(原創) 我的Design Pattern之旅[3]:使用template改進Strategy Pattern (OO) (Design Pattern) (C/C++) (template)...
在strategy pattern中,為了讓各strategy能方便存取原來物件的所有public member function,我們常用*this將整個物件傳給各strategy,這樣的設計並沒有什麼不好,但各strategy和原物件過於tight coupling,導致各strategy難以再和其他各物件搭配,本文使用template解決此問題。
在(原創) 我的Design Pattern之旅:Strategy Pattern (初級) (Design Pattern) (C++) (OO C++) (Template C++)中,我們使用了strategy pattern讓Grapher能畫Triangle、Circle和Square
因為需求再次改變,:D,我們希望Grapher能將文字印在各Shape中,執行結果如下
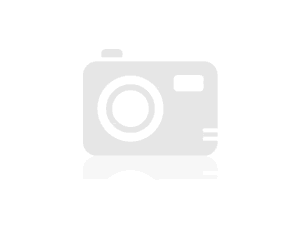
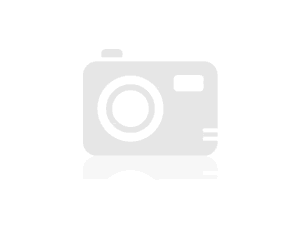
為了達到此需求,我們可以將IShape interface改成
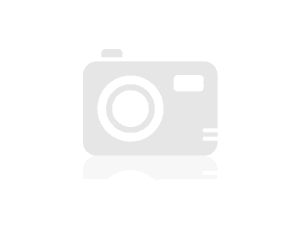
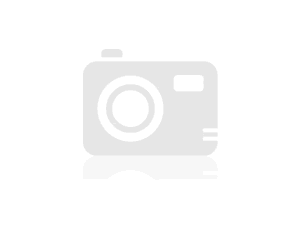



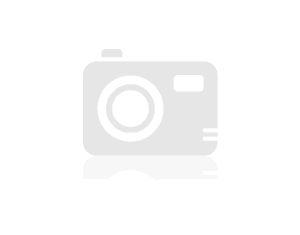
但若將來需求再改變,希望畫的不是文字,而是一張圖片,那interface又必須再變動,為了一勞永逸,我們會將整個物件傳給各strategy,IShape interface改成如下
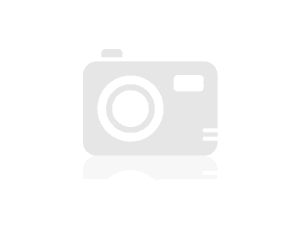
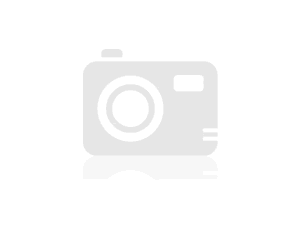



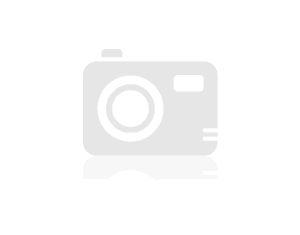
Grapher::drawShpae()將*this傳給各strategy
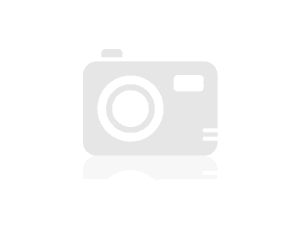
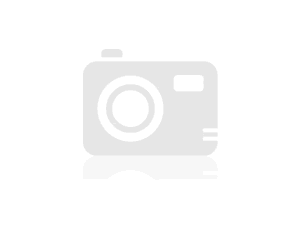


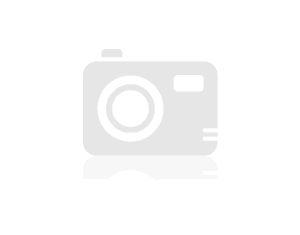
完整的程式碼如下
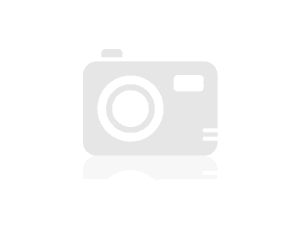
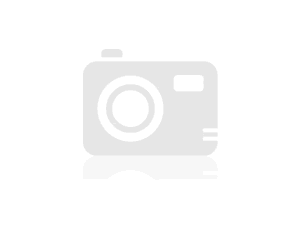
2

