the existing mixins
and variables that Bootstrap provides. The output will be a customized Bootstrap file. However, it’s just one way of writing stylesheets which may differ from project to project.
将Bootstrap mixins
和变量导入到styles.less
是使用Bootstrap提供的现有mixins
和变量。 输出将是自定义的Bootstrap文件。 但是,这只是编写样式表的一种方法,这可能因项目而异。
Let’s list the steps we have to take for our asset compilation:
让我们列出资产汇编所必须采取的步骤:
Step 1: Load Elixir and other required modules into our
gulpfile.js
步骤1:将 Elixir和其他所需模块加载到我们的
gulpfile.js
Step 2: Configure Elixir using
elixir.json
步骤2:使用
elixir.json
配置ElixirStep 3: Compile the
styles.less
file topublic/build/styles.css
步骤3:将
styles.less
文件编译为public/build/styles.css
Step 4: Compile all the
.coffee
files to JavaScript code and save the output aspublic/build/js/app.js
.步骤4:将所有
.coffee
文件编译为JavaScript代码,并将输出保存为public/build/js/app.js
Step 5: Compile the
.jade
files to.blade.php
templates, so they can be rendered by Laravel’s blade engine.步骤5:将
.jade
文件编译为.blade.php
模板,以便可以使用Laravel的刀片引擎来呈现它们。Step 6: Versioning the files for cache busting.
第6步:对文件进行版本控制以清除缓存。
编写任务 (Writing the Tasks)
The beauty of Elixir is that whatever we do, it is defined in gulpfile.js
. That means we can mix Elixir’s syntax with that of Gulp’s, especially when we are extending Elixir.
Elixir的gulpfile.js
在于,无论我们做什么,它都在gulpfile.js
定义。 这意味着我们可以将Elixir的语法与Gulp的语法混合使用,尤其是在扩展Elixir时。
步骤1:加载所需的模块 (Step 1: Loading Required Modules)
In our gulpfile.js
, we write the following code:
在gulpfile.js
,我们编写以下代码:
var elixir = require('laravel-elixir');
require('laravel-elixir-jade');
First, we loaded Elixir
and put it in the elixir
variable for later reference. Then, we loaded the laravel-elixir-jade
package for compiling our jade
files.
首先,我们加载了Elixir
,并将其放入elixir
变量中以供以后参考。 然后,我们加载了laravel-elixir-jade
软件包来编译我们的jade
文件。
步骤2:设定 (Step 2: Configuration)
Since our source files are located under a path different than the default base path, we need to customize some defaults.
由于我们的源文件位于与默认基本路径不同的路径下,因此我们需要自定义一些默认值。
To do this, we create a file in our project root directory named elixir.json
with the following content:
为此,我们在项目根目录中创建一个名为elixir.json
,其内容如下:
{
assetsPath: 'resources/assets/src',
publicPath: 'public/build'
}
步骤3:编译较少的文件 (Step 3: Compiling Less Files)
elixir(function(mix) {
mix.less('styles.less');
});
步骤4:编译CoffeeScript文件 (Step 4: Compiling CoffeeScript Files)
elixir(function(mix) {
mix.coffee([
'app.coffee',
'base.coffee',
'controllers.coffee',
'directives.coffee',
'services.coffee'
]);
});
The above task will compile all .coffee
files to the public/build/js
directory as app.js
.
上面的任务会将所有.coffee
文件作为app.js
编译到public/build/js
目录中。
步骤5:编译Jade文件 (Step 5: Compiling Jade Files)
In our resources/jade
directory, we have two sub directories, incs
and layouts
, which contain the layout files and a few partials for the navigation bar and the footer section. We also have some files for the views, including the login page, dashboard page and a list.
