如何选择优化算法遗传算法_用遗传算法优化垃圾收集策略
如何选择优化算法遗传算法
Genetic Algorithms are a family of optimisation techniques that loosely resemble evolutionary processes in nature. It may be a crude analogy, but if you squint your eyes, Darwin’s Natural Selection does roughly resemble an optimisation task where the aim is to craft organisms perfectly suited to thrive in their environments. While it may have taken many millennia for humans to develop opposable thumbs and eagles to develop 20/4 vision, in this article I will show how to implement a Genetic Algorithm in Python for “evolving” a rubbish collecting robot in a couple of hours.
遗传算法是一系列优化技术,与自然界的进化过程大致相似。 这可能是一个粗略的类比,但是如果您起眼睛,达尔文的《自然选择》确实类似于优化任务,其目的是制造出非常适合在其环境中繁衍生息的生物。 虽然人类可能需要花费数千年的时间才能开发出相对的拇指和鹰来开发20/4视觉,但在本文中,我将展示如何在Python中实现遗传算法,以在几小时内“进化”垃圾收集机器人。
背景 (Background)
The best example I’ve come across to demonstrate how Genetic Algorithms work comes from a fantastic book on complex systems by Melanie Mitchell called “Complexity: A Guided Tour” (highly recommended). In one chapter, Mitchell introduces a robot named Robby whose sole purpose in life is to pick up rubbish and describes how to optimise a control strategy for Robby using a GA. Below I will explain my approach to solving this problem and show how to implement the algorithm in Python. There are some great packages for constructing these kinds of algorithms (such as DEAP) but in this tutorial, I will only be using base Python, Numpy and TQDM (optional).
我碰到的最好的例子演示了遗传算法的工作原理,这是梅拉妮·米切尔(Melanie Mitchell)撰写的一本关于复杂系统的出色著作,名为“复杂性:导览”(强烈推荐)。 在一个章节中,米切尔介绍了一个名为Robby的机器人,该机器人的唯一目的是捡拾垃圾,并描述了如何使用GA优化Robby的控制策略。 下面,我将解释解决此问题的方法,并展示如何在Python中实现该算法。 有一些很棒的软件包可用于构建这类算法(例如DEAP ),但是在本教程中,我将仅使用基本的Python,Numpy和TQDM(可选)。
While this is only a toy example, GAs are used in a number of real-world applications. As a data scientist, I most often use them for hyper-parameter optimisation and model choice. While they can be computationally expensive, GAs allow us to explore multiple areas of a search space in parallel and are a good option when computing a gradient is difficult.
尽管这只是一个玩具示例,但GA已在许多实际应用中使用。 作为数据科学家,我经常将它们用于超参数优化和模型选择。 尽管它们的计算成本可能很高,但GA允许我们并行探索搜索空间的多个区域,当难以计算梯度时,它们是一个不错的选择。
问题描述 (Problem Description)
A robot named Robby lives in a two-dimensional grid world full of rubbish and surrounded by 4 walls (shown below). The aim of this project is to evolve an optimal control strategy for Robby that will allow him to pick up rubbish efficiently and not crash into walls.
名为Robby的机器人生活在一个充满垃圾的二维网格世界中,周围环绕着4面墙(如下所示)。 该项目的目的是为Robby制定最佳的控制策略,使他能够有效地捡拾垃圾而不撞墙。

Robby can only see the four squares NESW of himself as well as the square he is in and there are 3 options for each square; it can be empty, have rubbish in it or be a wall. Therefore there are 3⁵ = 243 different scenarios Robby can be in. Robby can perform 7 different actions; move NESW, move randomly, pickup rubbish or stay still. Robby’s control strategy can therefore be encoded as a “DNA” string of 243 digits between 0 and 6 (corresponding to the action Robby should take in each of the 243 possible situations).
