wordpress api_掌握WordPress分类API
wordpress api
In a previous SitePoint article, I provided an introduction to the WordPress Categories API thanks to the function wp_list_categories()
. As we saw, this function is full of different options, and that’s why it’s a good idea to use it if we want to display a list of our categories.
在上一篇SitePoint文章中,由于功能wp_list_categories()
,我提供了WordPress Categories API的简介 。 正如我们所看到的,此函数包含许多不同的选项,因此,如果要显示类别列表,最好使用它。
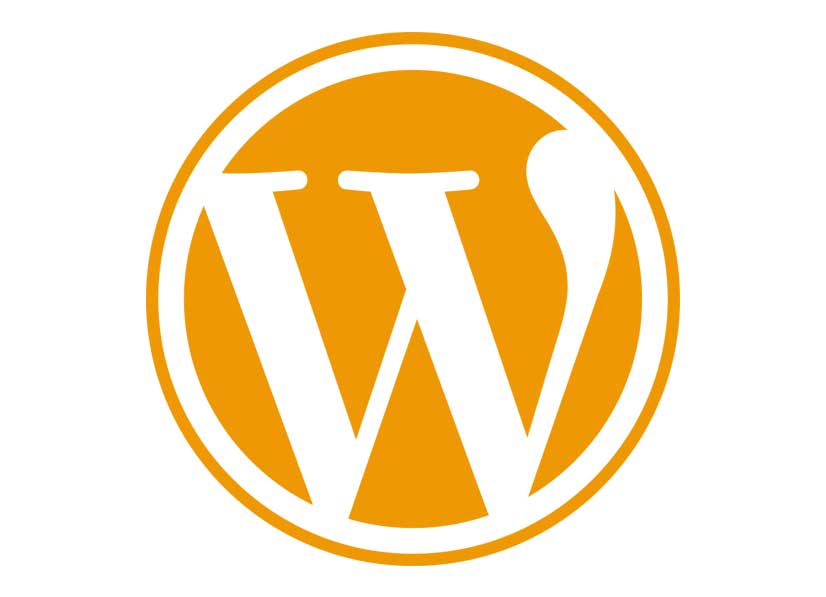
However, it can’t cover all the possible cases, and that’s the reason why we find other functions in the Categories API. These functions allow us to retrieve raw data: in place of a HTML list of our categories, we can retrieve arrays containing these same categories.
但是,它无法涵盖所有可能的情况,这就是为什么我们在Categories API中找到其他功能的原因。 这些函数使我们能够检索原始数据:代替类别HTML列表,我们可以检索包含这些相同类别的数组。
The consequence is that it’s simpler (and proper!) to display this data in a special way, whatever you can imagine as a DOM tree. We will begin here with functions that return objects containing all data relative to our categories. Then, we’ll be interested in functions that only return specific information.
结果是,以特殊的方式显示此数据更加简单(也很合适!),无论您将其想象成DOM树如何。 我们将从此处的函数开始,这些函数返回包含与类别相关的所有数据的对象。 然后,我们将对仅返回特定信息的函数感兴趣。
检索某些类别作为对象 (Retrieving Some Categories as Objects)
Like links in the Links Manager, we can retrieve our categories as objects that contain all of their properties. We’ll begin by describing these objects thanks to a function which only returns one category. Then, we’ll use our newly acquired knowledge to play with all the categories we want!
像“链接管理器”中的链接一样,我们可以将类别检索为包含其所有属性的对象。 我们将通过一个仅返回一个类别的函数来描述这些对象。 然后,我们将使用我们新获得的知识来处理我们想要的所有类别!
检索具有其ID的给定类别 (Retrieving a given Category with Its ID)
The first function we’ll cover here is get_category()
. Basically, it allows you to get an object representing the category you pass the ID in as a parameter.
我们将在这里介绍的第一个函数是get_category()
。 基本上,它允许您获得一个对象,该对象表示将ID作为参数传递的类别。
$cat = get_category(14);
Then, $cat
contains the wanted object. We’ll now describe some of its properties that are of interest to us.
然后, $cat
包含所需对象。 现在,我们将描述一些我们感兴趣的属性。
类别编号 (Categories ID’s)
First, the ID of the category we wanted. You can find this value in the cat_ID
property.
