关于Remoting信道的通信的问题
现在把程序发上来,希望大家能为我解决问题。
远程类:
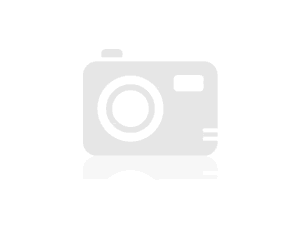
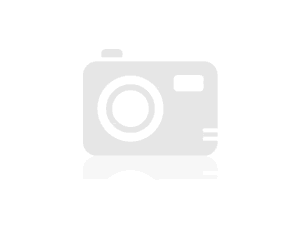
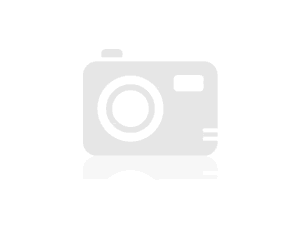
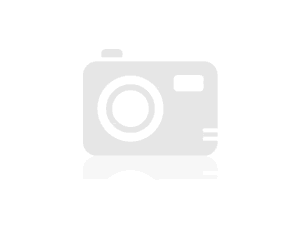
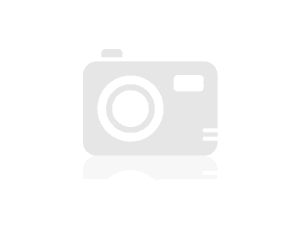
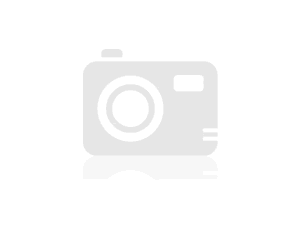