罗比只能看到他自己的四个NESW以及他所在的正方形,每个正方形有3个选项; 它可以是空的,里面有垃圾或可以是墙壁。 因此,Robby可能有3种= 243种不同的场景。Robby可以执行7种不同的动作; 移动NESW,随机移动,捡垃圾或保持静止。 因此,Robby的控制策略可以被编码为0到6之间的243位数的“ DNA”字符串(对应于Robby在243种可能的情况下应采取的措施)。
方法 (Approach)
At a high level, the steps for optimisation with any GA are:
从总体上讲,使用任何GA进行优化的步骤如下:
- An initial “population” of random solutions to a problem are generated生成问题的随机解决方案的初始“填充”
- The “fitness” of each individual is assessed based on how well it solves the problem根据每个人解决问题的能力来评估每个人的“适应性”
- The fittest solutions “reproduce” and pass on “genetic” material to offspring in the next generation最合适的解决方案“繁殖”并“遗传”材料传给下一代
- Repeat steps 2 and 3 until we are left with a population of optimised solutions重复步骤2和3,直到剩下优化解决方案为止
For our task, you create a first generation of Robbys initialised to random DNA strings (corresponding to random control strategies). You then simulate letting these robots run around in randomly assigned grid worlds and see how they perform.
对于我们的任务,您将创建初始化为随机DNA字符串(对应于随机控制策略)的第一代Robby。 然后,您可以模拟这些机器人在随机分配的网格世界中运行,并观察它们的性能。
适合度 (Fitness)
A robot’s fitness is a function of how many pieces of rubbish it picked up in n moves and how many times it crashed into a wall. In our example, we award 10 points to a robot for every piece of rubbish picked up and subtract 5 points every time it crashes into a wall. The robots then “mate” with probabilities linked to their fitness score (i.e. robots that picked up lots of rubbish are more likely to reproduce) and a new generation is created.
机器人的适应性取决于它在n步中捡起多少垃圾以及撞到墙壁的次数。 在我们的示例中,对于每捡起的垃圾,我们会为机器人奖励10分,每次撞到墙壁时,机器人都会减去5分。 然后,机器人将与其适应度得分相关联的概率“配对”(即,捡拾大量垃圾的机器人更有可能繁殖),并创造了新一代。
交配 (Mating)
There are a few different ways that “mating” can be implemented. In Mitchell’s version she took the 2 parent DNA strings, randomly spliced them and then joined them together to create a child for the next generation. In my implementation, I assigned each gene probabilistically from each parent (i.e. for each of the 243 genes, I flip a coin to decide which parent will pass on their gene). For example, here are the first 10 genes of 2 parents and a possible child using my method:
有几种不同的方法可以实现“匹配”。 在米切尔(Mitchell)的版本中,她采用了2个父DNA字符串,随机拼接它们,然后将它们结合在一起,为下一代创造了一个孩子。 在我的实现中,我按概率从每个亲本中分配了每个基因(即,对于243个基因中的每个基因,我掷硬币决定哪一个亲本将通过其基因)。 例如,以下是使用我的方法的2个父母和一个可能的孩子的前10个基因:
Parent 1: 1440623161Parent 2: 2430661132Child: 2440621161
突变 (Mutation)
Another concept from natural selection that we replicate with this algorithm is that of “mutation”. While the vast majority of genes in a child will be passed down from a parent, I have also built in a small possibility that the gene will mutate (i.e. will be assigned at random). This mutation rate gives us the ability to control the trade-off between exploration and exploitation.
我们使用该算法复制的自然选择的另一个概念是“变异”。 虽然孩子中的绝大多数基因会从父母那里遗传下来,但我也认为基因突变的可能性很小(即随机分配)。 这种突变率使我们能够控制勘探与开发之间的权衡。
Python实现 (Python Implementation)
The first step is to import the required packages and set our parameters for this task. I have chosen these parameters as a starting point, but they can be adjusted and I encourage you to experiment with them.
