spring rmi_Spring远程支持和开发RMI服务
spring rmi
远程方法调用(RMI) :Spring通过RmiProxyFactoryBean和RmiServiceExporter支持RMI。 RmiServiceExporter将任何Spring管理的bean导出为RMI服务并进行注册。 RmiProxyFactoryBean是一种工厂bean,可为RMI服务创建代理。 该代理对象代表客户端与远程RMI服务进行通信。
Spring的HTTP调用程序: Spring HTTP调用程序使用标准的Java序列化机制通过HTTP公开服务。 Spring通过HttpInvokerProxyFactoryBean和HttpInvokerServiceExporter支持HTTP调用程序基础结构。 HttpInvokerServiceExporter,它将指定的服务bean导出为HTTP调用程序服务终结点,可通过HTTP调用程序代理访问。 HttpInvokerProxyFactoryBean是用于HTTP调用程序代理的工厂bean。
Hessian: Hessian提供了一个基于HTTP的二进制远程协议。 Spring通过HessianProxyFactoryBean和HessianServiceExporter支持Hessian。
粗麻布:粗麻布是Caucho的基于XML的粗麻布替代品。 Spring提供了支持类,例如BurlapProxyFactoryBean和BurlapServiceExporter。
JAX-RPC: Spring通过JAX-RPC(J2EE 1.4的Web服务API)为Web服务提供远程支持。
JAX-WS: Spring通过JAX-WS(Java EE 5和Java 6中引入的JAX-RPC的继承者)为Web服务提供远程支持。
JMS: JmsInvokerServiceExporter和JmsInvokerProxyFactoryBean类提供了Spring中的JMS远程支持。
让我们看一下Spring RMI支持以开发Spring RMI Service&Client。
二手技术:
JDK 1.6.0_31
春天3.1.1
Maven的3.0.2
步骤1:建立已完成的专案
创建一个Maven项目,如下所示。 (可以使用Maven或IDE插件创建)。
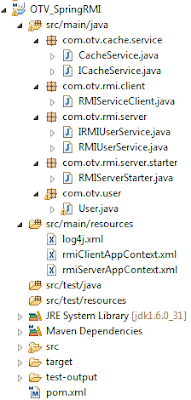
步骤2:图书馆
Spring依赖项已添加到Maven的pom.xml中。
<!-- Spring 3.1.x dependencies -->
<properties><spring.version>3.1.1.RELEASE</spring.version>
</properties><dependencies><dependency><groupId>org.springframework</groupId><artifactId>spring-core</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-context</artifactId><version>${spring.version}</version></dependency>
<dependencies>
步骤3:建立使用者类别
创建一个新的用户类。
package com.otv.user;import java.io.Serializable;/*** User Bean** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public class User implements Serializable {private long id;private String name;private String surname;/*** Get User Id** @return long id*/public long getId() {return id;}/*** Set User Id** @param long id*/public void setId(long id) {this.id = id;}/*** Get User Name** @return long id*/public String getName() {return name;}/*** Set User Name** @param String name*/public void setName(String name) {this.name = name;}/*** Get User Surname** @return long id*/public String getSurname() {return surname;}/*** Set User Surname** @param String surname*/public void setSurname(String surname) {this.surname = surname;}@Overridepublic String toString() {StringBuilder strBuilder = new StringBuilder();strBuilder.append("Id : ").append(getId());strBuilder.append(", Name : ").append(getName());strBuilder.append(", Surname : ").append(getSurname());return strBuilder.toString();}
}
步骤4:建立ICacheService介面
创建了代表远程缓存服务接口的ICacheService接口。
package com.otv.cache.service;import java.util.concurrent.ConcurrentHashMap;import com.otv.user.User;/*** Cache Service Interface** @author onlinetechvision.com* @since 27 Feb 2012* @version 1.0.0**/
public interface ICacheService {/*** Get User Map** @return ConcurrentHashMap User Map*/public ConcurrentHashMap<long, user> getUserMap();}
步骤5:创建CacheService类
CacheService类是通过实现ISchedulerService接口创建的。 它提供对远程缓存的访问…
package com.otv.cache.service;import java.util.concurrent.ConcurrentHashMap;import com.otv.user.User;/*** Cache Service Implementation** @author onlinetechvision.com* @since 6:04:49 PM* @version 1.0.0**/
public class CacheService implements ICacheService {//User Map is injected...ConcurrentHashMap<long, user> userMap;/*** Get User Map** @return ConcurrentHashMap User Map*/public ConcurrentHashMap<long, user> getUserMap() {return userMap;}/*** Set User Map** @param ConcurrentHashMap User Map*/public void setUserMap(ConcurrentHashMap<long, user> userMap) {this.userMap = userMap;}}
步骤6:建立IRMIUserService接口
创建了代表RMI服务接口的IRMIUserService接口。 此外,它为RMI客户端提供了远程方法。
package com.otv.rmi.server;import java.util.List;import com.otv.user.User;/*** RMI User Service Interface** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public interface IRMIUserService {/*** Add User** @param User user* @return boolean response of the method*/public boolean addUser(User user);/*** Delete User** @param User user* @return boolean response of the method*/public boolean deleteUser(User user);/*** Get User List** @return List user list*/public List<User> getUserList();}