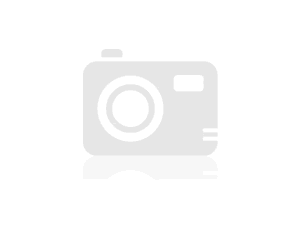




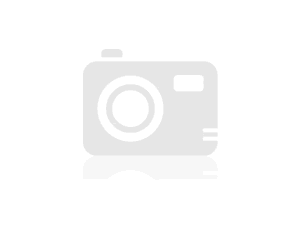





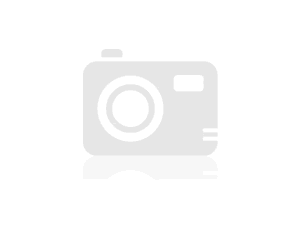



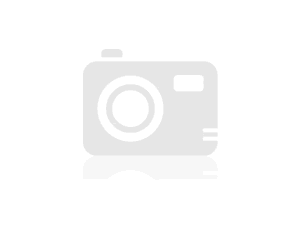








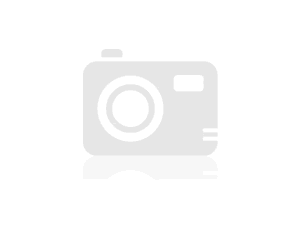




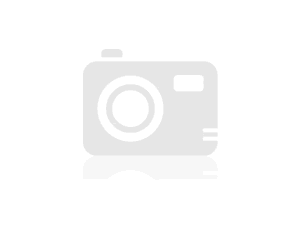







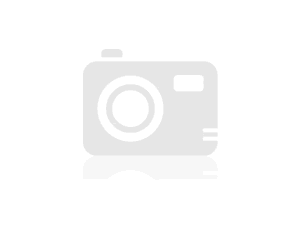






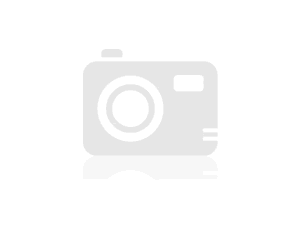





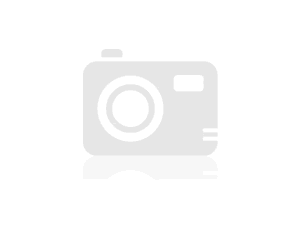






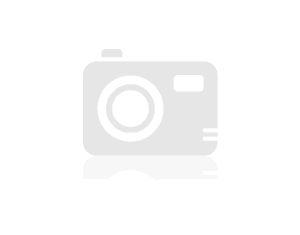
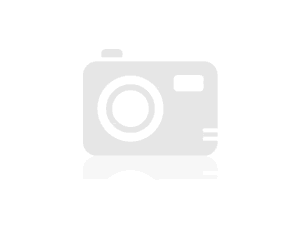
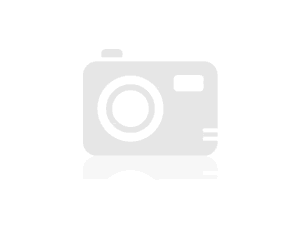
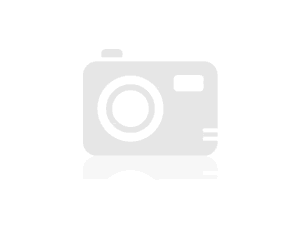
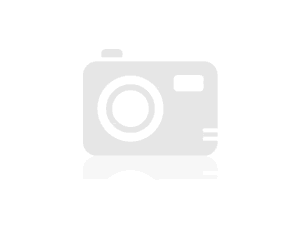
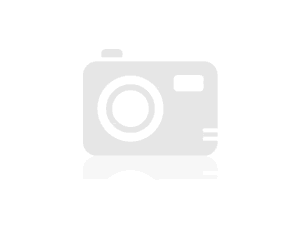
服务端:
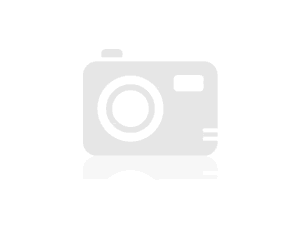
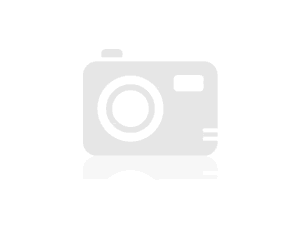
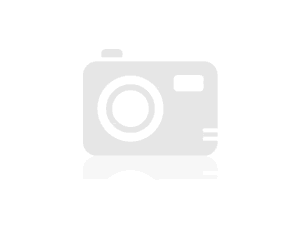
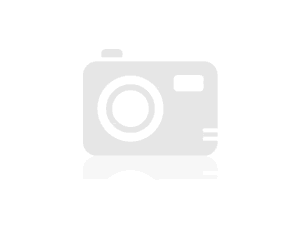
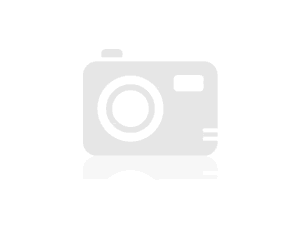
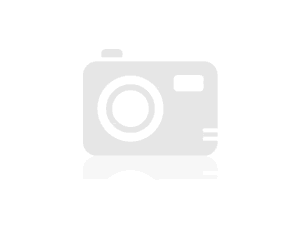
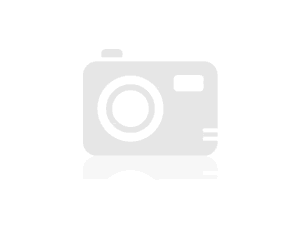
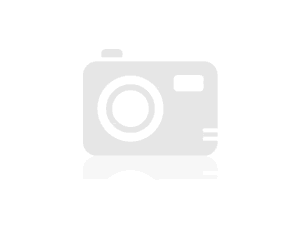
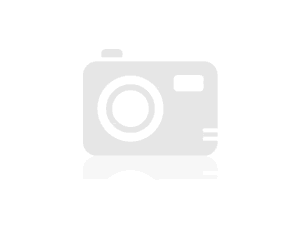
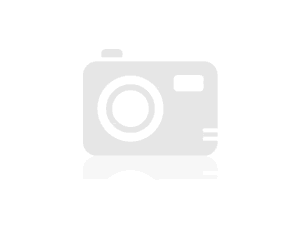
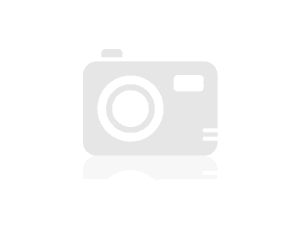
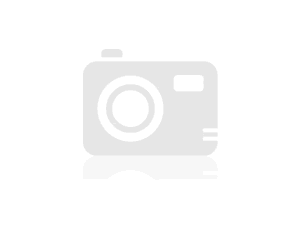