首先,我们想要的类别的ID。 您可以在cat_ID
属性中找到该值。
$id = $cat->cat_ID;
Note that this value is not very useful here, as we already knew the ID (we passed it as the parameter of the function!). But we’ll see below that there are other ways to retrieve a category: in that case, knowing how to get the ID in a category object can be useful.
请注意,此值在这里不是很有用,因为我们已经知道ID(我们将其作为函数的参数传递了!)。 但是我们将在下面看到还有其他检索类别的方法:在这种情况下,了解如何在类别对象中获取ID可能很有用。
If your category is a child of another one, you can also retrieve the ID of its parent thanks to the property category_parent
. Note that if your category has no parent, this value is set to 0
.
如果您的类别是另一个类别的子类别,则还可以使用category_parent
属性检索其父category_parent
的ID。 请注意,如果您的类别没有父类别,则此值设置为0
。
获取所有数据! (Get ALL the Data!)
When we display information about a category, one essential piece of information is its name. You can retrieve it thanks to the property cat_name
. In the same way, you can retrieve the category’s description thanks to category_description
.
当我们显示有关类别的信息时,一条必不可少的信息就是其名称。 您可以通过属性cat_name
检索它。 以同样的方式,你可以检索类别的描述感谢category_description
。
<h1><?php echo $cat->cat_name; ?></h1>
<p><?php echo $cat->category_description; ?></p>
The ID is not the unique identifier of a category: the slug is another one, in the shape of a string (generally the name of the category itself, in lowercase, without spaces or special characters). You can retrieve the slug with the property category_nicename
, or slug
(both are valid).
ID不是类别的唯一标识符:该段是字符串形式的另一个(通常是类别本身的名称,小写,没有空格或特殊字符)。 您可以使用属性category_nicename
或slug
(两者均有效)来检索该Slug。
Finally, you can also see how many posts are contained into this category thanks to the property category_count
. Note that this counter doesn’t take into account the number of posts we can found in children categories.
最后,由于属性category_count
,您还可以查看此类别包含多少个帖子。 请注意,此计数器未考虑我们在子类别中可以找到的帖子数。
我对你撒谎 (I Lied to You)
The title of this part is a lie. In fact, objects are not the only available representation of our categories. You can also choose to retrieve them in arrays, thanks to a second argument given to get_category()
.
这部分的标题是谎言。 实际上,对象并不是我们类别的唯一可用表示。 您还可以选择以数组形式检索它们,这要归功于get_category()
提供的第二个参数。
// Get an object (default behavior)
$cat = get_category(15, OBJECT);
// Get an associative array
$cat = get_category(15, ARRAY_A);
// Get an numbered array
$cat = get_category(15, ARRAY_N);
The keys in the associative array are the properties of the object. However, things are more complicated in the numbered array: the order is the same, but you can’t have clear indexes.
关联数组中的键是对象的属性。 但是,编号数组中的情况更加复杂:顺序相同,但是您无法获得清晰的索引。
检索没有其ID的类别 (Retrieving a Category Without Its ID)
As we said above, ID is not the only identifier of a category, and the slug of a category can also be used to retrieve its information. This can be achieved with the function get_category_by_slug()
. As you can guess, it accepts the slug as a parameter.
如上所述,ID不是类别的唯一标识符,类别的标签也可以用于检索其信息。 这可以通过功能get_category_by_slug()
来实现。 如您所料,它接受了slug作为参数。
$cat = get_category_by_slug('my-goldfish');
We won’t describe the returned object, as it’s the same as we had with get_category()
. However, you don’t have a choice here: object is the only available type and you won’t be able to retrieve an array with this function. Finally, note that get_category_by_slug()
returns false
if there is no category with the indicated slug.
我们不会描述返回的对象,因为它与get_category()
。 但是,这里您别无选择:object是唯一可用的类型,您将无法使用此函数检索数组。 最后,请注意,如果没有带有指示的子类别的类别,则get_category_by_slug()
返回false
。
检索几个类别 (Retrieving Several Categories)
We’ll see two functions to get different lists of categories. The first function allows you to retrieve any list of categories, while the second will help you retrieve the categories associated to a given post.