第一步是导入所需的程序包并为此任务设置参数。 我选择了这些参数作为起点,但是可以对其进行调整,建议您尝试一下。
"""
Import Packages
"""
import numpy as np
from tqdm.notebook import tqdm"""
Set Parameters
"""
# Simulation Settings
pop_size = 200 # number of robots per generation
num_breeders = 100 # number of robots who can mate in each generation
num_gen = 400 # total number of generations
iter_per_sim = 100 # number of rubbish-collection simulations per robot
moves_per_iter = 200 # number of moves robot can make per simulation# Grid Settings
rubbish_prob = 0.5 # probability of rubbish in each grid square
grid_size = 10 # size of grid (excluding walls)# Evolution Settings
wall_penalty = -5 # fitness points deducted for crashing into wall
no_rub_penalty = -1 # fitness points deducted for trying to pickup rubbish in empty square
rubbish_score = 10 # fitness points awarded for picking up rubbish
mutation_rate = 0.01 # probability of a gene mutating
Next, we define a class for the grid-world environment. We represent each cell by the tokens ‘o’, ‘x’ and ‘w’ corresponding to an empty cell, a cell with rubbish and a wall respectively.
接下来,我们为网格世界环境定义一个类。 我们用标记“ o”,“ x”和“ w”表示每个单元,分别对应一个空单元,一个带有垃圾的单元和一个壁。
class Environment:"""Class for representing a grid environment full of rubbish. Each cell can be:'o': empty'x': rubbish'w': wall"""def __init__(self, p=rubbish_prob, g_size=grid_size):self.p = p # probability of a cell being rubbishself.g_size = g_size # excluding walls# initialise grid and randomly allocate rubbishself.grid = np.random.choice(['o','x'], size=(self.g_size+2,self.g_size+2), p=(1 - self.p, self.p))# set exterior squares to be wallsself.grid[:,[0,self.g_size+1]] = 'w'self.grid[[0,self.g_size+1], :] = 'w'def show_grid(self):# print the grid in current stateprint(self.grid)def remove_rubbish(self,i,j):# remove rubbish from specified cell (i,j)if self.grid[i,j] == 'o': # cell already emptyreturn Falseelse:self.grid[i,j] = 'o'return Truedef get_pos_string(self,i,j):# return a string representing the cells "visible" to a robot in cell (i,j)return self.grid[i-1,j] + self.grid[i,j+1] + self.grid[i+1,j] + self.grid[i,j-1] + self.grid[i,j]
Next, we create a class to represent our robots. This class includes methods for performing actions, calculating fitness and generating new DNA from a pair of parent robots.
接下来,我们创建一个代表机器人的类。 该课程包括执行动作,计算适应性以及从一对父机器人生成新DNA的方法。
class Robot:"""Class for representing a rubbish-collecting robot"""def __init__(self, p1_dna=None, p2_dna=None, m_rate=mutation_rate, w_pen=wall_penalty, nr_pen=no_rub_penalty, r_score=rubbish_score):self.m_rate = m_rate # mutation rateself.wall_penalty = w_pen # penalty for crashing into a wallself.no_rub_penalty = nr_pen # penalty for picking up rubbish in empty cellself.rubbish_score = r_score # reward for picking up rubbishself.p1_dna = p1_dna # parent 1 DNAself.p2_dna = p2_dna # parent 2 DNA# generate dict to lookup gene index from situation stringcon = ['w','o','x'] # wall, empty, rubbishself.situ_dict = dict()count = 0for up in con:for right in con:for down in con:for left in con:for pos in con:self.situ_dict[up+right+down+left+pos] = countcount += 1# initialise dnaself.get_dna()def get_dna(self):# initialise dna string for robotif self.p1_dna is None:# when no parents (first gen) initialise to random stringself.dna = ''.join([str(x) for x in np.random.randint(7,size=243)])else:self.dna = self.mix_dna()def mix_dna(self):# generate robot dna from parentsmix_dna = ''.join([np.random.choice([self.p1_dna,self.p2_dna])[i] for i in range(243)])#add mutationsfor i in range(243):if np.random.rand() > 1 - self.m_rate:mix_dna = mix_dna[:i] + str(np.random.randint(7)) + mix_dna[i+1:]return mix_dnadef simulate(self, n_iterations, n_moves, debug=False):# simulate rubbish collectiontot_score = 0for it in