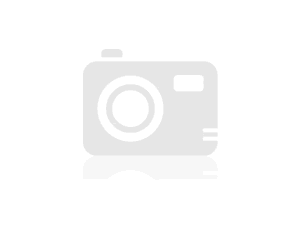



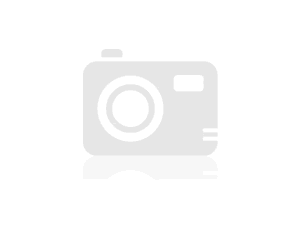




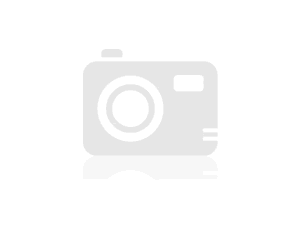














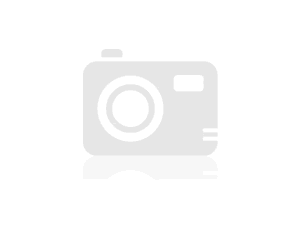

























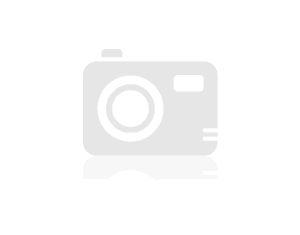
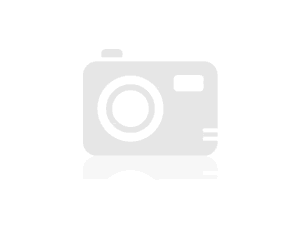
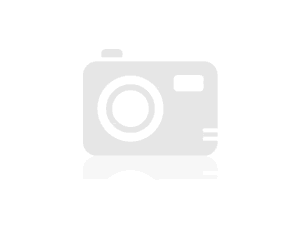
Annoncer:
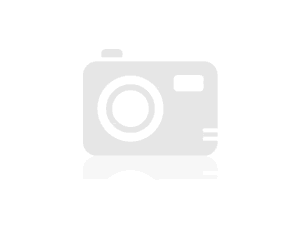
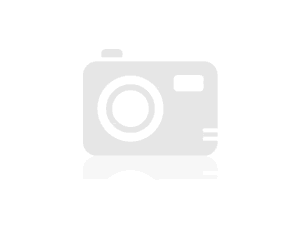
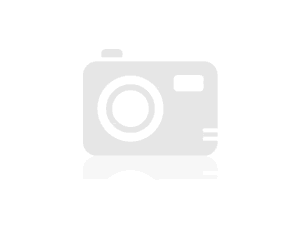
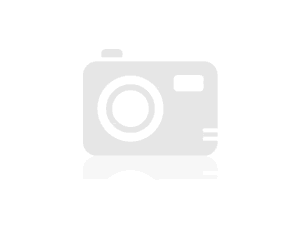
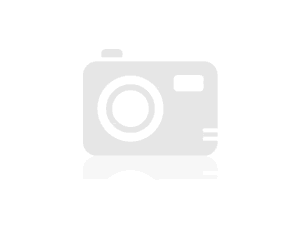
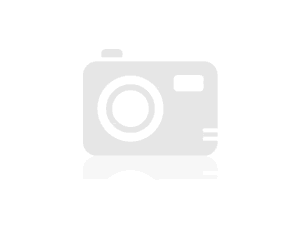
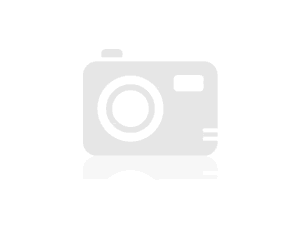
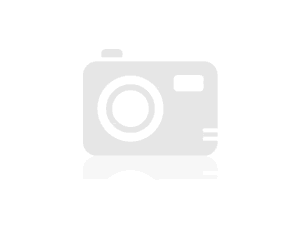
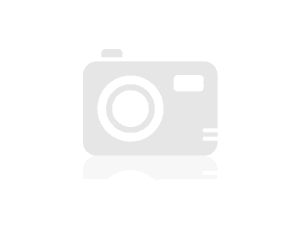
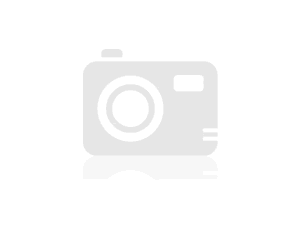