3

4

5

6

7

8
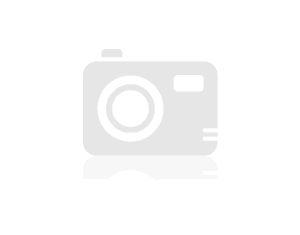
9
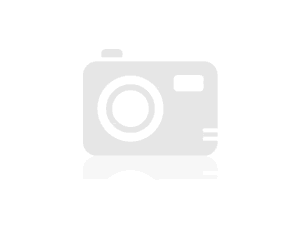
10
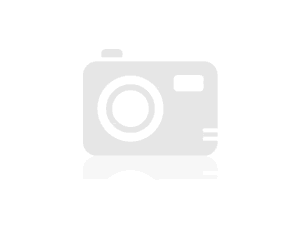
11
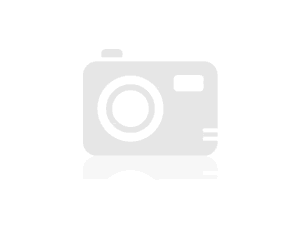
12
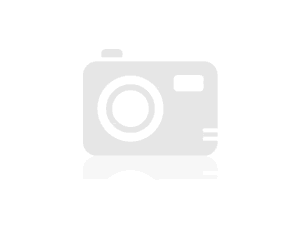
13
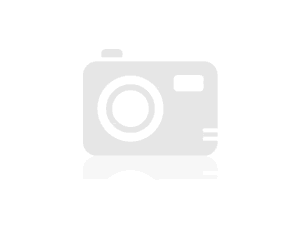
14
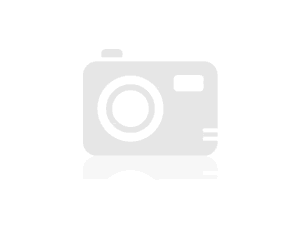
15
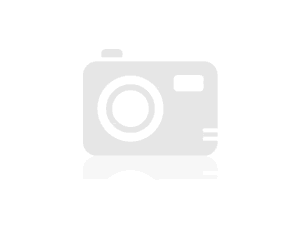
16
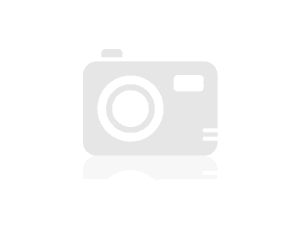
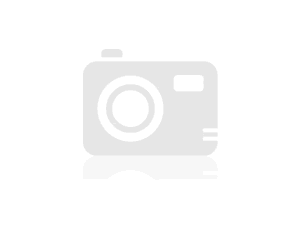

17

18

19
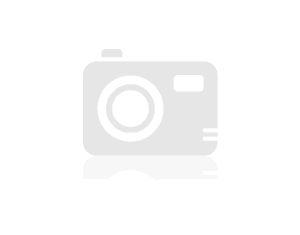
20
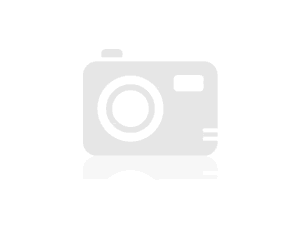
21
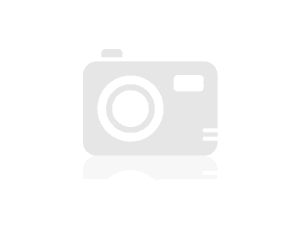
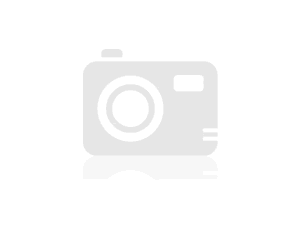