步骤7:创建RMIUserService类
RMIUserService类(又名RMI对象)是通过实现IRMIUserService接口创建的。
package com.otv.rmi.server;import java.util.ArrayList;
import java.util.List;
import org.apache.log4j.Logger;import com.otv.cache.service.ICacheService;
import com.otv.user.User;/*** RMI User Service Implementation** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public class RMIUserService implements IRMIUserService {private static Logger logger = Logger.getLogger(RMIUserService.class);//Remote Cache Service is injected...ICacheService cacheService;/*** Add User** @param User user* @return boolean response of the method*/public boolean addUser(User user) {getCacheService().getUserMap().put(user.getId(), user);logger.debug("User has been added to cache. User : "+getCacheService().getUserMap().get(user.getId()));return true;}/*** Delete User** @param User user* @return boolean response of the method*/public boolean deleteUser(User user) {getCacheService().getUserMap().put(user.getId(), user);logger.debug("User has been deleted from cache. User : "+user);return true;}/*** Get User List** @return List user list*/public List<User> getUserList() {List<User> list = new ArrayList<User>();list.addAll(getCacheService().getUserMap().values());logger.debug("User List : "+list);return list;}/*** Get RMI User Service** @return IRMIUserService RMI User Service*/public ICacheService getCacheService() {return cacheService;}/*** Set RMI User Service** @param IRMIUserService RMI User Service*/public void setCacheService(ICacheService cacheService) {this.cacheService = cacheService;}
}
步骤8:创建RMIServerStarter类别
RMI服务器启动程序类已创建。 它启动RMI服务器。
package com.otv.rmi.server.starter;import org.springframework.context.support.ClassPathXmlApplicationContext;/*** RMI Server Starter** @author onlinetechvision.com* @since 27 Feb 2012* @version 1.0.0**/
public class RMIServerStarter {public static void main(String[] args) {//RMI Server Application Context is started...new ClassPathXmlApplicationContext("rmiServerAppContext.xml");}
}
步骤9:创建rmiServerAppContext.xml
RMI服务器应用程序上下文的内容如下所示。
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans-3.0.xsd"><!-- Beans Declaration --><bean id="UserMap" class="java.util.concurrent.ConcurrentHashMap" /><bean id="CacheService" class="com.otv.cache.service.CacheService"><property name="userMap" ref="UserMap"/></bean> <bean id="RMIUserService" class="com.otv.rmi.server.RMIUserService" ><property name="cacheService" ref="CacheService"/></bean><!-- RMI Server Declaration --><bean class="org.springframework.remoting.rmi.RmiServiceExporter"><!-- serviceName represents RMI Service Name --><property name="serviceName" value="RMIUserService"/><!-- service represents RMI Object(RMI Service Impl) --><property name="service" ref="RMIUserService"/><!-- serviceInterface represents RMI Service Interface exposed --><property name="serviceInterface" value="com.otv.rmi.server.IRMIUserService"/><!-- defaults to 1099 --><property name="registryPort" value="1099"/></bean></beans>
步骤10:创建RMIServiceClient类
RMIServiceClient类已创建。 它调用RMI用户服务并执行用户操作。
package com.otv.rmi.client;import org.apache.log4j.Logger;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;import com.otv.rmi.server.IRMIUserService;
import com.otv.rmi.server.RMIUserService;