我们将看到两个函数来获取不同的类别列表。 第一个功能允许您检索类别的任何列表,而第二个功能将帮助您检索与给定帖子关联的类别。
获取您想要的所有类别 (Get All the Categories You Want)
If you read the previous part you won’t be disoriented by the returned values of get_categories()
, as it returns an array listing all the wanted categories, each represented by an object which is exactly the one we described above.
如果您阅读了上一部分,则不会因get_categories()
的返回值而迷失方向,因为它会返回一个列出所有所需类别的数组,每个类别均由一个对象表示,该对象正是我们上面所述的对象。
Without any argument, this function returns all the categories you created. If you write the line below:
没有任何参数,此函数将返回您创建的所有类别。 如果您在下面写这行:
$cats = get_categories();
then $cats
is an array containing all your categories, in alphabetical order. As an example, if I want to retrieve the name of the second category, all I have to write is $cats[1]->cat_name
(see the previous part about the category object for more info).
那么$cats
是一个包含您所有类别的数组,按字母顺序排列。 例如,如果要检索第二个类别的名称,我只需要写$cats[1]->cat_name
(有关更多信息,请参阅上一部分的类别对象)。
If you did a print_r()
on the $cats
variable, maybe you noticed something weird: in some cases, this array has some “holes”. For example, we can directly step from index 0
to 4
, without seeing 1
, 2
and 3
. What happened? What is this weird way of counting?
如果在$cats
变量上执行了print_r()
,也许您会注意到一些奇怪的东西:在某些情况下,此数组有一些“漏洞”。 例如,我们可以直接从步骤索引0
至4
,没有看到1
, 2
和3
。 发生了什么? 这种奇怪的计数方法是什么?
Well, in fact, this is not weird: if you effectively noticed this “bug”, maybe you also noticed that the missing categories are exactly the ones which are empty: by default, WordPress hides the empty categories. Hurray, it’s not a bug!
好吧,事实上,这并不奇怪:如果您有效地注意到了这个“错误”,也许您还注意到丢失的类别恰好是空的:默认情况下,WordPress隐藏了空类别。 万岁,这不是错误!
We can modify this behavior, along with some other things we can customize thanks to the argument we can send to get_categories()
. As usual, this unique argument is an array containing all the options we want.
由于可以发送给get_categories()
的参数,我们可以修改此行为以及可以自定义的其他一些东西。 像往常一样,此唯一参数是一个包含我们想要的所有选项的数组。
I recommend you to read our previous article about the Categories API, where we described wp_list_categories()
. In fact, (almost) all the available options for get_categories()
are options we saw for wp_list_categories()
, so we won’t describe what they do twice.
我建议您阅读我们先前有关Categories API的文章 ,其中描述了wp_list_categories()
。 实际上,(几乎) get_categories()
)的所有可用选项都是我们在wp_list_categories()
看到的选项,因此我们不会get_categories()
描述它们的作用。
As for wp_list_categories()
you can choose the order you want with orderby
and order
, limit the number of returned categories with number
or again choose to get the empty categories with hide_empty
. You also have access to the options include
and exclude
to get the right categories.
至于wp_list_categories()
您可以使用orderby
和order
选择想要的order
,使用number限制返回类别的number
或者再次使用hide_empty
选择获取空类别。 您还可以访问include
和exclude
选项以获取正确的类别。
Maybe the hierarchical
option deserves an explanation: set by default to 1
, you can cancel its effects by setting it to 0
. Set to 1
, this option can return some empty categories, even if hide_empty
is set to 1
. However, you’ll only see empty categories with children categories which are not empty (that way you can build the entire tree).
也许hierarchical
选项值得解释:默认设置为1
,可以通过将其设置为0
来取消其效果。 设置为1
,此选项可以返回一些空的类别,即使hide_empty
设置为1
。 但是,您只会看到带有子类别的空类别,这些子类别不为空(这样您就可以构建整个树)。
The pad_counts
option is still here, so is the child_of
one. But a new option is here: parent
which is like child_of
, with the difference that parent
only returns the direct children of the selected category: if these children themselves have children categories, they won’t be returned.
pad_counts
选项仍在此处, child_of
也是。 但是,这里有一个新选项: parent
类似于child_of
,区别在于parent
仅返回所选类别的直接子项:如果这些子项本身具有子项类别,则不会返回它们。
获取与帖子相关的类别 (Get Categories Associated to a Post)
You can associate more than one category to a post. When you use get_the_category()
, you can retrieve an array listing of all the categories you set for a given post.