22

23

24

25

26

27

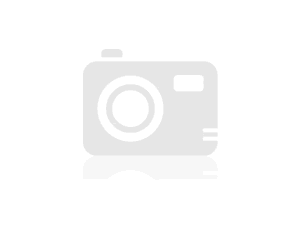

28

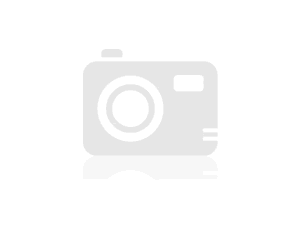

29

30

31

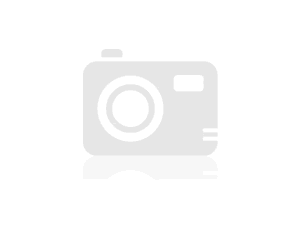

32

33

34

35

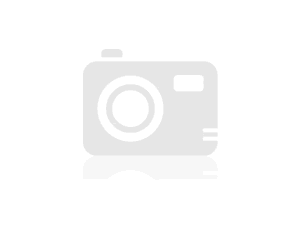

36

37

38

39

40

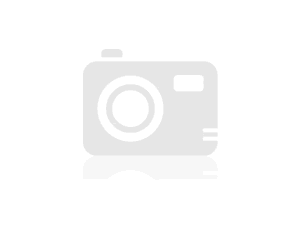

41

42

43
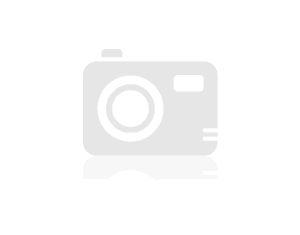
44
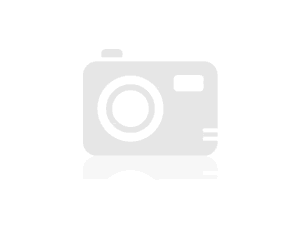
45
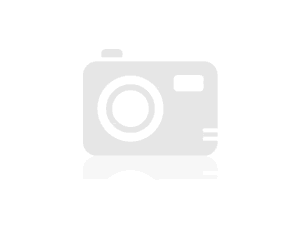
46
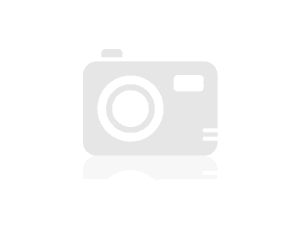
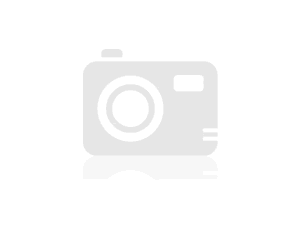

47

48

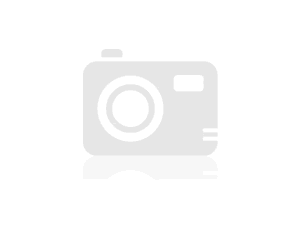

49

50

51
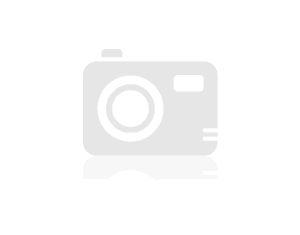
52
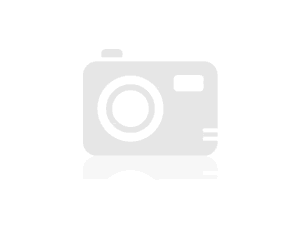
53
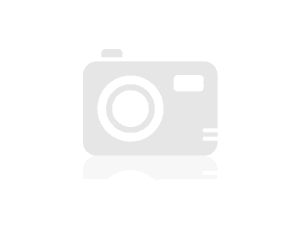
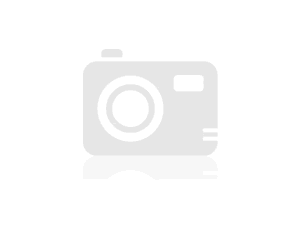

54

55

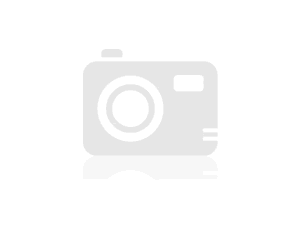

56

57

58
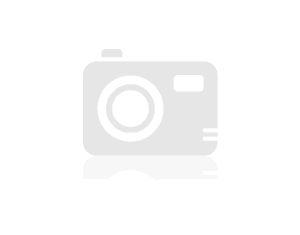
59
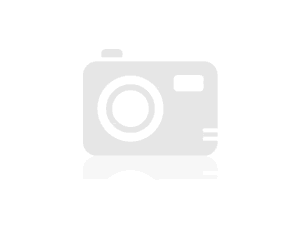
60
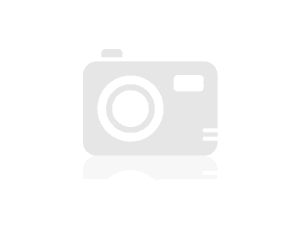
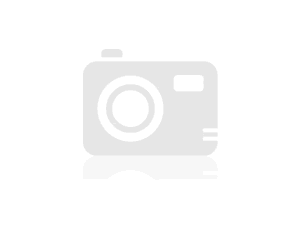