import com.otv.user.User;/*** RMI Service Client** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public class RMIServiceClient {private static Logger logger = Logger.getLogger(RMIUserService.class);/*** Main method of the RMI Service Client**/public static void main(String[] args) {logger.debug("RMI Service Client is starting...");//RMI Client Application Context is started...ApplicationContext context = new ClassPathXmlApplicationContext("rmiClientAppContext.xml");//Remote User Service is called via RMI Client Application Context...IRMIUserService rmiClient = (IRMIUserService) context.getBean("RMIUserService");//New User is created...User user = new User();user.setId(1);user.setName("Bruce");user.setSurname("Willis");//The user is added to the remote cache...rmiClient.addUser(user);//The users are gotten via remote cache...rmiClient.getUserList();//The user is deleted from remote cache...rmiClient.deleteUser(user);logger.debug("RMI Service Client is stopped...");}
}
步骤11:创建rmiClientAppContext.xml
RMI客户端应用程序上下文的内容如下所示。
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans-3.0.xsd"><!-- RMI Client Declaration --><bean id="RMIUserService" class="org.springframework.remoting.rmi.RmiProxyFactoryBean"><!-- serviceUrl represents RMI Service Url called--><property name="serviceUrl" value="rmi://x.x.x.x:1099/RMIUserService"/><!-- serviceInterface represents RMI Service Interface called --><property name="serviceInterface" value="com.otv.rmi.server.IRMIUserService"/><!-- refreshStubOnConnectFailure enforces automatic re-lookup of the stub if acall fails with a connect exception --><property name="refreshStubOnConnectFailure" value="true"/></bean></beans>
步骤12:运行项目
如果运行RMI Server时启动了RMI Service Client,则将在RMI Server输出日志下方显示。 同样,可以通过IDE打开两个单独的控制台来运行RMI Server和Client。 :
....
04.03.2012 14:23:15 DEBUG (RmiBasedExporter.java:59) - RMI service [com.otv.rmi.server.RMIUserService@16dadf9] is an RMI invoker
04.03.2012 14:23:15 DEBUG (JdkDynamicAopProxy.java:113) - Creating JDK dynamic proxy: target source is SingletonTargetSource for target object [com.otv.rmi.server.RMIUserService@16dadf9]
04.03.2012 14:23:15 INFO (RmiServiceExporter.java:276) - Binding service 'RMIUserService' to RMI registry: RegistryImpl[UnicastServerRef [liveRef: [endpoint:[192.168.1.7:1099](local),objID:[0:0:0, 0]]]]
04.03.2012 14:23:15 DEBUG (AbstractAutowireCapableBeanFactory.java:458) - Finished creating instance of bean 'org.springframework.remoting.rmi.RmiServiceExporter#0'
04.03.2012 14:23:15 DEBUG (AbstractApplicationContext.java:845) - Unable to locate LifecycleProcessor with name 'lifecycleProcessor': using default [org.springframework.context.support.DefaultLifecycleProcessor@3c0007]
04.03.2012 14:23:15 DEBUG (AbstractBeanFactory.java:245) - Returning cached instance of singleton bean 'lifecycleProcessor'04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.addUser
04.03.2012 14:25:43 DEBUG (RMIUserService.java:33) - User has been added to cache. User : Id : 1, Name : Bruce, Surname : Willis
04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.addUser04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.getUserList
04.03.2012 14:25:43 DEBUG (RMIUserService.java:57) - User List : [Id : 1, Name : Bruce, Surname : Willis]
04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.getUserList04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.deleteUser
04.03.2012 14:25:43 DEBUG (RMIUserService.java:45) - User has been deleted from cache. User : Id : 1, Name : Bruce, Surname : Willis
04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.deleteUser04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.getUserList
04.03.2012 14:25:43 DEBUG (RMIUserService.java:57) - User List : []
04.03.2012 14:25:43 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of RmiServiceExporter remote call: com.otv.rmi.server.IRMIUserService.getUserList
步骤13:下载
OTV_SpringRMI
参考: Online Technology Vision博客中的JCG合作伙伴 Eren Avsarogullari的Spring Remoting支持和RMI服务开发 。
翻译自: https://www.javacodegeeks.com/2012/04/spring-remoting-support-and-developing.html
spring rmi
spring rmi_Spring远程支持和开发RMI服务相关推荐
- Spring远程支持和开发RMI服务
Spring远程支持简化了启用远程服务的开发. 当前,Spring支持以下远程技术:远程方法调用(RMI),HTTP调用程序,Hessian,Burlap,JAX-RPC,JAX-WS和JMS. 远程 ...
