Spring AOP + AspectJ Annotation Example---reference
In this tutorial, we show you how to integrate AspectJ annotation with Spring AOP framework. In simple, Spring AOP + AspectJ allow you to intercept method easily.
Common AspectJ annotations :
- @Before – Run before the method execution
- @After – Run after the method returned a result
- @AfterReturning – Run after the method returned a result, intercept the returned result as well.
- @AfterThrowing – Run after the method throws an exception
- @Around – Run around the method execution, combine all three advices above.
For Spring AOP without AspectJ support, read this build-in Spring AOP examples.
1. Directory Structure
See directory structure of this example.
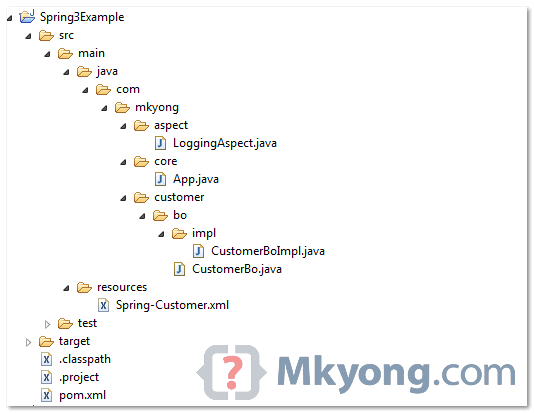
2. Project Dependencies
To enable AspectJ, you need aspectjrt.jar, aspectjweaver.jar and spring-aop.jar. See following Maven pom.xml
file.
This example is using Spring 3, but the AspectJ features are supported since Spring 2.0.
File : pom.xml
<project ...><properties><spring.version>3.0.5.RELEASE</spring.version></properties><dependencies><dependency><groupId>org.springframework</groupId><artifactId>spring-core</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-context</artifactId><version>${spring.version}</version></dependency><!-- Spring AOP + AspectJ --><dependency><groupId>org.springframework</groupId><artifactId>spring-aop</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.aspectj</groupId><artifactId>aspectjrt</artifactId><version>1.6.11</version></dependency><dependency><groupId>org.aspectj</groupId><artifactId>aspectjweaver</artifactId><version>1.6.11</version></dependency></dependencies> </project>
3. Spring Beans
Normal bean, with few methods, later intercept it via AspectJ annotation.
package com.mkyong.customer.bo;public interface CustomerBo {void addCustomer();String addCustomerReturnValue();void addCustomerThrowException() throws Exception;void addCustomerAround(String name); }package com.mkyong.customer.bo.impl;import com.mkyong.customer.bo.CustomerBo;public class CustomerBoImpl implements CustomerBo {public void addCustomer(){System.out.println("addCustomer() is running ");}public String addCustomerReturnValue(){System.out.println("addCustomerReturnValue() is running ");return "abc";}public void addCustomerThrowException() throws Exception {System.out.println("addCustomerThrowException() is running ");throw new Exception("Generic Error");}public void addCustomerAround(String name){System.out.println("addCustomerAround() is running, args : " + name);} }
4. Enable AspectJ
In Spring configuration file, put “<aop:aspectj-autoproxy />
“, and define your Aspect (interceptor) and normal bean.
File : Spring-Customer.xml
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "><aop:aspectj-autoproxy /><bean id="customerBo" class="com.mkyong.customer.bo.impl.CustomerBoImpl" /><!-- Aspect --><bean id="logAspect" class="com.mkyong.aspect.LoggingAspect" /></beans>
4. AspectJ @Before
In below example, the logBefore()
method will be executed before the execution of customerBo interface, addCustomer()
method.
AspectJ “pointcuts” is used to declare which method is going to intercept, and you should refer to this Spring AOP pointcuts guide for full list of supported pointcuts expressions.
File : LoggingAspect.java
package com.mkyong.aspect;import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before;@Aspect public class LoggingAspect {@Before("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))")public void logBefore(JoinPoint joinPoint) {System.out.println("logBefore() is running!");System.out.println("hijacked : " + joinPoint.getSignature().getName());System.out.println("******");}}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");customer.addCustomer();
Output
logBefore() is running!
hijacked : addCustomer
******
addCustomer() is running
5. AspectJ @After
In below example, the logAfter()
method will be executed after the execution of customerBo interface, addCustomer()
method.