61

62

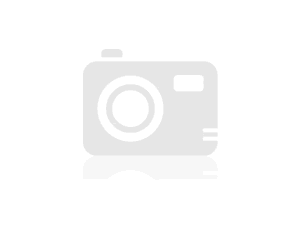

63

64

65
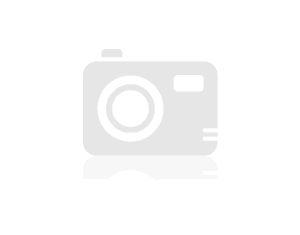
66
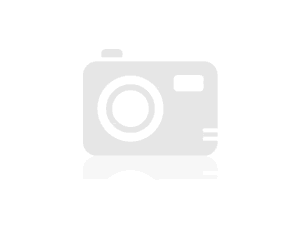
67
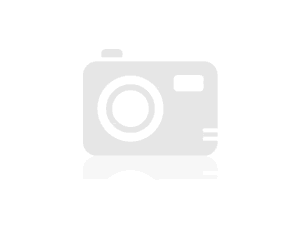
68
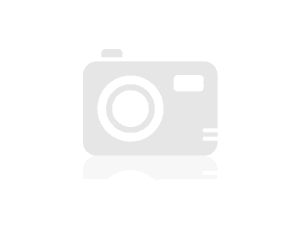
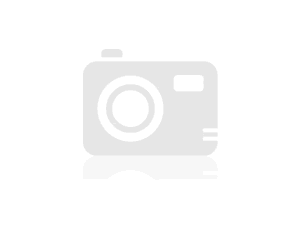

69

70

71

72

73

74
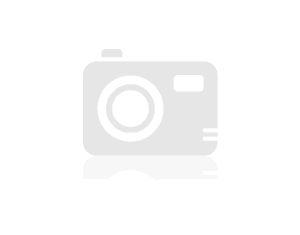
執行結果
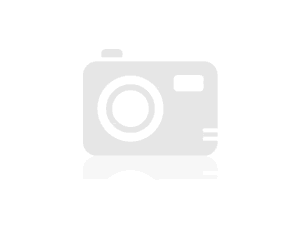
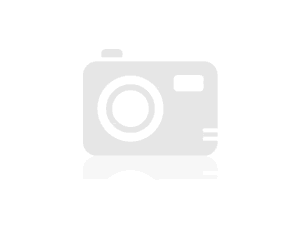
這樣的設計看似完美,但IShape和Grapher相依程度太高,若將來有個Painter class,和Grapher完全不同,沒有任何繼承或多型的關係,但想重複使用IShape interface的strategy,這樣的設計就無法讓Painter使用了。若我們能讓IShape interface的draw()不再只限定Grapher型別,改用template,就能讓將來所有型別都能使用IShape interface。
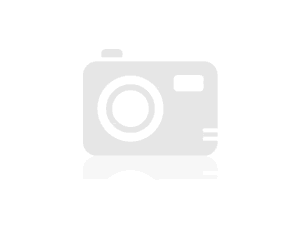
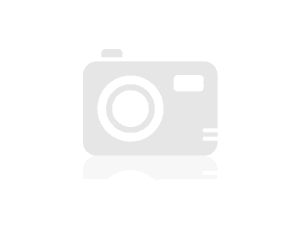
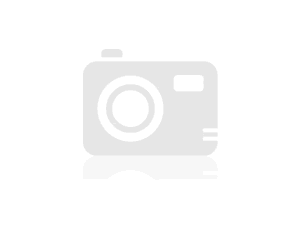



