使用OpenCV python模块读取图像并将其另存为灰度系统
In Python, we can use an OpenCV library named cv2. Python does not come with cv2, so we need to install it separately.
在Python中,我们可以使用名为cv2的OpenCV库 。 Python没有随附cv2 ,因此我们需要单独安装它。
For Windows:
对于Windows:
pip install opencv-python
For Linux:
对于Linux:
sudo apt-get install python-opencv
In the below given program, we are using following three functions:
在下面给出的程序中,我们使用以下三个功能:
imread():
imread():
It takes an absolute path/relative path of your image file as an argument and returns its corresponding image matrix.
它以图像文件的绝对路径/相对路径作为参数,并返回其对应的图像矩阵。
imshow():
imshow():
It takes the window name and image matrix as an argument in order to display an image in the display window with a specified window name.
它以窗口名称和图像矩阵为参数,以便在具有指定窗口名称的显示窗口中显示图像。
cv2.cvtcolor():
cv2.cvtcolor():
It takes image matrix and a flag for changing color-space from one color space to another(in this case we are using BGR2GRAY color-space conversion) and returns the newly converted image matrix.
它需要图像矩阵和用于将颜色空间从一种颜色空间更改为另一种颜色的标志(在这种情况下,我们使用BGR2GRAY颜色空间转换),并返回新转换的图像矩阵。
Imwrite() :
Imwrite():
It takes an absolute path/relative path (of the desired location where you want to save a modified image) and image matrix as an argument.
它采用绝对路径/相对路径(要保存修改后图像的所需位置)和图像矩阵作为参数。
使用OpenCV python模块读取图像并将其另存为灰度系统的Python代码 (Python code to read an image and save it as grayscale system using OpenCV python module)
# open-cv library is installed as cv2 in python
# import cv2 library into this program
import cv2
# read an image using imread() function of cv2
# we have to pass only the path of the image
img = cv2.imread(r'C:/Users/user/Desktop/pic6.jpg')
# displaying the image using imshow() function of cv2
# In this : 1st argument is name of the frame
# 2nd argument is the image matrix
cv2.imshow('original image',img)
# converting the colourfull image into grayscale image
# using cv2.COLOR_BGR2GRAY argument of
# the cvtColor() function of cv2
# in this :
# ist argument is the image matrix
# 2nd argument is the attribute
gray_img = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
# save the image at specified location
cv2.imwrite(r"image\gray_img.jpg",gray_img)
Output
输出量
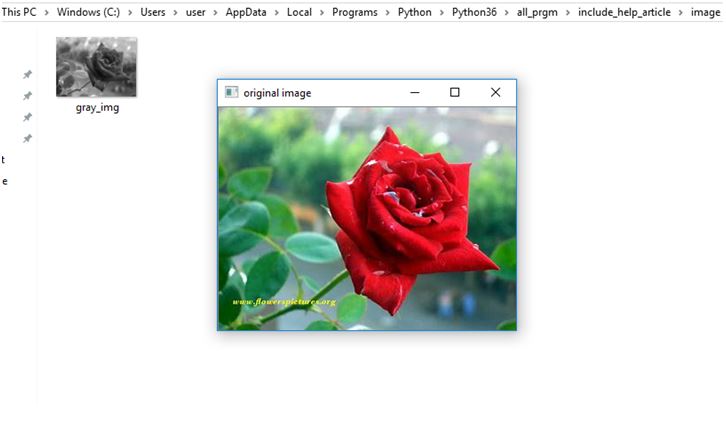
翻译自: https://www.includehelp.com/python/read-an-image-and-save-it-as-grayscale-system-using-opencv-python-module.aspx
使用OpenCV python模块读取图像并将其另存为灰度系统相关推荐
- pillow支持python 2和python 3_python3读取图像并可视化的方法(PIL/Pillow、opencv/cv2)...
原图: 使用TensorFlow做图像处理的时候,会对图像进行一些可视化的操作.下面,就来列举一些我知道的图像读取并可视化的方法. 1. Pillow模块 1.1 Pillow模块的前生 Pillow ...
- 如何把OpenCV Python获取的图像传递到C层处理
原文:https://blog.csdn.net/yushulx/article/details/52788051 用OpenCV Python来开发,如果想要用到一些C/C++的图像处理库,就需要创 ...
- python 快速读取图像宽高信息
python 快速读取图像宽高信息 1. 背景 2. 方法 2.1 仅读取图像文件头部信息 2.2 采用特定 python 包读取 1. 背景 存在100w张JPG图像,需要获取它们的宽高信息.如果用 ...
- [转载] python+opencv4读取图像
参考链接: Python Opencv 基础 1: imread 读取图像 1安装opencv 2安装jupyter Notebook 1读取图片 import cv2 #opencv读取的格式是BG ...
- OpenCV使用教程-读取图像imread使用说明
1.方法说明: import cv2 as cv img1 =cv.imread(filename[, flags]) 参数 说明 filename 图片路径地址 flags 读取图片方式 2.目前支 ...
- python各种读取图像方法
一.读取图像 使用 matplotlib. image from matplotlib import image as mping from matplotlib import pyplot as p ...
- OpenCV+python:读取图片
1,源码: import cv2 as cv #导入OpenCV库 import numpy as np #导入numpy科学计算包 print("--------- Python + Op ...
- python PIL读取图像转换为灰度图及二值图像
目录 以下操作都是在windows环境下进行. 一.读取数据 1. 读取单个图像: 2. 批量读取: 3. 看一下是否读取成功: 二.模式"RGB"转换为'L'模式灰度图 三.模式 ...
- OpenCV入门:读取图像以及对图像进行一些操作,比如只提取某颜色部分的东西
需要说明的是 我的环境:Anaconda下的Spyder,使用的模块是OpenCV(4.2.0)+numpy(1.15.1) 我下面的代码运用的图片是下面这只猫 (cat1.jpg) 还有一辆车 (c ...
最新文章
- 7 orm 有批量更新_ORM之SQLAlchemy
- 皮一皮:现在真是键盘侠的年代阿....
- spring security默认登录页面登录用户,和自定义数据源
- 目标检测系列(七)——CornerNet:detecting objects as paired keypoints
- 最有效的创建大数据模型的6个技巧
- hdu 1159(最长公共子序列)
- 向银行贷款20万, 分期三年买50万的车,个人借款40万, 贷款10年买200万的房子,再贷款120万分创业...
- WebService只能在本地使用,无法通过网络访问的解决办法
- Neo4j HA环境配置
- IDEA build时出现Artifact contains illegal characters的解决
- linux虚拟机模板部署模板,创建和部署基于 Linux 的虚拟机模板
- docsify,文档生成利器!
- C++ Primer 5th 第15章 面向对象程序设计
- 联想服务器自动关机_联想电脑老是自动关机怎么回事
- Qt for Mac苹果开发中,使用Apple Developer文档
- vue 页面刷新404
- uniapp App跳转微信小程序并互相传递参数、接收微信小程序传递的参数
- TOJ 1335 优先队列
- windows下同一台电脑安装两个mysql数据库
- 边缘计算使能智慧电网