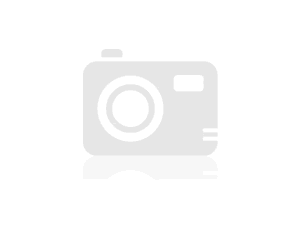





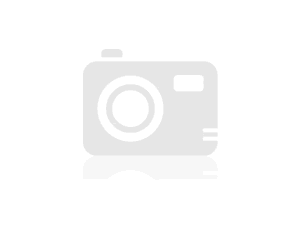


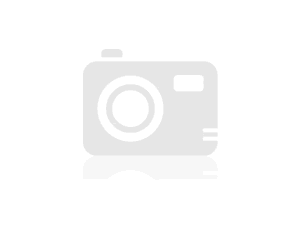










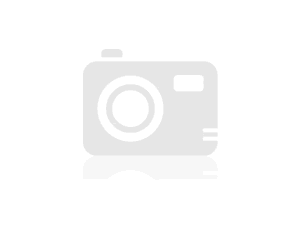



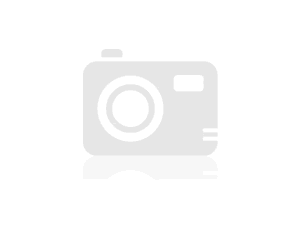









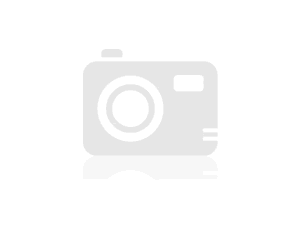








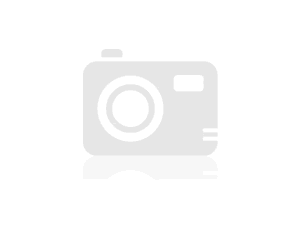






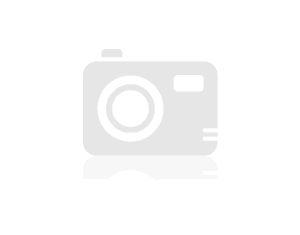
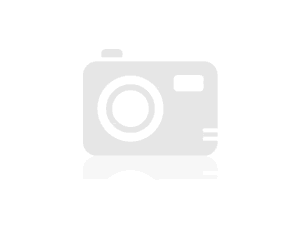
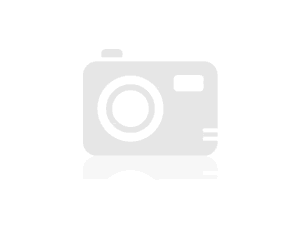
客户端:
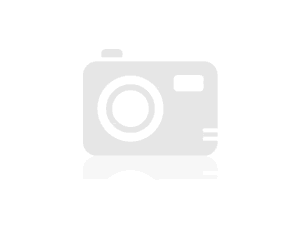
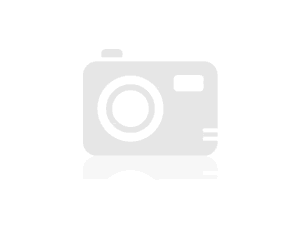
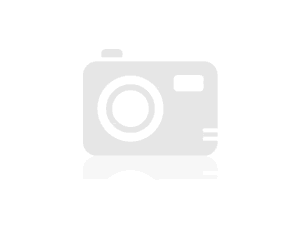
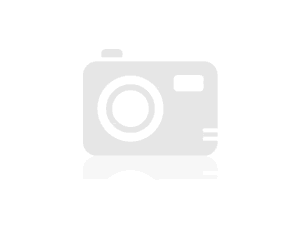
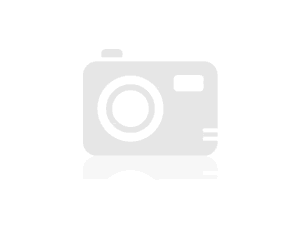
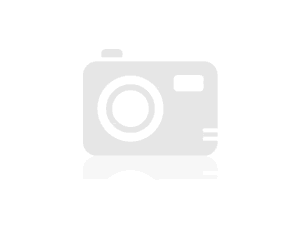
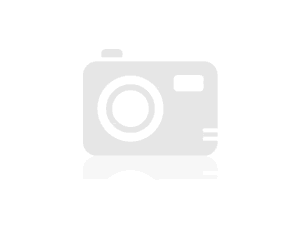
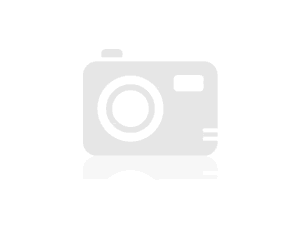
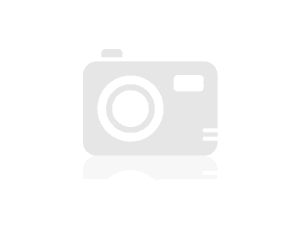
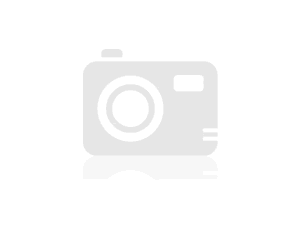