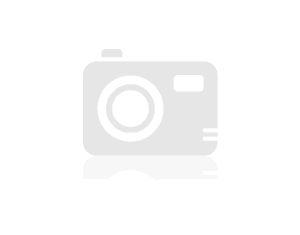
我們用泛型T取代了Grapher,任何型別都可傳進IShape::draw()。
完整程式碼如下
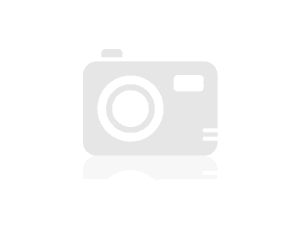
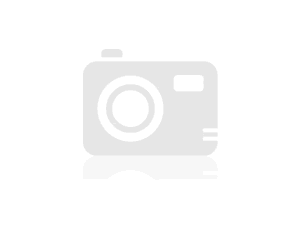
2

3

4

5

6

7

8
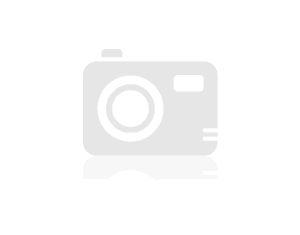
9
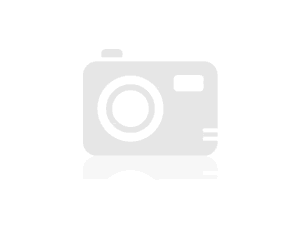
10
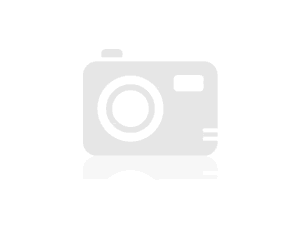
11
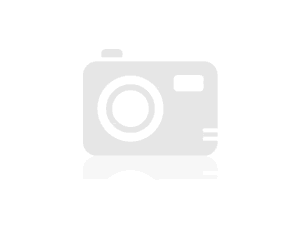
12
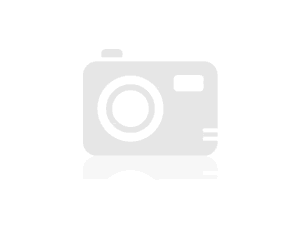
13
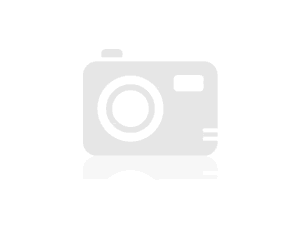
14
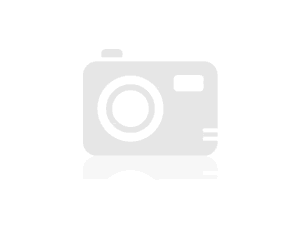
15
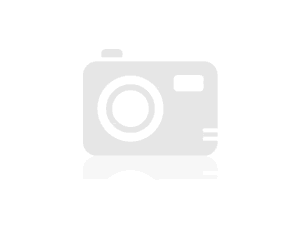
16
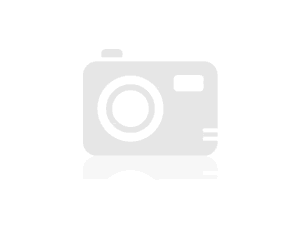
17
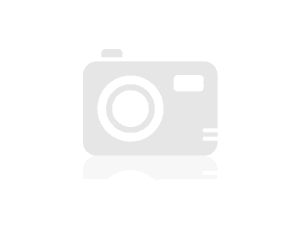
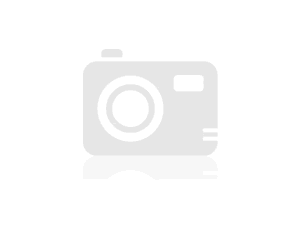

18

19

20
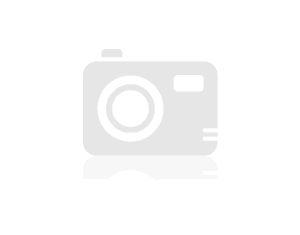
21
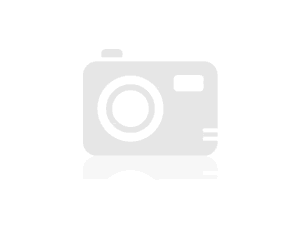
22
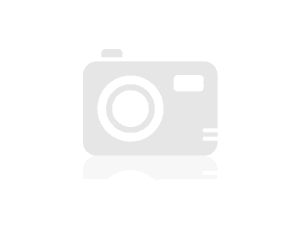
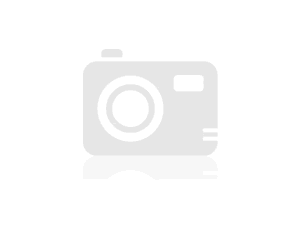