您可以将多个类别关联到帖子。 使用get_the_category()
,您可以检索为给定帖子设置的所有类别的数组列表。
By default, this function returns all the categories associated with the current post (you have to be in the loop to enable this behavior). However, you can retrieve the categories associated to any post by passing its ID as a parameter.
默认情况下,此函数返回与当前帖子关联的所有类别(您必须处于循环中才能启用此行为)。 但是,您可以通过传递其ID作为参数来检索与任何帖子关联的类别。
// Get the categories of the post with ID 139
$cats = get_the_category(139);
检索某些特定数据 (Retrieving Some Specific Data)
Sometimes, we just need specific information, like the name of a category, or its ID. In these cases, retrieving an entire object to get only one lot of information is useless. That’s why there are some other functions for these specific needs.
有时,我们只需要特定信息,例如类别名称或其ID。 在这些情况下,检索整个对象仅获取大量信息是没有用的。 这就是为什么还有其他一些功能可以满足这些特定需求的原因。
检索类别的ID (Retrieving the ID of a Category)
It’s always easier to remember the name of a category instead of its ID (which is never the same following the installation). The good news is that you can retrieve the ID of a category if you know its name. To do that we can use the get_cat_ID()
function: you pass it the name of a category and it returns its ID. If no category was found, the returned value is 0
.
记住类别名称而不是其ID总是更容易(安装后再也不会一样)。 好消息是,如果您知道类别的名称,则可以检索该类别的ID。 为此,我们可以使用get_cat_ID()
函数:将类别的名称传递给它,然后返回其ID。 如果未找到类别,则返回值为0
。
// Yes, I love this category
$cat_id = get_cat_ID('My goldfish');
If you already have the ID of a category but don’t know the IDs of its parents you can also use get_ancestors()
. It returns an array containing all the ancestors’ IDs, from the nearest to the farthest.
如果您已经具有某个类别的ID,但不知道其父类别的ID,则也可以使用get_ancestors()
。 它返回一个数组,其中包含从最近到最远的所有祖先的ID。
An example will be clearer. Assume that we have “My goldfish” (with ID 15) as a child category of “My life” (with ID 14). Now we add a child to “My goldfish“: “The feed of my goldfish” (with ID 16). Then the following line:
一个例子将更加清楚。 假设我们将“ 我的金鱼 ”(ID为15)作为“ 我的生活 ”(ID 14)的子类别。 现在,我们在“ 我的金鱼 ”中添加一个孩子:“ 我的金鱼的饲料 ”(ID为16)。 然后下一行:
$cats_id = get_ancestors(16, 'category');
returns this:
返回此:
Array
(
[0] => 15
[1] => 14
)
Note that we have to indicate to get_ancestors()
that we’re talking about categories. In fact, this function is more general and can include more fields than categories (but it’s not what we’re interested in here).
注意,我们必须向get_ancestors()
表示我们正在谈论类别。 实际上,此功能更通用,可以包含比类别更多的字段(但这不是我们在此感兴趣的内容)。
检索一些字符串 (Retrieving Some Strings)
We can retrieve the ID of a category from its name, but we can also do the opposite: that’s what get_cat_name()
does.
我们可以从类别的名称中检索类别的ID,但我们也可以执行相反的操作:这就是get_cat_name()
作用。
// Returns the name of the category with ID 15
$cat_name = get_cat_name(15);
The same thing can be done with the description of a category, thanks to category_description()
.
多亏了category_description()
,使用类别的描述也可以完成同样的事情。
// Returns the description of the category with ID 15
$cat_desc = category_description(15);
Did you notice that, since the beginning of this article, we haven’t talked about the URL of a category? It’s an interesting topic, as its shape can change following the installation (URL Rewriting? No URL Rewriting?). To be honest, I don’t understand why this information is not contained into the object we described above. However, we can retrieve the URL of a category thanks to get_category_link()
.