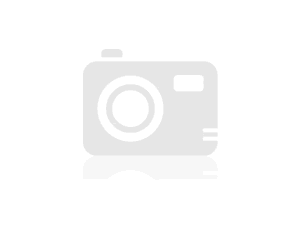




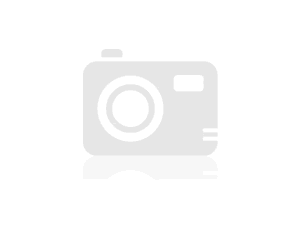





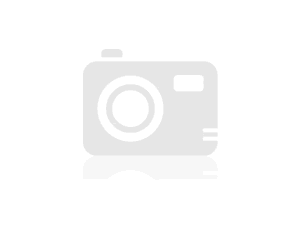






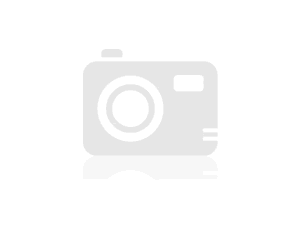



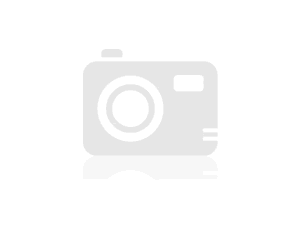















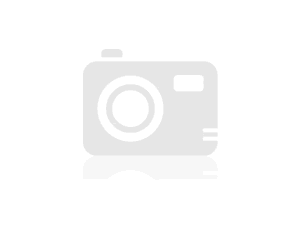










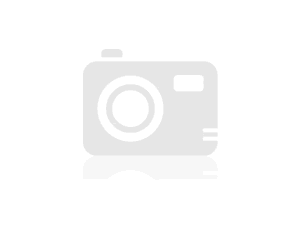






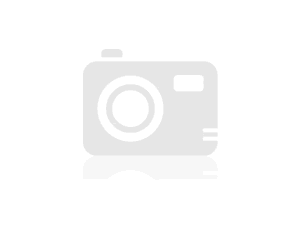





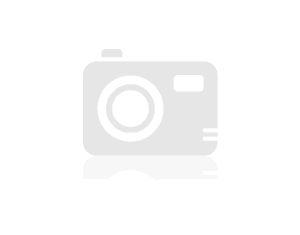
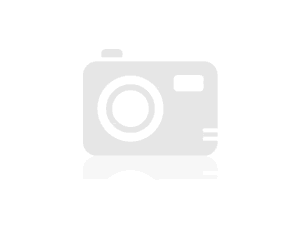
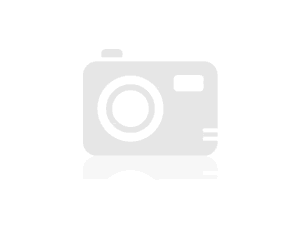
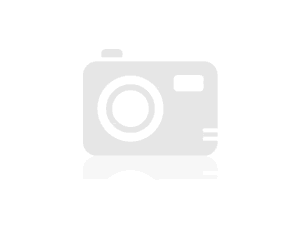
在客户端如果用配置文件就报错,说<channels>附进有错误。
客户端配置文件:
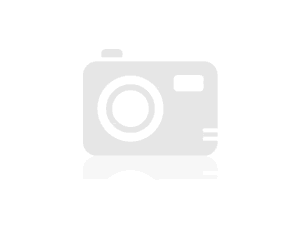
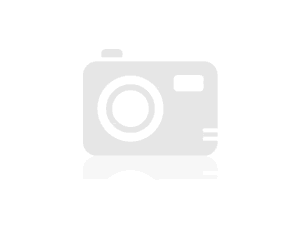
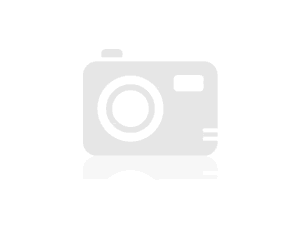
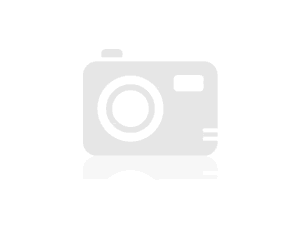
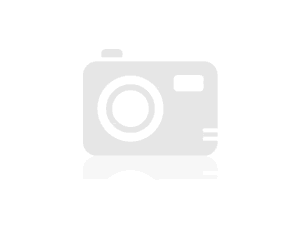
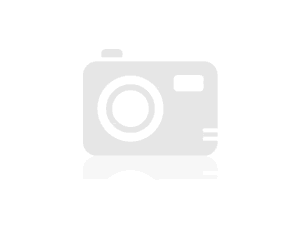
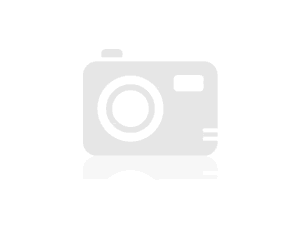
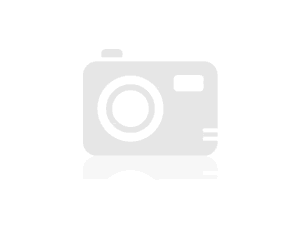
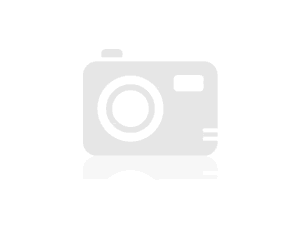
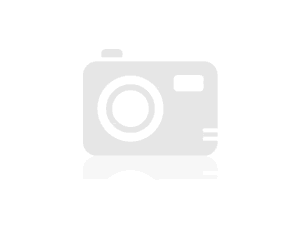
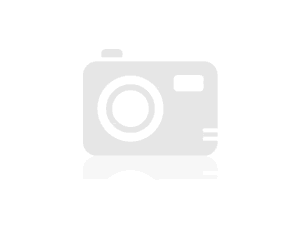
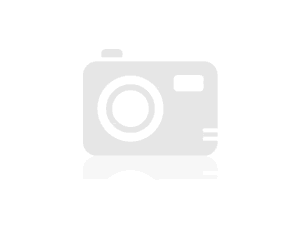