23

24

25

26

27

28

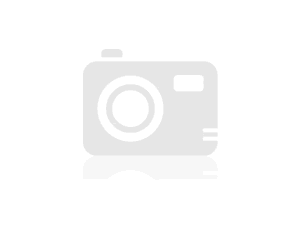

29

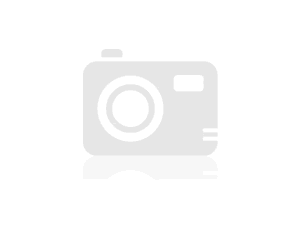

30

31

32

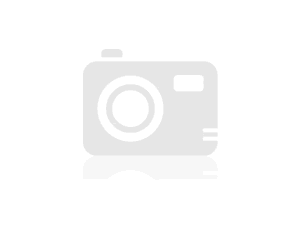

33

34

35

36

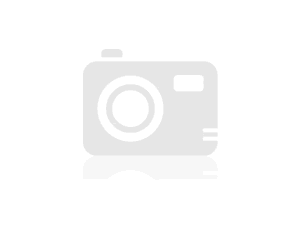

37

38

39

40

41

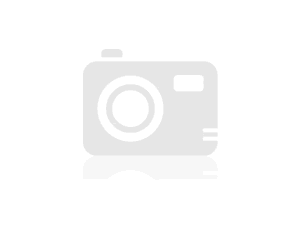

42

43

44
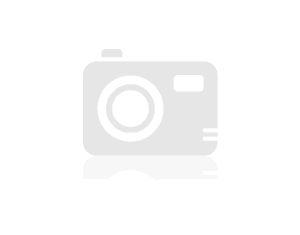
45
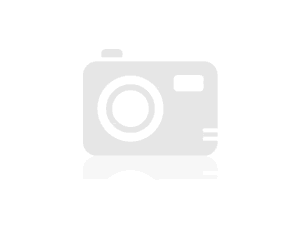
46
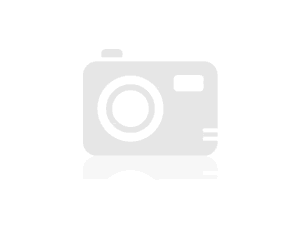
47
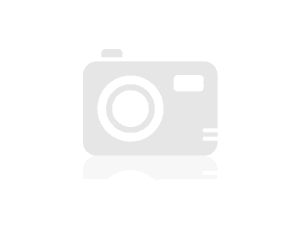
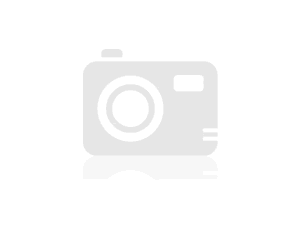

48

49

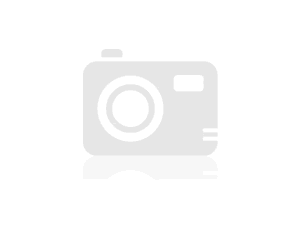

50

51

52
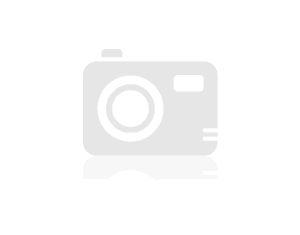
53
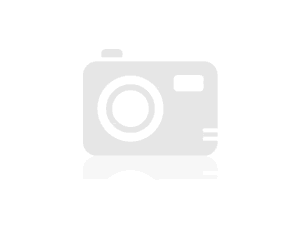
54
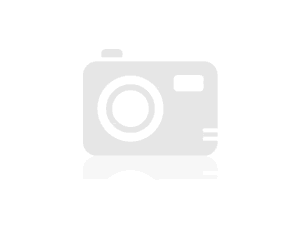
55
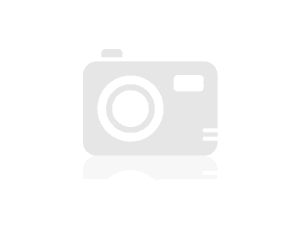
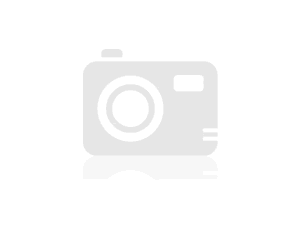