File : LoggingAspect.java
package com.mkyong.aspect;import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.After;@Aspect
public class LoggingAspect {@After("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))")public void logAfter(JoinPoint joinPoint) {System.out.println("logAfter() is running!");System.out.println("hijacked : " + joinPoint.getSignature().getName());System.out.println("******");}}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");customer.addCustomer();
Output
addCustomer() is running
logAfter() is running!
hijacked : addCustomer
******
6. AspectJ @AfterReturning
In below example, the logAfterReturning()
method will be executed after the execution of customerBo interface,addCustomerReturnValue()
method. In addition, you can intercept the returned value with the “returning” attribute.
To intercept returned value, the value of the “returning” attribute (result) need to be same with the method parameter (result).
File : LoggingAspect.java
package com.mkyong.aspect;import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.AfterReturning;@Aspect
public class LoggingAspect {@AfterReturning(pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerReturnValue(..))",returning= "result")public void logAfterReturning(JoinPoint joinPoint, Object result) {System.out.println("logAfterReturning() is running!");System.out.println("hijacked : " + joinPoint.getSignature().getName());System.out.println("Method returned value is : " + result);System.out.println("******");}}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");customer.addCustomerReturnValue();
Output
addCustomerReturnValue() is running
logAfterReturning() is running!
hijacked : addCustomerReturnValue
Method returned value is : abc
******
7. AspectJ @AfterReturning
In below example, the logAfterThrowing()
method will be executed if the customerBo interface,addCustomerThrowException()
method is throwing an exception.
File : LoggingAspect.java
package com.mkyong.aspect;import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.AfterThrowing;@Aspect
public class LoggingAspect {@AfterThrowing(pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerThrowException(..))",throwing= "error")public void logAfterThrowing(JoinPoint joinPoint, Throwable error) {System.out.println("logAfterThrowing() is running!");System.out.println("hijacked : " + joinPoint.getSignature().getName());System.out.println("Exception : " + error);System.out.println("******");}
}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");customer.addCustomerThrowException();
Output
addCustomerThrowException() is running
logAfterThrowing() is running!
hijacked : addCustomerThrowException
Exception : java.lang.Exception: Generic Error
******
Exception in thread "main" java.lang.Exception: Generic Error//...
8. AspectJ @Around
In below example, the logAround()
method will be executed before the customerBo interface, addCustomerAround()
method, and you have to define the “joinPoint.proceed();
” to control when should the interceptor return the control to the original addCustomerAround()
method.
File : LoggingAspect.java
package com.mkyong.aspect;import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Around;@Aspect
public class LoggingAspect {@Around("execution(* com.mkyong.customer.bo.CustomerBo.addCustomerAround(..))")public void logAround(ProceedingJoinPoint joinPoint) throws Throwable {System.out.println("logAround() is running!");System.out.println("hijacked method : " + joinPoint.getSignature().getName());System.out.println("hijacked arguments : " + Arrays.toString(joinPoint.getArgs()));System.out.println("Around before is running!");joinPoint.proceed(); //continue on the intercepted methodSystem.out.println("Around after is running!");System.out.println("******");}}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");customer.addCustomerAround("mkyong");
Output
logAround() is running!
hijacked method : addCustomerAround
hijacked arguments : [mkyong]
Around before is running!
addCustomerAround() is running, args : mkyong
Around after is running!
******
Conclusion
It’s always recommended to apply the least power AsjectJ annotation. It’s rather long article about AspectJ in Spring. for further explanations and examples, please visit the reference links below.
No worry, AspectJ supported XML configuration also, read this Spring AOP + AspectJ XML example.