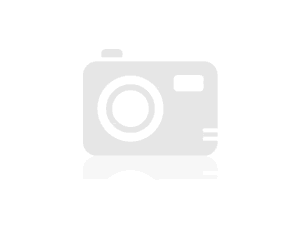
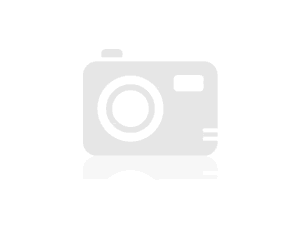
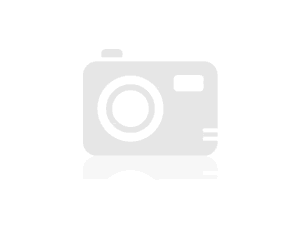
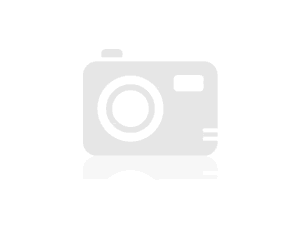
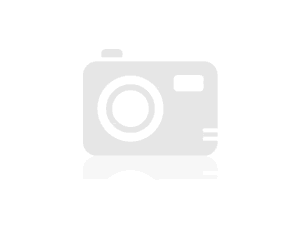
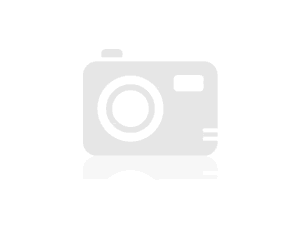
转载于:https://www.cnblogs.com/liuwenjun830/archive/2006/04/19/378733.html
关于Remoting信道的通信的问题相关推荐
- [Remoting]dotNet Framework升级后Remoting信道使用的安全问题
[Remoting]dotNet Framework升级后Remoting信道使用的安全问题<?xml:namespace prefix = o ns = "urn:schemas-m ...
- 【U8】读取数据源出现未知错误:remoting信道异常
U8登录提示"找不到请求的服务",再次点击数据源的位置提示"读取数据源出现未知错误:remoting信道异常" 解决: 方法一: 1.打开系统管理,初始化数据库 ...
- 【计算机网络】物理层 : 数据通信 ( 数据通信模型 | 信源 | 信宿 | 信道 | 通信方式 | 单工 | 半双工 | 全双工 | 数据传输方式 | 串行 | 并行 )
文章目录 一.数据通信模型示例 二.数据通信模型 三.数据通信模型 分类 四.数据通信 术语 五.三种通信方式 六.数据传输方式 一.数据通信模型示例 数据通信模型 示例 : ① 通信场景 : 两台计 ...
- 信道分类、信道复用技术、CSMA/CD 协议、PPP 协议、MAC 地址、局域网、以太网、交换机、虚拟局域网
1.信道分类 1.1 广播信道 一对多通信,一个节点发送的数据能够被广播信道上所有的节点接收到. 所有的节点都在同一个广播信道上发送数据,因此需要有专门的控制方法进行协调,避免发生冲突(冲突也叫碰撞) ...
- 【旧文章搬运】无Device的驱动如何通信
原文发表于百度空间,2009-07-14 ========================================================================== 标准的驱 ...