56

57

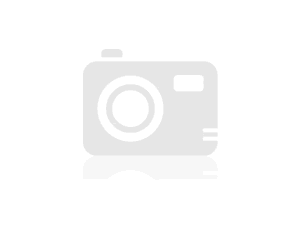

58

59

60
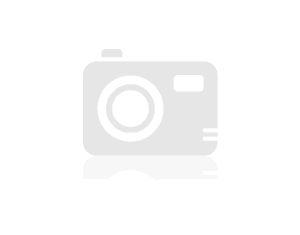
61
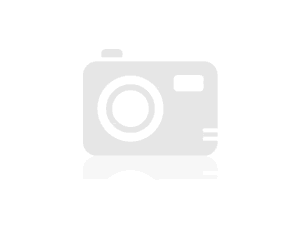
62
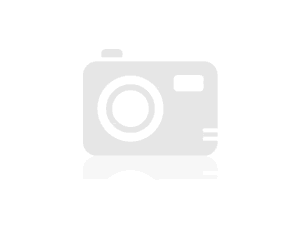
63
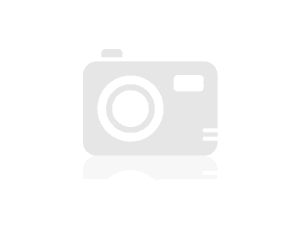
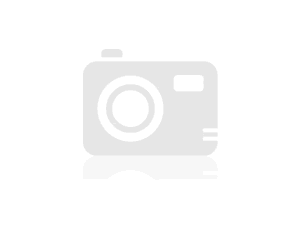

64

65

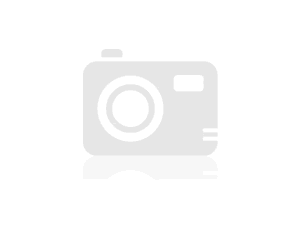

66

67

68
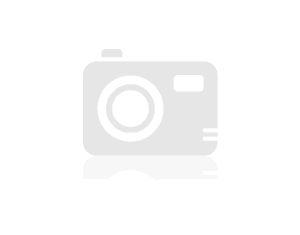
69
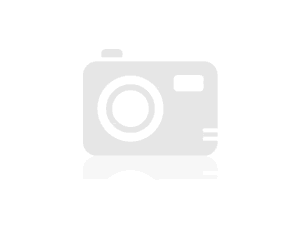
70
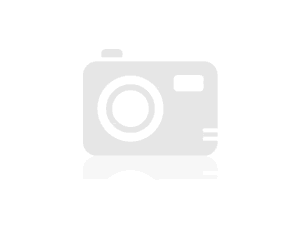
71
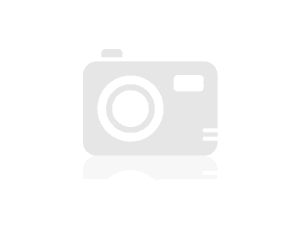
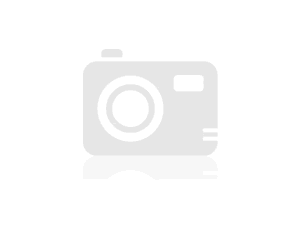