Download Source Code
原文地址:http://www.mkyong.com/spring3/spring-aop-aspectj-annotation-example/
转载于:https://www.cnblogs.com/davidwang456/p/4121750.html
Spring AOP + AspectJ Annotation Example---reference相关推荐
- 关于 Spring AOP (AspectJ) 你该知晓的一切
[版权申明]未经博主同意,谢绝转载!(请尊重原创,博主保留追究权) http://blog.csdn.net/javazejian/article/details/54629058 出自[zejian ...
- Spring AOP / AspectJ AOP 的区别?
Spring AOP / AspectJ AOP 的区别? Spring AOP属于运行时增强,而AspectJ是编译时增强. Spring AOP基于代理(Proxying),而AspectJ基于字 ...
- Spring AOP,AspectJ,CGLIB 有点晕
AOP(Aspect Orient Programming),作为面向对象编程的一种补充,广泛应用于处理一些具有横切性质的系统级服务,如事务管理.安全检查.缓存.对象池管理等.AOP 实现的关键就在于 ...
- Spring AOP AspectJ
本文讲述使用AspectJ框架实现Spring AOP. 再重复一下Spring AOP中的三个概念, Advice:向程序内部注入的代码. Pointcut:注入Advice的位置,切入点,一般为某 ...
- Spring AOP AspectJ 代码实例
本文参考来源 http://examples.javacodegeeks.com/enterprise-java/spring/aop/spring-aop-aspectj-example/ http ...
- Spring AOP AspectJ Pointcut Expressions With Examples--转
原文地址:http://howtodoinjava.com/spring/spring-aop/writing-spring-aop-aspectj-pointcut-expressions-with ...
- spring aop 中@annotation()和自定义注解的使用
在自定义个注解之后,通过这个注解,标注需要切入的方法,同时把需要的参数传到切面去.那么我们怎么在切面使用这个注解. 我们使用这个自定义注解一方面是为了传一些参数,另一方面也是为了省事. 具体怎么省事, ...
- Spring AOP技术(基于AspectJ)的Annotation开发
Spring AOP技术(基于AspectJ)的Annotation开发 @(Spring)[aop, spring, xml, Spring, annotation, aspectJ] Spring ...
- Spring aop 循环依赖 Is there an unresolvable circular reference?
问题描述 在使用Spring通过注解方式实现AOP时报出循环依赖错误 完整的报错信息: Caused by: org.springframework.beans.factory.BeanCurrent ...
最新文章
- 10个最常用 Windows Vista运行命令
- ID3、C4.5、CART树算法简介,这几个算法有什么区别?对于异常值和分类值有什么需要注意的?
- 7-26复习重载并实现重载部分符号
- Hibernate程序性能优化的考虑要点
- openjdk 使用_如何在OpenJDK中使用ECC
- Linux -Ubuntu安装 Tomcat
- golang channel的一些总结
- html5声称需要大写吗,html5中有没有规定字母标签是用大写还是小写?
- Java实现二分查找法
- left和offsetLeft
- 图像加密之灰度加密:基于 密钥 × 解钥 ≡ 1 mod 灰度级 的一轮加密算法例子——lena图
- Python 计算变上限二重积分的数值模拟基础
- C语言报错警告合集(转)
- Signing for ‘xxx‘ requires a development team.
- 用最新版的Android Studio和Gradle把自己开发的Android包发布到JitPack上
- (以Windows 7 引导的)Windows 和Ubuntu双系统安装
- 游戏产业制作名人录(一)
- Mali GPU OpenGL ES 应用性能优化--基本方法
- 解决Android10和Android11创建文件失败问题
- 虚拟拨号数据网认证服务器,怎么设置虚拟拨号服务器
热门文章
- aspx 判断字符串是否为decimal_python3之判断字符串是否只为数字!isdigit()、isnumeric()方法...
- win8iis和php,Win8下IIS装PHP扩展
- redis linux 文件位置,Linux下Redis的安装和部署
- C#控制台程序生成文件分析
- ubuntu16.04 耳机没声音解决办法
- python super理解(二)
- Python 非线性方程组
- mysql监测攻击_如何检测SQL注入技术以及跨站脚本攻击
- 字典数(前缀树)的实现
- MATLAB应用实战系列(七十七)-【图像处理】COVID-19 防疫应用口罩检测