range(n_iterations):self.score = 0 # fitness scoreself.envir = Environment()self.i, self.j = np.random.randint(1,self.envir.g_size+1, size=2) # randomly allocate starting positionif debug:print('before')print('start position:',self.i, self.j)self.envir.show_grid()for move in range(n_moves):self.act()tot_score += self.scoreif debug:print('after')print('end position:',self.i, self.j)self.envir.show_grid()print('score:',self.score)return tot_score / n_iterations # average fitness score across n iterationsdef act(self):# perform action based on DNA and robot situationpost_str = self.envir.get_pos_string(self.i, self.j) # robot's current situationgene_idx = self.situ_dict[post_str] # relevant idx of DNA for current situationact_key = self.dna[gene_idx] # read action from idx of DNAif act_key == '5':# move randomlyact_key = np.random.choice(['0','1','2','3'])if act_key == '0':self.mv_up()elif act_key == '1':self.mv_right()elif act_key == '2':self.mv_down()elif act_key == '3':self.mv_left()elif act_key == '6':self.pickup()def mv_up(self):# move up one squareif self.i == 1:self.score += self.wall_penaltyelse:self.i -= 1def mv_right(self):# move right one squareif self.j == self.envir.g_size:self.score += self.wall_penaltyelse:self.j += 1def mv_down(self):# move down one squareif self.i == self.envir.g_size:self.score += self.wall_penaltyelse:self.i += 1def mv_left(self):# move left one squareif self.j == 1:self.score += self.wall_penaltyelse:self.j -= 1def pickup(self):# pickup rubbishsuccess = self.envir.remove_rubbish(self.i, self.j)if success:# rubbish successfully picked upself.score += self.rubbish_scoreelse:# no rubbish in current squareself.score += self.no_rub_penalty
Finally it’s time to run the Genetic Algorithm. In the code below we generate an initial population of robots and let Natural Selection run its course. I should mention that there are certainly much faster ways to implement this algorithm (e.g. utilising parallelisation) but for the purpose of this tutorial I have sacrificed speed for clarity.
最后是时候运行遗传算法了。 在下面的代码中,我们生成了机器人的初始填充,然后让Natural Selection运行其过程。 我应该提到,当然有更快的方法来实现该算法(例如,利用并行化),但是出于本教程的目的,我为了清晰起见而牺牲了速度。
# initial population
pop = [Robot() for x in range(pop_size)]
results = []# run evolution
for i in tqdm(range(num_gen)):scores = np.zeros(pop_size)# iterate through all robotsfor idx, rob in enumerate(pop):# run rubbish collection simulation and calculate fitnessscore = rob.simulate(iter_per_sim, moves_per_iter)scores[idx] = scoreresults.append([scores.mean(),scores.max()]) # save mean and max scores for each generationbest_robot = pop[scores.argmax()] # save the best robot# limit robots who are able to mate to top num_breedersinds = np.argpartition(scores, -num_breeders)[-num_breeders:] # get indices of top robots based on fitnesssubpop = []for idx in inds:subpop.append(pop[idx])scores = scores[inds]# square and normalise fitness scoresnorm_scores = (scores - scores.min()) ** 2 norm_scores = norm_scores / norm_scores.sum()# create next generation of robotsnew_pop = []for child in range(pop_size):# choose parents with probability proportionate to squared fitness scoresp1, p2 = np.random.choice(subpop, p=norm_scores, size=2, replace=False)new_pop.append(Robot(p1.dna, p2.dna))pop = new_pop
While initially most of the robots pick up no rubbish and consistently crash into walls, within a few generations we start to see simple strategies (such as “if in square with rubbish, pick it up” and “if next to wall, don’t move into wall”) propagate through the population. After a few hundred iterations, we are left with a generation of incredible rubbish-collecting geniuses!
最初,大多数机器人没有捡拾垃圾,并且始终撞到墙壁上,但在几代人之后,我们开始看到简单的策略(例如“如果与垃圾成一直线,捡拾垃圾”和“如果靠近墙壁,不要捡垃圾”)。进入墙壁”)在人群中传播。 经过几百次迭代,我们剩下了一代令人难以置信的垃圾收集天才!