您是否注意到,自从本文开始以来,我们没有谈论类别的URL? 这是一个有趣的主题,因为其形状会在安装后发生变化(URL重写?是否没有URL重写?)。 老实说,我不明白为什么我们上面描述的对象中没有包含此信息。 但是,由于get_category_link()
,我们可以检索类别的URL。
// I want to display a link to my favorite category
$cat_id = get_cat_ID('My goldfish');
$cat_link = get_category_link($cat_id);
Then all I have to do is using $cat_link
which contains the right URL.
然后,我要做的就是使用包含正确URL的$cat_link
。
<a href="<?php echo $cat_link; ?>">My goldfish</a>
短名单 (Short Lists)
If you’ve been developing WordPress themes, chances are that you already know the_category()
. This function allows you to echo a list of the categories used by the current post (or any other). This list is a string, with all the links one after the other, separated by the string you want (indicated in the first parameter).
如果您一直在开发WordPress主题,则the_category()
可能已经知道the_category()
。 此功能允许您回显当前帖子(或任何其他帖子)使用的类别列表。 此列表是一个字符串,所有链接都一个接一个,由所需的字符串分隔(在第一个参数中指示)。
// Get a comma-separated list of the used categories
the_category(', ');
The third parameter of this function is the post ID we want: if you’re not in the loop, you must use this parameter. The second parameter is quite particular.
此函数的第三个参数是我们想要的帖子ID:如果您不在循环中,则必须使用此参数。 第二个参数非常特殊。
It allows you to control the way relationships between parents and children are displayed and is useful only in the case where the concerned post is in a category that has a parent category. By default this parameter is set to an empty string and only the child category is shown. If you change this to single
, then the name of the parent category will also be displayed, in the same link of its child. Finally, you can also choose multiple
: with this value, the parent and the child category are shown, in two separate links.
它使您可以控制显示父母与孩子之间的关系的方式,并且仅在相关帖子处于具有父类别的类别中时才有用。 默认情况下,此参数设置为空字符串,并且仅显示子类别。 如果将其更改为single
,则父类别的名称也会显示在其子类别的同一链接中。 最后,您还可以选择multiple
:使用此值,在两个单独的链接中显示父类别和子类别。
If you prefer storing the result into a variable before echoing it, then you can use get_the_category_list()
instead (it can be used in the same way than the_category()
).
如果您希望在回显结果之前将结果存储到变量中,则可以使用get_the_category_list()
代替(可以使用与the_category()
相同的方式)。
结论 (Conclusion)
The Categories API is a very comprehensive, it contains many very useful functions. Whatever you need when you want to play with categories, chances are great that a function already exists.
Categories API是非常全面的,它包含许多非常有用的功能。 想要玩类别游戏时,无论您需要什么,很有可能已经存在一个功能。
In fact, I chose to describe some of the most commonly used functions, but this API contains a large number of others. For example, there’s a function to check if a post is in a category. There’s also a function that displays a list composed by checkboxes for every category (useful for administration panels).
实际上,我选择描述一些最常用的功能,但是此API包含大量其他功能。 例如,有一个功能可以检查帖子是否在类别中。 还有一个功能可以显示由每个类别的复选框组成的列表(对管理面板有用)。
Describing all of them will be more indigestible than anything else. That’s why, if you can’t find the function in this article, don’t hesitate to ask for help below.
描述所有这些将比其他任何东西都更加难懂。 这就是为什么如果您在本文中找不到该函数,请随时在下面寻求帮助。
翻译自: https://www.sitepoint.com/mastering-wordpress-categories-api/
wordpress api
wordpress api_掌握WordPress分类API相关推荐
- wordpress 独立 php,wordpress怎么把所有文章分类单独在一个页面显示
如何让wordpress把所有文章分类单独在一个页面显示? 1.复制一个page.php文件改为page-abc.php,并在WordPress后台新建一个页面,固定链接地址改为abc(这个abc可随 ...
- woocommerce分类页面模板_怎样让wordpress网站的不同分类页面,调用不同的banner图片?...
在我们的wordpress网站模板开发中,我们可能有这样的一个需求,就是能在自己的wordpress网站的不同的分类目录页面,能调用不同的banner图片.比如:医院类网站,医院科室比较多,男科分类调 ...