在resources/jade
目录中,我们有两个子目录incs
和layouts
,它们包含布局文件以及导航栏和页脚部分的一些部分。 我们还为视图提供了一些文件,包括登录页面,仪表板页面和列表。
To compile the .jade
files, we use the jade
method like so:
要编译.jade
文件,我们使用jade
方法,如下所示:
elixir(function(mix) {
mix.jade({
search: '**/*.jade'
});
});
The above task will look into ./resources/jade
directory and sub directories, and compile all the .jade
files to .blade.php
files.
上面的任务将查看./resources/jade
目录和子目录,并将所有.jade
文件编译为.blade.php
文件。
步骤6:版本化文件 (Step 6: Versioning the Files)
In our final step, let’s version the files to take care of the cache busting:
在最后一步中,让我们对文件进行版本控制以解决缓存破坏问题:
elixir(function(mix) {
mix.version([
'./public/css/styles.css',
'./public/js/app.js'
]);
});
And that’s all there is to it. Our gulpfile.js
is ready!
这就是全部。 我们的gulpfile.js
已经准备好!
This is the complete code:
这是完整的代码:
var elixir = require('laravel-elixir');
require('laravel-elixir-jade');
elixir(function(mix) {
mix.less('styles.less')
.coffee([
'app.coffee',
'base.coffee',
'controllers.coffee',
'directives.coffee',
'services.coffee'
]);
.jade({
search: '**/*.jade'
})
.version([
'build/css/styles.css',
'build/js/app.js'
]);
});
Elixir allows method chaining as shown in the final code above.
Elixir允许方法链接 ,如上面的最终代码所示。
运行任务 (Running The Tasks)
To run the tasks, we just need to call gulp
:
要运行任务,我们只需要调用gulp
:
gulp
This will run all the tasks registered with Elixir.
这将运行在Elixir中注册的所有任务。
We can also watch
files for changes to automate running of the tasks, so we won’t have to run gulp
whenever we make a change in a file:
我们还可以watch
文件中的更改以自动执行任务,因此,只要在文件中进行更改,就不必运行gulp
:
gulp watch
运行个人任务 (Running Individual Tasks)
Whenever we add a task in Elixir, behind the scenes, a task of the same name is registered with Gulp. As an example, if we use the Elixir’s less()
method, the Gulp task name would be less
. This means we will be able to run the tasks individually, as we normally do with Gulp.
每当我们在Elixir中添加任务时,都会在后台向Gulp注册同名任务。 例如,如果我们使用Elixir的less()
方法,则Gulp任务名称将为less
。 这意味着我们将能够像通常使用Gulp一样单独运行任务。
gulp less
缩小呢? (What About Minification?)
One common use of task runners like Grunt or Gulp is to minify files content to make them smaller and save bandwidth. By using Elixir, the only thing we need to do is to send the --production
option when running the gulp
command:
任务运行程序(例如Grunt或Gulp)的一种常见用法是最小化文件内容以使其更小并节省带宽。 通过使用Elixir,我们唯一需要做的就是在运行gulp
命令时发送--production
选项:
gulp --production
进阶 (Going Advanced)
自定义任务和扩展 (Custom Tasks and Extensions)
Elixir provides the basic tasks out of the box. In most cases, these are more than enough. However, we might encounter situations when existing tasks can’t handle specific operations, which means it’s time to extend Elixir!
Elixir开箱即用提供基本任务。 在大多数情况下,这些绰绰有余。 但是,我们可能会遇到现有任务无法处理特定操作的情况,这意味着该扩展Elixir了!
For this purpose, Elixir exposes the extend
method. The extend
method accepts two parameters. The first one is the name of the custom task and the second is a callback, in which we write a Gulp task.