结果 (Results)
The plot below shows that we were able to “evolve” a successful rubbish collection strategy in 400 generations.
下图显示了我们能够在400代内“演变”成功的垃圾收集策略。

To assess the quality of the evolved control strategy, I manually created a benchmark strategy with some intuitively sensible rules:
为了评估改进的控制策略的质量,我手动创建了一些具有直观意义的规则的基准策略:
- If rubbish is in current square, pick it up如果垃圾在当前方块中,请捡起
- If rubbish is visible in an adjacent square, move to that square如果在相邻的正方形中可见垃圾,请移至该正方形
- If next to a wall, move in the opposite direction如果靠近墙壁,请朝相反方向移动
- Else, move in a random direction否则,朝随机方向移动
On average, this benchmark strategy achieved a fitness score of 426.9 but this was no match for our final “evolved” robot which had an average fitness score of 475.9.
平均而言,该基准策略的适应度得分为426.9,但这与我们最终的“进化”机器人的平均适应度得分为475.9并不匹配。
策略分析 (Strategy Analysis)
One of the coolest things about this approach to optimisation is that you can find counter-intuitive solutions. Not only were the robots able to learn sensible rules that a human might design, they also spontaneously came up with strategies that humans might never consider. One sophisticated technique that emerged was the use of “markers” to overcome short-sightedness and a lack of memory. For example, if a robot is currently in a square with rubbish in it and can see rubbish in the squares to the east and west, a naive approach would be to immediately pickup the rubbish in the current square and then move to one of the adjacent squares. The problem with this strategy is that once the robot has moved (say to the west), he has no way of remembering that there is rubbish 2 squares to the east. To overcome this problem, we observed our evolved robots performing the following steps:
关于这种优化方法的最酷的事情之一是,您可以找到违反直觉的解决方案。 机器人不仅能够学习人类可能设计的明智规则,而且还自发提出了人类可能永远不会考虑的策略。 一种新兴的先进技术是使用“标记”来克服近视和缺乏记忆。 例如,如果一个机器人当前在一个有垃圾的正方形中并且可以看到东西方的正方形中的垃圾,那么幼稚的方法是立即在当前正方形中拾取垃圾,然后移到相邻的一个正方形中。方块。 这种策略的问题在于,一旦机器人移动(例如向西移动),他就无法记住东边有2个正方形的垃圾。 为了克服这个问题,我们观察了我们进化的机器人执行以下步骤:
- move to the west (leave the rubbish in the current square as a marker)向西移动(将垃圾放在当前广场中作为标记)
- pick up the rubbish and move back to the east (it can see the rubbish left as a marker)捡起垃圾并向东移动(可以看到垃圾作为标记)
- pick up the rubbish and move to the east捡起垃圾,然后移到东方
- pick up the final piece of rubbish捡起最后一块垃圾
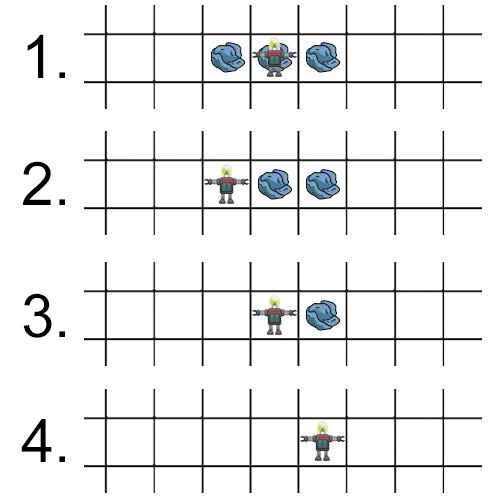
Another example of counter-intuitive strategies emerging from this kind of optimisation is shown below. OpenAI taught agents to play hide and seek using reinforcement learning (a more sophisticated optimisation approach). We see that the agents learn “human” strategies at first but eventually learn novel solutions such as “Box Surfing” that exploit the physics of OpenAI’s simulated environment.