- 计算机网络(九)-物理层(补充)-傅里叶变换-信道复用
一.几个术语 1.码元------在使用时间域(时域)的波形表示数字信号时,代表不同离散数值的基本波形. 2.调制------把数字信号转换为模拟信号的过程. 3.解调------把模拟信号转换为数字 ...
- 密码学技术如何选型?终探量子计算通信的安全模型
经典密码学技术的应用寿命将至?量子计算何以破解现有隐私保护方案?量子通信对隐私保护方案设计有何启示?如何有效应对量子计算通信技术给隐私保护带来的挑战? 这里,我们将进入安全模型分析三部曲的最终章,跳出 ...
- 密码学技术如何选型?终探量子计算通信的安全模型|第6论
作者:严强 来源:微众银行区块链 经典密码学技术的应用寿命将至?量子计算何以破解现有隐私保护方案?量子通信对隐私保护方案设计有何启示?如何有效应对量子计算通信技术给隐私保护带来的挑战? 这里,我们 ...
- m利用SIMILINK仿真模块实现多径信道的动态仿真模拟
目录 1.算法描述 2.仿真效果预览 3.MATLAB部分代码预览 4.完整MATLAB程序 1.算法描述 在过去的几十年里,无线通信技术得到了迅猛的发展和广泛的应用.第三代.第四代等移动通信系统给人 ...
最新文章
- python文件指针放在文件的开头_将文件指针倒带到上一个lin的开头
- linux 设计一个程序,要求打开文件 pass 所有者,第二章 Linux 文件操作
- Kaggle 入门练习 -- Titanic
- pycharm同时注释多行代码快捷键
- Spring新注解详解
- 根据前一个元素的check状态决定其他元素disable
- redis和zookeeper安装教程并配置开机自启
- 列表与元组——Python基础语法
- MySQL数据类型详解
- 程序员必备智力题集锦 (典藏版)
- 【20保研】四川大学网络空间安全学院2019年优秀大学生暑期夏令营招生简章
- Spring学习资料
- 接口测试通用测试用例
- kylin 维度优化,Aggregation Group,Joint,Hierachy,Mandatory等解析
- 2021阿里淘系工程师推荐书单
- postfix邮箱服务器安装和配置
- “博客之星”年度评选
- 心知天气Android开发,H5 实现天气效果(心知天气插件)
- 中国最美丽的地方排行榜国家地理
- python --003--流程控制while,for