- wordpress 首页调用指定分类文章_怎样给wordpress网站分类目录页面,添加文章列表和分页效果?...
在前面的章节中,我们完成了wordpress网站首页模板数据的调用,也创建好了wordpress网站的公共模板.今天,我们再来给wordpress网站的分类目录页模板archive.php文件添加左侧 ...
- WordPress CMS百度快速收录 API 提交代码以及配置教程
百度快速收录的提交代码,百度快速收录的功能上线,是全面继承百度移动专区天级收录功能,并且天级提交功能于 5 月 18 日已经暂停使用了. 污水流量计 使用方法 将下面代码添加到你的 WordPress ...
- WordPress站点健康提示REST API遇到了错误
最近有几个Autumn-Pro主题用户反馈来一个问题,网站后台提示REST API遇到了错误. 虽然提示REST API遇到了错误,但测试API接口的时候还是可以正常使用的. 经过排查,我发现Word ...
- code-server、docker-compose安装wordpress+mysql、wordpress公式插件、markdown插件、目录插件、调序插件、统计插件、分享点赞打赏插件
文章目录 缘起 一.code-server 1.1 code-server简介 1.2 code-server安装和启动 1.3 code-server安全配置 1.3.1 域名申请和备案 1.3.2 ...
- Wp模板,免费WordPress模板,WordPress插件详解
Wp模板,最近很多站长问我有没有对SEO优化友好的WordPress模板主题.不要问有没有WordPress模板主题,问就是有wp模板主题.但是我们站长如果想做好SEO优化,我们光有"漂亮& ...
- wordpress采集插件-wordpress关键词插件-wordpress百度推送插件-wordpress蜘蛛统计分析插件
wordpress插件,最近很多站长问我有没有好用的wordpress插件热门插件推荐.主要是针对SEO优化这块的wordpress插件.wordpress采集插件.wordpress热门关键词插件. ...
- Oracle EBS FA重分类API调用示例
Oracle EBS FA重分类API调用示例 fa_reclass_pub.do_reclass declare l_trans_rec FA_API_TYPES.t ...
- 网课查题php接口,题库API_大学题库API网课查题题库接口API-在线授权
题库API_大学题库API网课查题题库接口API-在线授权 更多相关问题 阅读理解. Sunny English Club For students16:00 ~ 18:00Every Saturda ...
最新文章
- 多伦多大学和清华大学创新创业论坛,数据科学研究院建言献策
- 有程序在记录你的键盘输入_12个用Java编写基础小程序amp;经典案例(收藏)
- solaris查看主机信息
- python继承实现原理封装property
- 如何做到免驱打印_证卡打印机云打印方案
- 算法:Find First and Last Position of Element in Sorted Array(在有序数组中搜索第一个和最后一个找到的数字)
- 绘制直方图,计算CPK、PPK等数据
- systemverilog随机函数
- 苹果宣布前CEO史蒂夫·乔布斯逝世
- 2020微信小程序学习报告.2.17-3.1.(一)
- 一阶电路暂态响应的结果分析。_第八讲 线性电路的过渡过程分析二
- 重装win7系统后打开截图工具显示“截图工具当前未在计算机上运行”怎么办
- Android fastboot 基本操作命令(Android 刷机)
- 分子间相互作用——偶极作用dipolar-dopolar interaction
- 谈谈企业管理软件领域内那些很难稳定重现故障的处理技巧
- 接收Request请求Bearer令牌参数
- visio2019中文版(64位)
- 微擎在平台入口不显示安装的模块_【微擎系统】2.0.8更新说明
- 大数据专业怎么样?是什么?
- Arduino ESP32 flash数据存储结构
热门文章
- 《Data Algorithm》读书笔记七 — 购物篮分析
- 语言教案 小小计算机,大班语言小小播音员游戏教案
- 微信服务号开发说明:测试号申请、自定义菜单添加第三方连接
- python 大小写字母怎么用数字表示_python判断字符串是字母 数字 大小写(转载)...
- Greedy 贪心算法
- POI实现超大数据的Excel的读写操作,支持Excel最大行数。
- c语言列宽作用,c语言|格式化输入输出详解
- 苏宁易购执行总裁任峻在IT体系年会上的讲话
- robotframework 图片校验
- 【数学】有理分式的拆解技巧