这种优化产生的反直觉策略的另一个示例如下所示。 OpenAI教给代理商使用强化学习(一种更复杂的优化方法)玩捉迷藏的游戏。 我们看到代理首先学习“人性化”策略,但最终学习了新颖的解决方案,例如“盒式冲浪”,它们利用了OpenAI模拟环境的物理特性。
演示地址
结论 (Conclusion)
Genetic Algorithms combine biology and computer science in a unique way and while not necessarily the fastest algorithms, in my opinion they are among the most beautiful. All of the code featured in this article is available on my Github along with a demo notebook. Thanks for reading!
遗传算法以独特的方式结合了生物学和计算机科学,虽然不一定是最快的算法,但我认为它们是最漂亮的算法之一。 我的Github和演示笔记本都提供了本文中介绍的所有代码。 谢谢阅读!
翻译自: https://towardsdatascience.com/optimising-a-rubbish-collection-strategy-with-genetic-algorithms-ccf1f4d56c4f
如何选择优化算法遗传算法
http://www.taodudu.cc/news/show-7137370.html
相关文章:
- Repeated DNA Sequences @leetcode
- JVM:内存与垃圾回收篇
- JVM内存与垃圾回收 Day02
- 用遗传算法优化垃圾收集策略
- P3763 [TJOI2017]DNA(SAM+dfs)
- JVM——内存与垃圾回收
- DNA偶联PbSe量子点|近红外硒化铅PbSe量子点修饰脱氧核糖核酸DNA|PbSe-DNA QDs
- 这款DNA机器人,可以帮你分拣身体中的生物垃圾
- 计算机与生物技术的论文,DNA计算机生物技术论文
- DNA修饰金属锇Os纳米颗粒OsNPS-DNA|DNA修饰金属铱纳米颗粒IrNPS-DNA
- ZnS-DNA QDs近红外硫化锌ZnS量子点改性脱氧核糖核酸DNA|DNA修饰ZnS量子点
- 读书笔记-垃圾收集器
- DNA脱氧核糖核酸修饰四氧化三铁|DNA修饰氧化锌|使用方法
- DeeCamp 2021冠军用“垃圾DNA”预测癌症,李开复:医疗是AI的下一个突破点
- 转座子 垃圾DNA是指DNA中不编码蛋白质序列的片段,是DNA中最神秘的部分之一。
- 打印图案.
- 处理A4不能打印大图片
- 打印常见的图形
- Kaop打印项之图片
- 前端 打印
- 打印各种图案
- 艾永亮:马云的“野心之作”无人超市,结果真的如愿以偿吗
- 无人超市信息管理系统——问题定义
- 阿里无人超市亮相乌镇,微笑购物能打折!诺奖得主现场点赞!
- 你有一张阿里无人超市体验劵 请查收
- 综合案例-智能无人超市
- 无人超市之后,马云的小卖部也来了
- 阿里巴巴 iDST 首席科学家兼副院长任小枫:最看好计算机视觉在这四大新零售细分方向的应用...
- 14款奔驰R400升级ACC自适应巡航系统,增加您的行车安全性
- 22款奔驰E350升级ACC自适应巡航系统,解放您的双脚
如何选择优化算法遗传算法_用遗传算法优化垃圾收集策略相关推荐
- 【大论文】可扩展机器学习的并行与分布式优化算法综述_亢良伊2017
一.基础知识: 1.目标函数 机器学习要优化的目标函数一般表现为一下形式: 函数J(θ)为目标函数,f为表示真实值与拟合值之差的损失函数,r(θ)为正则项(防止过拟合问题,主要分为L1正则项.L2正则 ...
- 基于鲸鱼优化算法的Simulink仿真模型参数优化
目录 1.鲸鱼优化算法(WOA) 1.1算法原理 1.1.1 包围猎物 1.1.2 狩猎行为 1.1.3 搜索猎物 1.4 算法流程 2.如何用matlab .m文件脚本调用simulink模型并传入 ...