为此,Elixir公开了extend
方法。 extend
方法接受两个参数。 第一个是自定义任务的名称,第二个是回调,我们在其中编写了Gulp任务。
To keep things as bare bones as possible, let’s create a basic task which prints a text message to the console:
为了使事情尽可能的简单,让我们创建一个基本任务,该任务将文本消息打印到控制台:
var elixir = require('laravel-elixir'),
gulp = require('gulp'),
shell = require('gulp-shell');
Elixir.extend('saysHi', function(message) {
new Elixir.Task('saysHi', function() {
return gulp.src('').pipe(shell('Hi, I am a custom task!'));
})
.watch('./app/**');
});
watch()
registers a watcher. As a result, when we edit any files matching the regular expression, the task is run.
watch()
注册一个观察者。 结果,当我们编辑任何与正则表达式匹配的文件时,任务将运行。
We can put this block at the top of the gulpfile.js
file or put it in an external file, and import it in our gulpfile.js
:
我们可以将此块放在gulpfile.js
文件的顶部,或将其放在外部文件中,然后将其导入到gulpfile.js
:
var elixir = require('laravel-elixir');
require('./elixir-extensions');
The custom task is now accessible via the mix
object:
现在可以通过mix
对象访问自定义任务:
elixir(function(mix) {
mix.saysHi();
});
Now each time we run gulp saysHi
, the following text will be printed on the screen:
现在,每次我们运行gulp saysHi
,屏幕上都会打印以下文本:
gulp custom Task
[01:10:57] Using gulpfile ~/www/sites/elixir/gulpfile.js
[01:10:57] Starting 'saysHi'...
Hi, I am a custom task!
[01:10:57] Finished 'saysHi' after 370 μs
在刀片模板中使用资产 (Using the Assets in the Blade Templates)
To use the compiled files, we use the elixir
helper function, which is available across all the views.
要使用编译后的文件,我们使用elixir
帮助程序功能,该功能在所有视图中都可用。
@block('scripts')
<link rel="stylesheet" type="text/css" href="{{ elixir('css/styles.all.css') }}">
@stop
@block('styles')
<script src="{{ elixir('css/scripts.all.js') }}">
@stop
Now we have peace of mind about versions and can focus on the project!
现在我们对版本感到放心,可以专注于项目!
结语 (Wrapping up)
Elixir is a wrapper around Gulp.js providing a wide variety of functionality to help us with our assets compilation. In addition to Gulp’s standard tasks, it also provides several methods for versioning, testing, and copying the files, just to name a few. Elixir is very flexible. We can configure it, extend it and even combine it with Gulp’s standard syntax.
Elixir是Gulp.js的包装器,提供了多种功能来帮助我们进行资产编译。 除了Gulp的标准任务外,它还提供了几种版本控制,测试和复制文件的方法,仅举几例。 长生不老药非常灵活。 我们可以对其进行配置,扩展,甚至将其与Gulp的标准语法结合起来。
The main focus of this tutorial was on assets compilation. However, there are still several of Elixir’s functionalities which we haven’t covered in this tutorial like tasks for copying files, testing, and even running Laravel Artisan commands. To find out more, please refer to Elixir’s official documentation.
本教程的主要重点是资产编译。 但是,我们仍然没有在本教程中介绍Elixir的一些功能,例如复制文件,测试甚至运行Laravel Artisan命令的任务。 要了解更多信息,请参阅Elixir的官方文档 。
For those who always prefer PHP solutions for these sort of tasks and don’t like to switch context between JavaScript and PHP syntax sequentially, check out our article on using PHP solutions for a front end workflow without using Node.
对于那些始终喜欢使用PHP解决方案执行此类任务并且不喜欢顺序在JavaScript和PHP语法之间切换上下文的用户,请查看我们有关将PHP解决方案用于前端工作流而不使用Node的文章。
Thanks for reading. Leave your questions and comments below!
谢谢阅读。 在下面留下您的问题和评论!
翻译自: https://www.sitepoint.com/meet-elixir-the-laravel-way-of-compiling-assets/
elixir 教程
elixir 教程_认识Elixir,Laravel编译资产的方式相关推荐
- Elixir元编程-第三章 编译时代码生成技术进阶
Elixir元编程-第三章 编译时代码生成技术进阶 注:本章内容来自 Metaprogramming Elixir 一书,写的非常好,强烈推荐.内容不是原文照翻,部分文字采取意译,主要内容都基本保留, ...