- 使用 Spring HATEOAS 开发 REST 服务--转
原文地址:https://www.ibm.com/developerworks/cn/java/j-lo-SpringHATEOAS/index.html?ca=drs-&utm_source ...
- Spring Cloud Alibaba基础教程:支持的几种服务消费方式(RestTemplate、WebClient、Feign)
通过<Spring Cloud Alibaba基础教程:使用Nacos实现服务注册与发现>一文的学习,我们已经学会如何使用Nacos来实现服务的注册与发现,同时也介绍如何通过LoadBal ...
- 在ASP.NET Core中使用Apworks开发数据服务:对HAL的支持
HAL,全称为Hypertext Application Language,它是一种简单的数据格式,它能以一种简单.统一的形式,在API中引入超链接特性,使得API的可发现性(discoverable ...
- jax-rs jax-ws_极端懒惰:使用Spring Boot开发JAX-RS服务
jax-rs jax-ws 我认为可以公平地说,作为软件开发人员,我们一直在寻找编写更少的代码的方法,这些代码可以自动地或不能自动地完成更多的工作. 考虑到这一点,作为Spring产品组合的骄傲成员的 ...
- 极端懒惰:使用Spring Boot开发JAX-RS服务
我认为可以公平地说,作为软件开发人员,我们一直在寻找编写较少代码的方法,这些方法可以自动完成或不能自动完成更多工作. 考虑到这一点,作为Spring产品组合的骄傲成员的Spring Boot项目破坏了 ...
- Spring Boot 对基础 Web 开发的支持(下)2-2
接着上一课我们继续讲解. 数据校验 在很多时候,当我们要处理一个应用程序的业务逻辑时,数据校验是必须要考虑和面对的事情.应用程序必 须通过某种手段来确保输入进来的数据从语义上来讲是正确的.在 Java ...
- 使用 Spring HATEOAS 开发 REST 服务
使用 Spring HATEOAS 开发 REST 服务 Comments 1 绝大多数开发人员对于 REST 这个词都并不陌生.自从 2000 年 Roy Fielding 在其博士论文中创造出来这 ...
- 猿创征文 | 微服务 Spring Boot 整合Redis 实战开发解决高并发数据缓存
文章目录 一.什么是 缓存? ⛅为什么用缓存? ⚡如何使用缓存 二.实现一个商家缓存 ⌛环境搭建 ♨️核心源码 ✅测试接口 三.采用 微服务 Spring Boot 注解开启缓存 ✂️@CacheEn ...
最新文章
- 关于FluentNhibernate数据库连接配置,请教
- [考试]20151013搜索
- python培训中心-深圳Python培训
- 怎样把SharePoint中文备份恢复到英文版,修改sharepoint站点语言
- 《互联网运营智慧》十一月进展
- php如何让字母加1,如何使用PHP以任何顺序(从12个字母组成6个单词组成一个字母)进行字符搜索?...
- python 残差图_python 残差图
- endnote能自动翻译吗_自动挡和手自一体有啥区别?从外表能看出一辆车是哪种变速箱吗?...
- @程序员:你的颈椎和你的代码一样有救了
- java需求分析和设计,附面试题
- python第二十二课——list函数
- Pannellum:实例之为全景图添加标题和作者
- 融云 SDK 5.0.0 功能迭代
- 如何删除Word文档中的空白页
- 又是一次数据分析的例子(自杀分析)
- ORACLE 11GR2 配置GATEWAY FOR SERVER 问题
- 什么是抽象类?什么是抽象方法?
- JavaScript 和读取服务器cookie
- 9_1 法律法规标准化
- 简单工厂/工厂方法/抽象工厂
热门文章
- java实现人脸识别源码【含测试效果图】——实体类(Users)
- 配置struts.xml时extends=struts-default会报错,原因和解决
- linux 编译 expat,关于expat库的编译
- win 7 mysql 1067_win7系统登陆MySQL服务出现1067错误的解决方法
- IDEA开启Run Dashboard窗口
- Navicat Premium 11 12 闪退
- 16-1 Redis分布式缓存引入与保存缓存功能实现
- 亚信科技笔试面试2019届
- idea tomcat部署web项目_项目开发之部署帆软到Tomcat服务一
- 如何确定python开发环境已经配置好_搭建 python 开发环境 前面安装选位置我直接回车了现在我想测试查看目录该怎么办...