72

73

74

75

76

77
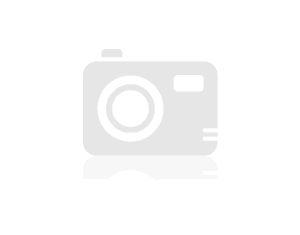
執行結果
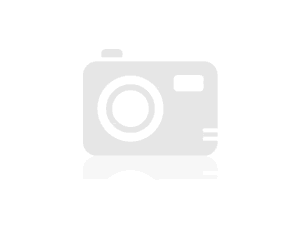
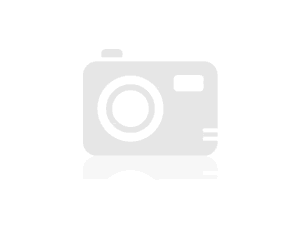
Conclusion
泛型的應用相當廣,在此範例僅僅是泛型的小小應用,在OOP世界使用strategy pattern,常將*this傳給strategy,若搭配GP可讓strategy pattern的resuse程度更高。
See Also
(原創) 我的Design Pattern之旅[1]:Strategy Pattern (初級) (Design Pattern) (C++) (OO C++) (Template C++)
(原創) 我的Design Pattern之旅[4]:使用Generic改進Strategy Pattern (高級) (Design Pattern) (C#) (Generic)
(原創) 我的Design Pattern之旅[3]:使用template改進Strategy Pattern (OO) (Design Pattern) (C/C++) (template)...相关推荐
- (原創) 如何破解ModelSim 6.1f? (IC Design) (ModelSim)
Abstract本文介紹如何破解ModelSim 6.1f. Introduction Step 1: 直行setup.exe安裝ModelSim. Step 2: 選擇Full Product St ...
- (原創) 如何讀取/寫入文字檔? (IC Design) (Verilog)
Abstract Verilog雖然為硬體描述語言,亦提供讀取/寫入文字檔的功能. Introduction為什麼需要用Verilog讀取/寫入文字檔呢?主要用在寫Testbench,並且有兩個優點: ...
- (原創) 如何將CMOS所擷取的影像傳到PC端? (SOC) (DE2) (TRDB-DC2)
AbstractDE2提供了Control Panel與Image Converter,可以將CMOS所擷取的影像傳到PC端, Introduction 版權聲明:文中所有範例皆出自DE2光碟,版權歸 ...
- (原創) Verilog入門書推薦2:數位系統實習 Quartus II (SOC) (Verilog)
Abstract 之前曾經推薦過一本Verilog的薄書,這次再推薦一本適合FPGA與Quartus II的Verilog入門書籍. Intrduction 作者:陸自強 出版社:儒林圖書公司 語言: ...
- (原創) 如何使用ModelSim-Altera對Nios II仿真? (SOC) (Nios II) (SOPC Builder) (ModelSim) (DE2)...
Abstract 在剛學習Nios II時,每次在Run As Nios II Hardware下方,看到Run As Nios II ModelSim就覺得很好奇,Nios II明明是嵌入式系統,怎 ...
- (原創) 如何解決DE2_LCM_CCD上下顛倒左右相反與無法設定曝光值的問題? (SOC) (DE2)...
AbstractDE2_LCM_CCD是友晶科技為DE2和其130萬像素CMOS與彩色LCD所寫的範例,但官方的範例會造成上下顛倒左右相反與曝光值無法設定的問題,本文提出解決方式. Introduct ...
- (原創) 如何將DE2_70_TV範例加上Sobel Edge Detector? (SOC) (Verilog) (Image Processing) (DE2-70)...
Abstract 本文將DE2-70平台的DE2_70_TV的範例加上Sobel Edge Detector. Introduction 使用環境:Quartus II 8.0 + DE2-70 (C ...
- (原創) ThinkPad X61安裝過程全紀錄 (NB) (ThinkPad) (X61)
Abstract 我的ThinkPad X61安裝過程詳細紀錄. Introduction Step 1: ThinkPad X61基本硬體 (原創) 如何自行在ThinkPad X61安裝Windo ...
- (原創) 如何使用SignalTap II觀察reg與wire值? (SOC) (Verilog) (Quartus II) (SignalTap II)
Abstract 撰寫Verilog時,雖然每個module都會先用ModelSim或Quartus II自帶的simulator仿真過,但真的將每個module合併時,一些不可預期的『run-tim ...
最新文章
- ajax invoke error,配置全局的异常捕获时,走ajax请求下面报错
- python自学笔记(三)python基本数据类型之列表list
- 关于模型复杂度的一个想法
- Hive记录-配置远程连接(JAVA/beeline)
- WebView 加载javaScript
- java 同步块 抛出异常_java问题合集(一)
- 分布式事务seate-server的docker镜像的制作与部署
- 7天拿到阿里安卓岗位offer,附高频面试题合集
- 计算机组成原理写一个运算器,计算机组成原理运算器的实现实验报告.doc
- Java堆分配参数总结
- malloc分配的内存空间是连续的吗
- 利用vs 分析DMP文件、pdb文件定位release下的异常崩溃
- java怎么缓存数据_java中的缓存技术该如何实现
- mosek安装及出现的问题解决方法
- 计算机丢失mscvr,教大家计算机丢失Msvcr100.dll的解决方法
- 教师心理压力测试软件,关注教师心理健康——教师版心理测评软件
- Java资深开发:这不得40k起步呀
- Windows 11如何使用IE浏览器
- Java生成指定范围的随机数
- 码云(gitee)的使用