- 只能输入字母的c语言程序设计教程课后答案,c语言程序设计基础教程_习题答案20120319...
<c语言程序设计基础教程_习题答案20120319>由会员分享,可在线阅读,更多相关<c语言程序设计基础教程_习题答案20120319(54页珍藏版)>请在技术文库上搜索. 1 ...
- Hadoop安装教程_单机/伪分布式配置_Hadoop2.6.0/Ubuntu14.04
给力星 追逐内心的平和 首页 笔记 搜藏 代码 音乐 关于 Hadoop安装教程_单机/伪分布式配置_Hadoop2.6.0/Ubuntu14.04 2014-08-09 (updated: 2016 ...
- 02Hadoop安装教程_单机、伪分布式配置
02 Hadoop2.7 安装教程_单机.伪分布式配置 文章目录 **02** Hadoop2.7 安装教程_单机.伪分布式配置 **1.hadoop基础知识** 1.1 hadoop是什么? 1.2 ...
- 计算机软件cd全称,cd刻录(刻录高音质cd完美教程_计算机软件及应用_IT/计算机_专业资料)...
cd刻录(刻录高音质cd完美教程_计算机软件及应用_IT/计算机_专业资料),哪吒游戏网给大家带来详细的cd刻录(刻录高音质cd完美教程_计算机软件及应用_IT/计算机_专业资料)介绍,大家可以阅读一 ...
- Cg教程_可编程实时图形权威指南(扫描清晰版)+部分unity shader 知识
Cg教程_可编程实时图形权威指南(扫描清晰版) .pdf (34.5 MB, 下载次数: 239) Shader Model(在 3D 图形领域常被简称SM)就是"优化渲染引擎模式&qu ...
- 只能输入字母的c语言程序设计教程课后答案,c语言程序设计基础教程_习题答案解析.doc...
c语言程序设计基础教程_习题答案解析.doc 习题答案 第1章 填空题 应用程序ONEFUNC.C中只有一个函数,这个函数的名称是__main . 一个函数由__函数头__和__函数体__两部分组成. ...
- 一段c语言的自加程序输出xyz,c语言程序设计基础教程_习题解答2012.doc
c语言程序设计基础教程_习题解答2012 C语言程序设计基础教程_习题答案2012 习题答案 第1章 填空题 应用程序ONEFUNCC中只有一个函数这个函数的名称是__main 一个函数由__函数头_ ...
- Vue教程_基础(一)
目录 章节 地址 Vue教程_tips https://blog.csdn.net/weixin_46349544/article/details/124082287 Vue教程_基础(一) http ...
最新文章
- 阅读类APP体验好不好?可从这些指标衡量
- DB2数据库安全的12条军规
- Jdk14 都要出了,Jdk9 的新特性还不了解一下?
- 风控模型中的变量替换
- Zoom 5.1.2及旧版本在 Win7 上的 DLL 劫持漏洞分析
- POJ1039+几何+直线于线段相交
- Apache ShenYu 网关正式支持 Dubbo3 服务代理
- 华为云ECS服务器中通过docker部署sentinel-dashboard
- 幂乘法求最大特征值和特征向量
- 蓝光光盘的区域位置代码
- 【IT之路】微信小程序之美化
- 【Shader】基于图像的光照(Image Based Lighting,IBL)
- delphi DCC32命令行方式编译delphi工程源码
- 设计模式之设计模式入门
- Advanced Practices:一款新型恶意监测工具的改进过程
- java系统随机产生10道加法运算,用户进行答题,答对一道题加10分,答错不加分
- 计算机sci有哪些AJCHE,最新SCI目录(大类加小类,齐全).xls
- 北斗导航 | 航空所需导航性能与完好性监测(理论):PBN、NPA、APV、CAT、RNAV、XPL、XAL
- 【BIM入门实战】Revit中的墙体层次以及常见问题解答
- 自考2020计算机系统结构题,2020年度10月自考02325计算机系统结构试卷及答案解释.doc...