- 智能优化算法:人工大猩猩部队优化算法-附代码
智能优化算法:人工大猩猩部队优化算法 文章目录 智能优化算法:人工大猩猩部队优化算法 1.算法原理 1.1勘探阶段 1.2 开发阶段 1.2.1 跟随银背大猩猩 1.2.2 竞争成年雌性 2.实验结果 ...
- 【优化算法】改进的灰狼优化算法(IGWO)【含Matlab源码 1349期】
一.获取代码方式 获取代码方式1: 完整代码已上传我的资源:[优化算法]改进的灰狼优化算法(IGWO)[含Matlab源码 1349期] 点击上面蓝色字体,直接付费下载,即可. 获取代码方式2: 付费 ...
- 【优化算法】多目标灰狼优化算法(MOGWO)【含Matlab源码 099期】
一.获取代码方式 获取代码方式1: 完整代码已上传我的资源:[优化算法]多目标灰狼优化算法(MOGWO)[含Matlab源码 099期] 点击上面蓝色字体,直接付费下载,即可. 获取代码方式2: 付费 ...
- gwo算法matlab源代码,智能优化算法应用:基于GWO优化BP神经网络 - 附代码
智能优化算法应用:基于GWO优化BP神经网络 - 附代码 智能优化算法应用:基于GWO优化BP神经网络 - 附代码 智能优化算法应用:基于GWO优化BP神经网络 文章目录智能优化算法应用:基于GWO优 ...
- 【优化算法】象群游牧优化算法(EHO)【含Matlab源码 1080期】
⛄一.获取代码方式 获取代码方式1: 完整代码已上传我的资源:[优化算法]象群游牧优化算法(EHO)[含Matlab源码 1080期] 点击上面蓝色字体,直接付费下载,即可. 获取代码方式2: 付费专 ...
- 【优化算法】细菌粒子群优化算法【含Matlab源码 1195期】
⛄一.获取代码方式 获取代码方式1: 完整代码已上传我的资源:[优化算法]细菌粒子群优化算法[含Matlab源码 1195期] 点击上面蓝色字体,直接付费下载,即可. 获取代码方式2: 付费专栏Mat ...
- 优化算法 | 随机漂移粒子群优化算法(附标准PSO算法Python工具包)
目录 ▎标准PSO算法 ▎RDPSO算法 ▎标准PSO算法Python工具包 01 | 工具包适用范围 02 | 工具包安装方法 03 | 工具包使用实例 ▎参考文献 今天为各位讲解一种改进的粒子群优 ...
- 【优化算法】多目标蚁狮优化算法(MOALO)【含Matlab源码 1598期】
⛄一.获取代码方式 获取代码方式1: 完整代码已上传我的资源:[优化算法]多目标蚁狮优化算法(MOALO)[含Matlab源码 1598期] 点击上面蓝色字体,直接付费下载,即可. 获取代码方式2: ...
最新文章
- Mongo、Redis、Memcached对比及知识总结
- [Hihocoder 1289] 403 Forbidden (微软2016校园招聘4月在线笔试)
- python测试代码怎么写_Python 单元测试
- Android 系统自动重启Bug(高通平台)
- mysql 与紫金桥_基于紫金桥组态软件与mysql数据库的连接简介
- 【python零基础入门学习】Python入门,带你快速学习,Python 中文编码
- Unity3D的几种坐标系
- Python之数据分析(规范数据生成器Faker,学习、数据分析、开发测试专用)
- pycharm2019新建python文件_PyCharm 2019安装教程
- python bool类型如何与整数比较_Python入门必读bool类型和比较运算符
- SpringBoot+Vue项目上手
- Atitit 项目文档规范化与必备文档与推荐文档列表
- CNN卷积神经网络原理详解(上)
- 使用Qt Designer来设计界面
- poi操作ppt创建表格
- 使用git提交到仓库使用commit指令出现问题Your branch and 'origin/master' have diverged,
- 五阶魔方公式java_五阶魔方花样有多漂亮,关键是有点难
- 格局打开,外贸牛人的价格谈判策略!
- 前端学习——HTML(一)
- 明德扬手把手教你设计VGA显示颜色